一、快速入门
准备
准备html页面,并引入Vue模块(官方提供)
创建Vue程序的应用实例
准备元素(div),被Vue控制
构建用户界面
准备数据
通过插值表达式渲染页面
在h1标签中的"{{ }}"就是插值表达式
mount("#app") :vue控制id属性值为app的内容
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<h1>{{msg}}</h1>
</div>
<!-- 引入vue模块 -->
<script type="module">
/* 引入 vue 的模块 按需引入createApp函数 */
import {createApp} from 'https://unpkg.com/vue@3/dist/vue.esm-browser.js';
/* 创建vue的应用实例 */
createApp({
data(){
return {
//定义数据
msg: "Hello vue3"
}
}
}).mount("#app")
</script>
</body>
</html>
二、常用指令
2.1 v-for
作用:列表渲染,遍历容器的元素或者对象的属性;基于数据循环,多次渲染整个元素
语法: v-for = "(item,index) in items"
items 为遍历的数组
item 为遍历出来的元素
index 为索引/下标,从0开始;可以省略,省略index语法: v-for = "item in intems"
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<table border="1" cellspacing="0">
<tr>
<th>文章标题</th>
<th>分类</th>
<th>发表时间</th>
<th>状态</th>
<th>操作</th>
</tr>
<!-- 那个元素要出现多次,v-for指令就添加到哪个元素上 -->
<tr v-for="(article, index) in articleList" :key="index">
<td>{{article.title}}</td>
<td>{{article.category}}</td>
<td>{{article.time}}</td>
<td>{{article.state}}</td>
<td>
<button>编辑</button>
<button>删除</button>
</td>
</tr>
</table>
</div>
<script type="module">
//导入vue模块
import { createApp } from 'https://unpkg.com/vue@3/dist/vue.esm-browser.js';
//创建应用实例
createApp({
data() {
return {
//定义数据
articleList: [
{
title: "医疗反腐绝非砍医护收入",
category: "时事",
time: "2023-09-05",
state: "已发布"
},
{
title: "中国男篮缘何一败涂地?",
category: "篮球",
time: "2023-09-05",
state: "草稿"
},
{
title: "华山景区已受大风影响阵风达7-8级,未来24小时将持续",
category: "旅游",
time: "2023-09-05",
state: "已发布"
}
]
}
}
}).mount("#app"); //控制页面元素
</script>
</body>
</html>
2.2 v-bind
作用:动态为HTML标签绑定属性值,如设置href , src , style 样式等
语法:v-bind:属性名="属性值"
简化: :属性名="属性值"
注意:v-bind所绑定的数据,必须在data中定义定义
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<!-- <a v-bind:href="url">黑马官网</a> -->
<a :href="url">黑马官网</a>
</div>
<script type="module">
//引入vue模块
import { createApp} from
'https://unpkg.com/vue@3/dist/vue.esm-browser.js'
//创建vue应用实例
createApp({
data() {
return {
url: "https://www.itheima.com"
}
}
}).mount("#app")//控制html元素
</script>
</body>
</html>
2.3 v-if & v-show
作用:这俩类指令,都是用来控制元素的显示和隐藏的
v-if
语法:v-if="表达式",表达式值为 true,显示;false,隐藏
其它:可以配合 v-else-if / v-else 进行链式调用条件判断
