总目录
前言
通常对于WPF
输入框 输入的控制无非以下方式
- 1 直接禁止输入(包括粘贴) 不符合要求的字符
- 如只可输入数字的输入框,当你输入字母的时候是无法输入的
- 2 输入后,校验内容是否符合要求,然后提示错误,禁止提交信息
- 如只可输入手机号的输入框,你输入了不符合要求的格式,会提示格式有误,提交信息的时候再次提示数据格式有误,纠正后提交
- 该方式一般通过
ErrorTemplate
来实现错误信息的展示,再配合IDataErrorInfo
+DataAnnotations
完成对数据的验证
- 3 使用方式1和方式2 配合完成输入限制
本文主要针对第一种输入控制方式进行介绍。
一、预备内容
1. 预备知识
- 熟悉 C# 和 WPF
- 大部分的输入控制都是基于正则表达式对于输入内容进行验证的,因此有兴趣的可以先阅读 C# 正则表达式 详解 一文进行了解。
2. 准备代码
- 新建一个WPF项目
- MainWindow.xaml 代码如下:
xml
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid Background="LightGray">
<TextBox Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"></TextBox>
</Grid>
</Window>
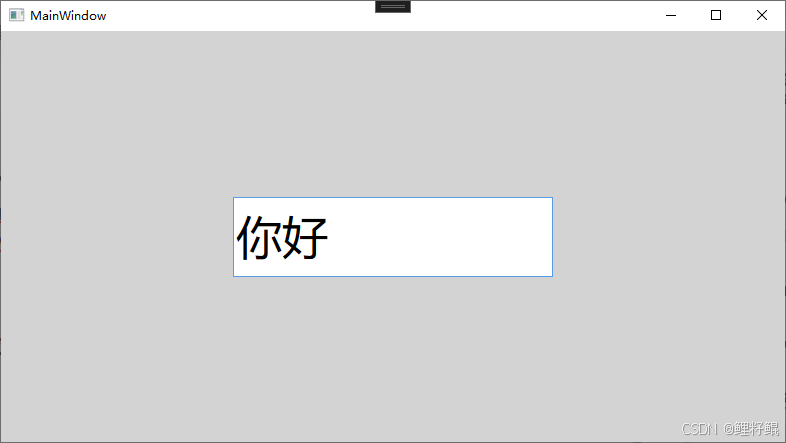
此时输入框,没有输入的限制,可以在输入框内随意输入我们想要输入的内容。
二、通过TextBox的相关事件实现输入限制
1. 通过 PreviewTextInput
事件
Occurs when this element gets text in a device-independent manner.
当此元素以 与设备(器件)无关的的方式 获取文本时发生。
或理解为 当输入框文本输入时发生。
1)实现只可输入数字
xml
<TextBox Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
PreviewTextInput="TextBox_PreviewTextInput">
</TextBox>
csharp
private void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
e.Handled = new Regex("[^0-9]+").IsMatch(e.Text);
}
e.Text
表示的是每次输入的单个字符,而非输入框内的整个内容- 当输入的Text 符合 正则表达式 则返回true
- 当
e.Handled = true
表示 不可输入,反之e.Handled = false
表示可输入 e.Handled = new Regex("[^0-9]+").IsMatch(e.Text);
表示,只可输入0-9
的数字
2)解决输入法的问题
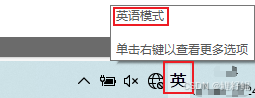
问题: 上面的案例中,当输入法为 英语模式 的时候,输入框可以控制,只可输入数字;
但是当我们切换到中文模式,输入框在中文模式 下 还是可以输入 其他字符,如何解决呢?
