搜索框-历史搜索记录
实现功能:
- 历史搜索记录
- 展示-历史搜索记录展示10条
- 点击跳转-点击历史搜索记录可同步到搜索框并自动搜索
- 全部删除-可一次性全部删除历史搜索记录
引言
在微信小程序中,一个带有历史记录的搜索框可以极大地提升用户体验。用户可以快速访问他们之前的搜索,而不必重新输入。本文将详细介绍如何在"我的咖啡店"小程序中实现这一功能。
实现搜索框与历史记录功能
1. 页面初始数据设置
在search.js
中,我们首先定义了页面的初始数据,包括搜索历史列表historyList
和当前搜索关键词keyword
。
javascript
// pages/search/search.js
Page({
data: {
historyList: [],
keyword: ""
},
// ...其他函数
});
2. 加载搜索历史
在页面加载时,我们从本地存储中获取搜索历史数组,并更新页面数据。
javascript
// pages/search/search.js
onLoad(options) {
let searchKeyArr = wx.getStorageSync('searchKeyArr') || [];
this.setData({ historyList: searchKeyArr });
wx.hideLoading();
},
3. 搜索关键词处理
当用户在搜索框中输入关键词并触发搜索时,我们将关键词添加到搜索历史列表中,并同步到本地存储。
javascript
// pages/search/search.js
onSearch() {
let currentKeyword = this.data.keyword.trim();
let hisArr = this.data.historyList || [];
if (currentKeyword && !hisArr.includes(currentKeyword)) {
hisArr.unshift(currentKeyword);
}
hisArr = [...new Set(hisArr)].slice(0, 10);
this.setData({
historyList: hisArr,
keyword: ''
});
wx.setStorageSync('searchKeyArr', hisArr);
},
4. 清空搜索历史
用户可以一次性清空所有搜索历史记录。
javascript
// pages/search/search.js
clearHistory() {
this.setData({
historyList: []
});
wx.removeStorageSync('searchKeyArr');
},
5. 点击历史记录触发搜索
当用户点击历史记录中的某个关键词时,该关键词将同步到搜索框并自动触发搜索。
javascript
// pages/search/search.js
onKeywordTap(e) {
this.setData({
keyword: e.currentTarget.dataset.keyword
});
this.onSearch();
},
6. WXML 结构
在search.wxml
中,我们使用<van-search>
组件创建搜索框,并使用<view>
组件创建历史记录列表。
XML
<!--pages/search/search.wxml-->
<view class="search">
<van-search value="{{ keyword }}" ...>
<view slot="action" bind:tap="onSearch">搜索</view>
</van-search>
<view class="history" wx:if="{{historyList.length}}">
<view class="title">
<view class="text">搜索历史</view>
<view class="remove" bindtap="clearHistory">
<van-icon name="delete-o" size="21" />
</view>
</view>
<view class="content">
