目录
11、元素定位隐形等待
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('http:/jd.com/')
time.sleep(2)
#元素隐性等待(多少秒内找到元素就立刻执行,没找到元素就报错)
#因为有些页面渲染太慢了,你操作某个元素时还没有渲染出来,所以会报错,建议加上隐性等待的时间
a1.implicitly_wait(10)
a1.find_element(By.XPATH,'//*[@id="feedContent0"]/li[1]/a/div[4]').click()
12、单选、多选、下拉、评星、日期
https://bahuyun.com/bdp/form/1335521405670260737
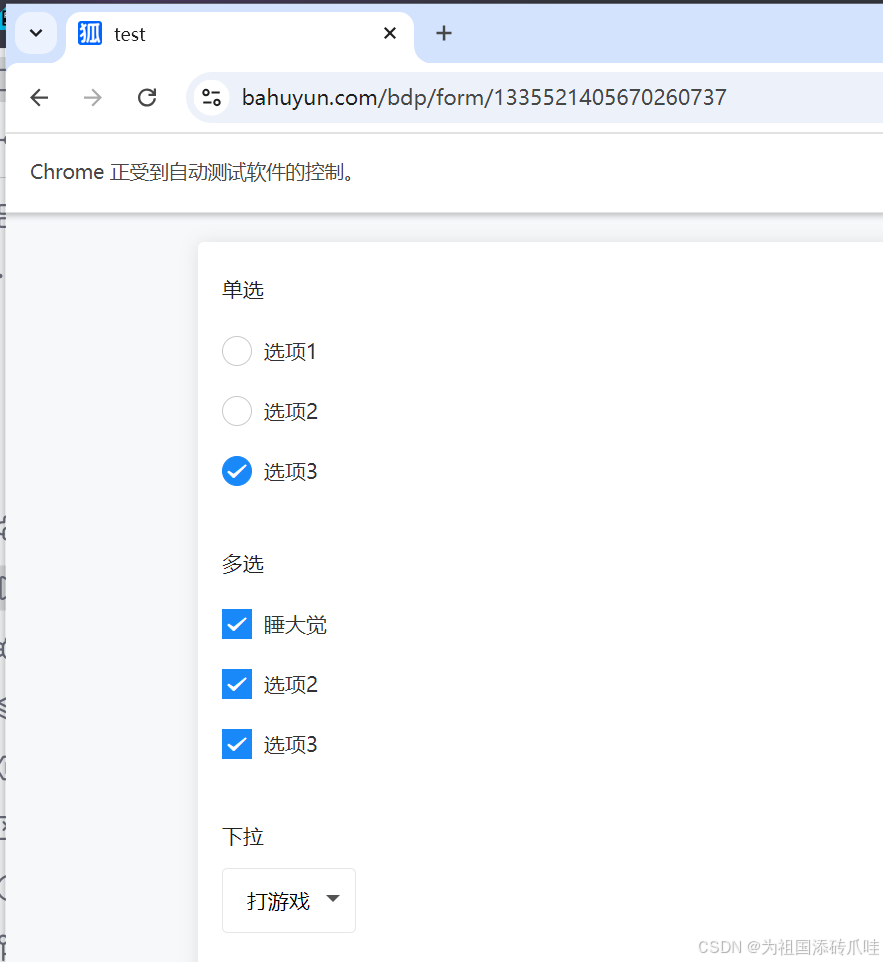
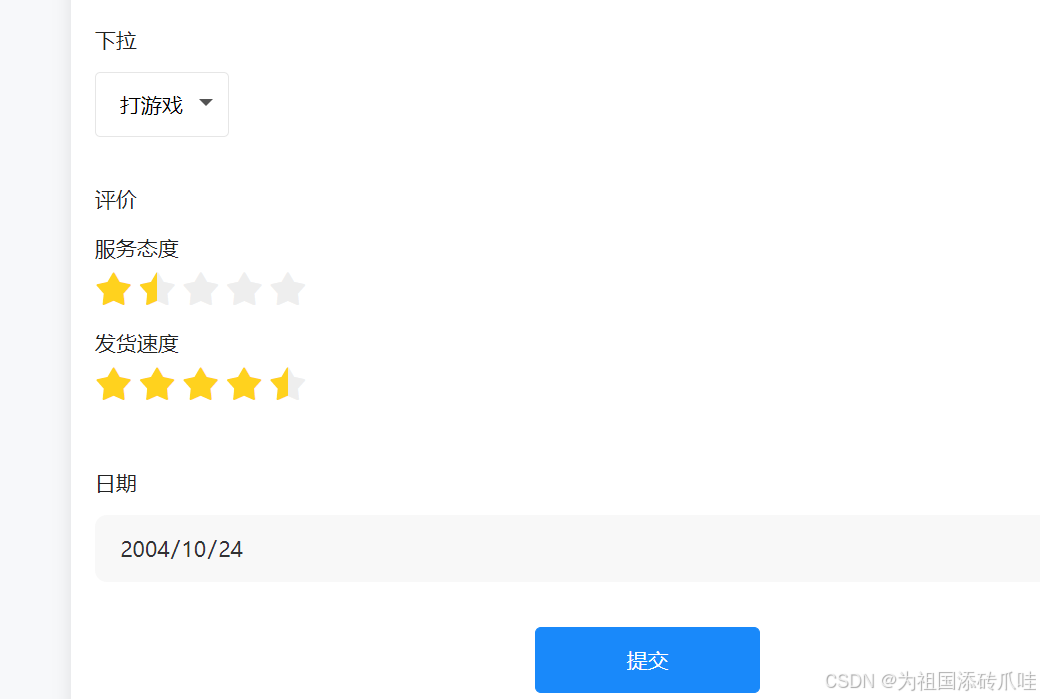
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://bahuyun.com/bdp/form/1335521405670260737')
time.sleep(2)
#操作单选框
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[1]').click()
time.sleep(2)
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[2]').click()
time.sleep(2)
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[3]').click()
#操作多选框
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[1]/span').click()
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[2]/span').click()
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[3]/span').click()
