XML界面设计
XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="姓名:" />
<EditText
android:id="@+id/etc_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="50px" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="班级:" />
<EditText
android:id="@+id/etc_class"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="50px" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="学号:" />
<EditText
android:id="@+id/etc_id"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="50px" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/btn_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="添加数据"
android:textAlignment="center" />
<Button
android:id="@+id/btn_show"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="全部显示"
android:textAlignment="center" />
<Button
android:id="@+id/btn_clr"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="清除数据"
android:textAlignment="center" />
<Button
android:id="@+id/btn_del"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="全部删除"
android:textAlignment="center" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ID:" />
<EditText
android:id="@+id/etc_id2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="2" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/btn_del_id"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="ID删除"
android:textAlignment="center" />
<Button
android:id="@+id/btn_show_id"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="ID查询"
android:textAlignment="center" />
<Button
android:id="@+id/btn_update_id"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="ID更新"
android:textAlignment="center" />
</LinearLayout>
<TextView
android:id="@+id/txt_end"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="" />
<ListView
android:id="@+id/list"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
People.java
java
package com.example.exp6;
public class People {
public int ID = -1;
public String Name;
public String Class;
public String Number;
//重载toString方法 返回值String类型
@Override
public String toString(){
String result = "";
result += "ID:" + this.ID + ",";
result += "姓名:" + this.Name + ",";
result += "班级:" + this.Class + ", ";
result += "学号:" + this.Number;
return result;
}
}
DBAdapter.java
java
package com.example.exp6;
import android.annotation.SuppressLint;
import android.content.*;
import android.database.Cursor;
import android.database.DatabaseErrorHandler;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteException;
import android.database.sqlite.SQLiteOpenHelper;
import androidx.annotation.Nullable;
//对数据库进行操作的类
public class DBAdapter {
private static final String DB_NAME = "student.db"; //数据库名称
private static final String DB_TABLE = "peopleinfo"; //表名
private static final int DB_VERSION = 1; //数据库版本
public static final String KEY_ID = "_id"; //数据库表的属性名称
public static final String KEY_NAME = "name";
public static final String KEY_CLASS = "class";
public static final String KEY_NUMBER = "number";
private SQLiteDatabase db; //数据库的实例db
private final Context context; //Context的实例化
private DBOpenHelper dbOpenHelper; //帮助类的实例化 dbOpenHelper
//内部类:对帮助类 构建
//继承SQLiteOpenHelper
//必须重载onCreate和onUpgrade方法
private static class DBOpenHelper extends SQLiteOpenHelper {
//帮助类的构造函数 -- 4个参数
//Code -> SQLiteOpenHelper 即可成功插入该构造函数
public DBOpenHelper(@Nullable Context context, @Nullable String name,
SQLiteDatabase.CursorFactory factory, int version) {
super(context, name, factory, version);
}
//static常量字符串--创建表的 sql 命令
//create table peopleinfo("id integer primary key autoincrement,name text not null,
// class text not null,number text not null")
private static final String DB_CREATE = "create table " +
DB_TABLE + " (" + KEY_ID + " integer primary key autoincrement, " +
KEY_NAME + " text not null, " + KEY_CLASS + " text not null," +
KEY_NUMBER + " text not null);";
//重载帮助类onCreate方法
//1个参数SQLiteDatabase类型
@Override
public void onCreate(SQLiteDatabase sqLiteDatabase) {
//execSQL()方法 1个String类型的 sql 建表命令
sqLiteDatabase.execSQL(DB_CREATE);
}
//重载帮助类onUpdate方法
//一般在升级的时候被调用
//删除旧的数据库表 并将数据转移到新版本的数据库表
//3个参数SQLiteDatabase类型、int i-旧版本号、int i1-新版本号
@Override
public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) {
//仅删除原表后建立新表
sqLiteDatabase.execSQL("DROP TABLE IF EXISTS " + DB_TABLE);
onCreate(sqLiteDatabase);
}
}
//对数据库进行操作的类DBAdapter的构造
//参数1个 Context类型
public DBAdapter(Context _context) {
context = _context;
}
//DBAdapter类的open方法
//抛出异常!!!!
