python获取zabbix图形
通过zabbix的api接口获取指定时间段的监控项图形
图片示例:
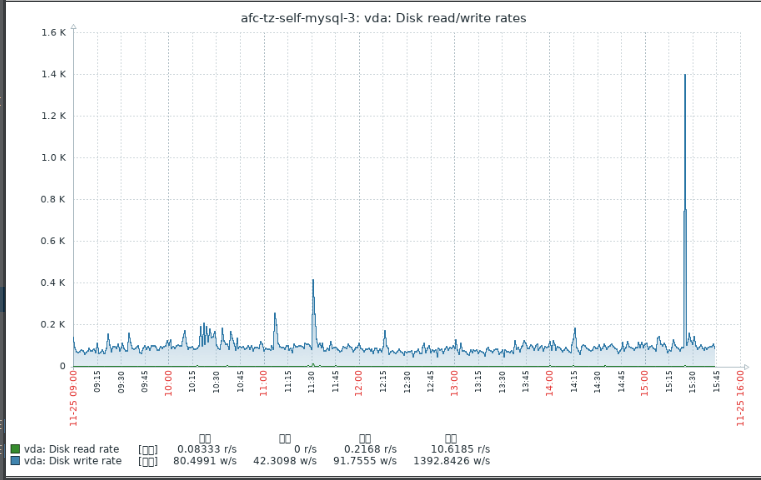
代码示例:
# -*- coding: UTF-8 -*-
#可根据监控项获取zabbix下所有主机的Itemid,
#通过zabbix库的graphs_items表 ,获取graphid
#本文只通过graphid直接获取图形
import requests
import datetime
# 配置 Zabbix API 相关参数
ZABBIX_API_URL = "http://xxxxxxxx/zabbix/api_jsonrpc.php"
ZABBIX_USER = "xxxxx"
ZABBIX_PASSWORD = "xxxxx"
# 手动登录 Zabbix 获取会话 cookie
def zabbix_manual_login():
session = requests.Session()
login_url = ZABBIX_API_URL.replace("/api_jsonrpc.php", "/index.php")
login_payload = {
"name": ZABBIX_USER,
"password": ZABBIX_PASSWORD,
"autologin": 1,
"enter": "Sign in",
}
response = session.post(login_url, data=login_payload)
if "zbx_sessionid" not in session.cookies:
raise Exception("登录失败,请检查用户名和密码。")
return session
# 获取 Zabbix 图形图片
def get_graph_image(session, graphid, start_time, end_time, output_file):
# 构建图形 URL
graph_url = ZABBIX_API_URL.replace("/api_jsonrpc.php", "/chart2.php")
params = {
"graphid": graphid,
"from": start_time,
"to" : end_time,
"width": 800, # 可调整图形宽度
"height": 400, # 可调整图形高度
"profileIdx": "web.graphs"
}
# 请求图片
response = session.get(graph_url, params=params, stream=True)
if response.headers.get("Content-Type") != "image/png":
print("获取图形失败,返回内容为:")
print(response.text)
raise Exception("未能正确获取图形图片,请检查参数或权限。")
# print(response.text)
# 保存图片到本地
with open(output_file, 'wb') as f:
for chunk in response.iter_content(chunk_size=8192):
f.write(chunk)
print(f"图形已保存到 {output_file}")
# 将时间转换为 Zabbix 的 Unix 时间戳格式
def convert_to_stime(dt):
return dt.strftime("%Y%m%d%H%M%S")
if __name__ == "__main__":
try:
# 手动登录获取会话
session = zabbix_manual_login()
print("登录成功,已获取会话 cookie。")
# 指定 graphid
graphid = "xxxxx"
# 设置时间范围(每天的 9 点到 16 点)
today = datetime.date.today()
start_time = datetime.datetime(today.year, today.month, today.day, 9, 0, 0)
end_time = datetime.datetime(today.year, today.month, today.day, 16, 0, 0)
# 保存图片的文件路径
output_file = f"zabbix_graph_{graphid}.png"
# 获取图形图片
get_graph_image(session, graphid, start_time, end_time, output_file)
except Exception as e:
print("发生错误:", str(e))