time.sleep(2)
#操作下拉元素
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[3]/div/div[2]/div/div/div/select/option[2]').click()
#操作评星
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[4]/div/div[2]/div/div[1]/div[2]/div[2]/i[1]').click()
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[4]/div/div[2]/div/div[2]/div[2]/div[5]/i[1]').click()
#操作日期
a1.find_element(By.XPATH,'//*[@id="input--W2vbG04d963X3l_Xyz_w"]').send_keys("0020031024")
13、获取句柄、切换标签页
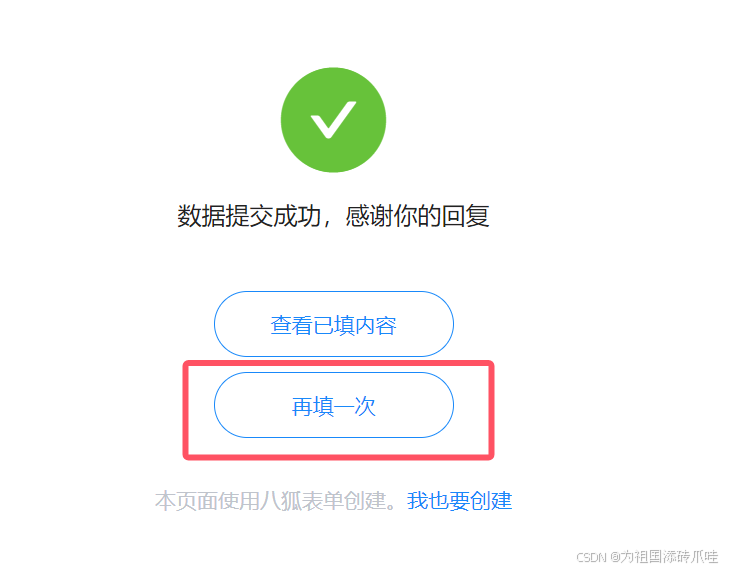
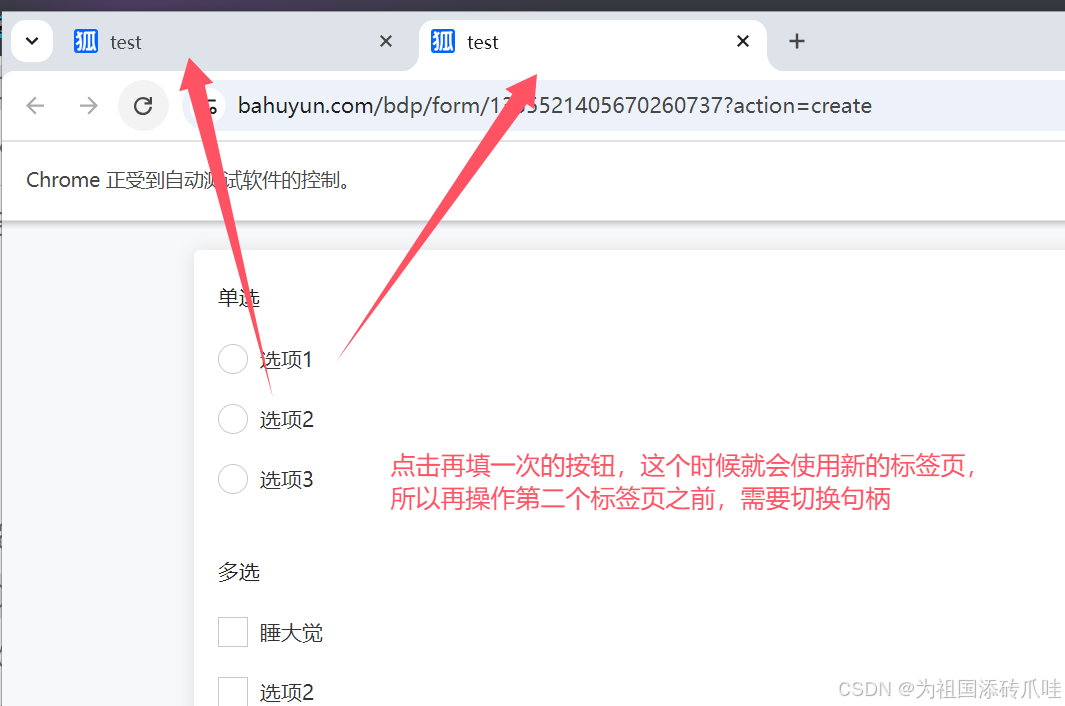
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://bahuyun.com/bdp/form/1335521405670260737')
time.sleep(2)
for x in range(2):
#操作单选框
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[1]').click()
time.sleep(2)
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[2]').click()
time.sleep(2)
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[3]').click()
#操作多选框
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[1]/span').click()
