创建如下的目录结构:
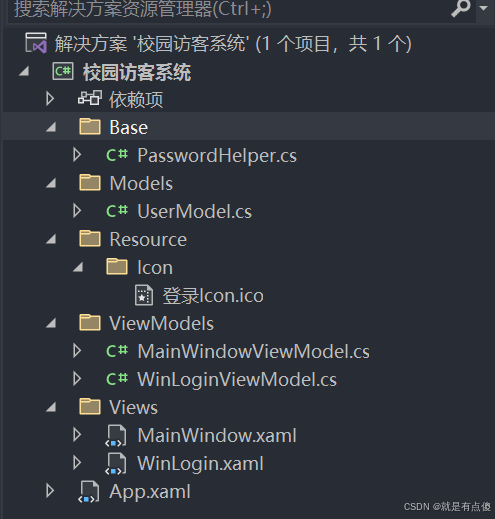
2.在App.xaml.cs中设置为先登录验证之后再进入主页面
cs
using Prism.Ioc;
using System.Windows;
using 校园访客系统.Views;
namespace 校园访客系统
{
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class App
{
protected override Window CreateShell()
{
return Container.Resolve<MainWindow>();
}
protected override void InitializeShell(Window shell)
{
if (Container.Resolve<Views.WinLogin>().ShowDialog() == true)
shell.ShowDialog();
Application.Current.Shutdown();
}
protected override void RegisterTypes(IContainerRegistry containerRegistry)
{
}
}
}
3.设计登录页面然后写对应的登录逻辑
WinLogin.XAML如下:
XML
<Window x:Class="校园访客系统.Views.WinLogin"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:校园访客系统.Views"
xmlns:prism="http://prismlibrary.com/"
prism:ViewModelLocator.AutoWireViewModel="True"
mc:Ignorable="d"
Title="账号登录"
Height="300"
Width="240"
xmlns:base="clr-namespace:校园访客系统.Base"
WindowStartupLocation="CenterScreen"
ResizeMode="NoResize"
FontFamily="Microsoft YaHei"
FontWeight="ExtraLight"
FontSize="12"
Icon="\Resource\Icon\登录Icon.ico">
<Window.Resources>
<Style TargetType="TextBox"
x:Key="UsernameTextBoxStyle">
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="{x:Type TextBox}">
<Border x:Name="border"
BorderBrush="#DDD"
BorderThickness="0,0,0,1"
Background="{TemplateBinding Background}"
SnapsToDevicePixels="True">
<Grid>
<TextBlock Text="账号/手机号/邮箱"
Grid.Column="1"
VerticalAlignment="Center"
Foreground="#DDD"
Name="markText"
Visibility="Collapsed"
FontSize="12"
Margin="2,0" />
<ScrollViewer x:Name="PART_ContentHost"
Focusable="false"
HorizontalScrollBarVisibility="Hidden"
VerticalScrollBarVisibility="Hidden"
Grid.Column="1"
VerticalAlignment="Center"
MinHeight="20" />
</Grid>
</Border>
<ControlTemplate.Triggers>
<Trigger Property="IsMouseOver"
Value="true">
<Setter Property="BorderBrush"
TargetName="border"
Value="#0b3d90" />
</Trigger>
<Trigger Property="IsKeyboardFocused"
Value="true">
<Setter Property="BorderBrush"
TargetName="border"
Value="#0b3d90" />
</Trigger>
<DataTrigger Binding="{Binding Path=Text,RelativeSource={RelativeSource Mode=Self}}"
Value="">
<Setter Property="Visibility"
TargetName="markText"
Value="Visible" />
</DataTrigger>
</ControlTemplate.Triggers>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
<Style x:Key="PasswordBoxStyle"
TargetType="{x:Type PasswordBox}">
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="{x:Type PasswordBox}">
<Border x:Name="border"
BorderBrush="#DDD"
BorderThickness="0,0,0,1"
Background="{TemplateBinding Background}"
SnapsToDevicePixels="True">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition />
<ColumnDefinition Width="20" />
</Grid.ColumnDefinitions>
<TextBlock Text="请输入密码"
VerticalAlignment="Center"
Foreground="#DDD"
Visibility="Collapsed"
Name="markText" />
<!--用来显示密文 * -->
<ScrollViewer x:Name="PART_ContentHost"
Focusable="false"
HorizontalScrollBarVisibility="Hidden"
VerticalScrollBarVisibility="Hidden"
VerticalAlignment="Center"
MinHeight="20" />
<!--用来显示是明文-->
<TextBox Text="{Binding UserInfo.Password,UpdateSourceTrigger=PropertyChanged}"
VerticalAlignment="Center"
BorderThickness="0"
Name="textBox"
Visibility="Collapsed"
Background="Transparent" />
<!--眼睛的样式修改,修改此控件的模板-->
<CheckBox Grid.Column="1"
VerticalAlignment="Center"
HorizontalAlignment="Center"
Name="checkBox" />
