知识点目标
1、基本的查询
查询所有字段
查询部分字段
字段起别名
And
Or
Between
IN
2、模糊查询
like
通配符
3、分组查询
4、聚合函数
5、表之间关系
6、外键约束
7、连接查询
8、子查询
基本的查询
查询所有字段
JAVA
select * from 表;
* 代表所有字段
查询部分字段
JAVA
select 列名,列名 from 表;
字段起别名
JAVA
select 列名 as 别名 from 表;
-- 查询字段起别名
select id as 编号,name as 名称,price as 价格,address as 产地 from product;
select id 编号,name 名称,price 价格,address 产地 from product;
多条件设置
多个条件可以使用and和or连接
and 两个条件同时成立
or 两个条件成立一个
JAVA
-- 查询产地为武汉或者广州的商品
select * from product where address = '武汉' or address = '广州';
-- 查询产地为武汉并且价格在200到500之间的商品
select * from product where address = '武汉' and price >= 200 and price <= 500;
IN关键字
一个字段的值在列表(多个值)中存在
JAVA
where 字段 in (值,值...)
Between关键字
表示字段值在两个值之间
JAVA
where 字段 between 最小值 and 最大值;
JAVA
-- 使用in关键字
select * from product where address in ('武汉','广州');
-- 使用between关键字
select * from product where address = '武汉' and price between 5000 and 8000;
模糊查询
非精确的查询,通过通配符查询到想要结果
JAVA
where 字段 like '字符串+统配符'
通配符
% 匹配任意长度的字符串
_ 匹配一个字符
JAVA
-- 小米开头
select * from product where name like '小米%';
-- 名字带米
select * from product where name like '%米%';
分组查询
查询时需要按照某些字段将数据分类,进行汇总
按字段分组
JAVA
select 聚合函数或分组字段 from 表 group by 字段;
聚合函数:
- count 计数 count(*) 字段有空值也统计 count(字段) 字段有空值不统计
- sum 求和
- avg 求平均值
- max 最大值
- min 最小值
JAVA
-- 每种商品的数量
select count(*) 数量,type 类型 from product group by type;
-- 每个产地的商品总价,平均价格,最高,最低价格
select address 产地,count(price) 数量,sum(price) 总价,avg(price) 平均价,max(price) 最高,min(price) 最低价 from product group by address;
多列分组
先按一个字段分组,再按第二个字段分组...
JAVA
group by 字段,字段;
-- 多列分组
select count(*) 数量,type 类型,address 产地 from product group by type,address;
having关键字
在分组之后进行条件筛选
where -----> group by -----> having
JAVA
-- 分组之后进行筛选
-- 商品数量超过2个的类型
select count(*) 数量,type 类型 from product group by type having count(*) > 2;
-- 产品总价在20000以上的产地
select sum(price) 总价,address 产地 from product group by address having sum(price) > 20000;
查询排序
按字段升序或降序排列
JAVA
order by 字段 asc或不写代表升序 desc代表降序
如果有where写在where条件后面
-- 多列排序
select * from product order by price asc,id desc;
分页查询
limit关键字作用是用于限制返回的记录行数
写sql语句的最后
JAVA
limit n 返回第一行到第n行的数据
limit m,n 返回从第m行向后n行的数据,行从0开始
-- 最便宜的三种商品
select * from product order by price limit 3;
-- 最贵的一种商品
select * from product order by price desc limit 1;
