现实世界中的例子
假设你有一个包含不同页面的网站,并且你需要允许用户更改主题。你会怎么做?是为每个主题创建每个页面的多个副本,还是只创建单独的主题并根据用户的偏好加载它们?桥接模式允许你采用后者。
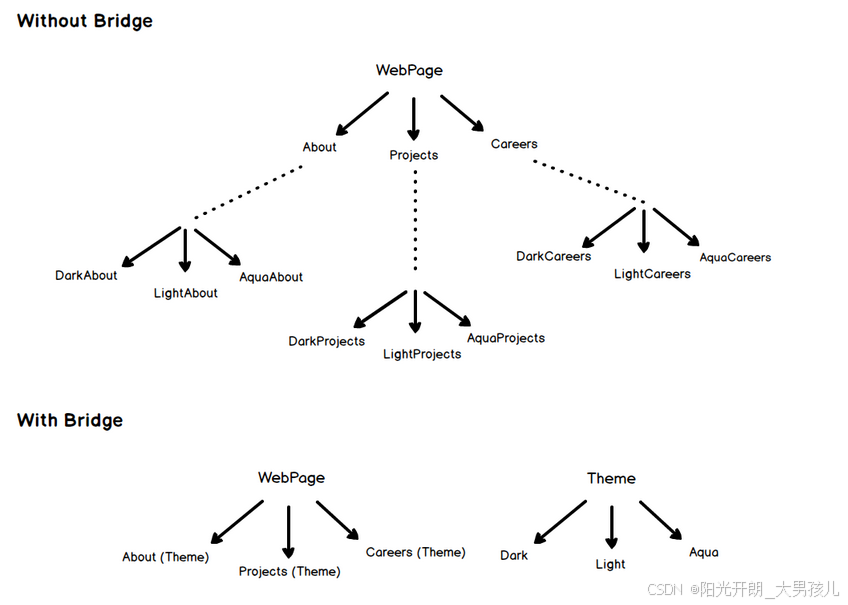
用简单的话来说
桥接模式是关于优先考虑组合而非继承的。它将实现细节从一个层次结构中提取出来,放入另一个对象层次结构中。
维基百科说
桥接模式是软件工程中使用的一种设计模式,旨在"将抽象部分与它的实现部分分离,使它们都可以独立地变化"。
程序示例
cpp
#include <iostream>
#include <string>
#include <memory>
// Theme interface
class Theme {
public:
virtual std::string getColor() const = 0;
virtual ~Theme() = default;
};
// DarkTheme implementation
class DarkTheme : public Theme {
public:
std::string getColor() const override {
return "Dark Black";
}
};
// LightTheme implementation
class LightTheme : public Theme {
public:
std::string getColor() const override {
return "Off white";
}
};
// AquaTheme implementation
class AquaTheme : public Theme {
public:
std::string getColor() const override {
return "Light blue";
}
};
// WebPage interface
class WebPage {
protected:
std::shared_ptr<Theme> theme;
public:
WebPage(std::shared_ptr<Theme> theme) : theme(theme) {}
virtual std::string getContent() const = 0;
virtual ~WebPage() = default;
};
// About page implementation
class About : public WebPage {
public:
About(std::shared_ptr<Theme> theme) : WebPage(theme) {}
std::string getContent() const override {
return "About page in " + theme->getColor();
}
};
// Careers page implementation
class Careers : public WebPage {
public:
Careers(std::shared_ptr<Theme> theme) : WebPage(theme) {}
std::string getContent() const override {
return "Careers page in " + theme->getColor();
}
};
int main() {
std::shared_ptr<Theme> darkTheme = std::make_shared<DarkTheme>();
std::shared_ptr<WebPage> about = std::make_shared<About>(darkTheme);
std::shared_ptr<WebPage> careers = std::make_shared<Careers>(darkTheme);
std::cout << about->getContent() << std::endl; // "About page in Dark Black"
std::cout << careers->getContent() << std::endl; // "Careers page in Dark Black"
return 0;
}
解释:
- 主题接口和实现:定义了Theme接口,并提供了不同的主题实现(DarkTheme、LightTheme、AquaTheme)。每个实现提供不同的颜色主题。
- 网页接口和实现:定义了WebPage接口,并提供了具体的页面实现(About、Careers),这些实现使用主题来生成内容。
- 组合优于继承:我们使用组合来注入不同的主题到页面对象中,而不是为每个页面的每个主题创建多个类。
- 动态绑定:使用std::shared_ptr进行动态绑定,允许在运行时设置或更改主题。