一、准备环境
首先,进入下载地址:Central Repository: cn/hutool/hutool-all/5.8.16下载jar包
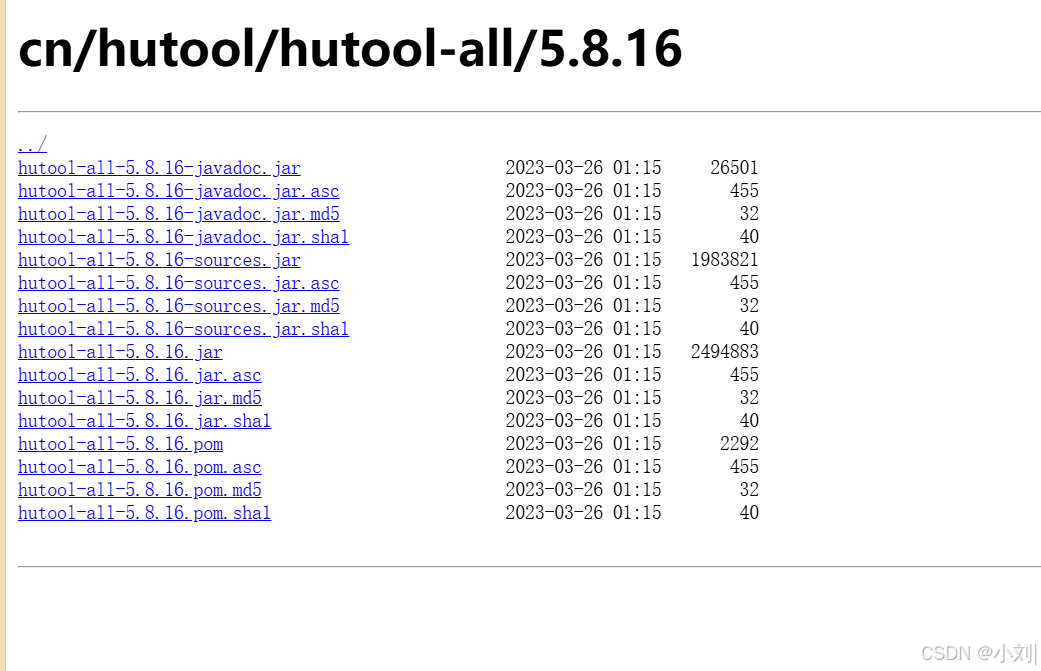
二、配置环境
将下载好的jar包放到eclipse的lib目录 (这里是eclipse软件中存放jar包的目录):
【WebContent-->WEB-INF-->lib】
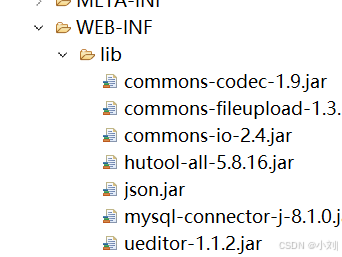
三、基础方法
(验证码实现的核心就是以下四条方法)
- createCode 创建验证码,实现类需同时生成随机验证码字符串和验证码图片
- getCode 获取验证码的文字内容
- verify 验证验证码是否正确,建议忽略大小写
- write 将验证码写出到目标流中
LineCaptcha 线段干扰的验证码
java
//定义图形验证码的长和宽
LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(200, 100);
//图形验证码写出,可以写出到文件,也可以写出到流
lineCaptcha.write("d:/line.png");
//输出code
Console.log(lineCaptcha.getCode());
//验证图形验证码的有效性,返回boolean值
lineCaptcha.verify("1234");
//重新生成验证码
lineCaptcha.createCode();
lineCaptcha.write("d:/line.png");
//新的验证码
Console.log(lineCaptcha.getCode());
//验证图形验证码的有效性,返回boolean值
lineCaptcha.verify("1234");
CircleCaptcha 圆圈干扰验证码
java
//定义图形验证码的长、宽、验证码字符数、干扰元素个数
CircleCaptcha captcha = CaptchaUtil.createCircleCaptcha(200, 100, 4, 20);
//CircleCaptcha captcha = new CircleCaptcha(200, 100, 4, 20);
//图形验证码写出,可以写出到文件,也可以写出到流
captcha.write("d:/circle.png");
//验证图形验证码的有效性,返回boolean值
captcha.verify("1234");
ShearCaptcha 扭曲干扰验证码
java
//定义图形验证码的长、宽、验证码字符数、干扰线宽度
ShearCaptcha captcha = CaptchaUtil.createShearCaptcha(200, 100, 4, 4);
//ShearCaptcha captcha = new ShearCaptcha(200, 100, 4, 4);
//图形验证码写出,可以写出到文件,也可以写出到流
captcha.write("d:/shear.png");
//验证图形验证码的有效性,返回boolean值
captcha.verify("1234");
写出到浏览器(Servlet输出)
java
ICaptcha captcha = ...;
captcha.write(response.getOutputStream());
//Servlet的OutputStream记得自行关闭哦!