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[2]/span').click()
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[3]/span').click()
time.sleep(2)
#操作下拉元素
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[3]/div/div[2]/div/div/div/select/option[2]').click()
#操作评星
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[4]/div/div[2]/div/div[1]/div[2]/div[2]/i[1]').click()
a1.find_element(By.XPATH,'//*[@id="my-node"]/div[4]/div/div[2]/div/div[2]/div[2]/div[5]/i[1]').click()
#操作日期
a1.find_element(By.XPATH,'//*[@id="input--W2vbG04d963X3l_Xyz_w"]').send_keys("0020031024")
#提交表单
a1.find_element(By.XPATH,'//*[@id="submit-button"]').click()
time.sleep(3)
#点击再填一次
a1.find_element(By.XPATH,'//*[@id="app"]/div/div/div[1]/div[2]/div[3]/button').click()
time.sleep(2)
#获取全部标签页句柄
a2=a1.window_handles
print(a2)
#通过句柄切换标签页
a1.switch_to.window(a2[1])
#获取当前标签页句柄
a2=a1.current_window_handle
print(f'当前标签页的句柄:{a2}')
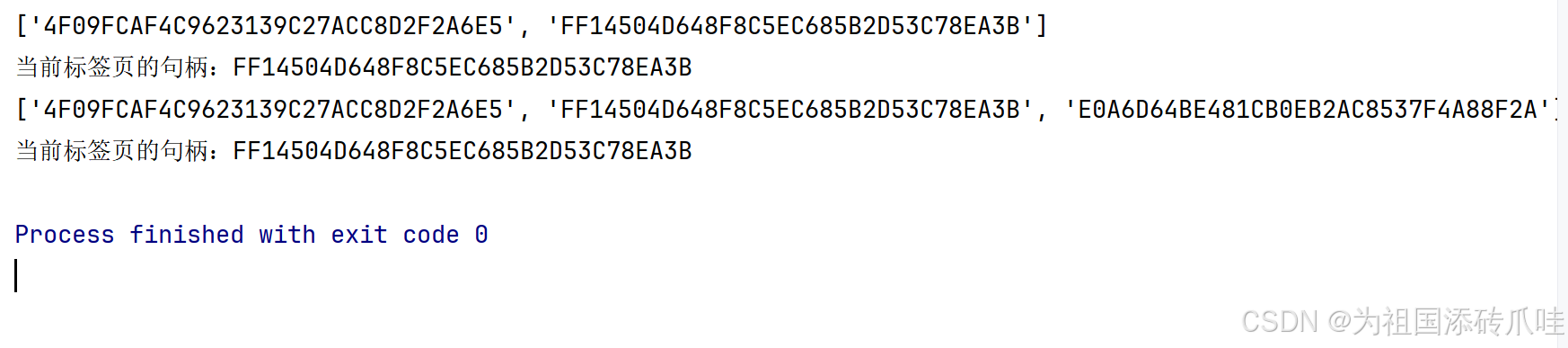