</Grid>
</Border>
<ControlTemplate.Triggers>
<Trigger Property="IsMouseOver"
Value="true">
<Setter Property="BorderBrush"
TargetName="border"
Value="#0b3d90" />
</Trigger>
<Trigger Property="IsKeyboardFocused"
Value="true">
<Setter Property="BorderBrush"
TargetName="border"
Value="#0b3d90" />
</Trigger>
<DataTrigger Binding="{Binding Path=UserInfo.Password}"
Value="">
<Setter Property="Visibility"
TargetName="markText"
Value="Visible" />
</DataTrigger>
<DataTrigger Binding="{Binding ElementName=checkBox,Path=IsChecked}"
Value="True">
<Setter TargetName="textBox"
Property="Visibility"
Value="Visible" />
<Setter TargetName="PART_ContentHost"
Property="Visibility"
Value="Collapsed" />
</DataTrigger>
</ControlTemplate.Triggers>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
<ControlTemplate TargetType="Button"
x:Key="LoginButtonTemplate">
<Grid>
<Border CornerRadius="3"
Background="DarkOrange"
Name="border">
<ContentPresenter VerticalAlignment="{TemplateBinding VerticalContentAlignment}"
HorizontalAlignment="{TemplateBinding HorizontalContentAlignment}" />
</Border>
</Grid>
<ControlTemplate.Triggers>
<Trigger Property="IsMouseOver"
Value="True">
<Setter Property="Background"
Value="orange"
TargetName="border" />
</Trigger>
<Trigger Property="IsPressed"
Value="True">
<Setter Property="Background"
Value="#99FF0F00"
TargetName="border" />
</Trigger>
</ControlTemplate.Triggers>
</ControlTemplate>
</Window.Resources>
<Grid>
<Grid Grid.Column="1"
VerticalAlignment="Center"
Margin="30,0,30,20">
<Grid.RowDefinitions>
<RowDefinition />
<RowDefinition />
<RowDefinition />
<RowDefinition />
<RowDefinition />
<RowDefinition Height="auto" />
<RowDefinition Height="auto" />
</Grid.RowDefinitions>
<TextBlock Text="用户登录"
Foreground="#0b3d90"
FontSize="13"
Margin="0,10"
FontWeight="Normal" />
<TextBlock Text="账号"
Grid.Row="1"
Foreground="#CC0b3d90"
Margin="0,10,0,5"
FontWeight="Normal" />
<TextBox Grid.Row="2"
Height="26"
Style="{StaticResource UsernameTextBoxStyle}"
Text="{Binding UserInfo.UserName,UpdateSourceTrigger=PropertyChanged}" />
<TextBlock Text="密码"
Grid.Row="3"
Foreground="#CC0b3d90"
Margin="0,10,0,5"
FontWeight="Normal" />
<PasswordBox Grid.Row="4"
Height="26"
Style="{StaticResource PasswordBoxStyle}"
Password=""
base:PasswordHelper.Attach="1"
base:PasswordHelper.Password="{Binding UserInfo.Password,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}" />
<Button Content="登 录"
Grid.Row="5"
Foreground="White"
Height="28"
Margin="0,10,0,5"
Template="{StaticResource LoginButtonTemplate}"
FontWeight="Normal"
Command="{Binding LoginCommand}"
CommandParameter="{Binding RelativeSource={RelativeSource AncestorType=Window}}" />
<CheckBox Content="记住密码"
Foreground="#BBB"
VerticalAlignment="Center"
HorizontalAlignment="Left"
VerticalContentAlignment="Center"
FontSize="11"
Grid.Row="6" />
<TextBlock HorizontalAlignment="Right"
VerticalAlignment="Center"
Grid.Row="6">
<Hyperlink>找回密码</Hyperlink>
</TextBlock>
</Grid>
<TextBlock Text="{Binding ErrorMessage}"
VerticalAlignment="Bottom"
HorizontalAlignment="Center"
TextAlignment="Center"
FontSize="11"
Foreground="Red"
TextWrapping="Wrap"
Margin="30,0,30,10" />
</Grid>
</Window>
配合WinLogin.XAML取得密码的PasswordHelper类如下:
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Controls;
using System.Windows;
