下面是一个包含登录页面和人事管理功能的简单OA系统示例。这个系统使用HTML和JavaScript实现。
1.页面展示
登录页面
首先,我们创建一个简单的登录页面。
OA系统页面
接下来是登录成功后的OA系统页面,包含新增、删除、修改公司人员的基本信息等功能,并且使用下拉框来选择学历和工作岗位。
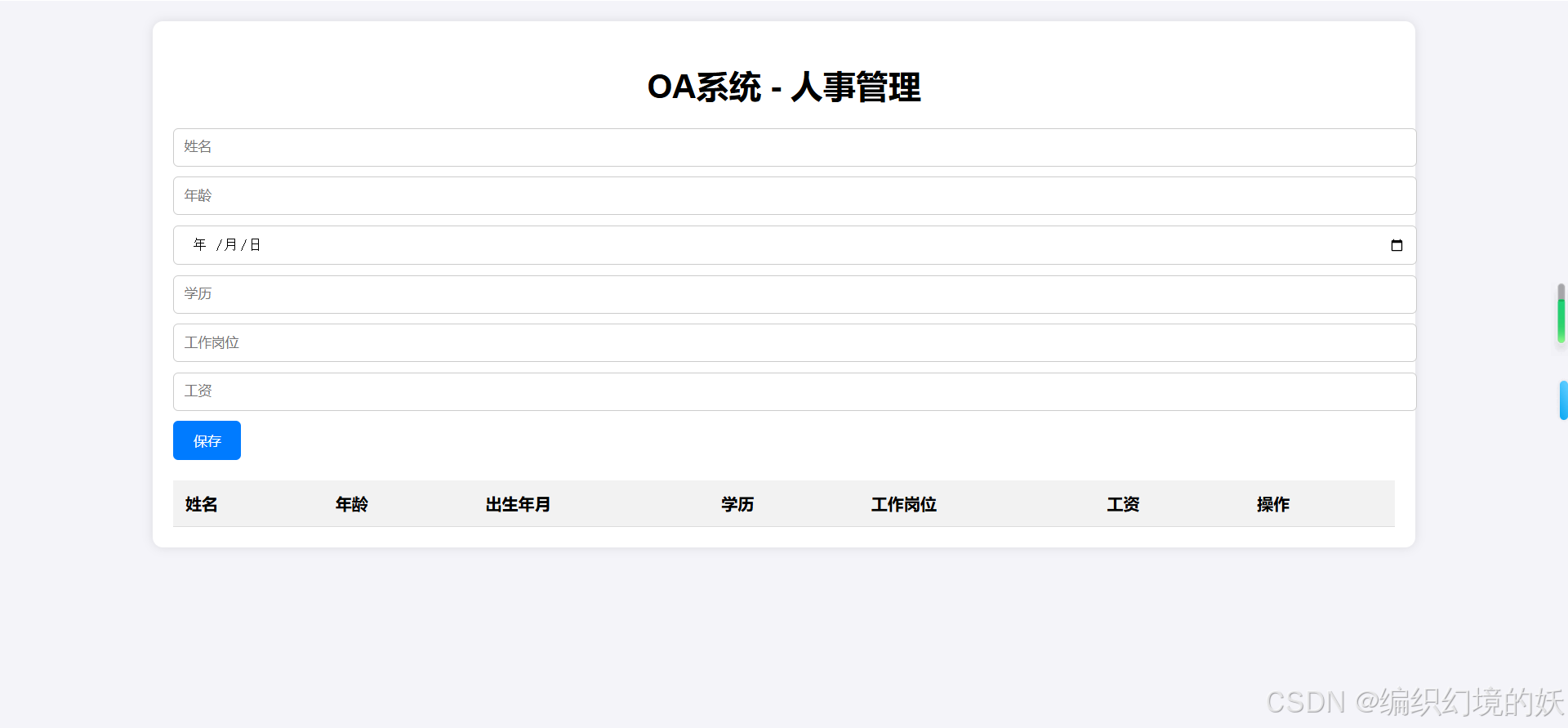
2.功能说明:
-
登录页面 (
login.html
):- 包含用户名和密码输入框。
- 点击"登录"按钮后,进行简单的验证(用户名为 "admin",密码为 "password")。
- 验证通过后,跳转到
oa-system.html
页面。
-
OA系统页面 (
oa-system.html
):- 包含一个表单用于输入员工的基本信息(姓名、年龄、学历、工作岗位、工资)。
- 学历和工作岗位使用下拉框选择。
- 表格展示了所有员工的信息,并提供了编辑和删除按钮。
- 点击"保存"按钮后,如果是在编辑模式下,则更新员工信息;否则,添加新员工。
- 点击"编辑"按钮后,表单会填充所选员工的信息以便编辑。
- 点击"删除"按钮后,会弹出确认对话框,确认后删除该员工。
- 表格中的每一行显示员工的所有信息,并且可以进行编辑和删除操作。
3.代码展示
login.html
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录页面</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.login-container {
background-color: white;
padding: 40px;
border-radius: 10px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.1);
width: 300px;
text-align: center;
}
.login-container h1 {
margin-bottom: 30px;
color: #333;
}
.login-container input[type="text"],
.login-container input[type="password"] {
width: 100%;
padding: 15px;
margin-bottom: 20px;
border: 1px solid #ccc;
border-radius: 10px;
font-size: 16px;
}
.login-container button {
padding: 15px 30px;
background-color: #007bff;
color: white;
border: none;
border-radius: 10px;
cursor: pointer;
font-size: 16px;
}
.login-container button:hover {
background-color: #0056b3;
}
.error-message {
color: red;
margin-top: 10px;
}
</style>
</head>
<body>
<div class="login-container">
<h1>登录</h1>
<input type="text" id="username" placeholder="用户名" required>
<input type="password" id="password" placeholder="密码" required>
<button onclick="login()">登录</button>
<div id="error-message" class="error-message"></div>
</div>
<script>
function login() {
const username = document.getElementById('username').value;
const password = document.getElementById('password').value;
// 示例验证:用户名为 "admin",密码为 "password"
if (username === 'admin' && password === 'password') {
window.location.href = 'oa-system.html';
} else {
document.getElementById('error-message').textContent = '用户名或密码错误';
}
}
</script>
</body>
</html>
oa-system.html
html
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>OA系统</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
margin: 0;
padding: 20px;
}
.container {
width: 80%;
margin: auto;
background-color: white;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
}
.form-section {
margin-bottom: 20px;
}
.form-section input[type="text"],
.form-section input[type="number"],
.form-section select {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
.form-section button {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.form-section button:hover {
background-color: #0056b3;
}
table {
width: 100%;
border-collapse: collapse;
margin-top: 20px;
}
th, td {
padding: 12px;
text-align: left;
border-bottom: 1px solid #ddd;
}
th {
background-color: #f2f2f2;
}
tr:hover {
background-color: #f1f1f1;
}
.action-buttons {
display: flex;
gap: 10px;
}
.action-buttons button {
padding: 5px 10px;
background-color: #dc3545;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.action-buttons button.edit {
background-color: #ffc107;
}
.action-buttons button:hover {
opacity: 0.8;
}
</style>
</head>
<body>
<div class="container">
<h1>OA系统 - 人事管理</h1>
<div class="form-section">
<input type="hidden" id="employee-id" value="">
