1.安装jlink
下载你需要的Jlink版本
JLink-Windows-V792k-x86-64
JLink-Windows-V810k-x86-64
https://download.csdn.net/download/hmxm6/90178195
2.Visual Studio创建WPF项目
如果没有这个选项请看
https://blog.csdn.net/hmxm6/article/details/132914337
创建完成后
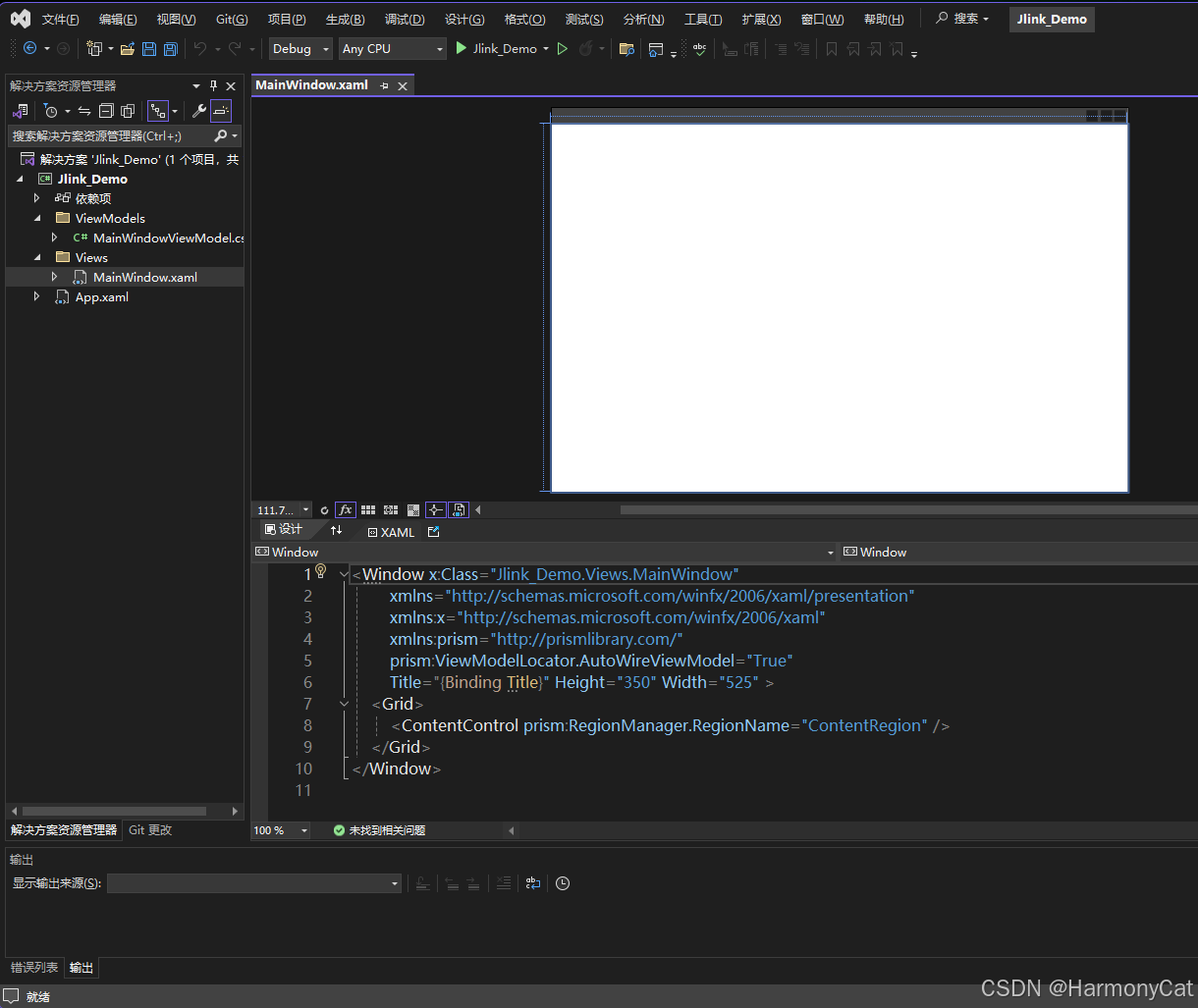
添加一些界面
MainWindow.xaml
html
<Window x:Class="Jlink_Demo.Views.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:prism="http://prismlibrary.com/"
prism:ViewModelLocator.AutoWireViewModel="True"
Title="{Binding Title}" Height="350" Width="525" >
<Grid>
<StackPanel Width="525" Height="350" HorizontalAlignment="Left">
<Button Width="100" Height="30" Command="{Binding connectJlink}">连接Jlink</Button>
<Button Width="100" Height="30" Command="{Binding closeJlink}">关闭Jlink连接</Button>
<TextBlock Width="200" Text="{Binding JLINKVersion}" FontSize="20"></TextBlock>
<Button Width="100" Height="30" Command="{Binding connectMCU}">连接MCU</Button>
<TextBlock Width="200" Text="{Binding HardwareId}" FontSize="20"></TextBlock>
<Button Width="100" Height="30" Command="{Binding resetMCU}">复位单片机</Button>
<Button Width="100" Height="30" Command="{Binding readFlash}">读取Flash</Button>
<Button Width="100" Height="30" Command="{Binding writeFlash}">写入Flash</Button>
<TextBox Width="100" FontSize="20" Text="{Binding FlashData}"></TextBox>
</StackPanel>
</Grid>
</Window>
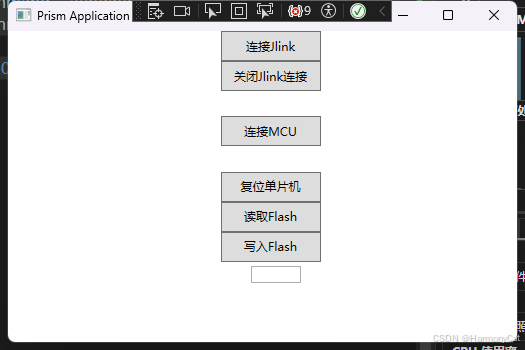
添加MVVM变量
MainWindowViewModel.cs
csharp
using Prism.Mvvm;
namespace Jlink_Demo.ViewModels
{
public class MainWindowViewModel : BindableBase
{
// 标题 MVVM
private string _title = "Prism Application";
public string Title
{
get { return _title; }
set { SetProperty(ref _title, value); }
}
// Jlink版本 MVVM
private string _JLINKVersion = "Jlink版本:";
public string JLINKVersion
{
get { return _JLINKVersion; }
set { SetProperty(ref _JLINKVersion, value); }
}
// 硬件ID MVVM
private string _HardwareId = "硬件ID:";
public string HardwareId
{
get { return _HardwareId; }
set { SetProperty(ref _HardwareId, value); }
}
// Flash数据 MVVM
private int _FlashData = 0;
public int FlashData
{
get { return _FlashData; }
set { SetProperty(ref _FlashData, value); }
}
public MainWindowViewModel()
{
}
}
}
3.加入jlink dll
在Jlink安装目录下有两个dll文件,请选择对应dll文件
把文件放入项目
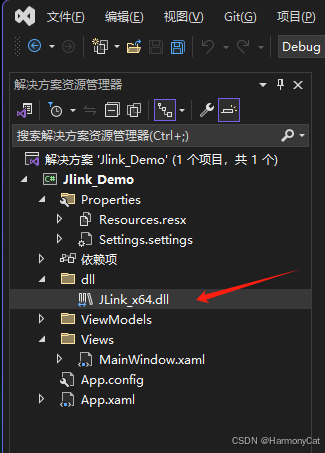
文件属性中,选择始终复制
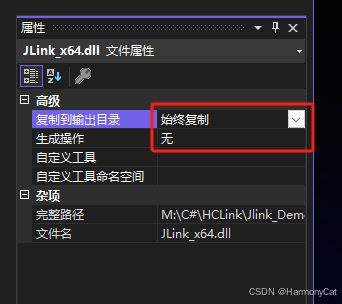
4.添加jlink工具类
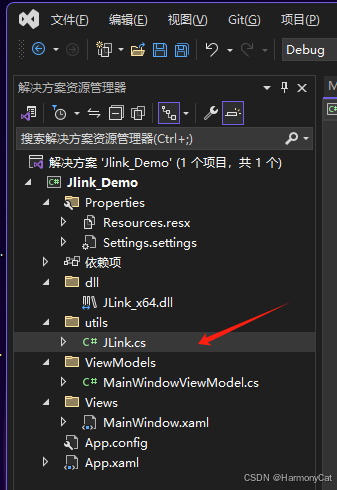
csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading.Tasks;
namespace Jlink_Demo.utils
{
public static partial class JLink
{
/// <summary>
/// 打开JLINK设备
/// </summary>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_Open();
/// <summary>
/// 关闭JLINK设备
/// </summary>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_Close();
/// <summary>
/// 连接设备