public void open() throws SQLiteException {
//帮助类实例化dbOpenHelper需要4个参数
dbOpenHelper = new DBOpenHelper(context, DB_NAME,
null, DB_VERSION);
//调用getWritableDatabase方法
try {
db = dbOpenHelper.getWritableDatabase();
} catch (SQLiteException ex) {
db = dbOpenHelper.getReadableDatabase();
}
}
//DBAdapter类的close方法
public void close() {
if (db != null) {
db.close();
db = null;
}
}
//DBAdapter类的insert方法
//参数1个:数据库储存的类型(本例是People
public long insert(People people) {
//用ContentValues类型
ContentValues newValues = new ContentValues();
//在put的时候不需要put Key_ID!!!
newValues.put(KEY_NAME, people.Name);
newValues.put(KEY_CLASS, people.Class);
newValues.put(KEY_NUMBER, people.Number);
//返回值是新数据插入的位置 ID值
//数据库对象.insert方法
//参数3个:表名 在null时的替换数据 ContentValues类型需要添加的数据
return db.insert(DB_TABLE, null, newValues);
}
//DBAdapter类的deleteAllData方法
//无参数
public long deleteAllData() {
//返回值是被删除的数据的数量
//参数3个:表名 删除条件(删除全部数据条件是null)
return db.delete(DB_TABLE, null, null);
}
