一、eslint9和prettier通用配置
安装必装插件
bash
pnpm add eslint@latest -D
ESlint配置 vue 规则 , typescript解析器
bash
pnpm add eslint-plugin-vue typescript-eslint -D
ESlint配置 JavaScript 规则
bash
pnpm add @eslint/js -D
配置所有全局变量 globals
bash
pnpm add globals -D
eslint 兼容prettier插件
bash
pnpm add eslint-plugin-prettier -D
prettier 插件
bash
pnpm add prettier -D
eslint.config.js通用配置文件(可直接复制使用)
javascript
import globals from 'globals'
import eslint from '@eslint/js'
import tseslint from 'typescript-eslint'
import eslintPluginVue from 'eslint-plugin-vue'
import vueParser from 'vue-eslint-parser'
import eslintPluginPrettierRecommended from 'eslint-plugin-prettier/recommended'
export default [
{
ignores: [
'node_modules',
'dist',
'.gitignore',
'package.json',
'package-lock.json',
'dist-ssr',
'*.local',
'.npmrc',
'.DS_Store',
'dev-dist',
'dist_electron',
'*.d.ts',
'src/assets/**'
]
},
/** js推荐配置 */
eslint.configs.recommended,
/** vue推荐配置 */
...eslintPluginVue.configs['flat/recommended'],
/** prettier 配置 */
eslintPluginPrettierRecommended,
//javascript 规则
{
files: ['**/*.{js,mjs,cjs,vue,ts}'],
rules: {
// 对象结尾逗号
'comma-dangle': 'off',
// 关闭未定义变量
'no-undef': 'off',
// 确保 Prettier 的行为不会被 ESLint 覆盖
quotes: ['error', 'single', { allowTemplateLiterals: true }],
// 关闭对未定义变量的警告
'no-undefined': 'off',
//不使用的变量不报错
'no-unused-vars': 'off',
// 禁止使用不规范的空格
'no-irregular-whitespace': 'off',
// 函数括号前的空格
'space-before-function-paren': 0,
// 箭头函数的空格
'arrow-spacing': [
2,
{
before: true,
after: true
}
],
// 代码块的空格
'block-spacing': [2, 'always'],
// 大括号风格
'brace-style': [
2,
'1tbs',
{
allowSingleLine: true
}
],
// 对象属性换行
'object-property-newline': 'off',
// JSX 引号风格
'jsx-quotes': [2, 'prefer-single'],
// 对象键值对之间的空格
'key-spacing': [
2,
{
beforeColon: false,
afterColon: true
}
],
// 关键字之间的空格
'keyword-spacing': [
2,
{
before: true,
after: true
}
],
// 构造函数首字母大写
'new-cap': [
2,
{
newIsCap: true,
capIsNew: false
}
],
// new 操作符使用时需要括号
'new-parens': 2,
// 禁止使用 Array 构造函数
'no-array-constructor': 2,
// 禁止调用 caller 和 callee
'no-caller': 2,
// 禁止重新分配类名
'no-class-assign': 2,
// 禁止条件中的赋值操作
'no-cond-assign': 2,
// 禁止 const 重新分配
'no-const-assign': 2,
// 正则表达式中的控制字符
'no-control-regex': 0,
// 禁止删除变量
'no-delete-var': 2,
// 禁止在函数参数中使用重复名称
'no-dupe-args': 2,
// 禁止在类中使用重复名称的成员
'no-dupe-class-members': 2,
// 禁止在对象字面量中使用重复的键
'no-dupe-keys': 2,
// 禁止重复的 case 标签
'no-duplicate-case': 2,
// 禁止空的字符类
'no-empty-character-class': 2,
// 禁止空的解构模式
'no-empty-pattern': 2,
// 禁止使用 eval
'no-eval': 2,
// 不允许出现空的代码块
'no-empty': 2,
// 禁止不必要的布尔转换
'no-extra-boolean-cast': 2,
// 禁止不必要的括号
'no-extra-parens': [2, 'functions'],
// 禁止 case 语句落空
'no-fallthrough': 2,
// 禁止在数字后面添加小数点
'no-floating-decimal': 2,
// 禁止对函数声明重新赋值
'no-func-assign': 2,
// 禁止出现歧义多行表达式
'no-unexpected-multiline': 2,
// 禁止不需要的转义
'no-useless-escape': 0,
// 数组的括号前后的间距
'array-bracket-spacing': [2, 'never']
}
},
// vue 规则
{
files: ['**/*.vue'],
languageOptions: {
parser: vueParser,
globals: { ...globals.browser, ...globals.node },
parserOptions: {
/** typescript项目需要用到这个 */
parser: tseslint.parser,
ecmaVersion: 'latest',
/** 允许在.vue 文件中使用 JSX */
ecmaFeatures: {
jsx: true
}
}
},
rules: {
'vue/component-definition-name-casing': 'off',
'vue/singleline-html-element-content-newline': ['off'],
