1.思维导图
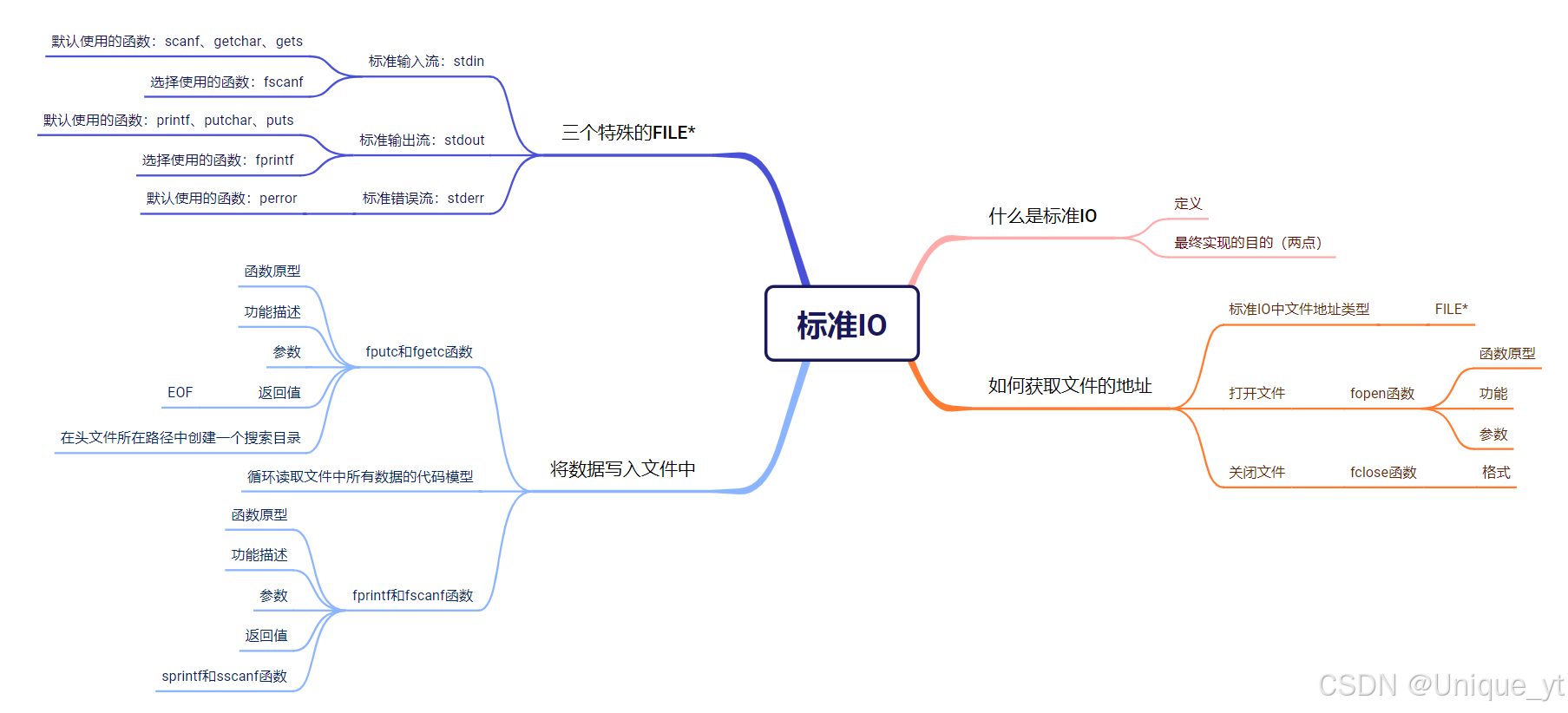
2.先编写以下结构体
struct Student
{
char name[20];
double math;
double chinese;
double english;
double physical;
double chemical;
double biological; };
第一步:创建一个 struct Student 类型的链表L,初始化该链表中3个学生的属性
第二步:编写一个叫做save的函数,功能为 将链表L中的3个学生的所有信息,保存到文件中去,使用fprintf实现
第三步:编写一个叫做load的函数,功能为 将文件中保存的3个学生信息,读取后,写入到另一个链表L1中去
第四步:编写一个叫做 show的函数,功能为 遍历输出 L或者 L1链表中的所有学生的信息
第五步:编写一个叫做 setMath 的函数,功能为 修改 文件中 所有学生的数学成绩
1>程序代码:
cs
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
//定义一个学生成绩的结构体类型
typedef struct Student
{
char name[20];
double math;
double chinese;
double english;
double physical;
double chemical;
double biological;
struct Student *next;
} Stu, *StuPtr;
//创建链表
StuPtr create()
{
StuPtr L = NULL;
return L;
}
//添加数据
StuPtr add(StuPtr L)
{
StuPtr s = (StuPtr)malloc(sizeof(Stu));
if (s == NULL)
{
printf("内存分配失败!\n");
return L;
}
if (L == NULL)
{
L = s;
s->next = NULL;
}
else
{
StuPtr p = L;
while (p->next!= NULL)
{
p = p->next;
}
p->next = s;
}
s->next = NULL;
printf("姓名:");
scanf("%s", s->name);
printf("数学:");
scanf("%lf", &s->math);
printf("语文:");
scanf("%lf", &s->chinese);
printf("英语:");
scanf("%lf", &s->english);
printf("物理:");
scanf("%lf", &s->physical);
printf("化学:");
scanf("%lf", &s->chemical);
printf("生物:");
scanf("%lf", &s->biological);
printf("\n");
return L;
}
//将数组链表L中学生信息保存到文件中
void save(StuPtr L, const char *filename)
{
if (L == NULL)
{
printf("保存失败!\n");
return;
}
FILE *fp = fopen(filename, "w");
if (fp == NULL)
{
printf("无法打开文件!\n");
return;
}
StuPtr p = L;
while (p!= NULL)
{
fprintf(fp, "%s\t%.2lf\t%.2lf\t%.2lf\t%.2lf\t%.2lf\t%.2lf\n", p->name, p->math, p->chinese, p->english, p->physical, p->chemical, p->biological);
