目录
0、定义
用原型实例指定创建对象的种类,并且通过复制这些原型创建新的对象。
1、原型模式的两种角色
- 抽象原型(Prototype):一个接口,负责定义对象复制自身方法。
- 具体原型(ConcretePrototype):实现Prototype的接口的类。具体原型 实现抽象原型中的方法,以便所创建的对象调用该方法复制自己。
2、原型模式的UML类图
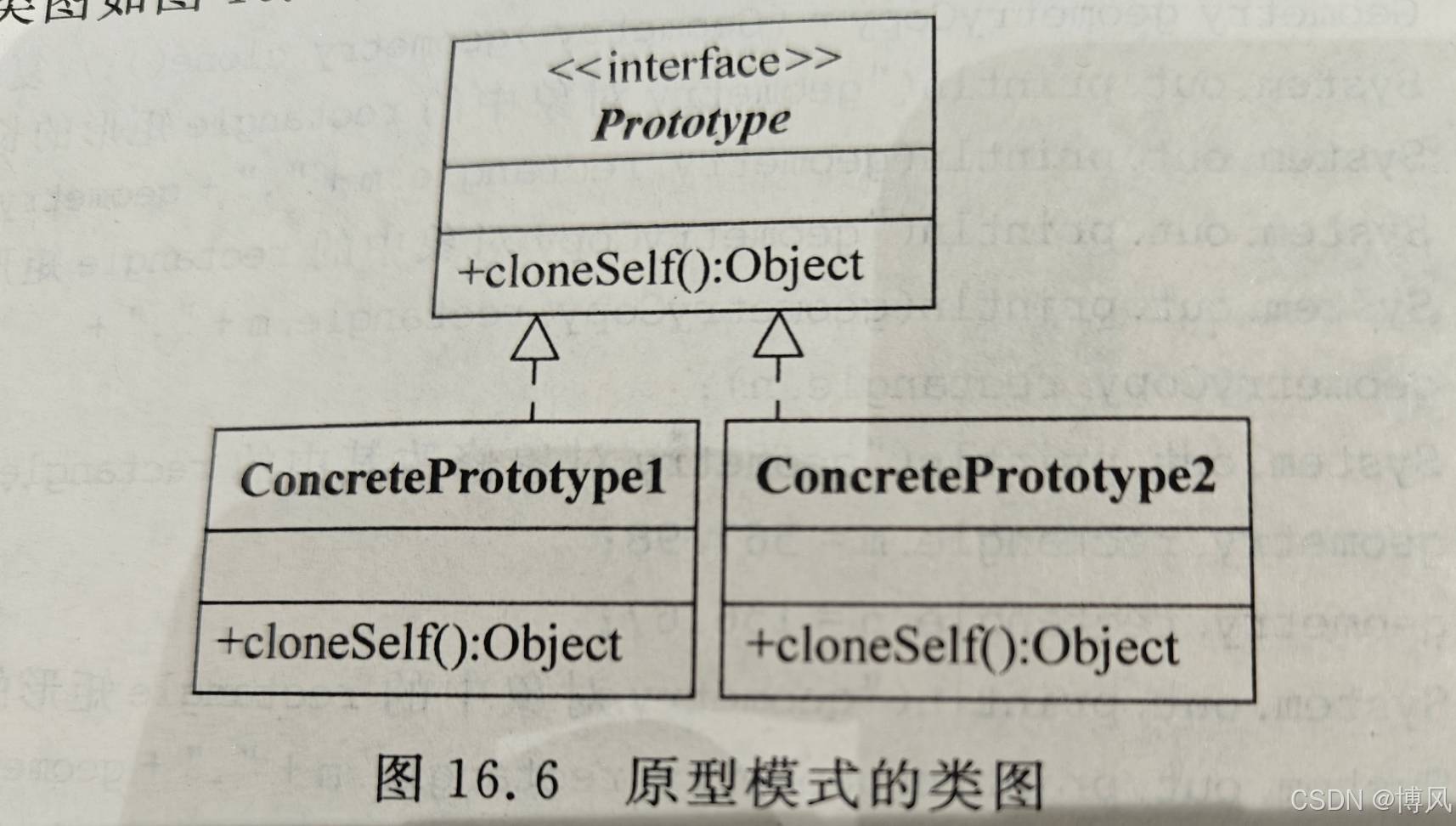
3、示例代码
抽象原型
java
package xyz.jangle.design.prototype;
public interface Prototype {
public Object cloneSelf() throws CloneNotSupportedException;
}
具体原型1
java
package xyz.jangle.design.prototype;
public class ConcretePrototype1 implements Prototype ,Cloneable{
int a,b,c;
public ConcretePrototype1(int a, int b, int c) {
super();
this.a = a;
this.b = b;
this.c = c;
}
@Override
public Object cloneSelf() throws CloneNotSupportedException {
return super.clone();
}
}
具体原型2
java
package xyz.jangle.design.prototype;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
public class ConcretePrototype2 implements Prototype, Serializable {
/**
*
*/
private static final long serialVersionUID = -1905246759025489290L;
private String str;
public ConcretePrototype2(String str) {
super();
this.str = str;
}
@Override
public Object cloneSelf() throws CloneNotSupportedException {
Object object = null;
try {
ByteArrayOutputStream out1 = new ByteArrayOutputStream();
ObjectOutputStream out2 = new ObjectOutputStream(out1);
out2.writeObject(this);
ByteArrayInputStream in1 = new ByteArrayInputStream(out1.toByteArray());
ObjectInputStream in2 = new ObjectInputStream(in1);
object = in2.readObject();
} catch (Exception e) {
e.printStackTrace();
}
return object;
}
public String getStr() {
return str;
}
public void setStr(String str) {
this.str = str;
}
}
具体原型3
java
package xyz.jangle.design.prototype;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
public class ConcretePrototype3 implements Prototype, Serializable {
/**
*
*/
private static final long serialVersionUID = 7486786403960307872L;
private AAA aaa;
public AAA getAaa() {
return aaa;
}
