1.找到需要三个 DLL
- Mono.Data.Sqlite.dll
- System.Data.dll
- sqlite3.dll
上面两个dll可在本地unity安装目录找到:
C:\Program Files\Unity\Hub\Editor\2022.3.xxf1c1\Editor\Data\MonoBleedingEdge\lib\mono\unityjit-win32
下面dll可在sqlite官网下载到:
https://www.sqlite.org/download.html
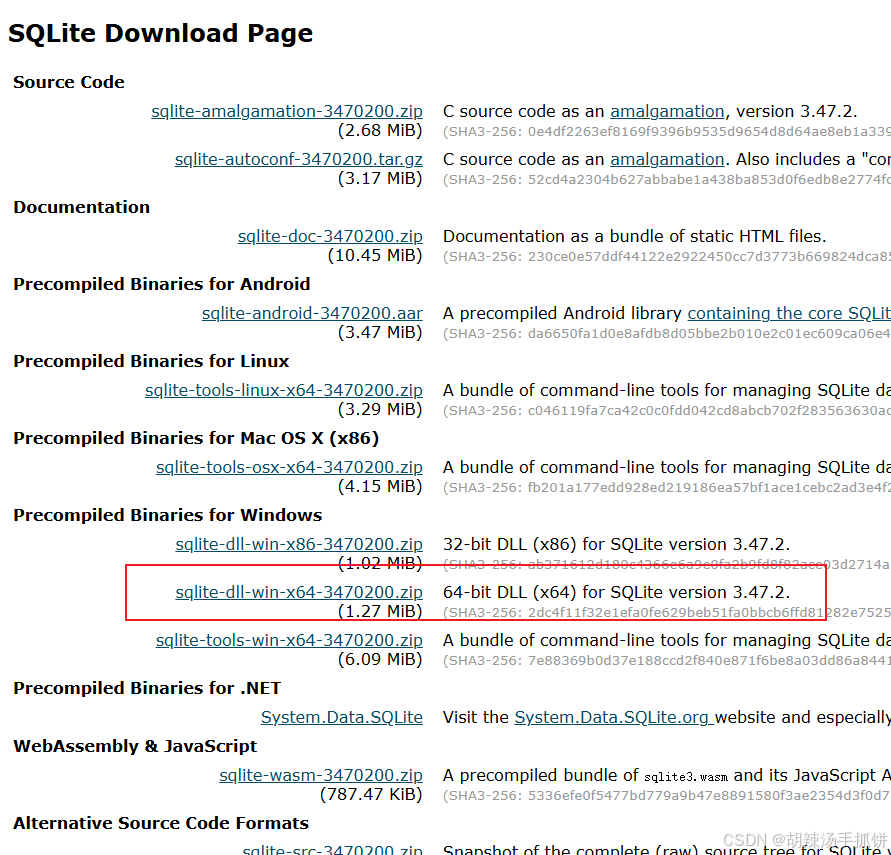
2.将以上三个dll放入unity的里面的Plugins里面
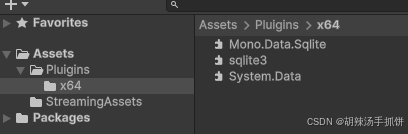
3.测试代码:
cs
using UnityEngine;
using Mono.Data.Sqlite;
using System.Data;
public class SQLiteTest : MonoBehaviour
{
private string dbPath;
void Start()
{
// SQLite 数据库的路径
dbPath = "URI=file:" + Application.streamingAssetsPath + "/example.db";
Debug.Log("Database Path: " + dbPath);
CreateTable();
InsertData("TestKey", "TestValue");
string value = GetData("TestKey");
Debug.Log("Retrieved Value: " + value);
}
private void CreateTable()
{
using (IDbConnection dbConnection = new SqliteConnection(dbPath))
{
dbConnection.Open();
using (IDbCommand command = dbConnection.CreateCommand())
{
command.CommandText = "CREATE TABLE IF NOT EXISTS MyTable (key TEXT PRIMARY KEY, value TEXT)";
command.ExecuteNonQuery();
}
}
}
private void InsertData(string key, string value)
{
using (IDbConnection dbConnection = new SqliteConnection(dbPath))
{
dbConnection.Open();
using (IDbCommand command = dbConnection.CreateCommand())
{
command.CommandText = "INSERT OR REPLACE INTO MyTable (key, value) VALUES (@key, @value)";
command.Parameters.Add(new SqliteParameter("@key", key));
command.Parameters.Add(new SqliteParameter("@value", value));
command.ExecuteNonQuery();
}
}
}
private string GetData(string key)
{
using (IDbConnection dbConnection = new SqliteConnection(dbPath))
{
dbConnection.Open();
using (IDbCommand command = dbConnection.CreateCommand())
{
command.CommandText = "SELECT value FROM MyTable WHERE key = @key";
command.Parameters.Add(new SqliteParameter("@key", key));
using (IDataReader reader = command.ExecuteReader())
{
if (reader.Read())
{
return reader.GetString(0);
}
}
}
}
return null;
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.T))
{
Debug.Log("写入数据");
InsertData("名字", "蜡笔小新");
InsertData("年龄", "5岁");
InsertData("职业", "幼儿园学生");
InsertData("爱好", "大姐姐");
}
if (Input.GetKeyDown(KeyCode.Space))
{
Debug.Log(GetData("名字"));
Debug.Log(GetData("年龄"));
Debug.Log(GetData("职业"));
Debug.Log(GetData("爱好"));
}
}
}
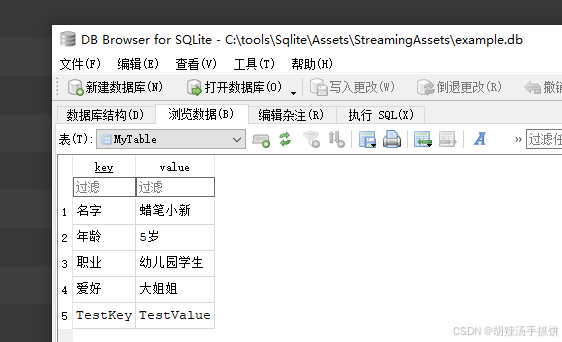
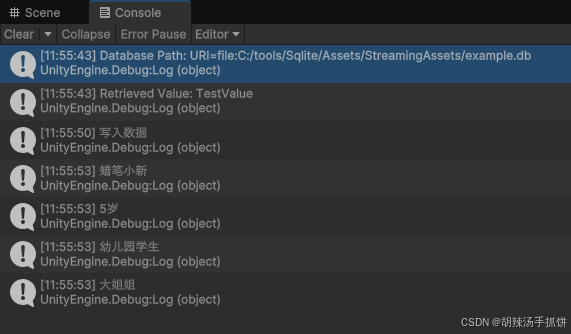