-- 最便宜的三到五位商品
select * from product order by price limit 2,3;
-- 分页查询
select * from product limit 0,5;
select * from product limit 5,5;
select * from product limit 10,5;
select * from product limit 15,5;
select * from product limit 20,5;
外键约束
MySQl属于关系型数据库管理系统,表和表之间有关系,把不同的数据存储到不同表中
外键约束实现引用完整性,从表的数据必须在主表中存在
如:订单表(订单id、商品id、订购数量、订购时间)要保证插入订单的商品id必须存在
外键约束的效果:
1)插入数据,先主后从
2)删除数据,先从后主
语法:
JAVA
创建表的时候创建外键
create table 表名(
字段 类型,
constraint 约束名 foreign key(外键列名) references 主表(主键)
);
create table t_order(
order_id int primary key auto_increment,
product_id int not null,
order_num int not null,
order_time datetime,
constraint fk_product_id foreign key(product_id) references product(id)
);
创建表之后创建外键
alter table 表名
add constraint 约束名 foreigin key(外键列名) references 主表(主键)
删除外键
alter table 表名 drop constraint 约束名
-- 删除约束
alter table t_order drop FOREIGN key fk_product_id;
-- 添加外键约束
alter table t_order add constraint fk_product_id foreign key(product_id) references product(id);
联合查询
将不同表的数据拼接到一起
JAVA
select 语句
union
select 语句
select * from goods
union
select * from product;
union 会自动合并完全相同的数据
union all 不会合并数据
select * from goods
union all
select * from product;
表之间的关系
关系型数据表之间的关系有:
1)一对一,人和身份证
给从表设置外键引用主表,给外键设置唯一约束
2)一对多,部门和员工、订单和商品、班级和学员
给从表设置外键引用主表
3)多对多,学生和课程
引入中间表,在中间表中设置外键分别引用两个主表
表的设计:理出表和表之间的关系,用图形的方式展示出来
E-R图 (Entity-Relationship 实体关系图)
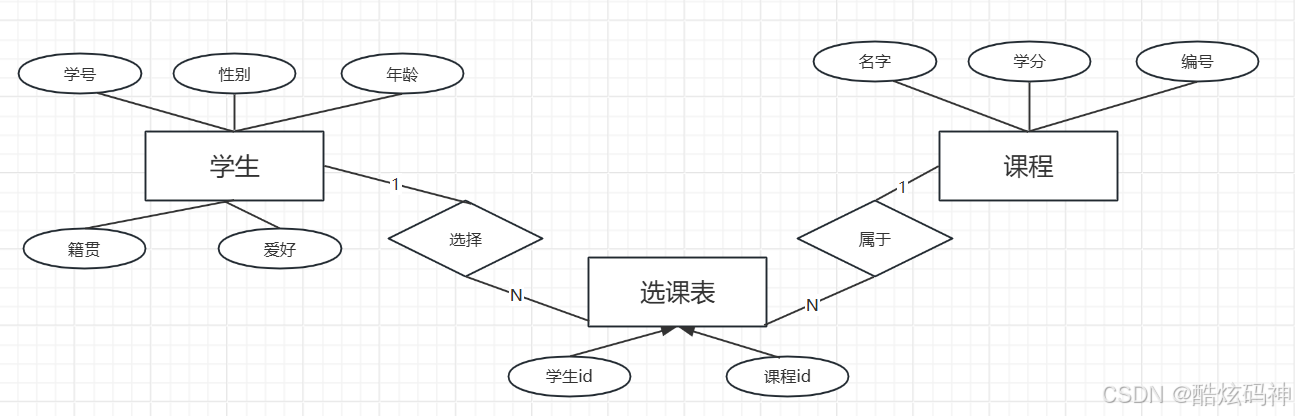
数据库范式:对数据库的设计进行规范
范式1:数据库表的字段不可再分
范式2:数据库表的字段都和主键相关,课程表:课程id、名字、学分、老师姓名、老师电话、老师QQ、食堂阿姨的电话。阿姨电话和课程没有任何关系,老师电话和课程有间接关系
范式3:数据库表的字段和主键直接相关,课程表:课程id、名字、学分、老师姓名、老师电话、老师QQ,老师的信息属于传递依赖,不符合第三范式
课程表:课程id、名字、学分、老师id
老师表:老师id、老师姓名、老师电话、老师QQ
数据库的设计一般符合第三范式
连接查询
将多张表的数据,连接起来一起查询
分为:
1、内连接
查询出两张表相关的数据(交集)
2、外连接
左外连接
包含所有左表的数据,以及相关的数据,不相关数据会显示null
右外连接
包含所有右表的数据,以及相关的数据,不相关数据会显示null
3、笛卡尔积
两个表的数据相乘,不设置连接条件
内连接
查询两张表都相关的数据
语法:
JAVA
select 两张表的字段 from 主表
[inner] join 从表 on 主表.主键 = 从表.外键;
-- 内连接查询订单和商品信息
select o.order_id 订单号,p.name 商品名称,p.price 商品价格,o.order_num 数量,o.order_time 购买时间
from t_order o inner join product p
on o.product_id = p.id;
select o.order_id 订单号,p.name 商品名称,p.price 商品价格,o.order_num 数量,o.order_time 购买时间
from t_order o , product p
where o.product_id = p.id;
外连接