14、多线程执行自动化(四开浏览器)
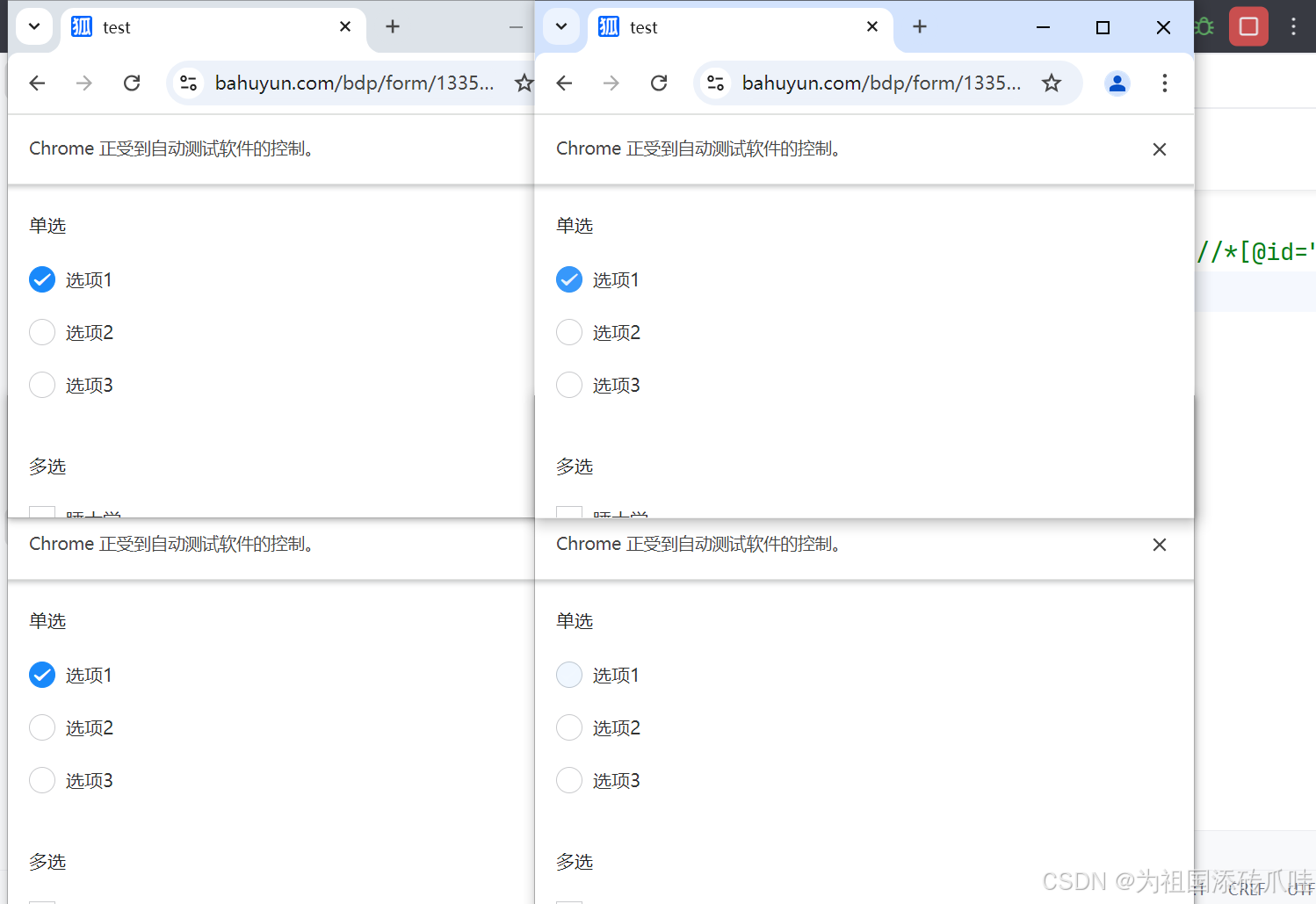
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
import threading
#多线程执行自动化------四开浏览
class A:
def __init__(self,x,y):
self.x=x
self.y=y
# 设置浏览器、启动浏览器
def func1(self):
q1 = Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach', True)
a1 = webdriver.Chrome(service=Service('chromedriver.exe'), options=q1)
a1.set_window_position(self.x,self.y)
a1.set_window_size(200,400)
a1.implicitly_wait(30)
a1.get('https://bahuyun.com/bdp/form/1335521405670260737')
return a1
#执行代码
def func2(self):
a1=self.func1()
for x in range(3):
time.sleep(2)
for x in range(2):
# 操作单选框
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[1]').click()
time.sleep(2)
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[2]').click()
time.sleep(2)
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[1]/div/div[2]/div/div/div[3]').click()
# 操作多选框
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[1]/span').click()