原理:基于条件判断,来控制创建或移除元素节点(条件渲染)
场景:要么显示,要么不显示,不频繁切换的场景
v-show
语法:v-show="表达式",表达式值为 true,显示;false,隐藏
原理:基于CSS样式display来控制显示与隐藏
场景:频繁切换显示隐藏的场景
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
手链价格为: <span v-if="customer.level>=0 && customer.level<=1">9.9</span>
<span v-else-if="customer.level>1 && customer.level<=2">19.9</span>
<span v-else>29.9</span>
<br/>
<hr/>
手链价格为: <span v-show="customer.level>=0 && customer.level<=1">9.9</span>
<span v-show="customer.level>1 && customer.level<=2">19.9</span>
<span v-show="customer.level>3">29.9</span>
</div>
<script type="module">
//导入vue模块
import { createApp} from 'https://unpkg.com/vue@3/dist/vue.esm-browser.js'
//创建vue应用实例
createApp({
data() {
return {
customer:{
name:"张三",
level:6
}
}
}
}).mount("#app")//控制html元素
</script>
</body>
</html>
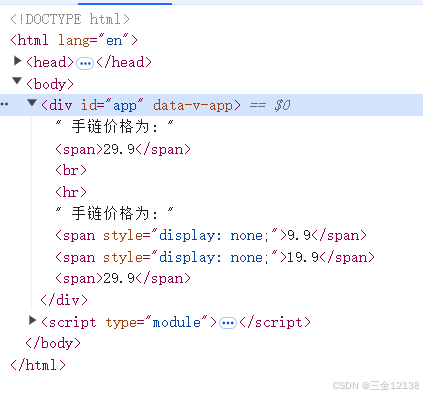
2.4 v-on
作用:为html标签绑定事件
语法:
v-on:事件名="函数名"
简写为:@事件名="函数名"
函数需要定义在methods选项内部
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<button v-on:click="moeny">点我有惊喜</button>
<button @click="love">再点更惊喜</button>
</div>
<script type="module">
//导入vue模块
import { createApp } from 'https://unpkg.com/vue@3/dist/vue.esm-browser.js'
//创建vue应用实例
createApp({
data() {
return {
//定义数据
}
},
methods: {
//定义方法
moeny: function () {
alert("恭喜你,获得1000元优惠券")
},
love: function () {
alert("爱你一万年")
}
}
}).mount("#app");//控制html元素
</script>
</body>
</html>
2.5 v-model
作用:在表单元素上使用,双向数据绑定 。可以方便的 获取 或者 设置表单项数据
语法: v-model="变量名"
注意:v-model中绑定的变量,必须在data中定义
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
文章分类: <input type="text" v-model="searchConditions.category"/> <span>{{searchConditions.category}}</span>
发布状态: <input type="text" v-model="searchConditions.state"/> <span>{{searchConditions.state}}</span>
<button>搜索</button>
<button v-on:click="clear">重置</button>
<br />
<br />
<table border="1 solid" colspa="0" cellspacing="0">
<tr>
<th>文章标题</th>
<th>分类</th>
<th>发表时间</th>
<th>状态</th>
<th>操作</th>
</tr>
<tr v-for="(article,index) in articleList">
<td>{{article.title}}</td>
<td>{{article.category}}</td>
<td>{{article.time}}</td>
<td>{{article.state}}</td>
<td>
<button>编辑</button>
<button>删除</button>
</td>
</tr>
</table>
</div>
<script type="module">
//导入vue模块
