首先,在项目的 components
目录下创建一个新的文件夹,例如 date-picker
,并在其中创建以下文件:
date-picker.wxml
date-picker.wxss
date-picker.js
date-picker.json
2.date-picker.wxml
html
<view class="date-picker">
<t-cell
title="{{title}}"
hover
note="{{dateText || '请选择'}}"
arrow
bindtap="showPicker"
t-class="panel-item"
/>
<t-date-time-picker
auto-close
title="选择日期"
visible="{{visible}}"
mode="date"
default-value="{{date}}"
format="YYYY-MM-DD"
start="{{start}}"
end="{{end}}"
filter="{{filter}}"
popup-props="{{popupProps}}"
bindchange="onConfirm"
bindpick="onColumnChange"
bindcancel="hidePicker"
bindclose="handleClose"
/>
</view>
date-picker.js
TypeScript
Component({
properties: {
title: { type: String, value: '请选择日期' }, // 标题
date: { type: String, value: '' }, // 默认为空,将在 attached 中设置
start: { type: String, value: '' },
},
data: {
visible: false, // 选择器是否可见
dateText: '', // 显示的日期文本
// 指定选择区间起始值
end: '2030-09-09 12:12:12',
filter(type, options) {
if (type === 'year') {
return options.sort((a, b) => b.value - a.value);
}
return options;
},
popupProps: {
usingCustomNavbar: true,
},
},
observers: {
'date': function (newVal) {
this.setData({ dateText: newVal || '' });
},
'start': function(newVal) {
if (newVal && this.data.end && newVal > this.data.end) {
this.setData({ end: newVal });
}
},
'end': function(newVal) {
if (newVal && this.data.start && newVal < this.data.start) {
wx.showToast({
title: '结束日期必须大于或等于开始日期',
icon: 'none'
});
this.setData({ end: '' });
}
}
},
methods: {
// 显示选择器
showPicker() {
this.setData({ visible: true });
},
// 隐藏选择器
hidePicker() {
this.setData({ visible: false });
},
// 确认选择
onConfirm(e) {
const selectedDate = e.detail.value;
if (this.data.title === '结束日期' && this.data.start && selectedDate < this.data.start) {
wx.showToast({
title: '结束日期必须大于或等于开始日期',
icon: 'none'
});
return;
}
this.setData({
date: selectedDate,
visible: false
});
this.triggerEvent('datechange', selectedDate); // 触发事件传递选择的日期
},
// 列变化(可选)
onColumnChange(e) {
// 处理列变化逻辑(可选)
},
// 关闭处理(可选)
handleClose() {
this.hidePicker();
}
}
});
date-picker.json
TypeScript
{
"component": true,
"usingComponents": {
"t-cell": "tdesign-miniprogram/cell/cell",
"t-date-time-picker": "tdesign-miniprogram/date-time-picker/date-time-picker"
}
}
使用组件
在需要使用日期选择器的页面中,引入并使用该组件。
开始时间为今天时间,结束时间大于等于开始时间。
TypeScript
{
"usingComponents": {
"date-picker":"/components/date-picker/date-picker",
}
}
html
<view class="title">
<date-picker title="开始日期" start="{{today}}" date="{{startDate}}" bind:datechange="onStartDateChange" />
</view>
<view class="title">
<date-picker title="结束日期" start="{{startDate}}" date="{{endDate}}" bind:datechange="onEndDateChange" />
</view>
javascript
data:{
//日期选择
startDate: '',
endDate: '',
today: '', // 在 onLoad 中设置
}
onLoad(options) {
this.setTodayDate();
},
//日期选择
setTodayDate() {
const today = new Date();
const year = today.getFullYear();
const month = String(today.getMonth() + 1).padStart(2, '0');
const day = String(today.getDate()).padStart(2, '0');
const formattedDate = `${year}-${month}-${day}`;
this.setData({ today: formattedDate, startDate: formattedDate });
},
onStartDateChange(e) {
this.setData({ startDate: e.detail });
},
onEndDateChange(e) {
this.setData({ endDate: e.detail });
},
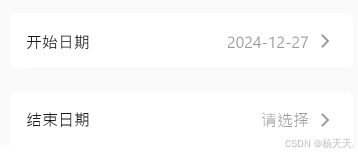