p = p->next;
}
fclose(fp);
}
//将文件中保存的学生信息,写入到另一个链表L1中
void load(StuPtr *L1, const char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL)
{
printf("无法打开文件!\n");
return;
}
*L1 = NULL;
while (1)
{
StuPtr s = (StuPtr)malloc(sizeof(Stu));
if (s == NULL)
{
printf("内存分配失败!\n");
break;
}
int res = fscanf(fp, "%s\t%lf\t%lf\t%lf\t%lf\t%lf\t%lf\n", s->name, &s->math, &s->chinese, &s->english, &s->physical, &s->chemical, &s->biological);
if (res == EOF)
{
free(s);
break;
}
s->next = *L1;
*L1 = s;
}
fclose(fp);
}
//展示链表内容
void show(StuPtr L)
{
StuPtr p = L;
if (p == NULL)
{
printf("展示失败!\n");
return;
}
printf("姓名\t数学\t语文\t英语\t物理\t化学\t生物\n");
while (p!= NULL)
{
printf("%s\t%.2lf\t%.2lf\t%.2lf\t%.2lf\t%.2lf\t%.2lf\n", p->name, p->math, p->chinese, p->english, p->physical, p->chemical, p->biological);
p = p->next;
}
printf("------------------------------------------------------\n");
}
//修改文件中所有学生的数学成绩
void setMath(const char *filename, double math)
{
StuPtr L = create();
load(&L, filename); // 修正这里,传递指针的指针
StuPtr p = L;
while (p!= NULL)
{
p->math = math;
p = p->next;
}
save(L, filename);
// 释放内存
while (L!= NULL)
{
StuPtr temp = L;
L = L->next;
free(temp);
}
}
int main(int argc, const char *argv[])
{
StuPtr L = create();
L = add(L);
L = add(L);
L = add(L);
save(L, "student.txt");
StuPtr L1 = create();
load(&L1, "student.txt"); // 修正这里,传递指针的指针
show(L);
show(L1);
setMath("student.txt", 100);
// 释放内存
while (L!= NULL)
{
StuPtr temp = L;
L = L->next;
free(temp);
}
while (L1!= NULL)
{
StuPtr temp = L1;
L1 = L1->next;
free(temp);
}
return 0;
}