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[2]/span').click()
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[2]/div/div[2]/div/div/div[3]/span').click()
time.sleep(2)
# 操作下拉元素
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[3]/div/div[2]/div/div/div/select/option[2]').click()
# 操作评星
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[4]/div/div[2]/div/div[1]/div[2]/div[2]/i[1]').click()
a1.find_element(By.XPATH, '//*[@id="my-node"]/div[4]/div/div[2]/div/div[2]/div[2]/div[5]/i[1]').click()
# 操作日期
a1.find_element(By.XPATH, '//*[@id="input--W2vbG04d963X3l_Xyz_w"]').send_keys("0020031024")
# 提交表单
a1.find_element(By.XPATH, '//*[@id="submit-button"]').click()
time.sleep(3)
# 点击再填一次
a1.find_element(By.XPATH, '//*[@id="app"]/div/div/div[1]/div[2]/div[3]/button').click()
s1=A(0,0)
s2=A(400,0)
s3=A(0,300)
s4=A(400,300)
threading.Thread(target=s1.func2).start()
threading.Thread(target=s2.func2).start()
threading.Thread(target=s3.func2).start()
threading.Thread(target=s4.func2).start()
15、警告框(alert)元素交互
https://sahitest.com/demo/alertTest.htm
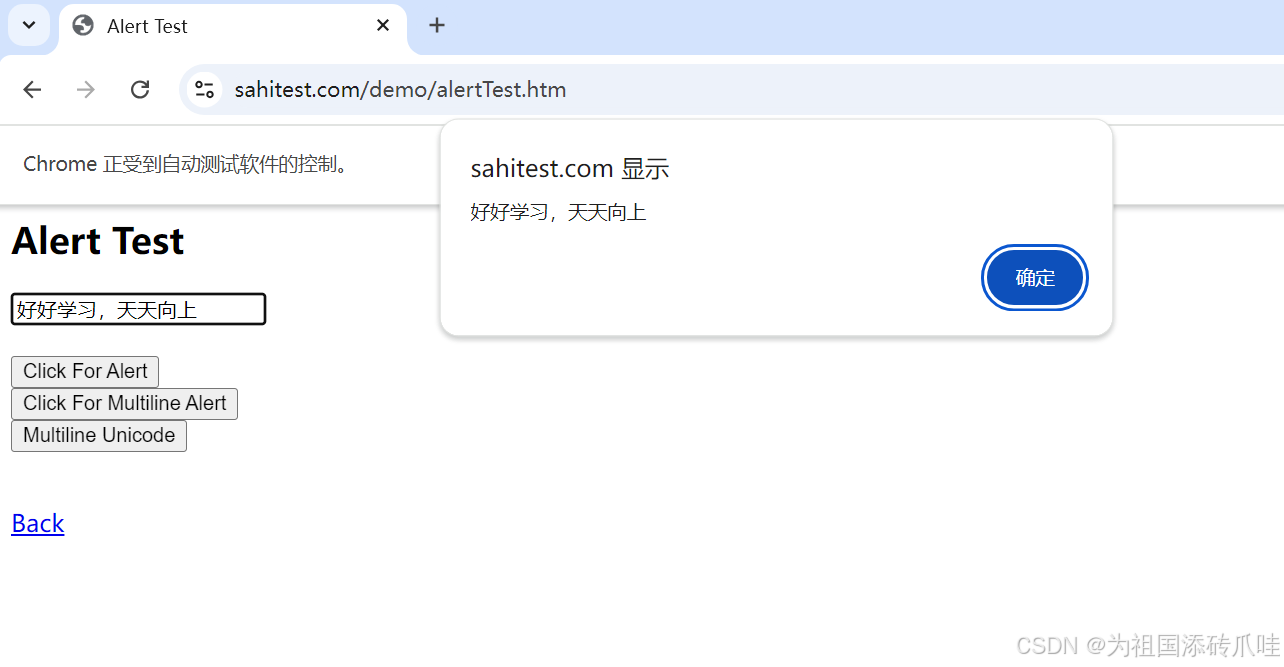
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://sahitest.com/demo/alertTest.htm')
a1.find_element(By.XPATH,'/html/body/form/input[1]').clear()
time.sleep(2)
a1.find_element(By.XPATH,'/html/body/form/input[1]').send_keys("好好学习,天天向上")
time.sleep(2)
a1.find_element(By.XPATH,'/html/body/form/input[2]').click()
time.sleep(3)
#获取弹窗内的文本内容
print(a1.switch_to.alert.text)
#点击弹窗确定按钮
a1.switch_to.alert.accept()
16、确认框(confirm)元素交互
https://sahitest.com/demo/confirmTest.htm
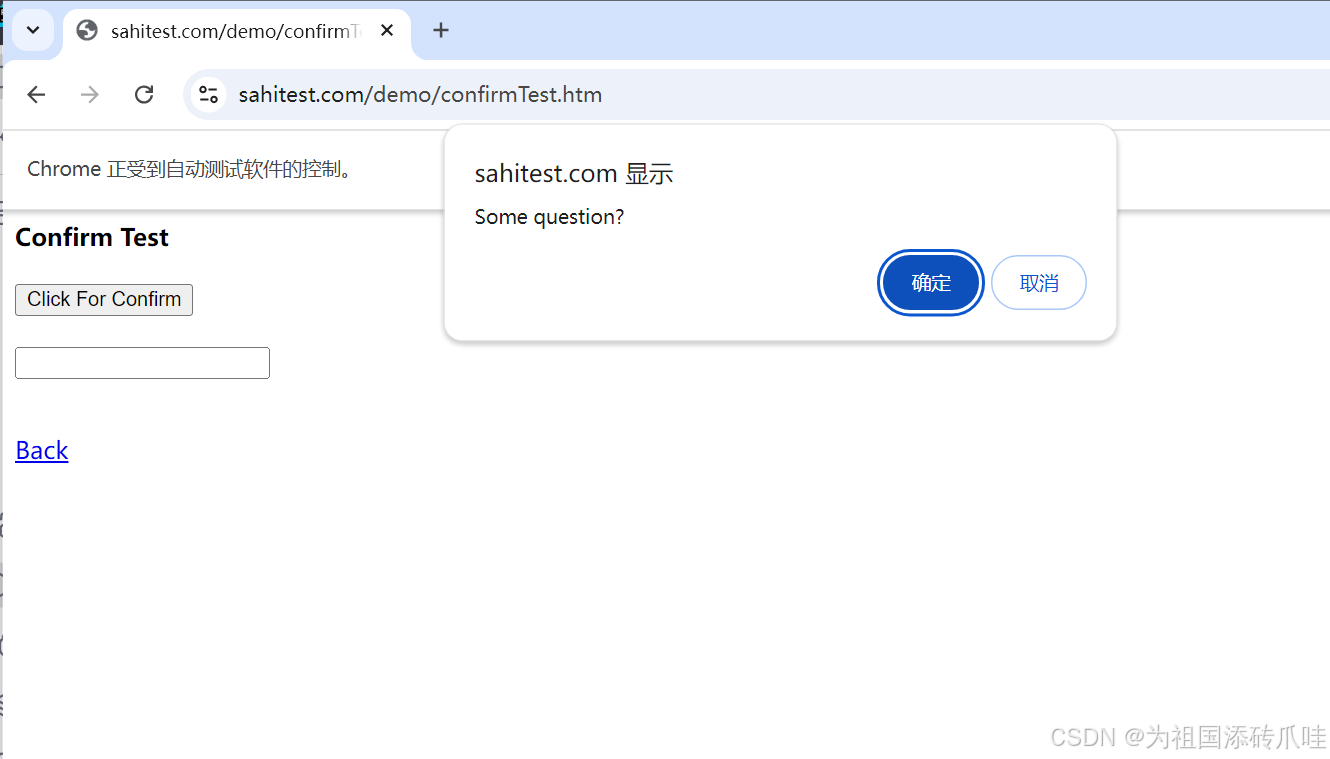
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://sahitest.com/demo/confirmTest.htm')
a1.find_element(By.XPATH,'/html/body/form/input[1]').click()
time.sleep(2)
#点击弹窗确定按钮
a1.switch_to.alert.accept()
time.sleep(2)
#点击弹窗取消按钮
#a1.switch_to.alert.dismiss()
17、提示框(prompt)元素交互
https://sahitest.com/demo/promptTest.htm