/// </summary>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_Connect();
/// <summary>
/// 开启RTT
/// </summary>
/// <param name="terminal"></param>
/// <param name="size"></param>
[DllImport("JLink_x64.dll", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINK_RTTERMINAL_Control(UInt32 CMD, UInt32 size);
/// <summary>
/// 从RTT回读数据
/// </summary>
/// <param name="terminal"></param>
/// <param name="rxBuffer"></param>
/// <param name="len"></param>
[DllImport("JLink_x64.dll", CharSet = CharSet.Auto, SetLastError = true, ExactSpelling = true)]
public static extern void JLINK_RTTERMINAL_Read(int terminal, [Out(), MarshalAs(UnmanagedType.LPArray)] byte[] rxBuffer, UInt32 size);
static string gbk_ansi(string str)
{
string keyword;
byte[] buffer = Encoding.GetEncoding("GB2312").GetBytes(str);
keyword = Encoding.UTF8.GetString(buffer);
return keyword;
}
public static string JLINKARM_ReadRTT_String()
{
try
{
byte[] aa = new byte[1000];
JLINK_RTTERMINAL_Read(0, aa, 1000);
ASCIIEncoding kk = new ASCIIEncoding();
//使用UTF-8编码
UTF8Encoding uu = new UTF8Encoding();
string ss = uu.GetString(aa);
if (ss.Length > 1)
{
Console.Write(ss);
}
return ss;
}
catch (Exception ex)
{
}
return String.Empty;
}
/// <summary>
/// 写数据到RTT
/// </summary>
/// <param name="terminal"></param>
/// <param name="txBuffer"></param>
/// <param name="len"></param>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINK_RTTERMINAL_Write(int terminal, [In(), MarshalAs(UnmanagedType.LPArray)] byte[] txBuffer, UInt32 size);
/// <summary>
/// 系统复位
/// </summary>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_Reset();
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_GoAllowSim();
/// <summary>
/// 执行程序
/// </summary>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_Go();
/// <summary>
/// 中断程序执行
/// </summary>
/// <returns></returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool JLINKARM_Halt();
/// <summary>
/// 单步执行
/// </summary>
/// <returns></returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool JLINKARM_Step();
/// <summary>
/// 清除错误信息
/// </summary>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ClrError();
/// <summary>
/// 设置JLINK接口速度
/// </summary>
/// <param name="speed"></param>
/// <remarks>0为自动调整</remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_SetSpeed(int speed);
/// <summary>
/// 设置JTAG为最高速度
/// </summary>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_SetMaxSpeed()
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt16 JLINKARM_GetSpeed()
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_GetVoltage()
;
/// <summary>
/// 当前MCU是否处于停止状态
/// </summary>
/// <returns></returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool JLINKARM_IsHalted()