<view class="item" wx:for="{{historyList}}" wx:key="index" data-keyword="{{item}}" bindtap="onKeywordTap">{{item}}</view>
</view>
</view>
</view>
7. WXSS 样式
在search.wxss
中,我们定义了搜索框和历史记录列表的样式。
css
/* pages/search/search.wxss */
/* 样式定义... */
结语
通过上述步骤,我们实现了一个带有历史记录的搜索框,用户可以查看历史记录,点击记录进行搜索,或者删除单个或全部历史记录。这个功能不仅提升了用户体验,也使得搜索过程更加便捷。希望这篇文章能帮助你在开发自己的微信小程序时,更好地实现搜索框和历史记录管理功能。
完整代码
search.js
javascript
// pages/search/search.js
Page({
// 页面的初始数据
data: {
// 搜索历史列表,初始化为空数组
historyList: [],
// 搜索关键词,初始化为空字符串
keyword: ""
},
// 生命周期函数--监听页面加载
onLoad(options) {
// 尝试从本地存储中获取搜索历史数组,如果不存在则默认为空数组
let searchKeyArr = wx.getStorageSync('searchKeyArr') || null;
// 更新搜索历史数据
this.setData({ historyList: searchKeyArr });
// 关闭加载提示
wx.hideLoading();
},
// 输入框内容改变时触发
onChange(e) {
// console.log(e);
// 更新页面数据中的 keyword 为输入框的当前值
this.setData({
keyword: e.detail
})
},
// 确认搜索
onSearch() {
// 获取输入的关键词并去除两端空格
let currentKeyword = this.data.keyword.trim();
// 在控制台输出当前的搜索关键词
console.log(currentKeyword);
// 获取当前的搜索历史列表,如果未定义则初始化为空数组
let hisArr = this.data.historyList || [];
// 将当前关键词添加到历史列表的开头
if (currentKeyword && currentKeyword !== '' && !hisArr.includes(currentKeyword)) {
hisArr.unshift(currentKeyword);
}
// 使用 Set 去除重复的关键词,然后展开到数组中
hisArr = [...new Set(hisArr)];
// 截取数组的前10项,限制历史列表的长度
hisArr = hisArr.slice(0, 10);
// 更新页面数据中的搜索历史列表
this.setData({
historyList: hisArr,
keyword: '' // 清空关键词输入
});
// 同步搜索历史列表到本地存储
wx.setStorageSync('searchKeyArr', hisArr);
},
// 清空历史
clearHistory() {
// 清空历史列表
this.setData({
historyList: []
});
// 同步清空历史列表到本地存储
wx.removeStorageSync('searchKeyArr');
},
// 点击历史关键词时触发的函数
onKeywordTap(e) {
// 赋值为 被点击的历史关键词
this.setData({
keyword: e.currentTarget.dataset.keyword
});
// 可以在这里直接触发搜索,或者让用户手动触发
this.onSearch();
},
});
search.json
javascript
{
"usingComponents": {
"van-icon": "@vant/weapp/icon/index"
}
}
search.wxml
XML