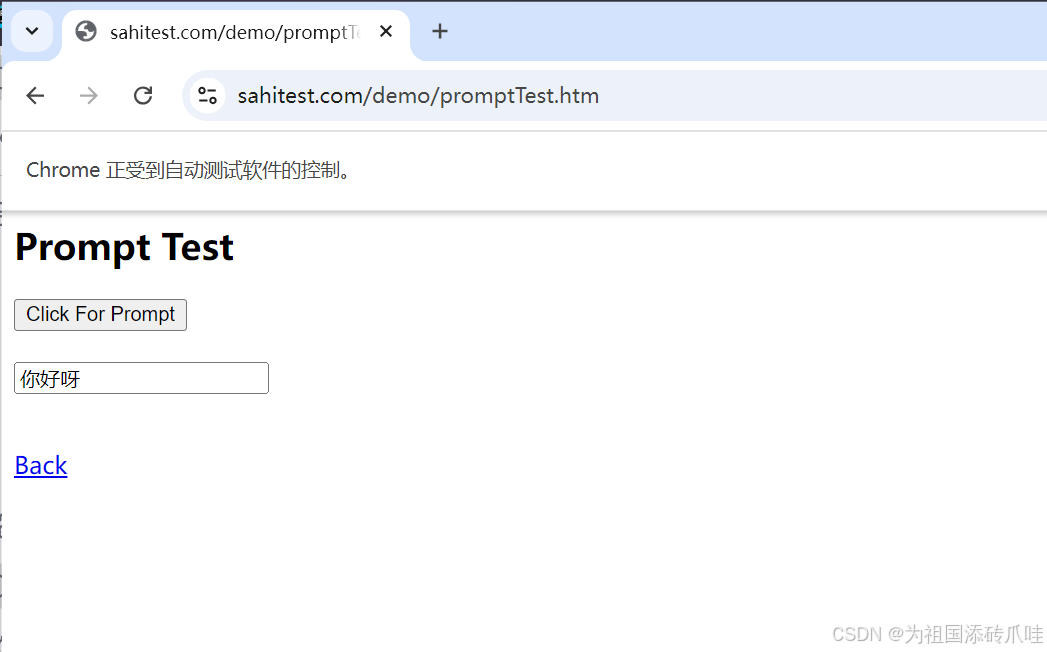
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://sahitest.com/demo/promptTest.htm')
a1.find_element(By.XPATH,'/html/body/form/input[1]').click()
time.sleep(2)
#弹窗输入内容
a1.switch_to.alert.send_keys('你好呀')
time.sleep(2)
#弹窗点击确定
a1.switch_to.alert.accept()
time.sleep(2)
18、iframe嵌套页面进入、退出
https://sahitest.com/demo/iframesTest.htm
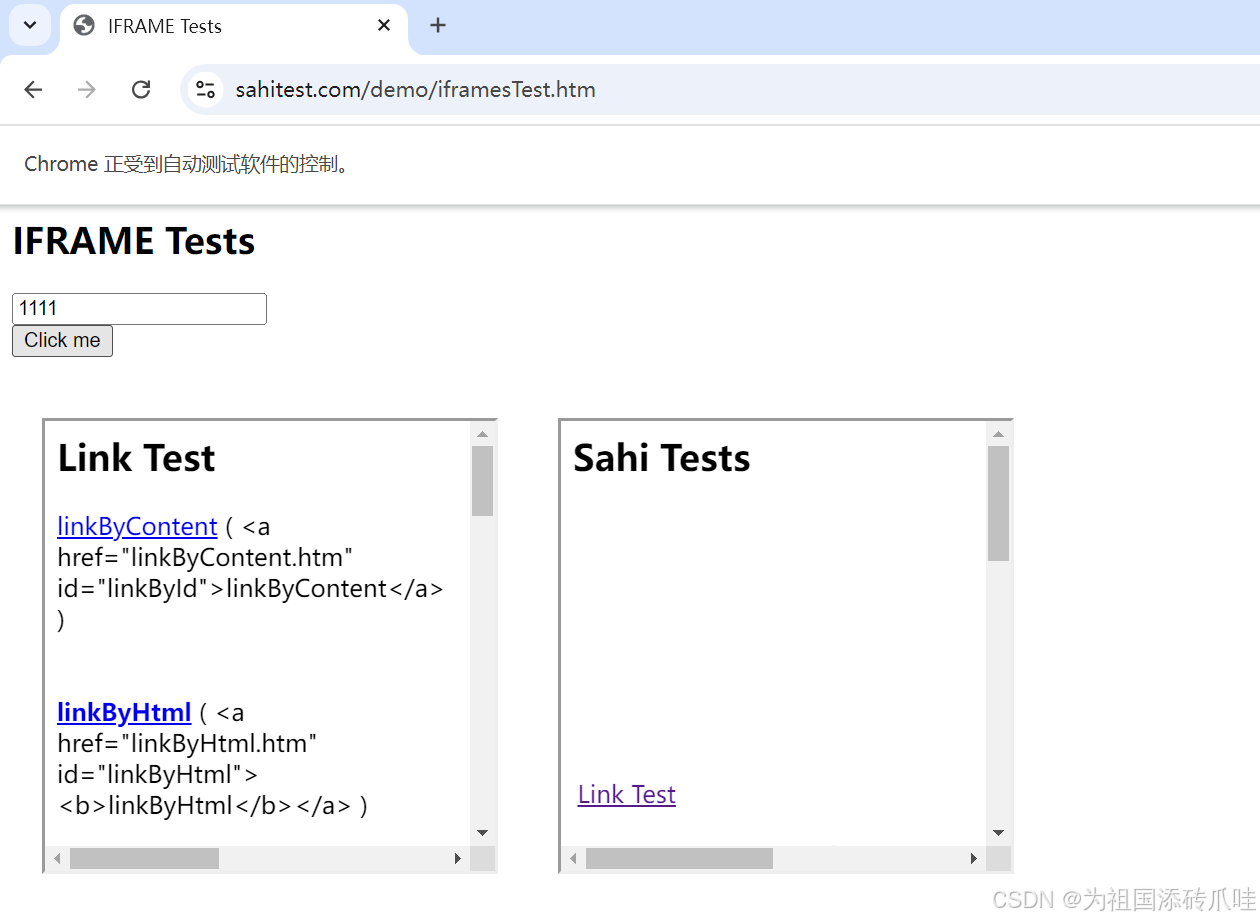
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://sahitest.com/demo/iframesTest.htm')
time.sleep(2)
#获取iframe元素
a2=a1.find_element(By.XPATH,'/html/body/iframe')
time.sleep(2)
#进入iframe嵌套页面
a1.switch_to.frame(a2)
time.sleep(2)
#进入iframe页面操作元素点击
a1.find_element(By.XPATH,'/html/body/table/tbody/tr/td[1]/a[1]').click()
#退出iframe嵌套页面(返回到默认页面)
a1.switch_to.default_content()
time.sleep(2)
a1.find_element(By.XPATH,'/html/body/input[2]').click()
19、获取元素文本内容、是否可见
https://h.xinhuaxmt.com/vh512/share/12294597?newstype=1001&homeshow=1