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool JLINKARM_IsConnected()
;
/// <summary>
/// JLINK是否已经可以操作了
/// </summary>
/// <returns></returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern bool JLINKARM_IsOpen()
;
/// <summary>
/// 取消程序断点
/// </summary>
/// <param name="index">断点序号</param>
/// <remarks>配合JLINKARM_SetBP()使用</remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ClrBP(UInt32 index)
;
/// <summary>
/// 设置程序断点
/// </summary>
/// <param name="index">断点序号</param>
/// <param name="addr">目标地址</param>
/// <remarks>建议使用JLINKARM_SetBPEx()替代</remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_SetBP(UInt32 index, UInt32 addr)
;
/// <summary>
/// 取消程序断点
/// </summary>
/// <param name="handle"></param>
/// <remarks>配合JLINKARM_SetBPEx()使用</remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ClrBPEx(int handle)
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
private static extern int JLINKARM_SetWP(UInt32 addr, UInt32 addrmark, UInt32 dat, UInt32 datmark, byte ctrl, byte ctrlmark)
;
/// <summary>
/// 取消数据断点
/// </summary>
/// <param name="handle"></param>
/// <remarks>配合JLINKARM_SetWP()使用</remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ClrWP(int handle)
;
/// <summary>
/// 写入一段数据
/// </summary>
/// <param name="addr"></param>
/// <param name="size"></param>
/// <param name="buf"></param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_WriteMem(UInt32 addr, UInt32 size, byte[] buf)
;
/// <summary>
/// 读取一段数据
/// </summary>
/// <param name="addr"></param>
/// <param name="size"></param>
/// <param name="buf"></param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ReadMem(UInt32 addr, UInt32 size, [Out(), MarshalAs(UnmanagedType.LPArray)] byte[] buf)
;
/// <summary>
/// 从调试通道获取一串数据
/// </summary>
/// <param name="buf"></param>
/// <param name="size">需要获取的数据长度</param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ReadDCCFast([Out(), MarshalAs(UnmanagedType.LPArray)] UInt32[] buf, UInt32 size)
;
/// <summary>
/// 从调试通道获取一串数据
/// </summary>
/// <param name="buf"></param>
/// <param name="size">希望获取的数据长度</param>
/// <param name="timeout"></param>
/// <returns>实际获取的数据长度</returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_ReadDCC([Out(), MarshalAs(UnmanagedType.LPArray)] UInt32[] buf, UInt32 size, int timeout)
;
/// <summary>
/// 向调试通道写入一串数据
/// </summary>
/// <param name="buf"></param>
/// <param name="size">需要写入的数据长度</param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_WriteDCCFast(UInt32[] buf, UInt32 size)
;
/// <summary>
/// 向调试通道写入一串数据
/// </summary>
/// <param name="buf"></param>
/// <param name="size">希望写入的数据长度</param>
/// <param name="timeout"></param>