//DBAdapter类的deleteOneData方法
//参数1个:long类型的id值
public long deleteOneData(long id) {
//返回值是被删除的数据的数量
//参数3个:表名 删除条件(删除形参给的id)
return db.delete(DB_TABLE, KEY_ID + "=" + id, null);
}
//DBAdapter类的UpdataOneData方法
//参数2个:long类型的id值 和 People类型
public long updateOneData(long id , People people){
//更新和插入一样用到了ContentValues类型
ContentValues updateValues = new ContentValues();
//同样不需要放Key_ID
updateValues.put(KEY_NAME, people.Name);
updateValues.put(KEY_CLASS, people.Class);
updateValues.put(KEY_NUMBER, people.Number);
//返回值是被更新的数据数量
//参数4个 表明 ContentValues 更新条件string类型 null
return db.update(DB_TABLE, updateValues, KEY_ID + "=" + id, null);
}
//private类型
//DBAdapter类的ConvertToPeople方法
//参数1个 Cursor类型
//返回People数组
//查询需要基于该 ConvertToPeople 方法(应该是自定义方法?)
@SuppressLint("Range")
private People[] ConvertToPeople(Cursor cursor){
//getCount方法返回查询结果总行数
int resultCounts = cursor.getCount();
//行数为0||moveToFirst方法返回false返回结果为空
if (resultCounts == 0 || !cursor.moveToFirst()){
//该方法返回null