很简单,给TextBox 设置 InputMethod.IsInputMethodEnabled="False"
xml
<TextBox Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
PreviewTextInput="TextBox_PreviewTextInput">
</TextBox>
通过设置 InputMethod.IsInputMethodEnabled="False"
禁用了输入法,那么当焦点在该输入框的时候,就会切换回 英语模式并且禁止切换输入模式。
3)解决粘贴的问题
问题: 上面的案例中,已经基本解决了我们的需求,但是还存在一个细节问题,如果我们需要从别的地方复制了 包含字母和中文的文本信息,我们发现可以粘贴进 TextBox 内,这就造成了输入内容不符合要求。
- 解决方案:取消 TextBox所有的粘贴事件
xml
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
PreviewTextInput="TextBox_PreviewTextInput">
</TextBox>
csharp
public MainWindow()
{
InitializeComponent();
DataObject.AddPastingHandler(this.tb, TextBoxPastingEvent);
}
private void TextBoxPastingEvent(object sender, DataObjectPastingEventArgs e)
{
//取消 粘贴命令
e.CancelCommand();
}
private void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
e.Handled = new Regex("[^0-9]+").IsMatch(e.Text);
}
- 解决方案2:扩展,选择性粘贴
- 通过
e.DataObject.GetData(typeof(string)) as string;
获取得到复制的文本 - 然后可以根据具体的需求,对复制的文本 做进一步的限制
- 如下案例实现:当复制的内容不符合正则要求的时候,取消粘贴,反之,符合要求,可以粘贴
- 通过
csharp
private void TextBoxPastingEvent(object sender, DataObjectPastingEventArgs e)
{
//string nums = "0123456789";
string clipboard = e.DataObject.GetData(typeof(string)) as string;
foreach (var item in clipboard)
{
//if (nums.Contains(item))
if (new Regex("[^0-9]+").IsMatch(item.ToString()))
{
e.CancelCommand();
break;
}
}
}
4)完整实现只可输入数字
完整的案例代码如下:
xml
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
PreviewTextInput="TextBox_PreviewTextInput">
</TextBox>
csharp
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
DataObject.AddPastingHandler(this.tb, TextBoxPastingEvent);
}
private void TextBoxPastingEvent(object sender, DataObjectPastingEventArgs e)
{
//string nums = "0123456789";
string clipboard = e.DataObject.GetData(typeof(string)) as string;
foreach (var item in clipboard)
{
//if (nums.Contains(item))
if (new Regex("[^0-9]+").IsMatch(item.ToString()))
{
e.CancelCommand();
break;
}
}
}
private void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
e.Handled = new Regex("[^0-9]+").IsMatch(e.Text);
}
}
2. 通过PreviewKeyDown
或KeyDown
事件
通过PreviewKeyDown
或KeyDown
事件实现输入限制,主要是针对Key
和ModifierKeys
的处理。
PreviewKeyDown
表示当焦点在该元素上时按下某个键后发生,
- Key是C#下的枚举类型,枚举了所有的键盘键
- 如D0到D9为0到9的按键, Key.Delete代表的是删除键, Key.OemPeriod为小数点。
- ModifierKeys也是C#下的枚举类型,包括Alt、Ctrl、Shift等键。表示组合键,如Ctrl+A 中的Ctrl
csharp
// 指定修改键集
public enum ModifierKeys
{
// 摘要: No modifiers are pressed.
None = 0,
// 摘要: The ALT key.
Alt = 1,
// 摘要: The CTRL key.
Control = 2,
// 摘要: The SHIFT key.
Shift = 4,
// 摘要: The Windows logo key.
Windows = 8
}
1)实现只可输入数字
TextBox通过PreviewKeyDown
捕获按下的键值,然后判断按下的键值是否符合要求,符合要求的可以键入,反之不可以键入。
具体实现代码如下:
csharp
