目录:
1、应用通知的表现形式
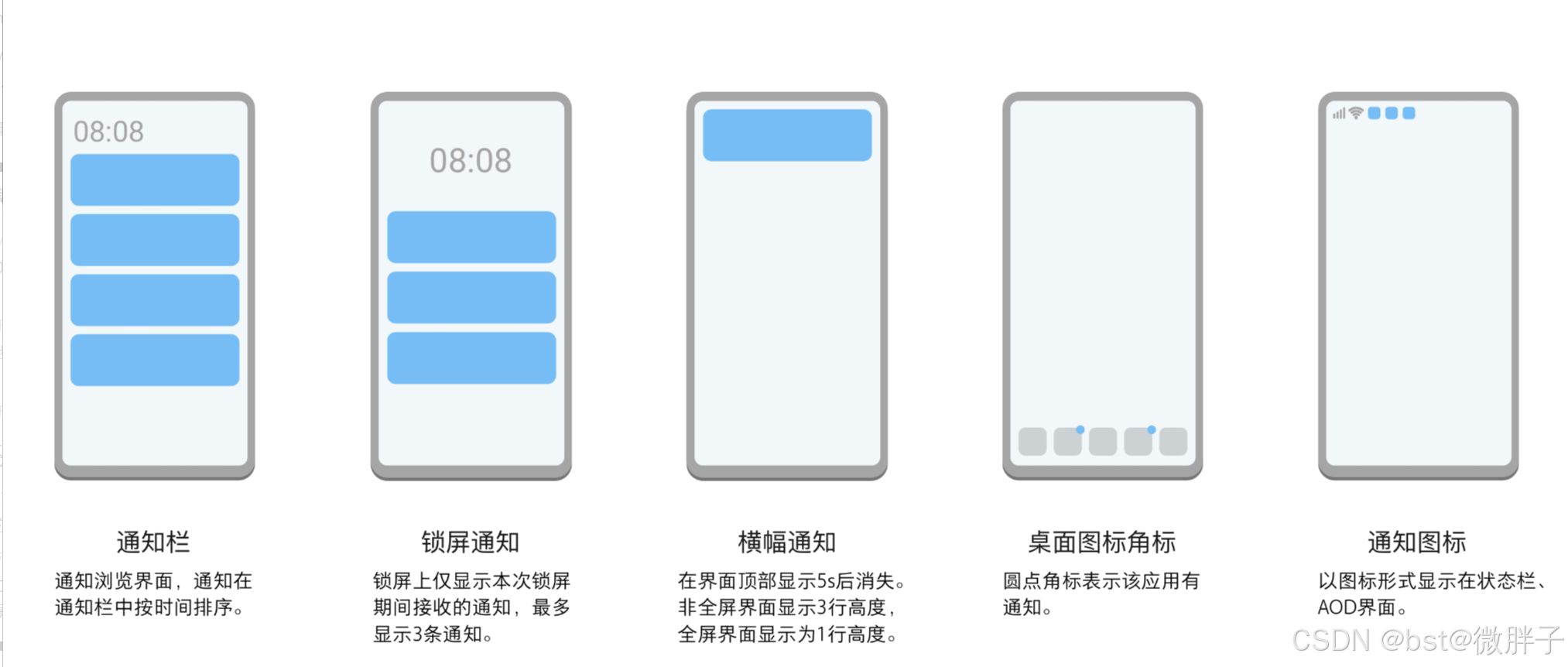
2、应用通知消息的实现
1、发布普通文本类型通知
基础类型通知主要应用于发送短信息、提示信息、广告推送等,支持普通文本类型、长文本类型、多行文本类型,可以通过ContentType指定通知的内容类型。下面以普通文本类型为例来介绍基础通知的发布。
需要设置ContentType类型为ContentType.NOTIFICATION_CONTENT_BASIC_TEXT
typescript
@Entry
@Component
struct NotificationDemo {
publishNotification() {
let notificationRequest: notificationManager.NotificationRequest = { // 描述通知的请求
id: 1, // 通知ID
content: { // 通知内容
notificationContentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT, // 普通文本类型通知
normal: { // 基本类型通知内容
title: '通知内容标题',
text: '通知内容详情'
}
}
}
notificationManager.publish(notificationRequest).then(() => { // 发布通知
console.info('publish success');
}).catch((err: Error) => {
console.error(`publish failed,message is ${err}`);
});
}
build() {
Column() {
Button('发送通知')
.onClick(() => {
this.publishNotification()
})
}
.width('100%')
}
}
效果图如下:
2、发布进度类型通知
进度条通知也是常见的通知类型,主要应用于文件下载、事务处理进度显示。目前系统模板仅支持进度条模板。
在发布进度类型通知前需要查询系统是否支持进度条模板:
typescript
notificationManager.isSupportTemplate('downloadTemplate').then(isSupport => {
if (!isSupport) {
promptAction.showToast({
message: $r('app.string.invalid_button_toast')
})
}
this.isSupport = isSupport;
});
构造进度条模板,name字段当前需要固定配置为downloadTemplate:
typescript
let template: notificationManager.NotificationTemplate = {
name: 'downloadTemplate',
data: {
progressValue: progress, // 当前进度值
progressMaxValue: 100 // 最大进度值
}
}
let notificationRequest: notificationManager.NotificationRequest = {
id: 1,
content: {
notificationContentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT,
normal: {
title: '文件下载:music.mp4',
text: 'senTemplate',
additionalText: '60%'
}
},
template: template
}
// 发布通知
notificationManager.publish(notificationRequest).then(() => {
console.info(`publish success`);
}).catch((err: Error) => {
console.error(`publish failed,message is ${err}`);
})
3、更新通知
在发出通知后,使用您之前使用的相同通知ID,再次调用notificationManager.publish来实现通知的更新。如果之前的通知是关闭的,将会创建新通知。
根据普通文本通知代码示例,但需notificationRequest构造参数任然使用之前的通知id再次调用就行了。
typescript
notificationManager.publish(notificationRequest).then(() => { // 发布通知
console.info('publish success');
}).catch((err: Error) => {
console.error(`publish failed,message is ${err}`);
});
}
4、移除通知
typescript
//通过通知ID和通知标签取消已发布的通知。
notificationManager.cancel(notificationId)
typescript
//取消所有已发布的通知。
notificationManager.cancelAll()
3、设置通知道通展示不同形式通知
typescript
@Entry
@Component
struct NotificationDemo {
publishNotification() {
let notificationRequest: notificationManager.NotificationRequest = { // 描述通知的请求
id: 1, // 通知ID
content: { // 通知内容
notificationContentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT, // 普通文本类型通知
normal: { // 基本类型通知内容
