代码仓库:share_harmonyos: 鸿蒙分享 鸿蒙api:12
axios开源库地址:OpenHarmony三方库中心仓
1.axios使用
1.安装
ohpm install @ohos/axios

2.添加网络权限
common--src--module.json5 添加如下:
"requestPermissions": [
{
"name": "ohos.permission.INTERNET"
}
]
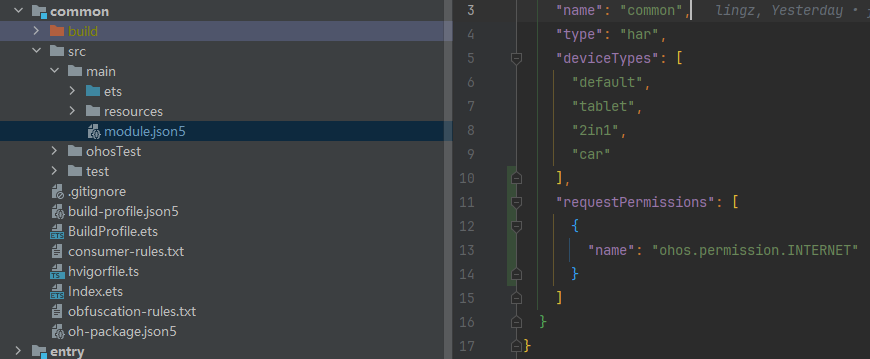
封装BaseHttp
其他代码查看代码仓库:share_harmonyos: 鸿蒙分享 - Gitee.com
import axios, { AxiosError, AxiosInstance, AxiosProgressEvent, AxiosRequestConfig, AxiosResponse, FormData } from '@ohos/axios';
import { LogUtil } from '@pura/harmony-utils';
import { BannerBean } from '../../bean/BannerBean';
import { OrderDetailBean } from '../../bean/OrderDetailBean';
import { UpLoadFileBean } from '../../bean/UpLoadFileBean';
import { BaseLog } from '../BaseLog';
import { BaseUrl } from '../BaseUrl';
import { BaseDialog } from '../dialog/BaseDialog';
import { BaseError } from './BaseError';
import { BaseRequestSet } from './BaseRequestSet';
import { BaseResponse } from './BaseResponse';
export class BaseHttp {
// axios 实例
private instance: AxiosInstance;
// axios 请求配置
private config: AxiosRequestConfig = {
baseURL: BaseUrl.BASE_URL, // http://xxxx/xxxx/
timeout: 10000
}
// 构造函数初始化
public constructor(config?: AxiosRequestConfig) {
if (!config) {
config = this.config
}
this.instance = axios.create(config);
//定义拦截器
this.interceptors();
//添加请求头
// this.getAxios().defaults.headers.common['code'] = BaseUrl.code;
// this.getAxios().defaults.headers.common['tid'] = BaseUrl.tid;
// this.getAxios().defaults.headers.common['Token'] = BaseUser.Token();
}
public getAxios() {
return this.instance;
}
request<T = BaseResponse>(
type: RequestType,
baseSet: BaseRequestSet,
success?: (result: T) => void,
fail?: (error: BaseError) => void,
complete?: () => void,
progress?: (progressEvent: AxiosProgressEvent, progress?: number) => void,
) {
if (baseSet.isLoading && type != RequestType.upload) {
this.showLoading(baseSet.loadingMsg)
}
let response: Promise<BaseResponse<T>>
switch (type) {
case RequestType.get:
response = this.instance.get<string, BaseResponse<T>>(baseSet?.url, { params: baseSet?.params })
break
case RequestType.post:
response = this.instance.post<string, BaseResponse<T>>(baseSet?.url, baseSet?.params)
break
case RequestType.put:
response = this.instance.put<string, BaseResponse<T>>(baseSet?.url, baseSet?.params)
break
case RequestType.delete:
response = this.instance.delete<string, BaseResponse<T>>(baseSet?.url, baseSet?.params)
break
//上传
case RequestType.upload:
response = this.instance.post<string, BaseResponse<T>, FormData>(baseSet?.url, baseSet?.formData, {
headers: {
'Content-Type': 'multipart/form-data'
},
onUploadProgress: (progressEvent) => {
if (progress && progressEvent && progressEvent.loaded && progressEvent.total) {
progressEvent.progress = Math.ceil(progressEvent.loaded / progressEvent.total * 100)
if (baseSet.isLoading) {
BaseDialog.showProgress(progressEvent.progress)
}
progress(progressEvent, progressEvent.progress)
}
}
})
break
default:
response = this.instance.get<string, BaseResponse<T>>(baseSet?.url, baseSet?.params)
break
}
response.then((res) => {
this.dealwithThen(res, success, fail, baseSet)
this.dealwithComplete(complete)
}).catch((error: AxiosError) => {
this.dealwithCatch(error, fail)
this.dealwithComplete(complete)
});
}
//处理成功
dealwithThen<T = BaseResponse>(res: BaseResponse<T>, success?: (result: T) => void, fail?: (error: BaseError) => void, baseSet?: BaseRequestSet) {
