一、卖电影票

java
public class Main {
public static void main(String[] args) {
//创建线程对象
Ticket t1=new Ticket("电影院一:");
Ticket t2=new Ticket("电影院二:");
t1.start();
t2.start();
}
}
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Ticket extends Thread{
//1000张电影票
private static int count=100;
public Ticket(){
}
public Ticket(String name){
super(name);
}
//创建一个锁
private static Lock lock=new ReentrantLock();
@Override
public void run() {
while(true){
//上锁
lock.lock();
try {
if(count==0){
System.out.println("电影票卖完了");
break;
}else {
Thread.sleep(30);
count--;
System.out.println(this.getName()+"卖了一张票,还剩下"+count+"张票");
}
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
//解锁
lock.unlock();
}
}
}
}
二、送礼物

java
public class Main {
public static void main(String[] args) {
//创建线程对象
Ticket t1=new Ticket("小明");
Ticket t2=new Ticket("小张");
t1.start();
t2.start();
}
}
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Ticket extends Thread{
//100张电影票
private static int count=100;
public Ticket(){
}
public Ticket(String name){
super(name);
}
//创建一个锁
private static Lock lock=new ReentrantLock();
@Override
public void run() {
while(true){
//上锁
lock.lock();
try {
if(count<=10){
System.out.println("不送啦");
break;
}else {
Thread.sleep(30);
count--;
System.out.println(this.getName()+"送出一个礼物,还剩下"+count+"个礼物");
}
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
//解锁
lock.unlock();
}
}
}
}
三、打印奇数数字

java
public class Main {
public static void main(String[] args) {
//创建线程对象
Ticket t1=new Ticket("计数器1:");
Ticket t2=new Ticket("计数器2:");
t1.start();
t2.start();
}
}
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Ticket extends Thread{
//100张电影票
private static int count=1;
public Ticket(){
}
public Ticket(String name){
super(name);
}
//创建一个锁
private static Lock lock=new ReentrantLock();
@Override
public void run() {
while(true){
//上锁
lock.lock();
try {
if(count>100){
//超出100则停止
break;
}else {
//未超出100,则继续
Thread.sleep(10);
if(IsJIShu(count))
System.out.println(this.getName()+count+"是奇数");
count++;
}
} catch (InterruptedException e) {
throw new RuntimeException(e);
} finally {
//解锁
lock.unlock();
}
}
}
public static boolean IsJIShu(int number){
//判断奇数
int count=0;
for(int i=1;i<=Math.sqrt(number);i++){
if((number%i)==0)
count++;
}
if(count==1)
return true;
return false;
}
}
四、抢红包
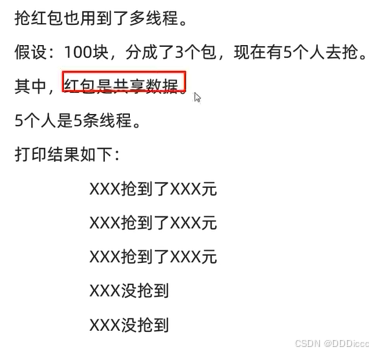
java
public class Main {
public static void main(String[] args) {
//创建线程对象
Player p1=new Player("玩家一");
Player p2=new Player("玩家二");
Player p3=new Player("玩家三");
Player p4=new Player("玩家四");
Player p5=new Player("玩家五");
p1.start();
p2.start();