/// <returns>实际写入的数据长度</returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_WriteDCC(UInt32[] buf, UInt32 size, int timeout)
;
/// <summary>
/// 获取JLINK的DLL版本号
/// </summary>
/// <returns></returns>
/// <remarks>使用10进制数表示</remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_GetDLLVersion()
;
/// <summary>
/// 执行命令
/// </summary>
/// <param name="oBuffer"></param>
/// <param name="a"></param>
/// <param name="b"></param>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ExecCommand([In(), MarshalAs(UnmanagedType.LPArray)] byte[] oBuffer, int a, int b);
/// <summary>
/// 选择接口,0是JTAG 1是SWD
/// </summary>
/// <param name="type"></param>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_TIF_Select(int type);
/// <summary>
/// 获取JLINK的固件版本号
/// </summary>
/// <returns></returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_GetHardwareVersion()
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
private static extern void JLINKARM_GetFeatureString([Out(), MarshalAs(UnmanagedType.LPArray)] byte[] oBuffer)
;
[DllImport("JLink_x64.dll", CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
private static extern void JLINKARM_GetOEMString([Out(), MarshalAs(UnmanagedType.LPArray)] byte[] oBuffer)
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_SetLogFile([In(), MarshalAs(UnmanagedType.LPArray)] byte[] oBuffer)
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern StringBuilder JLINKARM_GetCompileDateTime()
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_GetSN()
;
/// <summary>
/// 获取当前MCU的ID号
/// </summary>
/// <returns></returns>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern UInt32 JLINKARM_GetId()
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_ReadMemU32(UInt32 addr, UInt32 leng, ref UInt32 buf, ref byte status)
;
/// <summary>
/// 写入32位的数据
/// </summary>
/// <param name="addr"></param>
/// <param name="dat"></param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_WriteU32(UInt32 addr, UInt32 dat)
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
private static extern void JLINKARM_ReadMemU16(UInt32 addr, UInt32 leng, ref UInt16 buf, ref byte status)
;
/// <summary>
/// 写入16位的数据
/// </summary>
/// <param name="addr"></param>
/// <param name="dat"></param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_WriteU16(UInt32 addr, UInt16 dat)
;
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
private static extern void JLINKARM_ReadMemU8(UInt32 addr, UInt32 leng, ref byte buf, ref byte status)
;
/// <summary>
/// 写入8位的数据
/// </summary>
/// <param name="addr"></param>
/// <param name="dat"></param>
/// <remarks></remarks>
[DllImport("JLink_x64.dll", CallingConvention = CallingConvention.Cdecl, CharSet = CharSet.Ansi, SetLastError = true, ExactSpelling = true)]