public static bool IsInputNumber(KeyEventArgs e)
{
//表示可以使用Ctrl+C Ctrl+V 等等组合键
//如果禁止使用组合键,也可以注释这部分代码
if (e.KeyboardDevice.Modifiers != ModifierKeys.None)
{
return true;
}
// 表示可以键入 数字0-9 以及常用的Delete,Back等辅助按键
// 这里后续也可根据具体需求去调整
if ((e.Key >= Key.D0 && e.Key <= Key.D9)
|| (e.Key >= Key.NumPad0 && e.Key <= Key.NumPad9)
|| e.Key == Key.Delete
|| e.Key == Key.Back
|| e.Key == Key.Left
|| e.Key == Key.Right)
{
return true;
}
return false;
}
xml
<TextBox Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
PreviewKeyDown="TextBox_PreviewKeyDown">
</TextBox>
注意:这里也需要设置InputMethod.IsInputMethodEnabled="False"
csharp
private void TextBox_PreviewKeyDown(object sender, KeyEventArgs e)
{
e.Handled =!IsInputNumber(e);
}
2)解决粘贴的问题
- 方式1:使用第一小节中
DataObject.AddPastingHandler
的方法实现 - 方式2:继续完善
PreviewKeyDown
事件的键入逻辑
csharp
public static bool IsInputNumber(KeyEventArgs e)
{
//表示可以使用Ctrl+C Ctrl+V 等等组合键
//如果禁止使用组合键,也可以注释这部分代码
//if (e.KeyboardDevice.Modifiers != ModifierKeys.None&& e.KeyStates != Keyboard.GetKeyStates(Key.V))
if (e.KeyboardDevice.Modifiers != ModifierKeys.None && e.Key != Key.V)
{
return true;
}
// 表示可以键入 数字0-9 以及常用的Delete,Back等辅助按键
// 这里后续也可根据具体需求去调整
if ((e.Key >= Key.D0 && e.Key <= Key.D9)
|| (e.Key >= Key.NumPad0 && e.Key <= Key.NumPad9)
|| e.Key == Key.Delete
|| e.Key == Key.Back
|| e.Key == Key.Left
|| e.Key == Key.Right)
{
return true;
}
return false;
}
if (e.KeyboardDevice.Modifiers != ModifierKeys.None && e.Key != Key.V)
- 表示只要组合键中不含
V
的 都可以使用,那么Ctrl+V
就不可以使用
- 表示只要组合键中不含
3)解决输入框右键菜单的问题
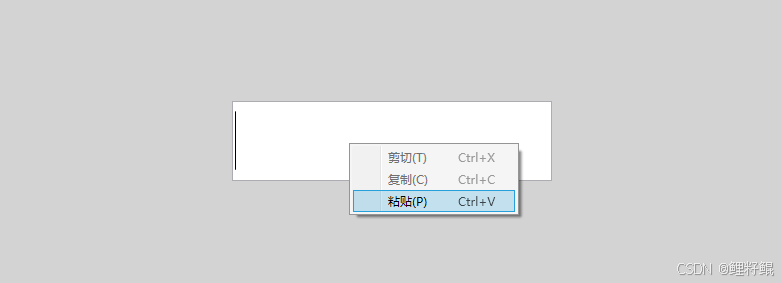
在上面的案例中虽然禁止了键盘上粘贴的问题,但是如果使用鼠标右键菜单,然后进行粘贴,还是会出现问题,因此我们需要对这部分进行处理。
处理这个问题也很简单,TextBox上设置 ContextMenu="{x:Null}"
即可
4)完整实现只可输入数字
xml
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
ContextMenu="{x:Null}"
PreviewKeyDown="TextBox_PreviewKeyDown">
</TextBox>
csharp
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void TextBox_PreviewKeyDown(object sender, KeyEventArgs e)
{
e.Handled = !IsInputNumber(e);
}
public static bool IsInputNumber(KeyEventArgs e)
{
//表示可以使用Ctrl+C Ctrl+V 等等组合键
//如果禁止使用组合键,也可以注释这部分代码
//if (e.KeyboardDevice.Modifiers != ModifierKeys.None&& e.KeyStates != Keyboard.GetKeyStates(Key.V))
if (e.KeyboardDevice.Modifiers != ModifierKeys.None && e.Key != Key.V)
{
return true;
}
// 表示可以键入 数字0-9 以及常用的Delete,Back等辅助按键
// 这里后续也可根据具体需求去调整
if ((e.Key >= Key.D0 && e.Key <= Key.D9)
|| (e.Key >= Key.NumPad0 && e.Key <= Key.NumPad9)
|| e.Key == Key.Delete
|| e.Key == Key.Back
|| e.Key == Key.Left
|| e.Key == Key.Right)
{
return true;
}
return false;
}
}
3. TextChanged
事件
TextChanged 事件:在文本元素中的内容更改的情况下发生。
通过以上的PreviewTextInput
等事件,可以很好的控制输入,但是如果TextBox的Text属性,并不是通过鼠标键盘等外在设备输入的,而是通过业务逻辑直接设置的呢?