import { createApp } from 'https://unpkg.com/vue@3/dist/vue.esm-browser.js'
//创建vue应用实例
createApp({
data() {
return {
//定义数据
searchConditions:{
category: "",
state: ""
},
articleList: [{
title: "医疗反腐绝非砍医护收入",
category: "时事",
time: "2023-09-5",
state: "已发布"
},
{
title: "中国男篮缘何一败涂地?",
category: "篮球",
time: "2023-09-5",
state: "草稿"
},
{
title: "华山景区已受大风影响阵风达7-8级,未来24小时将持续",
category: "旅游",
time: "2023-09-5",
state: "已发布"
}]
}
}
,
methods:{
clear:function(){
//清空category 和 state的数据
//在methods对应的方法里面,使用this就代表的是vue实例,可以使用this获取到vue实例中准备的数据
this.serchConditions.catagory = "";
this.serchConditions.state = "";
}
}
,
mounted(){
console.log("Vue挂载完毕,放松请求获取数据")
}
}).mount("#app")//控制html元素
</script>
</body>
</html>
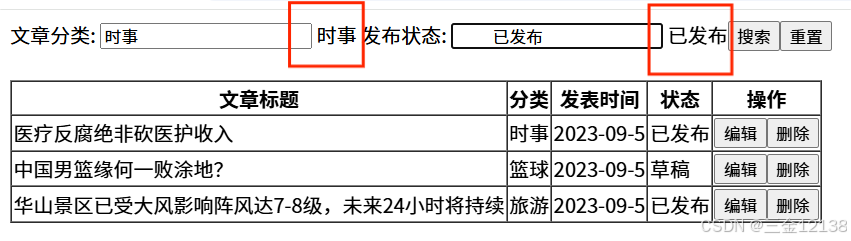
三、生命周期
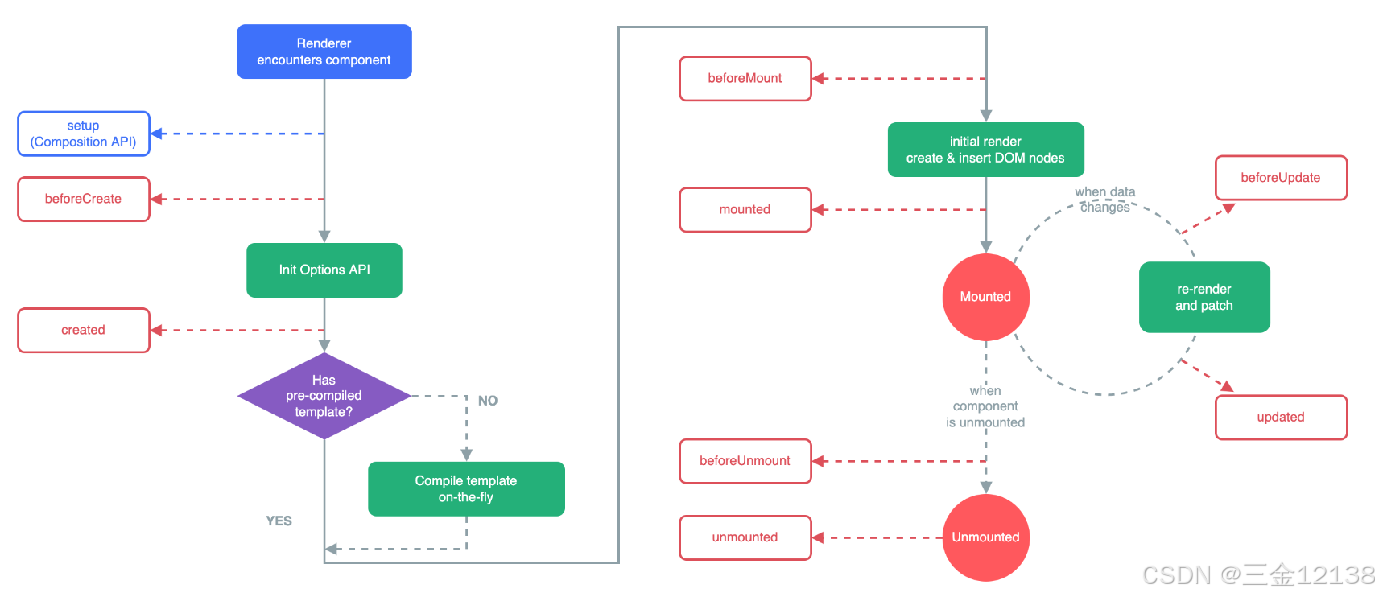
生命周期的八个阶段:每个阶段都会自动执行一个生命周期方法(钩子),让开发者有机会在特定的阶段执行自己的代码
四、Axios
Axios
介绍:Axios对原生的Ajax进行了封装,简化书写,快速开发
Axios使用步骤:
- 引入Axios的js文件(参照官网)
2.引入Axios发送请求,并获取相应结果
html
<!-- 引入axios的js文件 -->
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
method:请求方法,比如GET/POST
url:请求路径
data:请求的数据
html
axios({
method:"get",
url:"http://localhost:8080/article/getAll"
}).then(result =>{
//成功的回调
//result代表服务器响应的所有数据,包含了响应头,响应体,result.date 代表的的是接口响应的核心数据
console.log(result.data);
}).catch(err=>{
//失败的回调
console.log(err);
});
Axios-请求方式别名
为了方便起见,Axios已经为所有支持的请求方法提供了别名
格式: axios.请求方式(url[,data[,config]])
GET: axios.get(url).then((result) => {...}).catch(err => {...})
POST: axios.post(url,data).then((result) => {...}).catch(err => {...})
html
//别名的方式发送请求
axios.get("http://localhost:8080/article/getAll").then(result =>{
console.log(result.data);
}).catch(err =>{
console.log(err);
});
html
//别名的方式发送请求
axios.post("http://localhost:8080/article/add",article).then(result =>{
console.log(result.data);
}).catch(err =>{
console.log(err);
});
上述这种axios的写法推荐
综合案例:
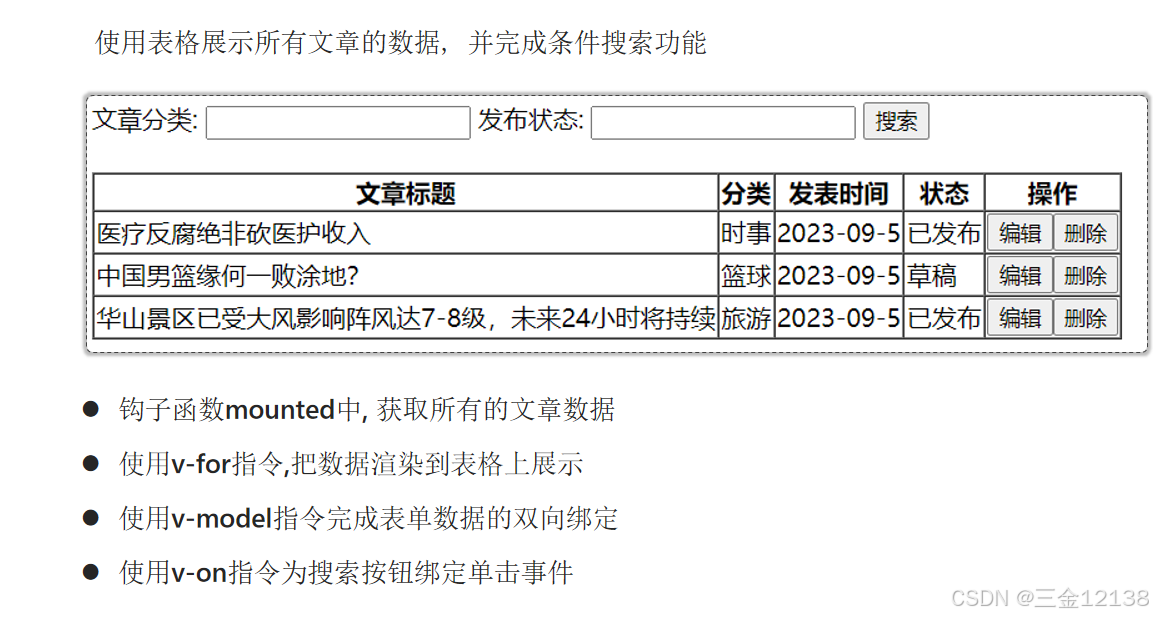
后端主要代码:
java
@RestController
@RequestMapping("/article")
@CrossOrigin//支持跨域
public class ArticleController {
private List<Article> articleList = new ArrayList<>();
{
articleList.add(new Article("医疗反腐绝非砍医护收入", "时事", "2023-09-5", "已发布"));
articleList.add(new Article("中国男篮缘何一败涂地", "篮球", "2023-09-5", "草稿"));