this.hintLoading()
if (res.code == 200) {
if (success) {
baseSet?.formData?.forEach((value: string, key) => {
(res.result as UpLoadFileBean).cachePath = value
})
success(res.result)
}
} else {
if (fail) {
let failResult: BaseError = {
message: res.message,
code: res.code
}
fail(failResult)
}
if (baseSet?.isShowToast) {
BaseDialog.showToast(res.message)
}
}
}
//下载文件
downloadFile(
baseSet: BaseRequestSet,
success?: (result?: string) => void,
fail?: (error: BaseError) => void,
complete?: () => void,
progress?: (progressEvent: AxiosProgressEvent, progress?: number) => void) {
axios({
url: baseSet.url,
method: 'get',
filePath: baseSet.filePath,
onDownloadProgress: (progressEvent: AxiosProgressEvent) => {
if (progress && progressEvent && progressEvent.loaded && progressEvent.total) {
progressEvent.progress = Math.ceil(progressEvent.loaded / progressEvent.total * 100)
if (baseSet.isLoading) {
BaseDialog.showProgress(progressEvent.progress)
}
progress(progressEvent, progressEvent.progress)
}
}
}).then((res: AxiosResponse<string>) => {
if (res.status == 200) {
if (success) {
success(res.config.filePath)
}
}
if (complete) {
if (baseSet.isLoading) {
BaseDialog.hideProgress()
}
complete()
}
}).catch((error: AxiosError) => {
let error3: BaseError = {
message: error.message,
code: error.code ?? error.status ?? 0
}
if (baseSet.isShowToast) {
BaseDialog.showToast(error3.message)
}
if (baseSet.isLoading) {
BaseDialog.hideProgress()
}
if (fail) {
fail(error3)
}
if (complete) {
complete()
}
})
}
//处理catch
dealwithCatch(error: AxiosError, fail?: (error: BaseError) => void) {
this.hintLoading()
if (fail) {
let error3: BaseError = {
message: error.message,
code: error.code ?? error.status ?? 0
}
LogUtil.info("接口请求失败:" + error.config?.url + ";" + JSON.stringify(error3) + ";" + JSON.stringify(error))
fail(error3)
}
}
//处理catch
dealwithComplete(Complete?: () => void) {
this.hintLoading()
if (Complete) {
Complete()
}
}
showLoading(msg: string = "") {
BaseDialog.showLoading(msg)
}
hintLoading() {
BaseDialog.hintLoading()
}
getRotationChartInfo(
success?: (result: Array<BannerBean>) => void,
fail?: (error: BaseError) => void,
complete?: () => void
) {
let baseSet = new BaseRequestSet()
baseSet.isLoading = false
baseSet.url = BaseUrl.BANNER_LIST // openAPI/xxx/xxxx
this.request<Array<BannerBean>>(RequestType.get, baseSet, success, fail, complete);
}
//提交订单
submitOrder(
classTypeId: string,
userCouponId: string,
success?: (result: OrderDetailBean) => void,
fail?: (error: BaseError) => void,
complete?: () => void
) {
let baseSet = new BaseRequestSet(Object({
classTypeId: classTypeId,
userCouponId: userCouponId,
}))
baseSet.isLoading = true
baseSet.url = BaseUrl.SUMMIT_ORDER
this.request<OrderDetailBean>(RequestType.post, baseSet, success, fail, complete);
}
//上传文件
uploadFile(
baseSet: BaseRequestSet,
success?: (result: UpLoadFileBean) => void,
fail?: (error: BaseError) => void,
complete?: () => void,
progress?: (progressEvent: AxiosProgressEvent, progress?: number) => void,
) {
baseSet.isLoading = true
baseSet.url = BaseUrl.UPLOAD_URL
this.request<UpLoadFileBean>(RequestType.upload, baseSet, success, fail, complete, progress);
}
private interceptors() {
// 请求返回处理
this.instance.interceptors.response.use((res: AxiosResponse) => {
BaseLog.i("请求返回拦截:" + JSON.stringify(res))
switch (res.data.code) {
case BaseUrl.TOKEN_CODE:
LogUtil.info("用户信息已失效,请重新登录.")