如: 在某个按钮点击事件中设置this.textBox.Text = "hello 123!";
,那么就无法触发PreviewTextInput
和PreviewKeyDown
或KeyDown
事件,这个时候如果需要检测设置的Text
是否符合要求,就需要通过TextChanged
事件 去完成。
如: 当使用 Backspace
按键,删除TextBox中Text 的时候,可以触发PreviewKeyDown
或KeyDown
事件,但是无法触发PreviewTextInput
事件,这个时候如果需要检测设置的Text
是否符合要求,通过TextChanged
事件 去完成就比较合理。
案例:点击按钮随机文本信息并复制给TextBox的Text属性,然后通过TextChanged对不符合要求的文本信息进行处理
xml
<Grid Background="LightGray">
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
ContextMenu="{x:Null}" TextChanged="tb_TextChanged">
</TextBox>
<Button Click="Button_Click" Height="50" Width="200" Tag="" VerticalAlignment="Bottom" Background="Red" Content="test"></Button>
</Grid>
csharp
private void tb_TextChanged(object sender, TextChangedEventArgs e)
{
string text = this.tb.Text;
if (!double.TryParse(text, out var result))
{
// 假设这里的文本信息需要可转为double 类型的数据
// 直接将不符合要求的信息,显示为空
this.tb.Text = string.Empty;
}
}
private void Button_Click(object sender, RoutedEventArgs e)
{
string[] values = { "Hello 123", "12123", "daw", "1200" };
this.tb.Text = values[new Random().Next(values.Length)];
}
我们可以通过TextChanged 检测TextBox的Text 属性变化,从而做出相关的业务处理。
4. 多个事件配合使用
由以上的案例我们可知,不同的事件侧重点是不一样的,如:
PreviewTextInput
事件 用于检测输入框内每次输入的单个字符,从而对输入的字符进行控制PreviewKeyDown
事件 用于检测在输入框内所有按下的按键,包括Alt Ctrl 等按键,从而对按下的键进行控制TextChanged
事件 用于检测输入框内文本信息的变化,只要变化了就会触发该事件,从而对文本信息进行处理和控制
通过以上案例还应该知道,对于某些复杂的输入限制,是需要我们多个事件配合使用的,而非仅仅使用某一个事件就能够完成,因此我们还是需要分别了解这些事件对应的特点,这样才有利于我们做好输入控制。
配合使用案例如下:
xml
<Grid Background="LightGray">
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
PreviewTextInput="TextBox_PreviewTextInput"
PreviewKeyDown="tb_PreviewKeyDown">
</TextBox>
<Button Click="Button_Click" Height="50" Width="200" Tag="" VerticalAlignment="Bottom" Background="Red" Content="test"></Button>
</Grid>
csharp
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
DataObject.AddPastingHandler(this.tb, TextBoxPastingEvent);
}
private void TextBoxPastingEvent(object sender, DataObjectPastingEventArgs e)
{
//string nums = "0123456789";
string clipboard = e.DataObject.GetData(typeof(string)) as string;
foreach (var item in clipboard)
{
//if (nums.Contains(item))
if (new Regex("[^0-9]+").IsMatch(item.ToString()))
{
e.CancelCommand();
break;
}
}
}
private void tb_TextChanged(object sender, TextChangedEventArgs e)
{
string text = this.tb.Text;
if (!double.TryParse(text, out var result))
{
this.tb.Text = string.Empty;
}
}
private void Button_Click(object sender, RoutedEventArgs e)
{
string[] values = { "Hello 123", "12123", "daw", "1200" };
this.tb.Text = values[new Random().Next(values.Length)];
}
private void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
e.Handled = new Regex("[^0-9]+").IsMatch(e.Text);
}
private void tb_PreviewKeyDown(object sender, KeyEventArgs e)
{
//禁止使用 空格键
if (e.Key == Key.Space)
{
e.Handled = true;
}
}
}
在该案例中:
- PreviewTextInput 负责校验输入的字符,排除不符合要求的字符
- PreviewKeyDown 负责校验按下的按键,排除了空格键
- TextChanged 负责检测文本的变化
- 通过多个事件组合使用,完整详细的实现了对应的输入限制需求
三、通过附加属性实现输入限制
- (本人)推荐这种方式,灵活性高,扩展性强,对业务代码的侵入性更小
- 假如我们需要新增一类输入控制 或者灵活配置输入控制
- 我们只需新增附加属性,然后在TextBox上设置附加属性的值即可完成输入框的输入限制
- 相较于使用事件去实现输入控制,就会需要在相关事件中代码中写相关的代码,如果另一个地方需要使用输入限制,还需要重新写一遍,这种方式灵活性和可扩展性并不高,代码也得不到复用。
- 其实这种方式本质上也是基于TextBox的各种实现实现的,不过封装的更好
1. 实现只可输入数字
csharp