public static extern void JLINKARM_WriteU8(UInt32 addr, byte dat)
;
}
}
5.编写按钮事件
MainWindowViewModel.cs
连接c并读取JLink版本号
csharp
private DelegateCommand _connectJlink;
public DelegateCommand connectJlink => _connectJlink ?? (_connectJlink = new DelegateCommand(ExecuteConnectJlink));
void ExecuteConnectJlink()
{
// 1.打开jlink
JLink.JLINKARM_Open();
// 读取jlink版本号
var dllVersion = JLink.JLINKARM_GetDLLVersion();
var hardVersion = JLink.JLINKARM_GetHardwareVersion();
this.JLINKVersion = "JLink版本号:" + hardVersion;
}
关闭连接JLink
csharp
private DelegateCommand _closeJlink;
public DelegateCommand closeJlink => _closeJlink ?? (_closeJlink = new DelegateCommand(ExecuteCloseJlink));
void ExecuteCloseJlink()
{
JLink.JLINKARM_Close();// 关闭jlink
this.JLINKVersion = "";
this.HardwareId = "";
}
连接单片机
csharp
private DelegateCommand _connectMCU;
public DelegateCommand connectMCU => _connectMCU ?? (_connectMCU = new DelegateCommand(ExecuteConnectMCU));
void ExecuteConnectMCU()
{
if (JLink.JLINKARM_IsOpen() == true)
{
JLink.JLINKARM_TIF_Select(1);// SWD连接方式
// 连接STM32F407VG
JLink.JLINKARM_ExecCommand(System.Text.Encoding.UTF8.GetBytes("device = STM32F407VG"), 0, 0);
JLink.JLINKARM_SetSpeed(4000);
Thread.Sleep(100);//休眠时间
var id = JLink.JLINKARM_GetId();
// 判断单片机连接状态
if (JLink.JLINKARM_IsConnected() == true)
{
// 通过MVVM设置界面上的单片机ID
this.HardwareId = "SUCCESS id:" + id;
}
else
{
this.HardwareId = "ERROR";
}
}
else
{
MessageBox.Show("请先打开烧录器");
}
}
复位单片机
csharp
private DelegateCommand _resetMCU;
public DelegateCommand resetMCU => _resetMCU ?? (_resetMCU = new DelegateCommand(ExecuteResetMCU));
void ExecuteResetMCU()
{
JLink.JLINKARM_Reset();
JLink.JLINKARM_Go();
}
读取Flash数据
csharp
private DelegateCommand _readFlash;
public DelegateCommand readFlash => _readFlash ?? (_readFlash = new DelegateCommand(ExecuteReadFlash));
void ExecuteReadFlash()
{
UInt32 dataBuff = 0;
byte status = 0;
if (JLink.JLINKARM_IsConnected() == true)
{
// 读取Flash数据
JLink.JLINKARM_ReadMemU32(0x080F0000, 1, ref dataBuff, ref status);
// 判断读取状态
if (status == 0)
{
// 通过MVVM设置界面上的值
this.FlashData = (int)dataBuff;
}
else
{
this.FlashData = 0;
}
}
}
写入Flash数据
csharp
private DelegateCommand _writeFlash;
public DelegateCommand writeFlash => _writeFlash ?? (_writeFlash = new DelegateCommand(ExecuteWriteFlash));
void ExecuteWriteFlash()
{
if (JLink.JLINKARM_IsConnected() == true)
{
JLink.JLINKARM_WriteU32(0x080D0000, (uint)this.FlashData);
}
}
完整代码MainWindowViewModel.cs
csharp
using Jlink_Demo.utils;
using Prism.Commands;
using Prism.Mvvm;
using System;
using System.Threading;
using System.Windows;
namespace Jlink_Demo.ViewModels
{
public class MainWindowViewModel : BindableBase
{
private string _title = "Prism Application";