左外连接,显示所有左表数据,右表不相关数据会显示空值
JAVA
select 两张表的字段 from 左表
left join 右表 on 主表.主键 = 从表.外键;
JAVA
-- 左外连接
select o.order_id 订单号,p.name 商品名称,p.price 商品价格,o.order_num 数量,o.order_time 购买时间
from product p left join t_order o
on o.product_id = p.id;
-- 查询没买过的商品
select o.order_id 订单号,p.name 商品名称,p.price 商品价格,o.order_num 数量,o.order_time 购买时间
from product p left join t_order o
on o.product_id = p.id where o.order_num is null;
-- 显示已卖出的商品的总销量
-- 1) 连接查询
-- 2) 按商品名称分组
-- 3)sum汇总购买数量
select p.name 商品名称,sum(o.order_num) 总销量
from product p join t_order o on p.id = o.product_id
group by p.name;
多表连接
JAVA
-- 创建用户表
create table t_user(
id int primary key auto_increment,
username varchar(20) not null,
password varchar(20) not null,
qq varchar(20) ,
phone varchar(20)
);
-- 创建商品表
create table t_product
(
id int primary key auto_increment,
name varchar(20) not null,
price float not null,
address varchar(20)
);
-- 创建订单表
create table t_order(
id int primary key auto_increment,
user_id int not null,
product_id int not null,
order_num int not null,
order_time datetime
);
insert into t_user(username,password,qq,phone) values('张三','123','82181821','15663321182'),('李四','123','5454543','15773321182');
insert into t_product(name,price,address) values('戴尔电脑',3000,'武汉'),('华为电脑',4000,'深圳'),('联想电脑',5000,'武汉');
insert into t_order(user_id,product_id,order_num,order_time)
values(1,1,1,'2024-11-11'),(2,2,1,'2024-11-11'),(1,3,1,'2024-12-12'),(2,2,1,'2024-12-12');
-- 显示用户姓名、电话、商品名称、价格、购买日期、数量
select u.username 用户姓名,u.phone 电话,p.name 商品名称,p.price 价格,o.order_time 购买日期,o.order_num 数量
from t_user u join t_order o on o.user_id = u.id join t_product p on o.product_id = p.id;
子查询
可以在查询语句内部嵌套查询语句
JAVA
select * from 表 where 字段 = (select 字段 from 表 where 条件);
先执行子查询再执行父查询
问题1:查找和戴尔电脑买的一样贵的电脑
JAVA
-- 查询戴尔电脑的价格
-- 再把价格作为条件,进行查询
select * from t_product where price = (select price from t_product where name = '戴尔电脑') and name != '戴尔电脑';
问题2:查找3000到5000之间的电脑
JAVA
select * from t_product where price between 3000 and 5000 and name like '%电脑%';
select * from (select * from t_product where price between 3000 and 5000) p where p.name like '%电脑%';
作业
创建学生表(id、姓名、年龄、性别、地址)、课程表(id、课程名、课时)、分数表(id、学生id、课程id、分数、考试时间)