public class TextBoxAttachedProperties
{
public static bool GetIsOnlyNumber(DependencyObject obj)
{
return (bool)obj.GetValue(IsOnlyNumberProperty);
}
public static void SetIsOnlyNumber(DependencyObject obj, bool value)
{
obj.SetValue(IsOnlyNumberProperty, value);
}
public static readonly DependencyProperty IsOnlyNumberProperty = DependencyProperty.RegisterAttached(
"IsOnlyNumber",
typeof(bool),
typeof(TextBoxAttachedProperties),
new PropertyMetadata(false, OnIsOnlyNumberChanged));
private static void OnIsOnlyNumberChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
if (d is TextBox textBox)
{
textBox.SetValue(InputMethod.IsInputMethodEnabledProperty, false);
//textBox.SetValue(TextBox.ContextMenuProperty,null);
bool value = (bool)e.NewValue;
if (value)
{
textBox.PreviewTextInput += TextBox_PreviewTextInput;
textBox.PreviewKeyDown += TextBox_PreviewKeyDown;
DataObject.AddPastingHandler(textBox, TextBox_PastingEvent);
}
else
{
textBox.PreviewTextInput -= TextBox_PreviewTextInput;
textBox.PreviewKeyDown -= TextBox_PreviewKeyDown;
DataObject.RemovePastingHandler(textBox, TextBox_PastingEvent);
}
}
}
private static void TextBox_PreviewKeyDown(object sender, KeyEventArgs e)
{
//禁止使用 空格键
if (e.Key == Key.Space)
{
e.Handled = true;
}
}
private static void TextBox_PastingEvent(object sender, DataObjectPastingEventArgs e)
{
//取消 粘贴命令
e.CancelCommand();
}
private static void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
e.Handled = new Regex("[^0-9]+").IsMatch(e.Text);
}
}
上面的代码中主要的业务逻辑:
- 1 在实例化
PropertyMetadata
时,通过附加属性的 依赖对象获取得到 TextBox - 2 再通过TextBox 使用SetValue相关属性值
- 3 再通过TextBox 注册PreviewTextInput 等事件对输入内容进行限制
使用附加属性:
xml
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
local:TextBoxAttachedProperties.IsOnlyNumber="True">
</TextBox>
2. 其他输入限制
通过上面的案例,我们就了解了使用附加属性实现输入限制了的基本逻辑。
有了基本逻辑,遇到相关需求,只需根据实际业务需求做出相关调整即可。
- 扩展:通过正则表达式实现通用的输入限制
csharp
public class TextBoxAttachedProperties
{
public static string GetRegexPattern(DependencyObject obj)
{
return (string)obj.GetValue(RegexPatternProperty);
}
public static void SetRegexPattern(DependencyObject obj, string value)
{
obj.SetValue(RegexPatternProperty, value);
}
public static readonly DependencyProperty RegexPatternProperty = DependencyProperty.RegisterAttached(
"RegexPattern",
typeof(string),
typeof(TextBoxAttachedProperties),
new PropertyMetadata(string.Empty, OnRegexPatternChanged));
private static void OnRegexPatternChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
if (d is TextBox textBox)
{
string value = (string)e.NewValue;
if (!string.IsNullOrEmpty(value))
{
textBox.PreviewTextInput += TextBox_PreviewTextInput;
textBox.PreviewKeyDown += TextBox_PreviewKeyDown;
textBox.TextChanged += TextBox_TextChanged;
DataObject.AddPastingHandler(textBox, TextBox_PastingEvent);
}
else
{
textBox.PreviewTextInput -= TextBox_PreviewTextInput;
textBox.PreviewKeyDown -= TextBox_PreviewKeyDown;
textBox.TextChanged -= TextBox_TextChanged;
DataObject.RemovePastingHandler(textBox, TextBox_PastingEvent);
}
}
}
private static void TextBox_TextChanged(object sender, TextChangedEventArgs e)
{
//TODO 相关业务处理
// ...
}
private static void TextBox_PreviewKeyDown(object sender, KeyEventArgs e)
{
//TODO 相关业务处理
// ...