return null;
}
//新建resultCounts个People对象
People[] peoples = new People[resultCounts];
//循环 resultCounts次
for (int i = 0 ; i<resultCounts; i++){
peoples[i] = new People();
peoples[i].ID = cursor.getInt(0);
//根据 列属性索引 得到 属性值
peoples[i].Name = cursor.getString(cursor.getColumnIndex(KEY_NAME));
peoples[i].Class = cursor.getString(cursor.getColumnIndex(KEY_CLASS));
peoples[i].Number = cursor.getString(cursor.getColumnIndex(KEY_NUMBER));
//游标/指针下移
cursor.moveToNext();
}
//返回People[]
return peoples;
}
//查询操作:
//DBAdapter类的getOneData方法
//参数1个:long类型的id值
public People[] getOneData(long id) {
//query方法
//参数7个(6String 1String[]):表名称 属性列-String[] 查询条件
//查询条件是否使用通配符 分组条件 分组过滤条件 排序方式 后4个全是null
//返回Cursor类型
Cursor results = db.query(DB_TABLE, new String[] { KEY_ID, KEY_NAME, KEY_CLASS, KEY_NUMBER},
KEY_ID + "=" + id, null, null, null, null);
//返回People[]
return ConvertToPeople(results);
}
//DBAdapter类的getAllData方法
public People[] getAllData() {
//参数查询条件为null 存在5个null