public void setAaa(AAA aaa) {
this.aaa = aaa;
}
@Override
public Object cloneSelf() throws CloneNotSupportedException {
Object object = null;
try {
ByteArrayOutputStream out1 = new ByteArrayOutputStream();
ObjectOutputStream out2 = new ObjectOutputStream(out1);
out2.writeObject(this);
ByteArrayInputStream in1 = new ByteArrayInputStream(out1.toByteArray());
ObjectInputStream in2 = new ObjectInputStream(in1);
object = in2.readObject();
} catch (Exception e) {
e.printStackTrace();
}
return object;
}
}
class AAA implements Serializable{
/**
*
*/
private static final long serialVersionUID = 963011062357603854L;
private int a,b;
public int getA() {
return a;
}
public void setA(int a) {
this.a = a;
}
public int getB() {
return b;
}
public void setB(int b) {
this.b = b;
}
}
客户端(使用)
java
package xyz.jangle.design.prototype;
public class AppMain16 {
public static void main(String[] args) {
try {
// 基本数据类型克隆
ConcretePrototype1 prototype1 = new ConcretePrototype1(1, 2, 3);
ConcretePrototype1 self = (ConcretePrototype1) prototype1.cloneSelf();
System.out.println("a:"+self.a+",b:"+self.b+",c:"+self.c);
// 字符串克隆
ConcretePrototype2 prototype2 = new ConcretePrototype2("123");
ConcretePrototype2 self2 = (ConcretePrototype2) prototype2.cloneSelf();
System.out.println(self2.getStr());
self2.setStr("456");
System.out.println(prototype2.getStr());
// 对象克隆
ConcretePrototype3 prototype3 = new ConcretePrototype3();
AAA aaa = new AAA();
aaa.setA(1);
aaa.setB(2);
prototype3.setAaa(aaa);
ConcretePrototype3 self3 = (ConcretePrototype3) prototype3.cloneSelf();
AAA aaa2 = self3.getAaa();
System.out.println(aaa2.getA()+","+aaa2.getB());
System.out.println(aaa==aaa2); //false 克隆出的2个引用不一样
aaa2.setA(7);
aaa2.setB(8);
System.out.println(aaa.getA()+","+aaa.getB()); // 1,2
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
}
}
输出结果:
bash
a:1,b:2,c:3
123
123
1,2
false
1,2