namespace 校园访客系统.Base
{
public class PasswordHelper
{
// 依赖附加属性
public static readonly DependencyProperty PasswordProperty = DependencyProperty.RegisterAttached("Password", typeof(string), typeof(PasswordHelper),
new PropertyMetadata(new PropertyChangedCallback(OnPropertyChanged)));
// 包装方法
public static string GetPassword(DependencyObject d)
{
return (string)d.GetValue(PasswordProperty);
}
public static void SetPassword(DependencyObject d, string value)
{
d.SetValue(PasswordProperty, value);
}
public static readonly DependencyProperty AttachProperty = DependencyProperty.RegisterAttached("Attach", typeof(string), typeof(PasswordHelper),
new PropertyMetadata(new PropertyChangedCallback(OnAttachChanged)));
public static string GetAttach(DependencyObject d)
{
return (string)d.GetValue(AttachProperty);
}
public static void SetAttach(DependencyObject d, string value)
{
d.SetValue(AttachProperty, value);
}
static bool _isUpdating = false;
private static void OnPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
PasswordBox pb = (d as PasswordBox);
pb.PasswordChanged -= Pb_PasswordChanged;
if (!_isUpdating)
pb.Password = e.NewValue.ToString();
pb.PasswordChanged += Pb_PasswordChanged;
}
private static void OnAttachChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
PasswordBox pb = (d as PasswordBox);
pb.PasswordChanged += Pb_PasswordChanged;
}
private static void Pb_PasswordChanged(object sender, RoutedEventArgs e)
{
PasswordBox pb = (sender as PasswordBox);
_isUpdating = true;
SetPassword(pb, pb.Password);
_isUpdating = false;
}
}
}
UserModel如下:
cs
using Prism.Mvvm;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 校园访客系统.Models
{
public class UserModel : BindableBase
{
private string _userName;
public string UserName
{
get { return _userName; }
set
{
SetProperty(ref _userName, value);
System.Diagnostics.Debug.WriteLine(value);
}
}
private string _password = "";
public string Password
{
get { return _password; }
set
{
SetProperty(ref _password, value);
System.Diagnostics.Debug.WriteLine(value);
}
}
}
}
WinLoginViewModel如下:
cs
using Prism.Mvvm;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Input;
using System.Windows;
using 校园访客系统.Models;
using Prism.Commands;
namespace 校园访客系统.ViewModels
{
public class WinLoginViewModel : BindableBase
{
public WinLoginViewModel()
{
LoginCommand = new DelegateCommand<object>(DoLogin);
}
public UserModel UserInfo { get; set; }=new UserModel();
private string _errorMessage;
public string ErrorMessage
{
get { return _errorMessage; }
set { SetProperty(ref _errorMessage, value); }
}
private bool _isLoading;
public bool IsLoading
{
get { return _isLoading; }
set { SetProperty(ref _isLoading, value); }
}
private string _loadingMessage;
public string LoadingMessage
{
get { return _loadingMessage; }
set { SetProperty(ref _loadingMessage, value); }
}
public ICommand LoginCommand { get; set; }
private void DoLogin(object obj)
{
if (string.IsNullOrEmpty(UserInfo.UserName))
{
this.ErrorMessage = "请输入用户名";
return;
}
if (string.IsNullOrEmpty(UserInfo.Password))
{
this.ErrorMessage = "请输入密码";
return;
}
}
}
}
4.效果如下:
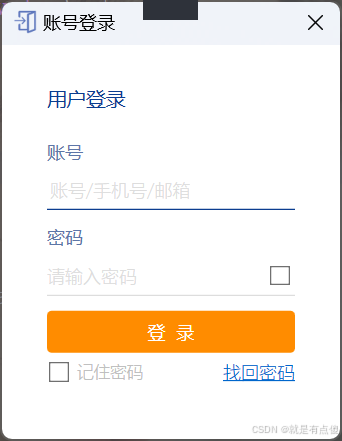
如果没有输入账号点击登录如下:
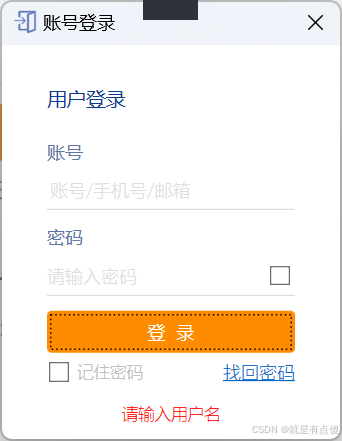
如果没有输入账号点击密码如下:
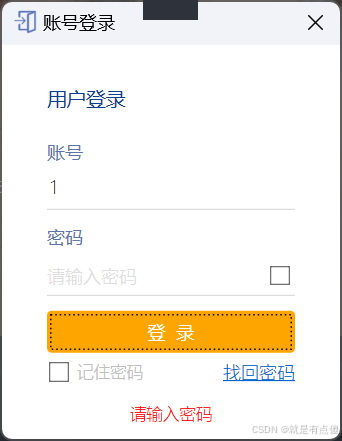
如果点击checkbox会显示明文密码:
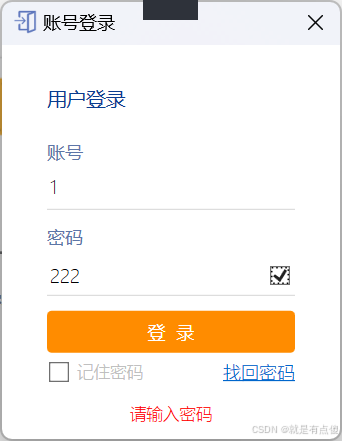