Cursor results = db.query(DB_TABLE, new String[] { KEY_ID, KEY_NAME, KEY_CLASS, KEY_NUMBER},
null, null, null, null, null);
return ConvertToPeople(results);
}
}
MainActivity.java
java
package com.example.exp6;
import android.database.sqlite.SQLiteDatabase;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
import java.util.List;
import android.widget.ArrayAdapter;
public class MainActivity extends AppCompatActivity {
EditText etc_name,etc_class,etc_id,etc_id2;
TextView txt_end;
ListView listView;
Button btn_add,btn_show,btn_clr,btn_del,btn_del_id,btn_show_id,btn_update_id;
DBAdapter dbAdapter;
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView=findViewById(R.id.list);
ArrayList<String> data=new ArrayList<String>();
//数组适配器
//实例化 参数3个
ArrayAdapter<String> adapter=new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1,data);
etc_name=findViewById(R.id.etc_name);
etc_class=findViewById(R.id.etc_class);
etc_id=findViewById(R.id.etc_id);
btn_add=findViewById(R.id.btn_add);
btn_show=findViewById(R.id.btn_show);
btn_clr=findViewById(R.id.btn_clr);
btn_del=findViewById(R.id.btn_del);
btn_del_id=findViewById(R.id.btn_del_id);
btn_show_id=findViewById(R.id.btn_show_id);
btn_update_id= findViewById(R.id.btn_update_id);
etc_id2=findViewById(R.id.etc_id2);
txt_end=findViewById(R.id.txt_end);
//处理数据库的类的实例对象
//参数1个 Context类型
dbAdapter=new DBAdapter(this);
//调用DBAdapter对象的 open 方法
dbAdapter.open();
btn_add.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//将文本框中的内容用来创建对象People
People t=new People();
t.Name=etc_name.getText().toString();
t.Class=etc_class.getText().toString();
t.Number=etc_id.getText().toString();
//插入一个对象People
//返回插入的位置id
long colunm=dbAdapter.insert(t);
if (colunm == -1 ){
txt_end.setText("添加过程错误!");
} else {
txt_end.setText("ID:"+String.valueOf(colunm)+" 姓名:"+
t.Name+" 班级:"+t.Class+" 学号:"+t.Number);
}
}
});
btn_show.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//调用得到数据库中全部的信息