p3.start();
p4.start();
p5.start();
}
}
import java.util.Random;
public class Player extends Thread{
private String name;
public static int Money=100;
public static int count=3;
public Player(){
}
public Player(String name){
this.name = name;
}
@Override
public void run() {
synchronized (Player.class){
if (count > 0) {
count--;
Random r = new Random();
if(count!=0){
int mon = r.nextInt(Money) + 1;
Money -= mon;
System.out.println(this.name + "抢到了" + mon + "元红包");
}else{
int mon=Money;
System.out.println(this.name + "抢到了" + mon + "元红包");
}
} else {
System.out.println(this.name + "没有抢到红包");
}
}
}
}
五、抽奖箱抽奖
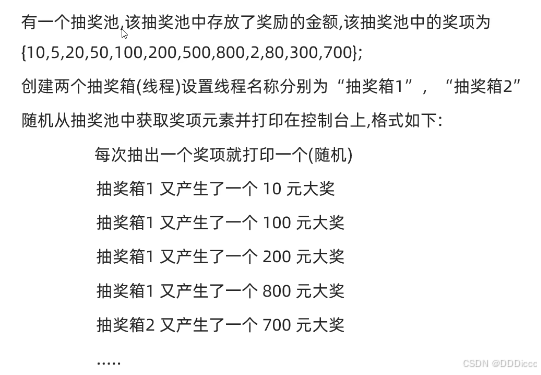
java
public class Main {
public static void main(String[] args) {
//创建线程对象
Box box=new Box();
Thread thread1=new Thread(box,"抽奖箱1");
Thread thread2=new Thread(box,"抽奖箱2");
thread2.start();
thread1.start();
}
}
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Box implements Runnable {
private static Lock lock=new ReentrantLock();
private static ArrayList<Integer> list=new ArrayList<>();
//静态代码块
static {
Collections.addAll(list,10,5,20,50,100,200,500,800,2,80,300,700);
}
@Override
public void run() {
if(!list.isEmpty()){
Thread thread=Thread.currentThread();
while (true) {
try {
lock.lock();
if (!list.isEmpty()) {
int count = list.size();
Random r = new Random();
int index = r.nextInt(count);
System.out.println(index);
System.out.println(list.size());
System.out.println(thread.getName() + "又产生了一个" + list.get(index) + "元大奖");
list.remove(index);
} else {
break;
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
lock.unlock();
}
}
}
}
}
六、抽奖箱进阶一
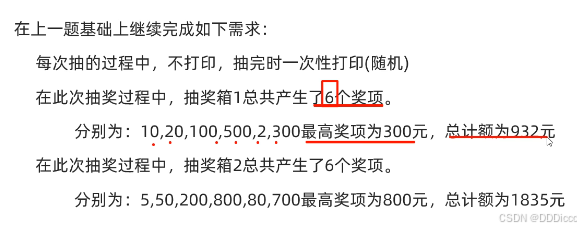
java
public class Main {
public static void main(String[] args) {
//创建线程对象
Box box1=new Box("抽奖箱一");
Box box2=new Box("抽奖箱二");
box2.start();
box1.start();
}
}
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Box extends Thread{
private static Lock lock=new ReentrantLock();
private static ArrayList<Integer> list=new ArrayList<>();
private String name;
private int big=0;
private int sum=0;
public Box(){
}
public Box(String name){
this.name=name;
}
private ArrayList<Integer> ownlist=new ArrayList<>();
//静态代码块
static {
Collections.addAll(list,10,5,20,50,100,200,500,800,2,80,300,700);
}
@Override
public void run() {
if(!list.isEmpty()){
Thread thread=Thread.currentThread();
while (true) {
try {
lock.lock();
if (!list.isEmpty()) {
int count = list.size();
Random r = new Random();
int index = r.nextInt(count);
//将抽奖结果存入集合中,等最后打印
this.ownlist.add(list.get(index));
sum+=list.get(index);
//当 big小于当前的抽奖结果时,将其赋值给big