'vue/no-mutating-props': [
'error',
{
shallowOnly: true
}
],
// 要求组件名称始终为 "-" 链接的单词
'vue/multi-word-component-names': 'off',
// 关闭 index.html 文件报 clear 错误
'vue/comment-directive': 'off',
// 关闭对 defineProps 的有效性检查
'vue/valid-define-props': 'off',
// 允许在一个文件中定义多个组件
'vue/one-component-per-file': 'off',
// 关闭 Prop 类型要求的警告
'vue/require-prop-types': 'off',
// 关闭属性顺序要求
'vue/attributes-order': 'off',
// 关闭对默认 Prop 的要求
'vue/require-default-prop': 'off',
// 关闭连字符命名检验
'vue/attribute-hyphenation': 'off',
// 关闭自闭合标签的要求
'vue/html-self-closing': 'off',
// 禁止在关闭的括号前有换行
'vue/html-closing-bracket-newline': 'off',
// 允许使用 v-html 指令
'vue/no-v-html': 'off'
}
}
]
.prettierrc.js 通用配置文件(可直接复制使用)
注意如prettier配置的格式没效果可更改文件后缀为.prettierrc.json或.prettierrc.yaml
javascript
module.exports = {
printWidth: 120, // 一行的字符数换行
tabWidth: 2, // 一个tab代表几个空格数
useTabs: false, // 是否使用tab进行缩进
singleQuote: true, // 字符串是否使用单引号
semi: false, // 行尾是否使用分号,默认为true
trailingComma: 'none', // 是否使用尾逗号
arrowParens: 'avoid', // 箭头函数单变量省略括号
bracketSpacing: true, // 对象大括号直接是否有空格,默认为true,效果:{ foo: bar }
endOfLine: 'auto', // 保留在 Windows 和 Unix 下的换行符
quoteProps: 'preserve' // 对象属性的引号使用
}
package.json 的scripts配置
bash
"scripts": {
"lint:fix": "eslint . --fix",
"lint": "eslint .",
},
.prettierignore 配置文件
bash
.DS_Store
node_modules
dist
dist-ssr
*.local
.npmrc
dev-dist
dist_electron
auto-imports.d.ts
二、配置git hooks提交检验
安装lint-staged
mrm 安装
lint-staged
的同时会安装husky
bash
pnpm install mrm -D
npx mrm lint-staged
然后它会自动在package.json文件中的script对象中生成
bash
"prepare": "husky install"
生成的可能只有一条或俩条,自己再加几条
bash
"lint-staged": {
"*.{js,jsx,vue,ts,tsx}": [
"eslint --fix",
"prettier --write"
],
"*.{scss,less,css,html,md}": [
"prettier --write"
],
"package.json": [
"prettier --write"
],
"{!(package)*.json,.!(browserslist)*rc}": [
"prettier --write--parser json"
]
}
同时在根目录也会生成.husky目录,如下图
配置 commitlint
bash
pnpm install @commitlint/cli @commitlint/config-conventional -D
bash
npx husky add .husky/commit-msg "npx --no-install commitlint --edit $1"
当运行之后就会生成这样如下图
如没有自动生成,手动创建文件,复制以下内容
bash
#!/usr/bin/env sh
. "$(dirname -- "$0")/_/husky.sh"
npx --no -- commitlint --edit ${1}
新建commitlint.config.cjs
bash
module.exports = {
extends: ['@commitlint/config-conventional'],
rules: {
'type-enum': [
// type枚举
2,
'always',
[
'build', // 编译相关的修改,例如发布版本、对项目构建或者依赖的改动
'feat', // 新功能
'fix', // 修补bug
'docs', // 文档修改
'style', // 代码格式修改, 注意不是 css 修改
'refactor', // 重构
'perf', // 优化相关,比如提升性能、体验
'test', // 测试用例修改
'revert', // 代码回滚
'ci', // 持续集成修改
'config', // 配置修改
'chore', // 其他改动
],
],
'type-empty': [2, 'never'], // never: type不能为空; always: type必须为空
'type-case': [0, 'always', 'lower-case'], // type必须小写,upper-case大写,camel-case小驼峰,kebab-case短横线,pascal-case大驼峰,等等
'scope-empty': [0],
'scope-case': [0],
'subject-empty': [2, 'never'], // subject不能为空
'subject-case': [0],
'subject-full-stop': [0, 'never', '.'], // subject以.为结束标记
'header-max-length': [2, 'always', 72], // header最长72
'body-leading-blank': [0], // body换行
'footer-leading-blank': [0, 'always'], // footer以空行开头
},
}
配置完成可提交代码测试
三、.editorconfig 编辑器配置
项目根目录创建 .editorconfig
文件
bash
# 修改配置后重启编辑器
# 配置项文档:https://editorconfig.org/
# 告知 EditorConfig 插件,当前即是根文件
root = true
# 适用全部文件
[*]