from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://h.xinhuaxmt.com/vh512/share/12294597?newstype=1001&homeshow=1')
time.sleep(2)
#获取元素文本内容 text
a2=a1.find_element(By.XPATH,'//*[@id="app"]/div[1]/div[3]/header/h1').text
print(a2)
#元素是否可见
a3=a1.find_element(By.XPATH,'//*[@id="app"]/div[1]/div[3]/div[2]/section/p[1]/span').is_displayed()
print(a3)
20、网页前进、后退
from selenium import webdriver #用于操作浏览器
from selenium.webdriver.chrome.options import Options #用于设置谷歌浏览器
from selenium.webdriver.chrome.service import Service #用于管理驱动
from selenium.webdriver.common.by import By
import time
#设置浏览器、启动浏览器
def func():
q1=Options()
q1.add_argument('--no-sandbox')
q1.add_experimental_option('detach',True)
a1=webdriver.Chrome(service=Service('chromedriver.exe'),options=q1)
return a1
a1=func()
#打开指定网址
a1.get('https://baidu.com')
a1.find_element(By.XPATH,'//*[@id="kw"]').send_keys('自动化测试')
time.sleep(2)
a1.find_element(By.XPATH,'//*[@id="su"]').click()
time.sleep(2)
#网页后退
a1.back()
time.sleep(2)
#网页前进
a1.forward()
time.sleep(2)