articleList.add(new Article("华山景区已受大风影响阵风达7-8级", "旅游", "2023-09-5", "已发布"));
}
//新增文章
@PostMapping("/add")
public String add(@RequestBody Article article) {
articleList.add(article);
return "新增成功";
}
//获取所有文章信息
@GetMapping("/getAll")
public List<Article> getAll(HttpServletResponse response) {
return articleList;
}
//根据文章分类和发布状态搜索
@GetMapping("/search")
public List<Article> search(String category, String state) {
return articleList.stream().filter(a -> a.getCategory().equals(category) && a.getState().equals(state)).collect(Collectors.toList());
}
}
java
@Data
@NoArgsConstructor
@AllArgsConstructor
public class Article {
private String title;
private String category;
private String time;
private String state;
}
前端代码:
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
文章分类: <input type="text" v-model="searchConditions.category">
发布状态: <input type="text" v-model="searchConditions.state">
<button @click="search">搜索</button>
<br />
<br />
<table border="1 solid" colspa="0" cellspacing="0">
<tr>
<th>文章标题</th>
<th>分类</th>
<th>发表时间</th>
<th>状态</th>
<th>操作</th>
</tr>
<tr v-for="(article,index) in articleList">
<td>{{article.title}}</td>
<td>{{article.category}}</td>
<td>{{article.time}}</td>
<td>{{article.state}}</td>
<td>
<button>编辑</button>
<button>删除</button>
</td>
</table>
</div>
<!-- 导入axios的文件 -->
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script type="module">
//导入vue模块
import {createApp} from 'https://unpkg.com/vue@3/dist/vue.esm-browser.js';
//创建vue应用示例
createApp({
data(){
return{
articleList:[],
searchConditions:{
category:"",
state:""
}
}
},
methods:{
search:function(){
//发送请求,完成搜索,携带搜索条件(字符串的拼接)
axios.get("http://localhost:8080/article/search?category=" + this.searchConditions.category
+ "&state=" +this.searchConditions.state)
.then(result => {
//成功回调, result.data
//把得到的数据赋值给article
this.articleList = result.data;
}).catch(err => {
//失败回调
console.log(err);
})
}
},
//钩子函数mounted中,获取所有的文章数据
mounted:function(){
axios.get("http://localhost:8080/article/getAll")
.then(result => {
//成功回调
this.articleList = result.data;
}).catch(err => {
//失败回调
console.log(err);
})
}
}).mount("#app");//控制html元素
</script>
</body>
</html>
就此局部使用vue的你就学完啦!