## 设置字符集
charset = utf-8
## 缩进风格 space | tab,建议 space(会自动继承给 Prettier)
indent_style = space
## 缩进的空格数(会自动继承给 Prettier)
indent_size = 2
## 换行符类型 lf | cr | crlf,一般都是设置为 lf
end_of_line = lf
## 是否在文件末尾插入空白行
insert_final_newline = true
## 是否删除一行中的前后空格
trim_trailing_whitespace = true
# 适用 .md 文件
[*.md]
insert_final_newline = false
trim_trailing_whitespace = false
四、 stylelint 配置
vscode 安装插件Stylelint
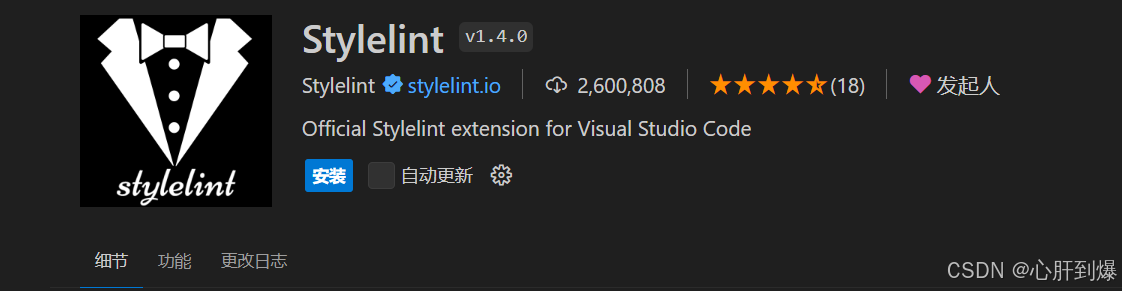
使用scss作为预处理器,安装以下依赖:
bash
pnpm add sass sass-loader stylelint postcss postcss-scss postcss-html stylelint-config-prettier stylelint-config-recess-order stylelint-config-recommended-scss stylelint-config-standard stylelint-config-standard-vue stylelint-scss stylelint-order stylelint-config-standard-scss -D
新建 .stylelintrc.cjs
在项目根目录下新建 .stylelintrc.cjs
,并填入如下代码:
bash
module.exports = {
extends: [
'stylelint-config-standard', // 配置stylelint拓展插件
'stylelint-config-html/vue', // 配置 vue 中 template 样式格式化
'stylelint-config-standard-scss', // 配置stylelint scss插件
'stylelint-config-recommended-vue/scss', // 配置 vue 中 scss 样式格式化
'stylelint-config-recess-order', // 配置stylelint css属性书写顺序插件,
'stylelint-config-prettier', // 配置stylelint和prettier兼容
],
overrides: [
{
files: ['**/*.(scss|css|vue|html)'],
customSyntax: 'postcss-scss',
},
{
files: ['**/*.(html|vue)'],
customSyntax: 'postcss-html',
},
],
ignoreFiles: [
'**/*.js',
'**/*.jsx',
'**/*.tsx',
'**/*.ts',
'**/*.json',
'**/*.md',
'**/*.yaml',
],
/**
* null => 关闭该规则
* always => 必须
*/
rules: {
'value-keyword-case': null, // 在 css 中使用 v-bind,不报错
'no-descending-specificity': null, // 禁止在具有较高优先级的选择器后出现被其覆盖的较低优先级的选择器
'function-url-quotes': 'always', // 要求或禁止 URL 的引号 "always(必须加上引号)"|"never(没有引号)"
'no-empty-source': null, // 关闭禁止空源码
'selector-class-pattern': null, // 关闭强制选择器类名的格式
'property-no-unknown': null, // 禁止未知的属性(true 为不允许)
'block-opening-brace-space-before': 'always', //大括号之前必须有一个空格或不能有空白符
'value-no-vendor-prefix': null, // 关闭 属性值前缀 --webkit-box
'property-no-vendor-prefix': null, // 关闭 属性前缀 -webkit-mask
'selector-pseudo-class-no-unknown': [
// 不允许未知的选择器
true,
{
ignorePseudoClasses: ['global', 'v-deep', 'deep'], // 忽略属性,修改element默认样式的时候能使用到
},
],
},
}
新建 .stylelintignore 文件
在项目根目录下新建 .stylelintignore
文件,并填入如下代码:
bash
/node_modules/*
/dist/*
/html/*
/public/*
添加脚本
在 packjson.json
文件的 script
字段中添加命令
bash
"lint:style": "stylelint src/**/*.{css,scss,vue} --cache --fix"
五、 项目中根目录下 .vscode 的配置
bash
┌─根目录
│ ├─.vscode
│ │ └─settings.json
settings.json
bash
{
"prettier.enable": true,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "explicit"
},
"[vue]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[javascript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[typescript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[json]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[jsonc]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[html]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[css]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[scss]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"eslint.validate": [
"javascript",
"vue",
"vue-html",
"typescript",
"typescriptreact",
"html",
"css",
"scss",
"less",
"json",
"jsonc",
"json5",
"markdown"
]
}