title: '张三',
text: '等会下班一起吃饭哦'
}
}
}
notificationManager.publish(notificationRequest).then(() => { // 发布通知
console.info('publish success');
}).catch((err: Error) => {
console.error(`publish failed,message is ${err}`);
});
}
build() {
Column() {
Button('发送通知')
.onClick(() => {
this.publishNotification()
})
}
.width('100%')
}
}
//这里添加不通类型通道,上面的通知信息以及发布信息依然要有
notificationManager.addSlot(notificationManager.SlotType.SOCIAL_COMMUNICATION).then(() => {
console.info("addSlot success");
}).catch((err: Base.BusinessError) => {
console.error(`addSlot fail: ${JSON.stringify(err)}`);
});
通知通道类型主要有以下几种:
- SlotType.SOCIAL_COMMUNICATION:社交类型,状态栏中显示通知图标,有横幅和提示音。
- SlotType.SERVICE_INFORMATION:服务类型,状态栏中显示通知图标,没有横幅但有提示音。
- SlotType.CONTENT_INFORMATION:内容类型,状态栏中显示通知图标,但没有横幅或提示音。
- SlotType.OTHER_TYPES:其它类型,状态栏中不显示通知图标,且没有横幅或提示音。
效果如下:
4、设置通知组
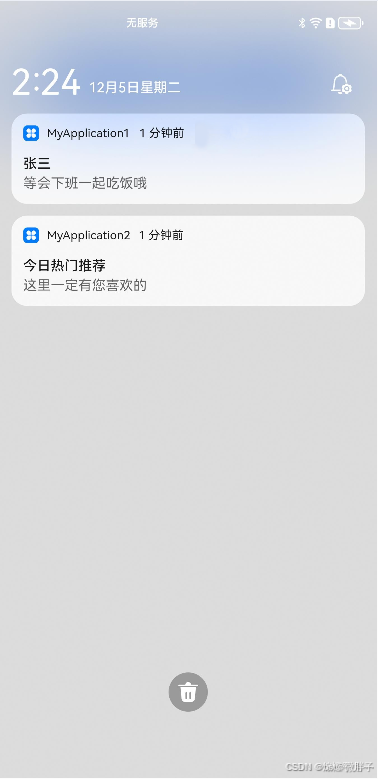
typescript
let notifyId = 0;
let chatRequest: notificationManager.NotificationRequest = {
id: notifyId++,
groupName:'ChatGroup',
content: {
//...
}
};
let productRequest: notificationManager.NotificationRequest = {
id: notifyId++,
groupName: 'ProductGroup',
content: {
//...
}
};
5、为通知添加行为意图
WantAgent提供了封装行为意图的能力,这里所说的行为意图主要是指拉起指定的应用组件及发布公共事件等能力。给通知添加行为意图后,点击通知后可以拉起指定的UIAbility或者发布公共事件。
您可以按照以下步骤来实现:
1、导入模块
typescript
import { notificationManager } from '@kit.NotificationKit';
import { wantAgent, WantAgent } from '@kit.AbilityKit';
2、创建WantAgentInfo信息
a、拉起UIAbility
typescript
let wantAgentInfo = {
wants: [
{
bundleName: "com.example.notification",
abilityName: "EntryAbility"
}
],
operationType: wantAgent.OperationType.START_ABILITY,
requestCode: 100
}
b、发布公共事件
typescript
let wantAgentInfo = {
wants: [
{
action: 'event_name', // 设置事件名
parameters: {},
}
],
operationType: wantAgent.OperationType.SEND_COMMON_EVENT,
requestCode: 100,
wantAgentFlags: [wantAgent.WantAgentFlags.CONSTANT_FLAG],
}
3、创建WantAgent对象
typescript
let wantAgentObj = null;
wantAgent.getWantAgent(wantAgentInfo)
.then((data) => {
wantAgentObj = data;
})
.catch((err: Error) => {
console.error(`get wantAgent failed because ${JSON.stringify(err)}`);
})
4、构造NotificationRequest对象
typescript
let notificationRequest: notificationManager.NotificationRequest = {
id: 1,
content: {
notificationContentType: notificationManager.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT,
normal: {
title: "通知标题",
text: "通知内容"
}
},
wantAgent: wantAgentObj
};
5、发布WantAgent通知
typescript
notificationManager.publish(notificationRequest).then(() => { // 发布通知
console.info("publish success");
}).catch((err: Error) => {
console.error(`publish failed, code is ${err.code}, message is ${err.message}`);
});