public string Title
{
get { return _title; }
set { SetProperty(ref _title, value); }
}
private string _JLINKVersion = "Jlink版本:";
public string JLINKVersion
{
get { return _JLINKVersion; }
set { SetProperty(ref _JLINKVersion, value); }
}
private string _HardwareId = "硬件ID:";
public string HardwareId
{
get { return _HardwareId; }
set { SetProperty(ref _HardwareId, value); }
}
private int _FlashData = 0;
public int FlashData
{
get { return _FlashData; }
set { SetProperty(ref _FlashData, value); }
}
public MainWindowViewModel()
{
}
private DelegateCommand _connectJlink;
public DelegateCommand connectJlink => _connectJlink ?? (_connectJlink = new DelegateCommand(ExecuteConnectJlink));
void ExecuteConnectJlink()
{
// 1.打开jlink
JLink.JLINKARM_Open();
// 读取jlink版本号
var dllVersion = JLink.JLINKARM_GetDLLVersion();
var hardVersion = JLink.JLINKARM_GetHardwareVersion();
this.JLINKVersion = "JLink版本号:" + hardVersion;
}
private DelegateCommand _closeJlink;
public DelegateCommand closeJlink => _closeJlink ?? (_closeJlink = new DelegateCommand(ExecuteCloseJlink));
void ExecuteCloseJlink()
{
JLink.JLINKARM_Close();// 关闭jlink
this.JLINKVersion = "";
this.HardwareId = "";
}
private DelegateCommand _resetMCU;
public DelegateCommand resetMCU => _resetMCU ?? (_resetMCU = new DelegateCommand(ExecuteResetMCU));
void ExecuteResetMCU()
{
JLink.JLINKARM_Reset();
JLink.JLINKARM_Go();
}
private DelegateCommand _connectMCU;
public DelegateCommand connectMCU => _connectMCU ?? (_connectMCU = new DelegateCommand(ExecuteConnectMCU));
void ExecuteConnectMCU()
{
if (JLink.JLINKARM_IsOpen() == true)
{
JLink.JLINKARM_TIF_Select(1);// SWD连接方式
// 连接STM32F407VG
JLink.JLINKARM_ExecCommand(System.Text.Encoding.UTF8.GetBytes("device = STM32F407VG"), 0, 0);
JLink.JLINKARM_SetSpeed(4000);
Thread.Sleep(100);//休眠时间
var id = JLink.JLINKARM_GetId();
// 判断单片机连接状态
if (JLink.JLINKARM_IsConnected() == true)
{
this.HardwareId = "SUCCESS id:" + id;
}
else
{
this.HardwareId = "ERROR";
}
}
else
{
MessageBox.Show("请先打开烧录器");
}
}
private DelegateCommand _readFlash;
public DelegateCommand readFlash => _readFlash ?? (_readFlash = new DelegateCommand(ExecuteReadFlash));
void ExecuteReadFlash()
{
UInt32 dataBuff = 0;
byte status = 0;
if (JLink.JLINKARM_IsConnected() == true)
{
JLink.JLINKARM_ReadMemU32(0x080D0000, 1, ref dataBuff, ref status);
if (status == 0)
{
this.FlashData = (int)dataBuff;
}
else
{
this.FlashData = 0;
}
}
}
private DelegateCommand _writeFlash;
public DelegateCommand writeFlash => _writeFlash ?? (_writeFlash = new DelegateCommand(ExecuteWriteFlash));
void ExecuteWriteFlash()
{
if (JLink.JLINKARM_IsConnected() == true)
{
JLink.JLINKARM_WriteU32(0x080D0000, (uint)this.FlashData);
}
}
}
}
代码示例