实现步骤:
1. 引入依赖
2. 编写基本的配置信息
3. 编写生产者代码
4. 编写消费者代码
引入依赖
如代码:
<!--Spring MVC相关依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!--RabbitMQ相关依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
在这里我们也可以直接在创建项目的直接引入。
如图:
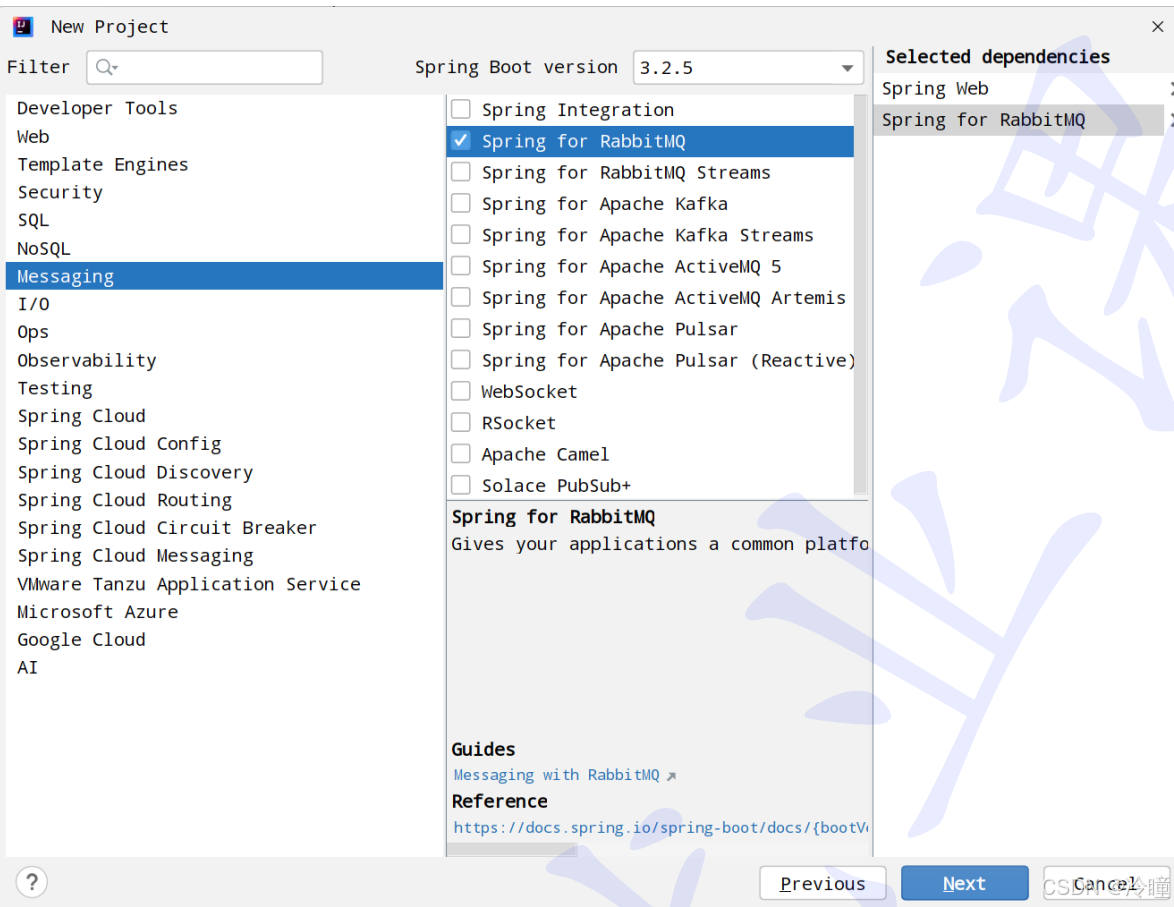
修改yml配置文件
如代码:
#配置RabbitMQ的基本信息
spring:
rabbitmq:
host: 110.41.51.65
port: 15673 #默认为5672
username: study
password: study
virtual-host: bite #默认值为 /
注意上面的host一定要改成自己云服务器的主机IP。
编写生产者代码
在常量类中声明队列模式。
如代码:
//work模式队列名称
public static final String WORK_QUEUE = "work_queue";
@Configuration
public class RabbitMQConfig {
//1. ⼯作模式队列
@Bean("workQueue")
public Queue workQueue() {
return QueueBuilder.durable(Constants.WORK_QUEUE).build();
}
}
编写生产者代码:
@RequestMapping("/producer")
@RestController
public class ProducerController {
@Autowired
private RabbitTemplate rabbitTemplate;
@RequestMapping("/work")
public String work(){
for (int i = 0; i < 10; i++) {
//使⽤内置交换机发送消息, routingKey和队列名称保持⼀致
rabbitTemplate.convertAndSend("", Constants.WORK_QUEUE, "hello
spring amqp: work...");
}
return "发送成功";
}
}
如上述代码所示,通过依赖注入的rabbitTemplate,通过这个对象我们可以直接调用,spring内部封装好的方法,直接发送消息。
编写消费者代码
如代码:
@Component
public class WorkListener {
@RabbitListener(queues = Constants.WORK_QUEUE)
public void listenerQueue(Message message){
System.out.println("listener 1["+Constants.WORK_QUEUE+"]收到消息:" +
message);
}
@RabbitListener(queues = Constants.WORK_QUEUE)
public void listenerQueue2(Message message){
System.out.println("listener 2["+Constants.WORK_QUEUE+"]收到消息:" +
message);
}
}
@RabbitListener 是Spring框架中用于监听RabbitMQ队列的注解,通过使用这个注解,可以定 义⼀个方法,以便从RabbitMQ队列中接收消息。该注解支持多种参数类型,这些参数类型代表了从 RabbitMQ接收到的消息和相关信息.