//禁止使用 空格键
//if (e.Key == Key.Space)
//{
// e.Handled = true;
//}
}
private static void TextBox_PastingEvent(object sender, DataObjectPastingEventArgs e)
{
//TODO 相关业务处理
// ...
//取消 粘贴命令
//e.CancelCommand();
}
private static void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
if (sender is TextBox textBox)
{
Regex regex = new Regex(GetRegexPattern(textBox));
//e.Handled = !regex.IsMatch(e.Text);
e.Handled = !regex.IsMatch(textBox.Text.Insert(textBox.SelectionStart, e.Text));
}
}
}
- 注意:
!regex.IsMatch(e.Text)
是检验输入框内输入的每个字符是否符合正则表达式,校验的是单个的字符!regex.IsMatch(textBox.Text.Insert(textBox.SelectionStart, e.Text));
校验的是输入当前字符后的整个文本信息,校验的是输入框的Text 属性值- 具体需要使用哪种方式进行校验,根据实际业务来定。不过一般对于PreviewTextInput 而言,校验输入的字符即可。
使用附加属性:
xml
<TextBox x:Name="tb" Height="80" Width="320" VerticalContentAlignment="Center" FontSize="46"
InputMethod.IsInputMethodEnabled="False"
local:TextBoxAttachedProperties.RegexPattern="^[0-9]+(\.[0-9]{2})?$" >
</TextBox>
3. 输入限制小结
- 以 有两位小数的正实数 为例,提供一个校验思路:
- 1 【字符的校验】通过 PreviewKeyDown 事件实现对所有按键和字符校验,实现只允许输入数字和小数点
- 或者直接在PreviewTextInput 事件中对于当前输入字符校验,实现只允许输入数字和小数点
- 2 【数据格式的校验】通过 TextChanged 事件实现对输入后格式的校验,对于不符合格式的予以提示或矫正处理
- 为啥使用 TextChanged 对输入后的格式进行校验,是因为TextChanged 可以检测文本的变化,而PreviewTextInput 只能检测文本输入的事件,对于使用Backspace 按键删除文本或逻辑赋值引起的文本变化不会触发该事件
- 注意:分清楚【字符的校验】和【数据格式的校验】的区别
csharp
private static void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
if (sender is TextBox textBox)
{
//校验输入的字符,数字或小数点可以输入
Regex regex1 = new Regex("^[0-9.]*$");
e.Handled = !regex1.IsMatch(e.Text);
}
}
csharp
private static void TextBox_TextChanged(object sender, TextChangedEventArgs e)
{
if (sender is TextBox textBox)
{
//校验输入当前字符后 文本的格式
//有两位小数的正实数:^[0-9]+(\.[0-9]{2})?$
Regex regex2 = new Regex("^[0-9]+(\\.[0-9]{2})?$");
var result = regex2.IsMatch(textBox.Text);
//这里以Debug.WriteLine 的方式予以提示,实际开发时可以显示到错误模板中
Debug.WriteLine($"textBox 当前文本信息:{textBox.Text}, 是否符合校验格式:{result} ");
}
}
以上代码的运行结果:
- 1 只可输入数字和小数点
- 2 校验信息(有两位小数的正实数)如下所示
csharp
textBox 当前文本信息:., 是否符合校验格式:False
textBox 当前文本信息:.1, 是否符合校验格式:False
textBox 当前文本信息:.12, 是否符合校验格式:False
textBox 当前文本信息:.1, 是否符合校验格式:False
textBox 当前文本信息:1, 是否符合校验格式:True
textBox 当前文本信息:1., 是否符合校验格式:False
textBox 当前文本信息:1.1, 是否符合校验格式:False
textBox 当前文本信息:1.12, 是否符合校验格式:True
更深入的数据验证,请查看 WPF 数据验证详解
结语
回到目录页: WPF 知识汇总
希望以上内容可以帮助到大家,如文中有不对之处,还请批评指正。
参考资料:
C# WPF下限制TextBox只输入数字、小数点、删除等键
WPF TextBox限制只能输入数字的两种方法
关于wpf控件的textbox只允许输入整数,小数,且只能允许输入一位小数点
C# Note12:WPF只允许数字的限制性TextBox
WPF TextBox仅输入数字(含小数)
WPF TextBox 只能输入数字,并限制输入大小
WPF TextBox 允许输入数字及字母的工具类
WPF文本框限制