判断布尔值
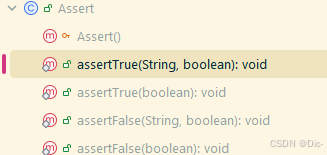
assertTrue
assertFalse
判断对象非空
assertNull(object);
案例:
java
PersistableBundle result = Util.getCarrierConfig(mockContext, subId);
assertNull(result);
判断是否相等
assertEquals("mocked_string", result.toString());
java
package org.junit;
public class Assert {
/**
* Asserts that two objects are equal. If they are not, an
* {@link AssertionError} without a message is thrown. If
* <code>expected</code> and <code>actual</code> are <code>null</code>,
* they are considered equal.
*
* @param expected expected value
* @param actual the value to check against <code>expected</code>
*/
public static void assertEquals(Object expected, Object actual) {
assertEquals(null, expected, actual);
}
}
可以用于字符串比较,比如下面的查询条件拼接
java
//测试类
public class ApnSettingsTest {
@Test
public void testGetFillListQuery_initialWhereEmpty() {
StringBuilder where = new StringBuilder();
int subId = 1;
// Mock the behavior of getSelection method
when(mFragment.getSelection("", subId)).thenReturn("mocked_selection");
StringBuilder result = mFragment.getFillListQuery(where, subId);
// Verify the `where` has the expected selection
assertEquals("mocked_selection", result.toString());
// Check log output (Could use a library or a custom handler to intercept logs)
// For simplicity, assuming log validation is part of the manual test validation process.
}
//TODO: 存在问题的测试接口,为什么其他两个getSelection可以返回预期??
@Test
public void testGetFillListQuery_initialWhereNonEmpty() {
StringBuilder where = new StringBuilder("existing_condition");
int subId = 1;
// Mock the behavior of getSelection method,没生效?
when(mFragment.getSelection("existing_condition", subId)).thenReturn(" AND mocked_selection");
StringBuilder result = mFragment.getFillListQuery(where, subId);
// Verify the `where` has the expected selection
// 校验存在问题
assertEquals("existing_condition AND mocked_selection", result.toString());
// Check log output similarly as mentioned in previous test
}
@Test
public void testGetFillListQuery_differentSubId() {
StringBuilder where = new StringBuilder();
int subId = 2;
// Mock the behavior of getSelection method
when(mFragment.getSelection("", subId)).thenReturn("different_selection");
StringBuilder result = mFragment.getFillListQuery(where, subId);
// Verify the `where` has the expected selection
assertEquals("different_selection", result.toString());
// Check log output similarly as mentioned in previous tests
}
}
//被测试类
public class DemoSettings {
@VisibleForTesting
StringBuilder getFillListQuery(StringBuilder where, int subId) {
String selection = "";
selection = getSelection(selection, subId);
where.append(selection); // 拼接新增的过滤条件
return where;
}
/**
* Customize the cursor select conditions with subId releated to SIM.
*/
String getSelection(String selection, int subId) {
boolean isCustomized = true;
Context context = getContext();
if (context != null) {
isCustomized = SubscriptionManager.getResourcesForSubId(getContext().getApplicationContext(), subId)
.getBoolean(R.bool.config_is_customize_selection);
}
if(!isCustomized) return selection;
// 注意有两个双引号的地方:第一个转义",第二个是字符串匹配
// 拼接的查询语句等效于:
// (type = 0 or sub_id = $传入值)
// SQL 查询语句:
// SELECT *
// FROM your_table
// WHERE status = 'active' AND (type = "0" OR sub_id = "subId");
String where = "(" + " type =\"" + "0" + "\"";
where += " or sub_id =\"" + subId + "\"";
where += " or type =\"" + "2" + "\"" + ")";
selection = selection + " and " + where;
return where;
}
// Additional tests could be written to consider edge cases, such as:
// - Negative subId values
// - Null or invalid StringBuilder where value
// In real scenario, depending on codebase other relevant checks can also be added.
}
上述代码校验存在问题,报错如下:
Expected :existing_condition AND mocked_selection
Actual :existing_condition and (type ="0" or sub_id ="1" or type ="2")
<Click to see difference>
org.junit.ComparisonFailure: expected:<existing_condition [AND mocked_selection]> but was:<existing_condition [and (type ="0" or sub_id ="1" or type ="2")]>
修改后pass的逻辑,因为内部会自己创建where值,不知道为什么不按 when.thenReturn 的返回 mocked_selection。
java
@Test
public void testGetFillListQuery_initialWhereNonEmpty() {
StringBuilder where = new StringBuilder("existing_condition");
int subId = 1;
// Mock the behavior of getSelection method
when(mFragment.getSelection("", subId))
.thenReturn(" and (type ='0' or sub_id ='1' or type ='2')");
StringBuilder result = mFragment.getFillListQuery(where, subId);
// Verify the `where` has the expected selection
assertEquals("existing_condition and (type ='0' or sub_id ='1' or type ='2')",
result.toString());
}