在搭建React项目时候,遇到了Echart官方文档中没有的水球图,此时该如何配置并将它显示到项目中呢?
目录
一、拓展网站
echarts图表集
"ECharts图表集" 网站页面。这个网站是一个ECharts的demo集和社区,用户可以在这里分享和查看各种ECharts的可视化作品。
我们的需要的水球图就在里面,搜索并在手机上看一分钟广告。我们终于拿到了水球图!
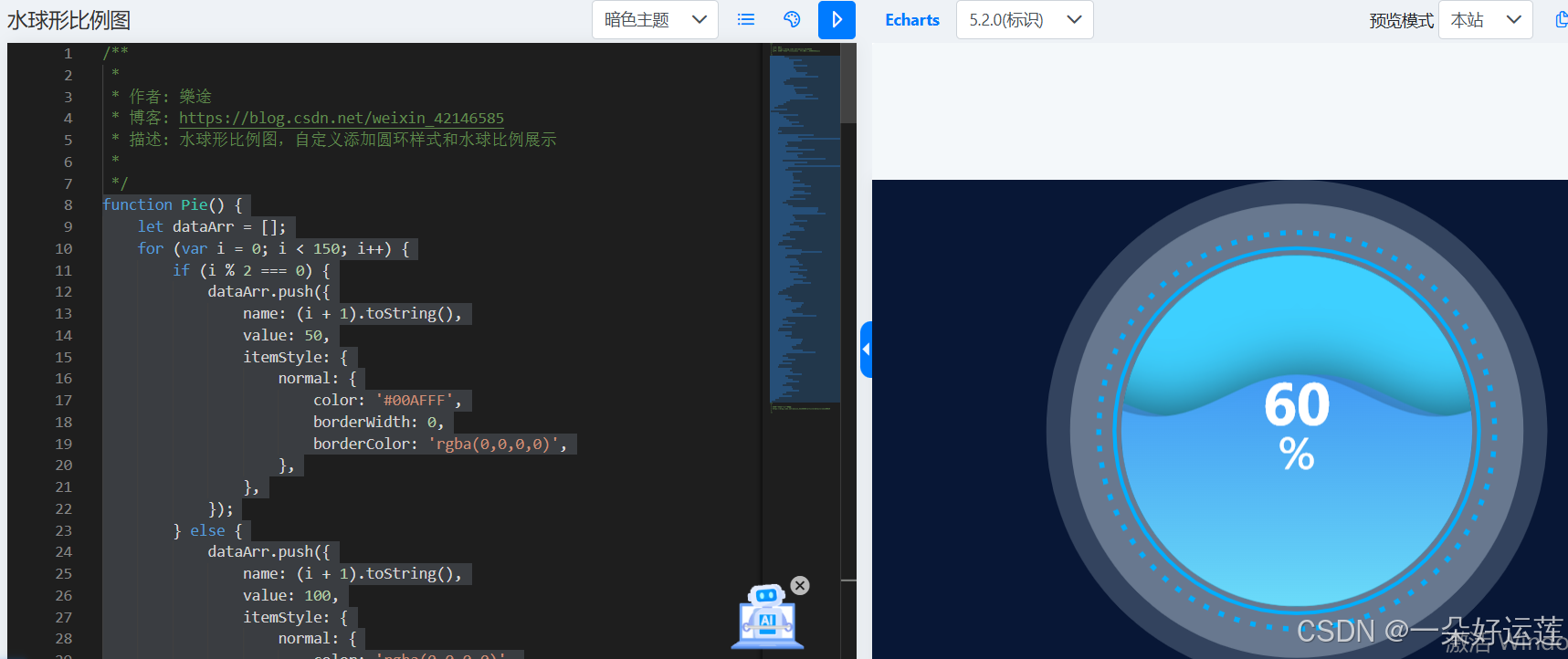
二、安装
npm install echarts
npm install echarts-liquidfill
echarts-liquidfill 是一个专门为 Apache ECharts 设计的扩展插件,用于创建水球图(Liquid Fill Chart)。这种图表通常用于以百分比形式展示数据,并具有动态波纹效果。
官网:
https://www.npmjs.com/package/echarts-liquidfill
三、React中引入
1、在components文件夹下新建一个组件
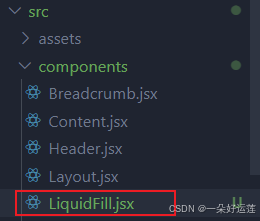
2、在组件中引入
javascript
import React from "react";
import * as echarts from "echarts";
import "echarts-liquidfill";
1;
class LiquidFill extends React.Component {
componentDidMount() {
this.chart = echarts.init(this.echartsReactNode);
this.chart.setOption(this.getOptions());
}
componentDidUpdate() {
this.chart.setOption(this.getOptions());
}
getOptions = () => {
const Pie = () => {
let dataArr = [];
for (var i = 0; i < 150; i++) {
if (i % 2 === 0) {
dataArr.push({
name: (i + 1).toString(),
value: 50,
itemStyle: {
normal: {
color: "#00AFFF",
borderWidth: 0,
borderColor: "rgba(0,0,0,0)",
},
},
});
} else {
dataArr.push({
name: (i + 1).toString(),
value: 100,
itemStyle: {
normal: {
color: "rgba(0,0,0,0)",
borderWidth: 0,
borderColor: "rgba(0,0,0,0)",