// BaseEmitter.post(BaseEmitter.id_to_login, "")
break
}
return res.data;
}, (error: AxiosError) => {
LogUtil.error("网络请求错误:", JSON.stringify(error))
return Promise.reject(error);
})
}
}
enum RequestType {
get = "get",
post = "post",
put = "put",
delete = "delete",
upload = "upload",
}
export default BaseHttp
BaseHttp使用:
1.设置baseURL
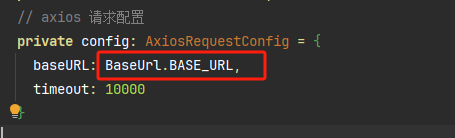
2.添加公共的请求头参数
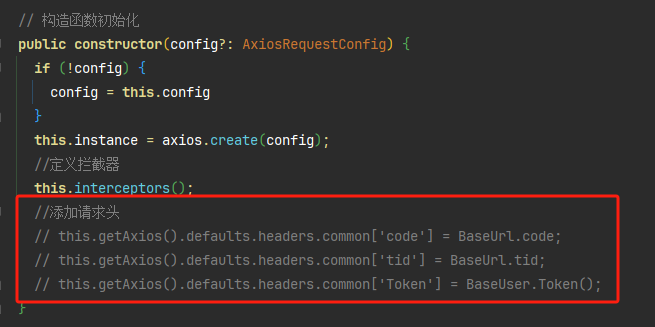
3.如果需要处理特定的错误代码
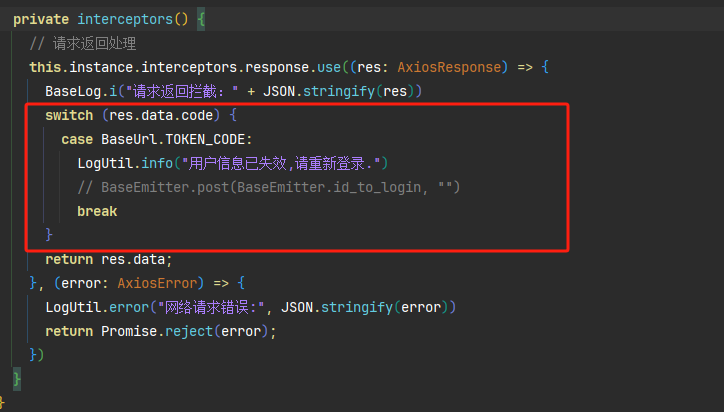
4.get请求 BaseHttp添加如下代码:
getRotationChartInfo(
success?: (result: Array<BannerBean>) => void,
fail?: (error: BaseError) => void,
complete?: () => void
) {
let baseSet = new BaseRequestSet()
baseSet.isLoading = false
baseSet.url = BaseUrl.BANNER_LIST // openAPI/xxx/xxxx
this.request<Array<BannerBean>>(RequestType.get, baseSet, success, fail, complete);
}
使用:
BaseText({
text: "get",
fontColor: $r('app.color.color_DD2942')
}).padding(12).margin(6).onClick(() => {
new BaseHttp().getRotationChartInfo(
(data) => {
//成功回调
},
(error) => {
//错误回调
},
() => {
//完成回调
},
)
})
5.post请求 BaseHttp添加如下代码:
//提交订单
submitOrder(
classTypeId: string,
userCouponId: string,
success?: (result: OrderDetailBean) => void,
fail?: (error: BaseError) => void,
complete?: () => void
) {
let baseSet = new BaseRequestSet(Object({
classTypeId: classTypeId,
userCouponId: userCouponId,
}))
baseSet.isLoading = true
baseSet.url = BaseUrl.SUMMIT_ORDER
this.request<OrderDetailBean>(RequestType.post, baseSet, success, fail, complete);
}
使用:
new BaseHttp().submitOrder(
"",
"-1",
(data) => {
},
(error) => {
},
() => {
}
)
6.上传文件:
BaseText({
text: "上传文件",
fontColor: $r('app.color.color_DD2942')
}).padding(12).margin(6).onClick(() => {
let baseRequestSet = new BaseRequestSet()
baseRequestSet.formData.append('file', "文件url")
new BaseHttp().uploadFile(baseRequestSet,
(success) => {
},
(error) => {
},
() => {
},
(progressEvent, progress) => {
}
)
})
7.下载文件:
BaseText({
text: "下载文件",
fontColor: $r('app.color.color_DD2942')
}).padding(12).margin(6).onClick(() => {
let baseRequestSet = new BaseRequestSet()
baseRequestSet.url = "下载地址"
baseRequestSet.filePath = "文件保存地址" // xxx/xxx.jpg
new BaseHttp().downloadFile(baseRequestSet,
(success) => {
},
(error) => {
},
() => {
},
(progressEvent, progress) => {
}
)
})