<input type="text" id="name" placeholder="姓名" required>
<input type="number" id="age" placeholder="年龄" min="0" required>
<select id="education" required>
<option value="">请选择学历</option>
<option value="小学">小学</option>
<option value="初中">初中</option>
<option value="高中">高中</option>
<option value="大专">大专</option>
<option value="本科">本科</option>
<option value="研究生">研究生</option>
<option value="硕士">硕士</option>
</select>
<select id="position" required>
<option value="">请选择工作岗位</option>
<option value="运维">运维</option>
<option value="开发">开发</option>
<option value="测试">测试</option>
<option value="行政">行政</option>
<option value="人事">人事</option>
<option value="财务">财务</option>
<option value="其他">其他</option>
</select>
<input type="number" id="salary" placeholder="工资" min="0" step="any" required>
<button onclick="saveEmployee()">保存</button>
</div>
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>学历</th>
<th>工作岗位</th>
<th>工资</th>
<th>操作</th>
</tr>
</thead>
<tbody id="employee-table-body">
</tbody>
</table>
</div>
<script>
let employees = [];
let currentEditIndex = -1;
function saveEmployee() {
const name = document.getElementById('name').value.trim();
const age = parseInt(document.getElementById('age').value, 10);
const education = document.getElementById('education').value;
const position = document.getElementById('position').value;
const salary = parseFloat(document.getElementById('salary').value);
if (!name || !age || !education || !position || isNaN(salary)) {
alert('请输入完整且有效的信息!');
return;
}
const employee = { name, age, education, position, salary };
if (currentEditIndex !== -1) {
employees[currentEditIndex] = employee;
currentEditIndex = -1;
document.getElementById('employee-id').value = '';
alert('员工信息已更新');
} else {
employees.push(employee);
alert('新员工已添加');
}
updateTable();
clearForm();
}
function updateTable() {
const tbody = document.getElementById('employee-table-body');
tbody.innerHTML = '';
employees.forEach((employee, index) => {
const row = document.createElement('tr');
row.innerHTML = `
<td>${employee.name}</td>
<td>${employee.age}</td>
<td>${employee.education}</td>
<td>${employee.position}</td>
<td>¥${employee.salary.toFixed(2)}</td>
<td class="action-buttons">
<button class="edit" οnclick="editEmployee(${index})">编辑</button>
<button οnclick="deleteEmployee(${index})">删除</button>
</td>
`;
tbody.appendChild(row);
});
}
function deleteEmployee(index) {
if (confirm('确定要删除该员工吗?')) {
employees.splice(index, 1);
updateTable();
alert('员工已删除');
}
}
function editEmployee(index) {
const employee = employees[index];
document.getElementById('employee-id').value = index;
document.getElementById('name').value = employee.name;
document.getElementById('age').value = employee.age;
document.getElementById('education').value = employee.education;
document.getElementById('position').value = employee.position;
document.getElementById('salary').value = employee.salary;
currentEditIndex = index;
}
function clearForm() {
document.getElementById('employee-id').value = '';
document.getElementById('name').value = '';
document.getElementById('age').value = '';
document.getElementById('education').value = '';
document.getElementById('position').value = '';
document.getElementById('salary').value = '';
}
// 初始化表格
updateTable();
</script>
</body>
</html>