this.big=this.big<list.get(index)?list.get(index):this.big;
// System.out.println(thread.getName() + "又产生了一个" + list.get(index) + "元大奖");
list.remove(index);
} else {
System.out.println("此次抽奖过程中,"+this.name+"总共产生了"+this.ownlist.size()+"个奖项");
System.out.print("分别为:");
for (Integer integer : this.ownlist) {
System.out.print(" "+integer+",");
}
System.out.println("最高奖项为"+this.big+",总计为 "+this.sum+" 元");
break;
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
lock.unlock();
}
}
}
}
}
七、抽奖箱进阶二
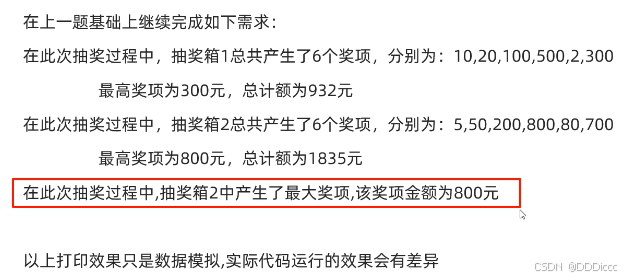
java
public class Main {
public static void main(String[] args) {
//创建线程对象
Box box1=new Box("抽奖箱一");
Box box2=new Box("抽奖箱二");
box2.start();
box1.start();
}
}
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class Box extends Thread{
private static Lock lock=new ReentrantLock();
private static ArrayList<Integer> list=new ArrayList<>();
private static int count=0;
private static ArrayList<String> biglist=new ArrayList<>();
private String name;
private int big=0;
private int sum=0;
public Box(){
}
public Box(String name){
this.name=name;
}
private ArrayList<Integer> ownlist=new ArrayList<>();
//静态代码块
static {
Collections.addAll(list,10,5,20,50,100,200,500,800,2,80,300,700);
}
@Override
public void run() {
if(!list.isEmpty()){
Thread thread=Thread.currentThread();
while (true) {
try {
lock.lock();
if (!list.isEmpty()) {
int count = list.size();
Random r = new Random();
int index = r.nextInt(count);
//将抽奖结果存入集合中,等最后打印
this.ownlist.add(list.get(index));
sum+=list.get(index);
//当 big小于当前的抽奖结果时,将其赋值给big
this.big=this.big<list.get(index)?list.get(index):this.big;
// System.out.println(thread.getName() + "又产生了一个" + list.get(index) + "元大奖");
list.remove(index);
Thread.yield();
} else {
System.out.println("此次抽奖过程中,"+this.name+"总共产生了"+this.ownlist.size()+"个奖项");
System.out.print("分别为:");
for (Integer integer : this.ownlist) {
System.out.print(" "+integer+",");
}
System.out.println("最高奖项为"+this.big+",总计为 "+this.sum+" 元");
count++;
biglist.add(this.name+","+this.big);
break;
}
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
lock.unlock();
}
}
if(count==2){
if(Integer.parseInt(biglist.get(0).split(",")[1])>Integer.parseInt(biglist.get(1).split(",")[1])){
System.out.println("在此次抽奖过程中,"+biglist.get(0).split(",")[0]+"产生了最大奖项,金额为"+Integer.parseInt(biglist.get(0).split(",")[1])+"元");
}else{
System.out.println("在此次抽奖过程中,"+biglist.get(1).split(",")[0]+"产生了最大奖项,金额为"+Integer.parseInt(biglist.get(1).split(",")[1])+"元");
}
}
}
}
}
另一种方法:

原理:
每个线程的run方法只会执行一次,run方法中的方法体,会在线程争夺到CPU使用权后进行。
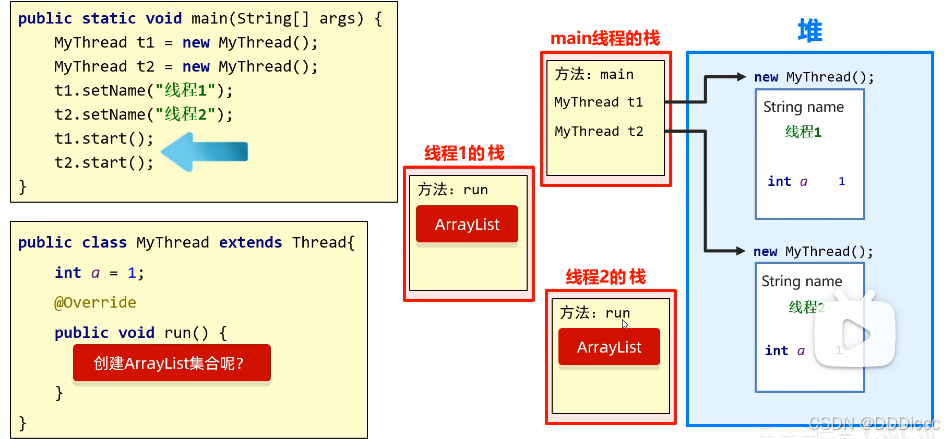