<!--pages/search/search.wxml-->
<view class="search">
<!-- 搜索框开始 -->
<van-search value="{{ keyword }}" shape="round" background="#fff" placeholder="{{defaultSearchHint}}" use-action-slot show-action bind:change="onChange" bind:search="onSearch" bind:cancel="onCancel" bind:clear="onClear">
<view slot="action" bind:tap="onSearch" style="padding:0 30rpx">搜索</view>
</van-search>
<!-- 搜索框结束 -->
<!-- 搜索历史视图 -->
<view class="history" wx:if="{{historyList.length}}">
<!-- 标题 -->
<view class="title">
<view class="text">搜索历史</view>
<view class="remove" bindtap="clearHistory">
<!-- 使用 van-icon 组件显示删除图标 -->
<van-icon name="delete-o" size="21" />
<!-- 清空历史的文本 -->
<!-- <text class="font">清空历史</text> -->
</view>
</view>
<!-- 内容区域 -->
<view class="content">
<!-- 循环显示搜索历史中的每一条记录 -->
<view class="item" wx:for="{{historyList}}" wx:key="index" data-keyword="{{item}}" bindtap="onKeywordTap">{{item}}</view>
</view>
</view>
</view>
search.wxss
css
/* pages/search/search.wxss */
/* 搜索历史容器样式 */
.history {
padding: 10rpx;
}
/* 搜索历史标题样式 */
.history .title {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
align-items: center;
font-size: 30rpx;
/* 灰色文字 */
color: #666;
}
/* 搜索历史标题中的文本样式 */
.history .title .text {
font-size: 25rpx;
}
/* 搜索历史标题中的清空按钮样式 */
.history .title .remove {
/* 较浅的灰色文字 */
color: #999;
display: flex;
align-items: center;
}
/* 搜索历史标题中的清空历史文本样式 */
.history .title .remove .font {
/* 左侧内边距 */
padding-left: 5rpx;
}
/* 搜索历史内容区域样式 */
.history .content {
/* 上下内边距 */
padding: 20rpx 0;
/* 使用弹性盒布局 */
display: flex;
/* 允许换行 */
flex-wrap: wrap;
}
/* 搜索历史内容项样式 */
.history .content .item {
/* 背景颜色 */
background: rgb(238, 236, 236);
/* 文字颜色 */
color: #333;
/* 字体大小 */
font-size: 30rpx;
/* 内边距 */
padding: 8rpx 25rpx;
/* 外边距 */
margin: 0 20rpx 20rpx 0;
/* 圆角 */
border-radius: 50rpx;
}
展示
无历史搜索时,不展示:
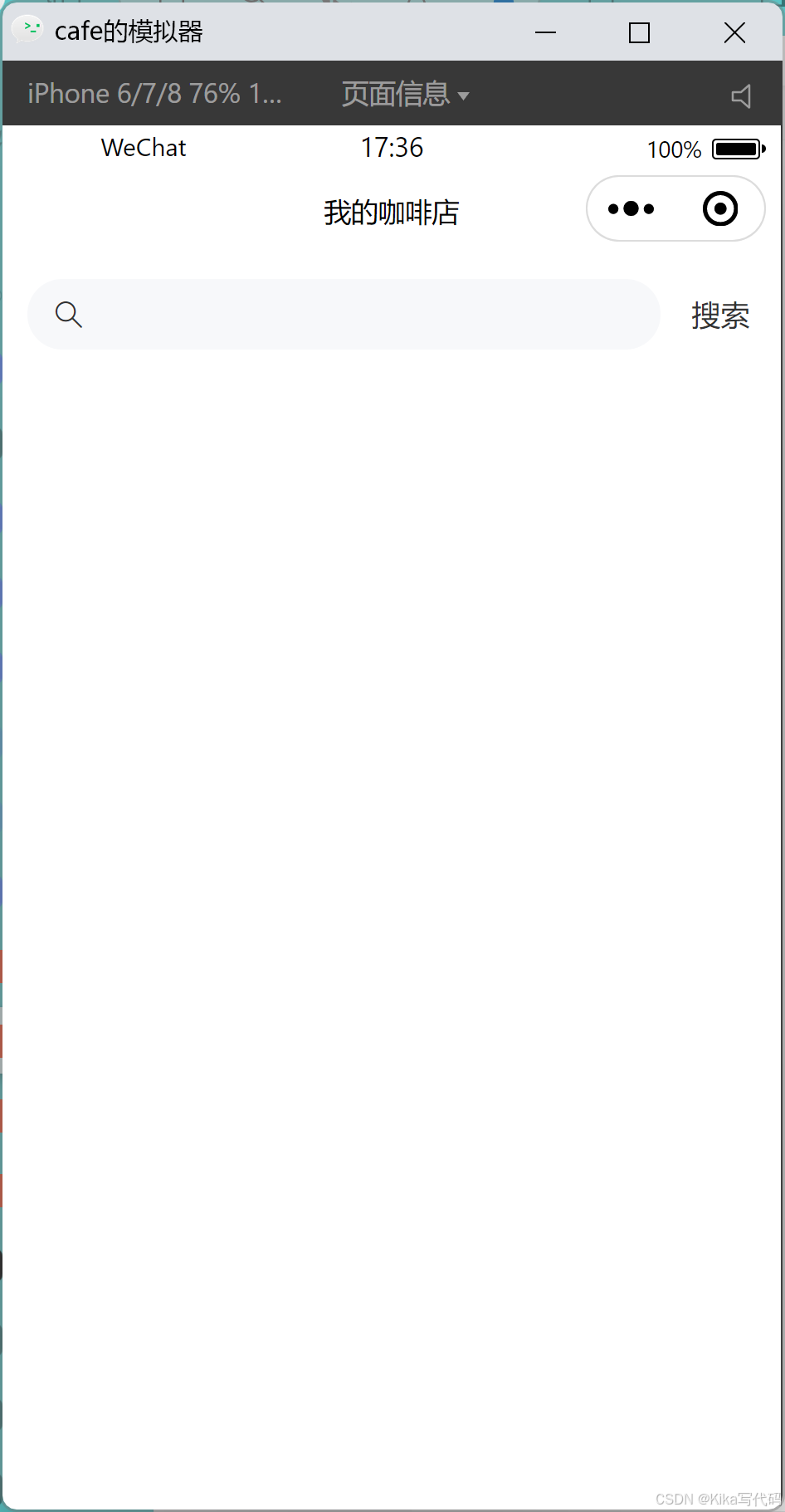