People [] peoples =dbAdapter.getAllData();
if (peoples == null){
txt_end.setText("数据库中没有数据");
return;
}
String t="数据库:\n";
for(int i=0;i<peoples.length;++i){
t += peoples[i].toString()+"\n";
}
txt_end.setText(t);
}
});
btn_clr.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
txt_end.setText("");
}
});
btn_del.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
dbAdapter.deleteAllData();
txt_end.setText("已删除所有数据!");
}
});
btn_del_id.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int id=Integer.parseInt(etc_id2.getText().toString());
//返回删除的数据的数量
//参数要求int类型的
long result=dbAdapter.deleteOneData(id);
String msg = "删除ID为"+etc_id2.getText().toString()+"的数据" + (result>0?"成功":"失败");
txt_end.setText(msg);
}
});
btn_show_id.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int id=Integer.parseInt(etc_id2.getText().toString());
//查找方法 参数id-int
//返回值是People[]
People people[]=dbAdapter.getOneData(id);
if(people==null){
txt_end.setText("Id为"+id+"的记录不存在!");
}
else{
//因为仅只查找了一条信息 所以是people[0]
//并且在People类型中已经重载了函数toString()
txt_end.setText("查询成功:\n"+people[0].toString());
}
}
});
btn_update_id.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int id=Integer.parseInt(etc_id2.getText().toString());
People t=new People();
t.Name=etc_name.getText().toString();
t.Class=etc_class.getText().toString();
t.Number=etc_id.getText().toString();
//更新方法
//参数2个 id-int people类型
//返回值 被更新的数据数量
long n=dbAdapter.updateOneData(id,t);
if (n<0){
txt_end.setText("更新过程错误!");
}else{
txt_end.setText("成功更新数据,"+String.valueOf(n)+"条");
}
}
});
}
@Override
protected void onStop() {
super.onStop();
dbAdapter.close();
}
}
结果
数据添加(朱迪&尼克狐尼克)
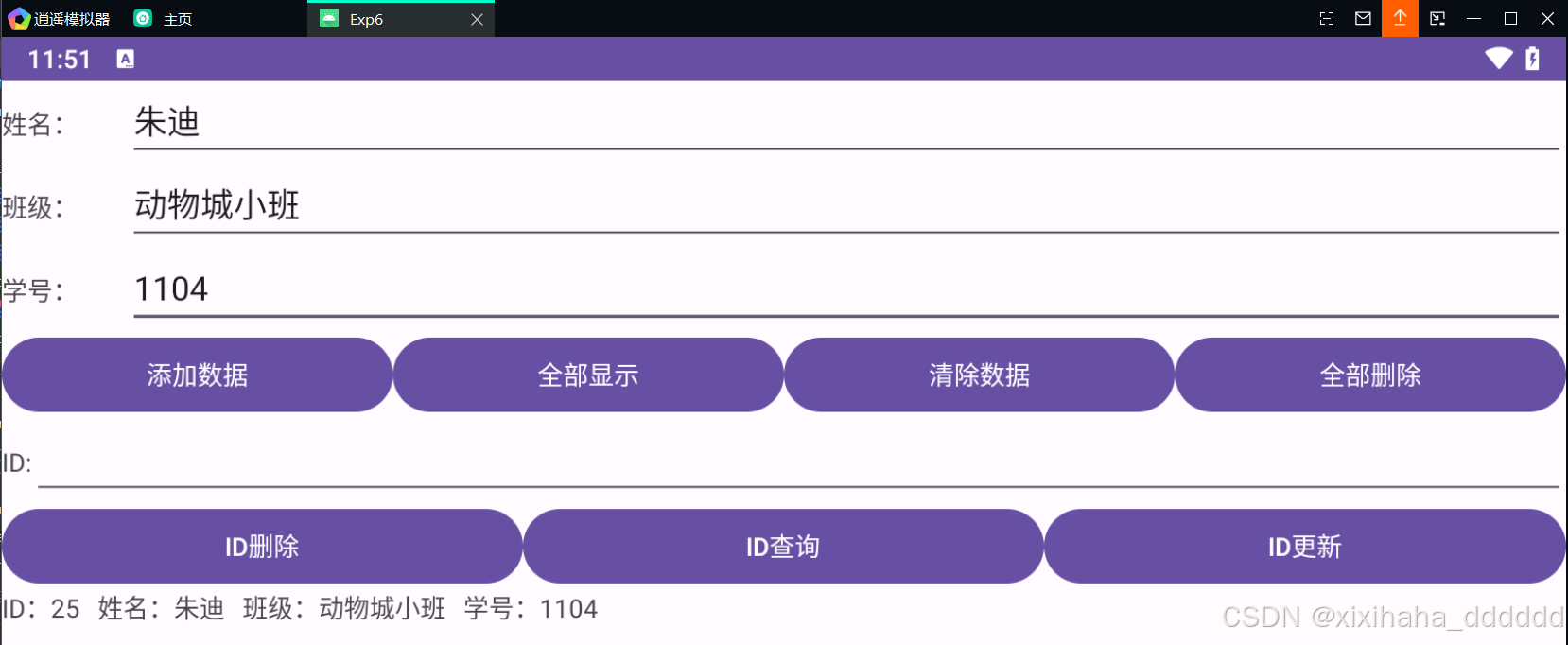
全部显示
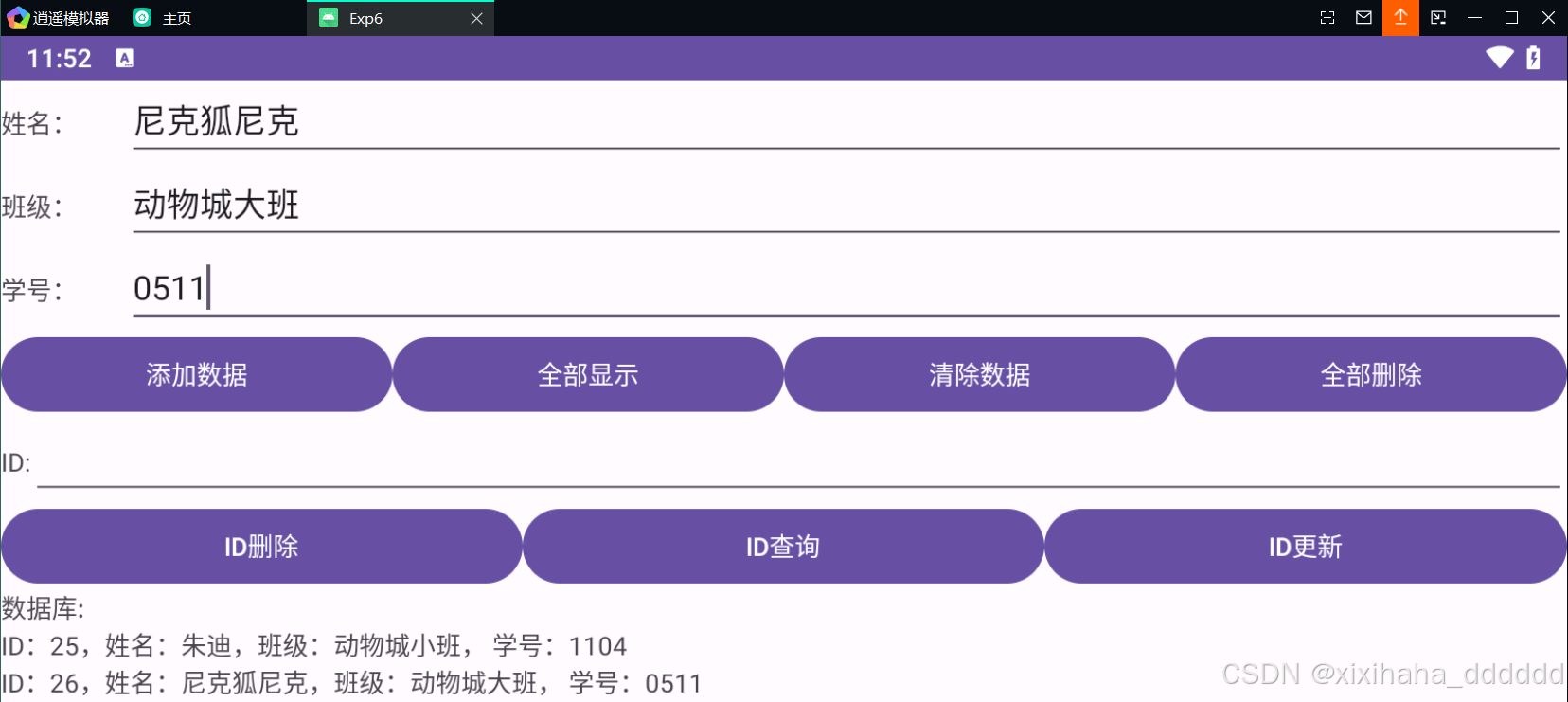
ID删除 (25-朱迪)
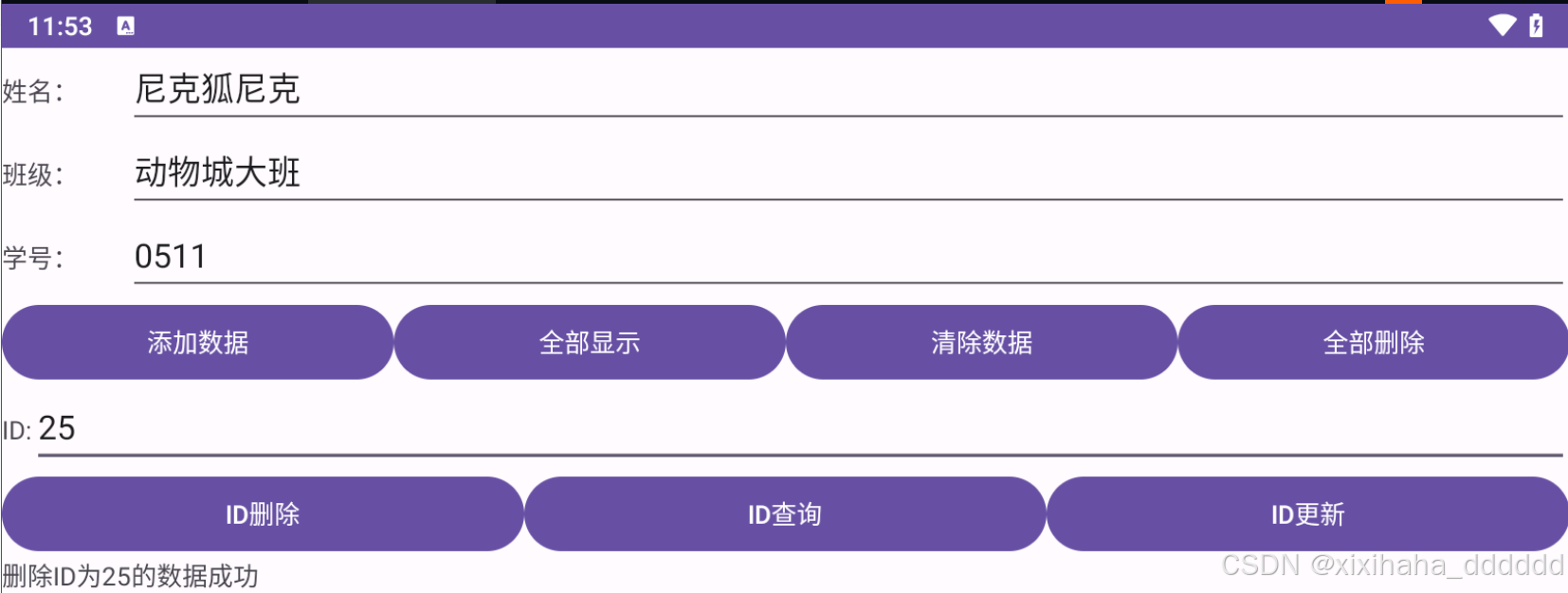
ID查询 (26)

ID更新 (26)
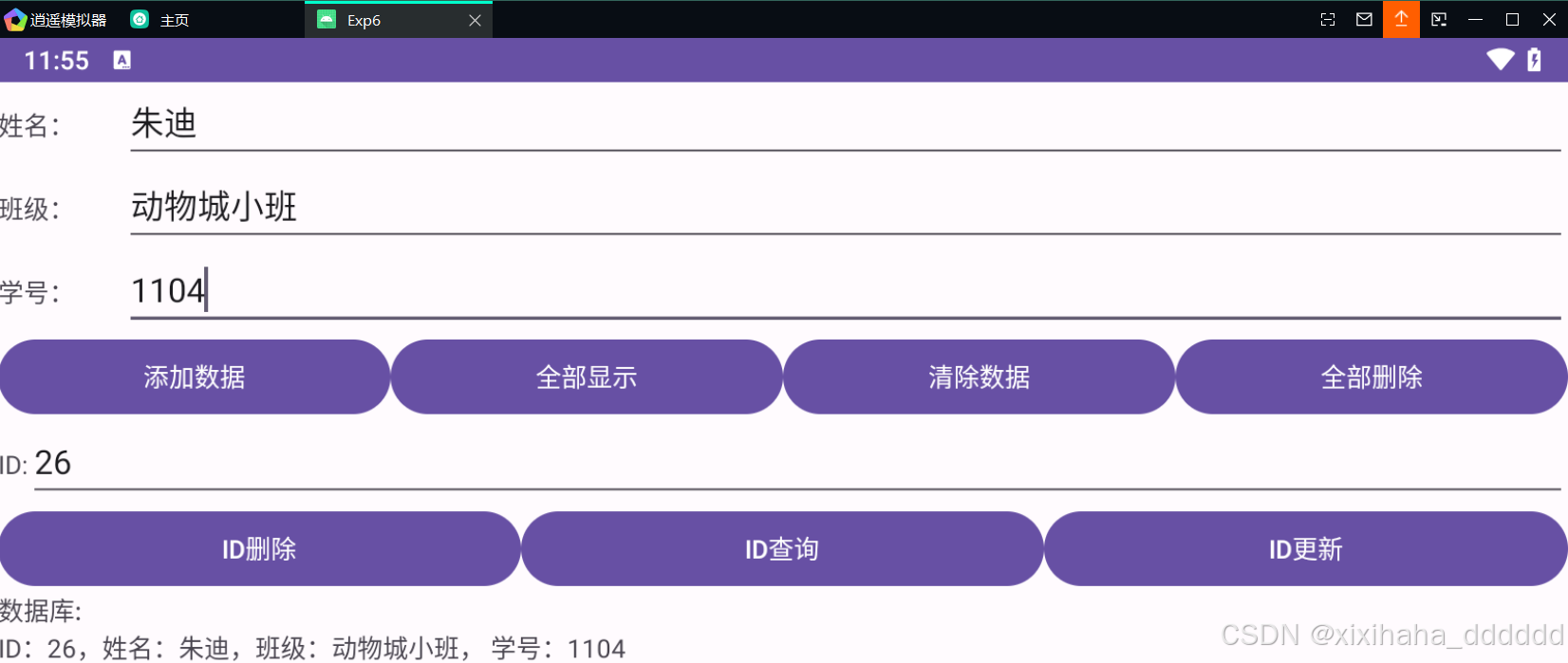
全部删除
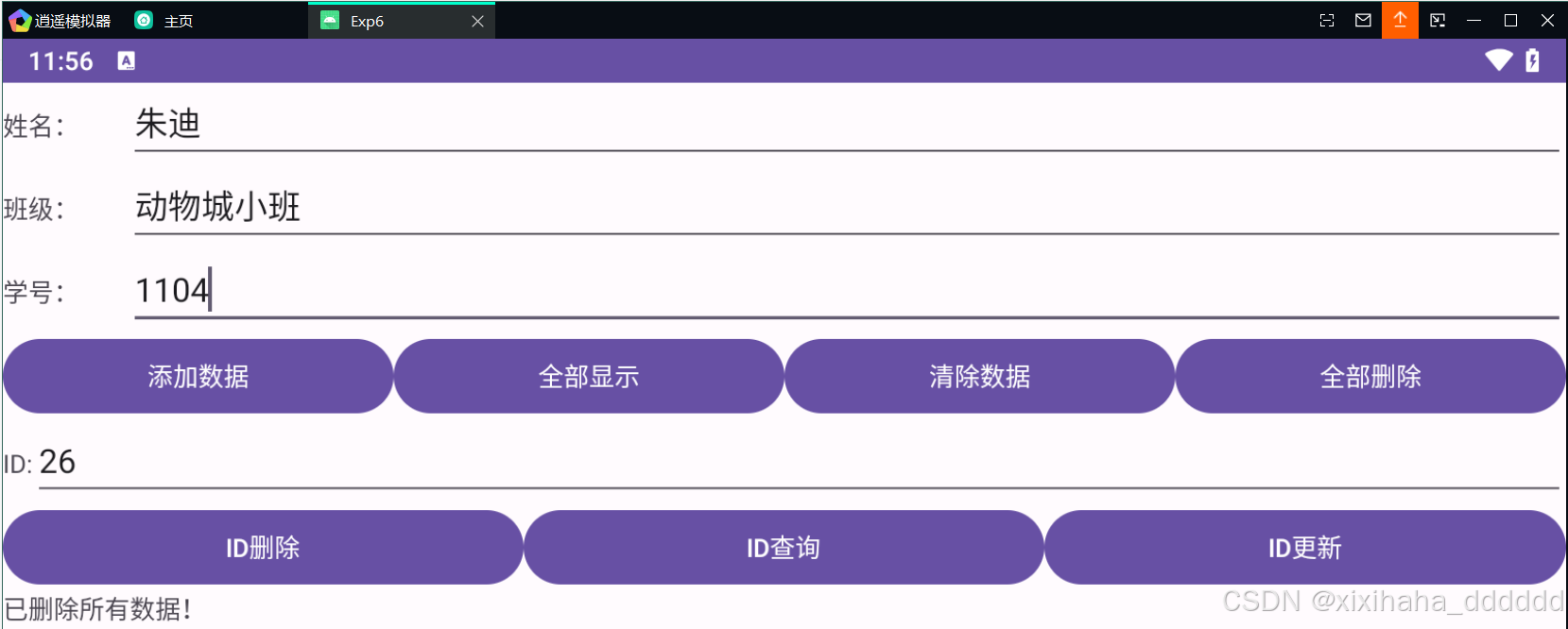
全部显示
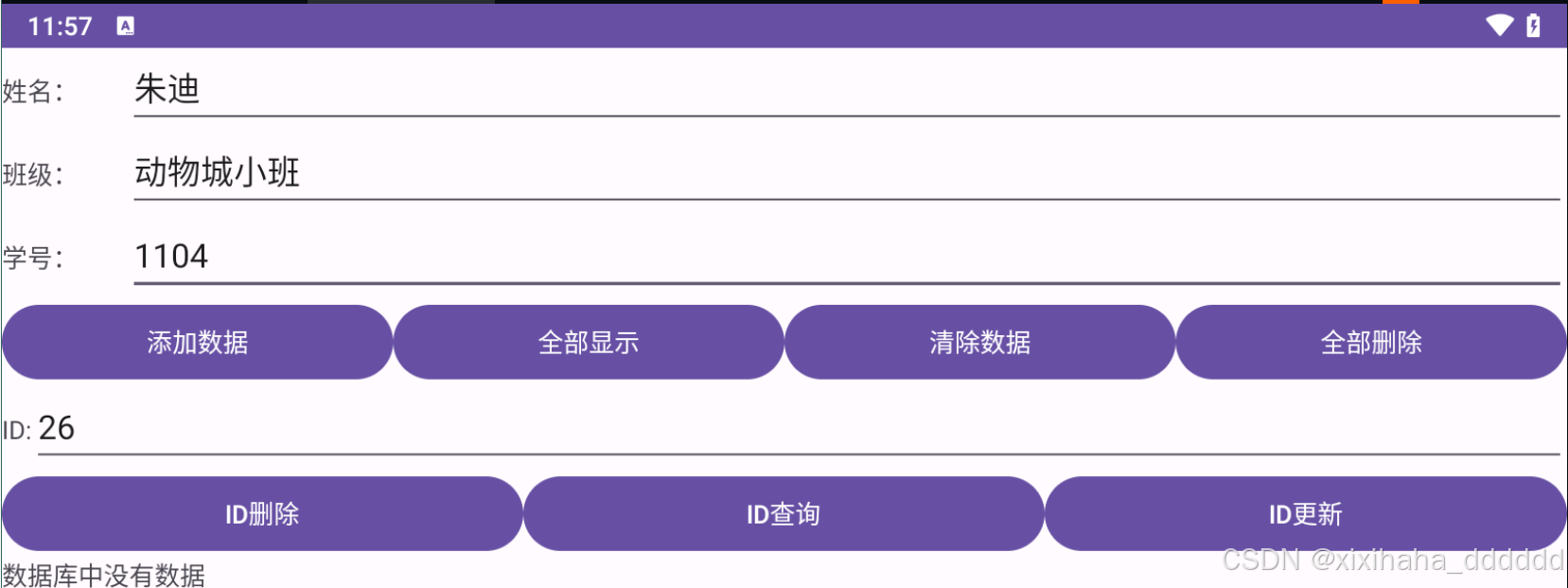