在Nuget解决方案中搜索itext7,进行安装
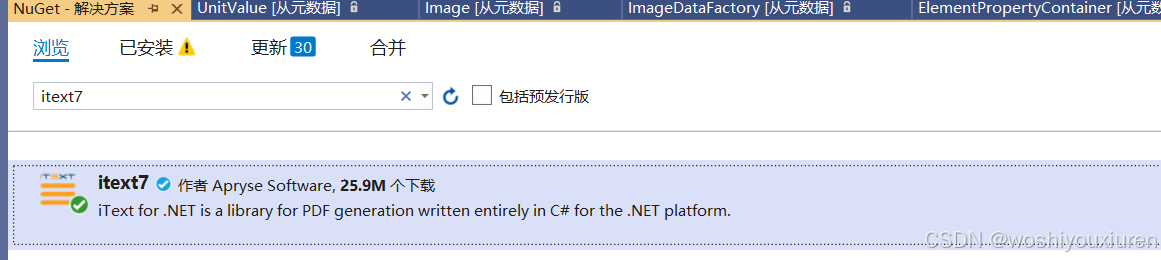
同时还要安装 itext7.bouncy-castle-adapter。
否则 PdfWriter writer = new PdfWriter(pdfOutputPath);执行时会报错unknown PdfException,然后生成一个空白的pdf,且显示已损坏。
捕获异常发现 ex.InnerException
{"Either com.itextpdf:bouncy-castle-adapter or com.itextpdf:bouncy-castle-fips-adapter dependency must be added in order to use BouncyCastleFactoryCreator"},安装itext7.bouncy-castle-adapter后可以了。
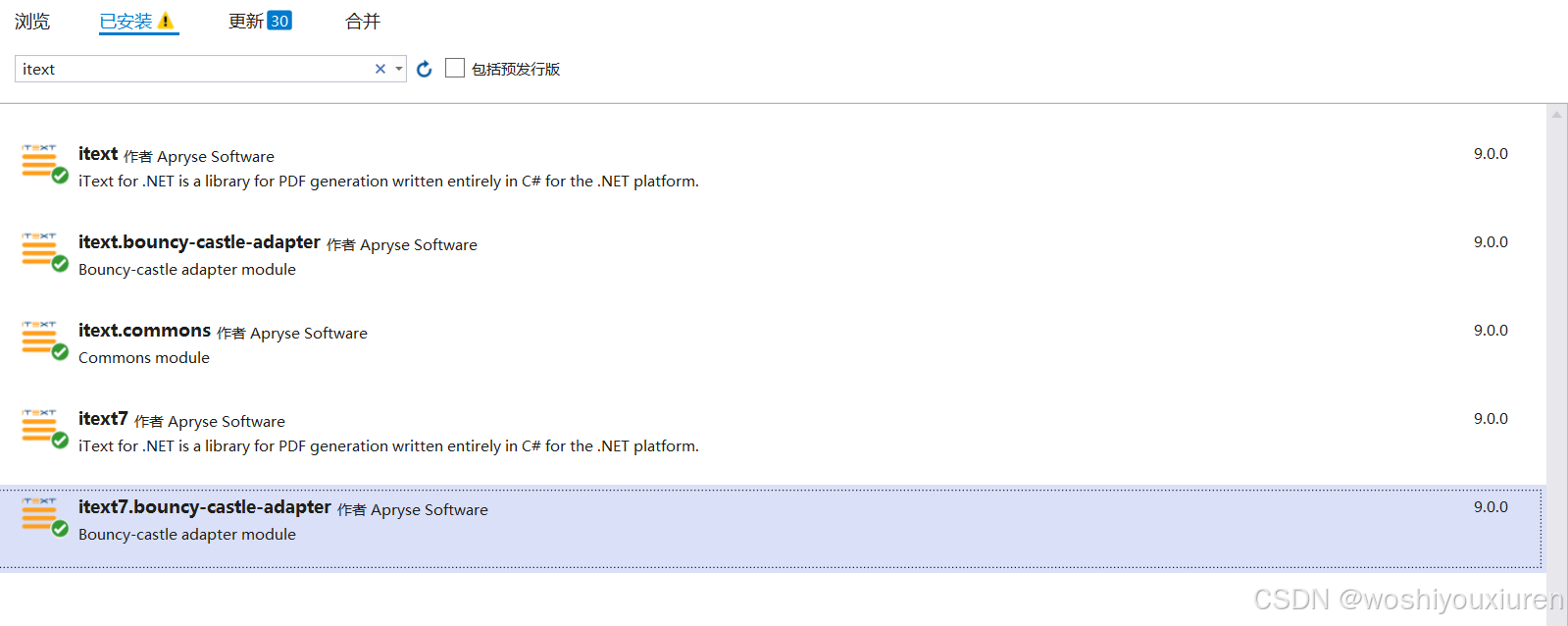
cs
using iText.Kernel.Pdf;
using iText.Layout;
using iText.Layout.Element;
using iText.Layout.Properties;
using iText.Layout.Borders;
using iText.IO.Font;
/// <summary>
/// 导出pdf
/// </summary>
/// <param name="pdfOutputPath">pdf导出路径</param>
private static void exportPdf(string pdfOutputPath)
{
PdfWriter writer = new PdfWriter(pdfOutputPath);
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
// 设置中文字体
iText.Kernel.Font.PdfFont font = iText.Kernel.Font.PdfFontFactory.CreateFont("C://Windows//Fonts//SIMFANG.TTF", PdfEncodings.IDENTITY_H);
document.Add(new Paragraph("添加段落").SetFont(font));
//创建表格
iText.Layout.Element.Table table = new iText.Layout.Element.Table(12) // 12列的表格
.SetWidth(UnitValue.CreatePercentValue(100)) // 表格宽度为100%
.SetMarginTop(10)
.SetMarginBottom(10)
.SetFont(font);
// 添加表头
table.AddCell(new Cell(1, 12).Add(new Paragraph("表头")).SetBackgroundColor(new DeviceRgb(217, 217, 217)).SetTextAlignment(TextAlignment.CENTER));
// 添加内容
table = fillTable(table, 1, 2, "test1:", 0, 15f);//fillTable 为自定义的方法
table = fillTable(table, 1, 4, "测试1", 0, 35f);
table = fillTable(table, 1, 2, "test2:", 0, 15f);
table = fillTable(table, 1, 4, "测试2", 0, 35f);
//单元格加入图片
string picPath = "E:/image/aaa.png";