},
},
});
}
}
return dataArr;
};
const option = {
backgroundColor: "#081736",
series: [
{
type: "liquidFill",
radius: "70%",
center: ["50%", "50%"],
data: [0.6, { value: 0.6, direction: "left" }],
backgroundStyle: {
borderWidth: 1,
color: "rgba(62, 208, 255, 1)",
},
amplitude: "6%",
color: [
{
type: "linear",
x: 0,
y: 0,
x2: 0,
y2: 1,
colorStops: [
{ offset: 1, color: "#6CDEFC" },
{ offset: 0, color: "#429BF7" },
],
globalCoord: false,
},
],
label: {
normal: {
formatter: 0.6 * 100 + "\n{d|%}",
rich: {
d: {
fontSize: 36,
},
},
textStyle: {
fontSize: 48,
color: "#fff",
},
},
},
outline: {
show: false,
},
},
// ... 其他 series 配置
{
type: "pie",
zlevel: 0,
silent: true,
radius: ["78%", "80%"],
z: 1,
label: {
normal: {
show: false,
},
},
labelLine: {
normal: {
show: false,
},
},
data: Pie(),
},
],
};
return option;
};
render() {
return (
<div
ref={(node) => (this.echartsReactNode = node)}
style={{ width: "100%", height: "400px" }}
/>
);
}
}
export default LiquidFill;
3、使用水波球组件
javascript
import LiquidFill from "../../../components/LiquidFill";
function MedicalEquipmentStatistics() {
return (
<div className="App">
<LiquidFill />
</div>
);
}
export default MedicalEquipmentStatistics;
完成效果(没显示的重启一下项目):
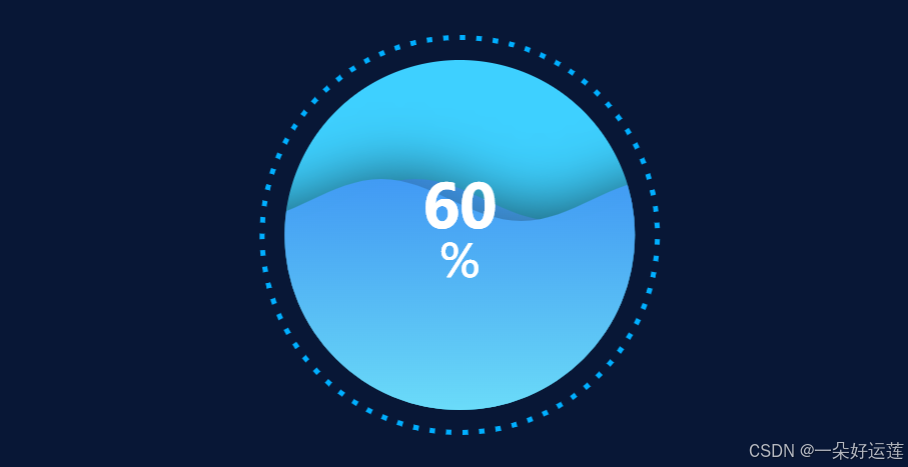