Image image = new Image(iText.IO.Image.ImageDataFactory.Create(picPath));
image.Scale(0.5f, 0.5f); //依照比例缩放,根据实际图片大小,太大了会超出所以进行缩放
image.SetMarginLeft(50f); //在单元格中的位置调整
Cell cell = new Cell(13, 12).Add(image).SetBorder(new SolidBorder(1));
table.AddCell(cell.SetWidth(UnitValue.CreatePercentValue(95f)).SetTextAlignment(TextAlignment.CENTER));
// 添加表格到文档
document.Add(table).SetFontSize(14);
document.Close();
}
/// <summary>
/// 填充表格
/// </summary>
/// <param name="table">表格</param>
/// <param name="rowspant">单元格行数</param>
/// <param name="colspant">列数</param>
/// <param name="content">内容</param>
/// <param name="bordert">边框 0无边框 1宽度为1的边框</param>
/// <param name="percentt">宽度占比 例如100%就传入100</param>
/// <returns></returns>
private static iText.Layout.Element.Table fillTable(iText.Layout.Element.Table table, int rowspant, int colspant, string content, float bordert, float percentt)
{
//边框判断
Border borderTemp = null;
if (bordert > 0)
{
borderTemp = new SolidBorder(bordert);
}
if (content == null || content.Equals(""))//单元格空值填充
{
table.AddCell(new Cell(rowspant, colspant).SetBorder(borderTemp).SetWidth(UnitValue.CreatePercentValue(percentt)).SetTextAlignment(TextAlignment.CENTER));
}
else
{
//new Cell(rowspant, colspant) //创建单元格为几行几列,(1,1)为单个单元格,(x,x)为合并单元格
//.Add(new Paragraph(content)) //添加单元格内容,如果为空不能写此项,否则报错
//.SetBorder(borderTemp) //设置单元格边框
//.SetWidth(UnitValue.CreatePercentValue(percentt)) //设置单元格宽度占比
//.SetTextAlignment(TextAlignment.CENTER) //设置单元格内容居中
table.AddCell(new Cell(rowspant, colspant).Add(new Paragraph(content)).SetBorder(borderTemp).SetWidth(UnitValue.CreatePercentValue(percentt)).SetTextAlignment(TextAlignment.CENTER));
}
return table;
}