自定义验证码
有时候标准的验证码不满足要求,比如我们希望使用纯字母的验证码、纯数字的验证码、加减乘除的验证码,此时我们就要自定义CodeGenerator
java
// 自定义纯数字的验证码(随机4位数字,可重复)
RandomGenerator randomGenerator = new RandomGenerator("0123456789", 4);
LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(200, 100);
lineCaptcha.setGenerator(randomGenerator);
// 重新生成code
lineCaptcha.createCode();
前端页面
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="css/login.css">
<script src="js/jquery.js"></script>
<script>
$(function(){
$(".btn").on("click",function(){
var a = $(".account").val().trim()
var p = $(".password").val().trim()
var i = $(".captchaInput").val().trim()
var role = $(".role").val().trim()
if(a==""){
alert("账号不能为空")
return
}
if(p==""){
alert("密码不能为空")
return
}
if(i==""){
alert("验证码不能为空")
return
}
$.ajax({
url:"LoginServlet",
type:"get",
data:{
account:a,
password:p,
captchaInput: i,
role:role,
},
success:function(value){
console.log(value)
alert(value.message)
if (value.code == 1) {
if (role === "admin") {
location.href = "main.html";
} else if (role === "user") {
location.href = "user_main.html";
} else if (role === "merchant") {
// 读取商家对应的cookie,这里假设你已经有获取和解析cookie的函数,比如 getCookie 函数
var merchantName = getCookie("account");
if (merchantName) {
// 根据merchant_id等信息来决定具体跳转的商家页面,这里简单示例为不同id跳转到不同页面,实际可能根据业务逻辑更复杂判断
location.href = "merchant_" + merchantName + ".html";
}
} else {
// 登录失败
$(".account").val("");
$(".password").val("");
$(".captchaInput").val("");
}
},
error:function(){
alert("请求失败")
}
})
})
})
</script>
</head>
<body>
<div class=" container">
<h2>登录</h2>
<input type="text" placeholder="请输入账号" class="account">
<input type="password" placeholder="请输入密码" class="password">
<div>
<input type="text" placeholder="请输入验证码" class="captchaInput">
<img src="CaptchaServlet" class='img'>
</div>
<select id='role' name='role' class="role">
<option value="user">用户</option>
<option value="admin">管理员</option>
<option value="merchant">商家</option>
</select>
<input type="button" value="登录" class="btn">
<span>没有账号?<a href="register.html">去注册</a></span>
</div>
</body>
</html>
css
css
*{
padding: 0;
margin: 0;
}
img{
width: 45px;
}
body{
height: 100vh;
background: linear-gradient(30deg,rgb(47,64,85),rgb(76,132,200));
}
.container{
width: 20%;
height: 30vh;
background: #fff;
margin: 35vh auto;
box-sizing: border-box;
min-width: 200px;
min-height: 300px;
border-radius: 30px;
display: flex;
flex-direction: column;
justify-content: space-around;
padding: 20px;
}
.container h2{
text-align: center;
color: rgb(47,64,85);
font-weight: 500;
}
.container input{
outline: none;
border: none;
border-bottom: 1px solid rgb(47,64,85);
padding: 4px 6px;
font-size: 13px;
}
.container div{
display: flex;
justify-content: space-between;
}
.container input[type=button]{
border: none;
color: #fff;
border-radius: 20px;
background: linear-gradient(30deg,rgb(47,64,85),rgb(76,132,200));
margin-top: 20px;
cursor: pointer;
}
.container input[type=button]:hover{
background: linear-gradient(-30deg,rgb(47,64,85),rgb(76,132,200));
}
.container span{
font-size: 12px;
color: rgb(47,64,85);
text-align: center;
cursor: pointer;
}
.container span a{
text-decoration: none;
color: rgb(76,132,200);
}
后端处理
java
package Servlet;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import cn.hutool.captcha.CaptchaUtil;
import cn.hutool.captcha.CircleCaptcha;
/**
* Servlet implementation class CaptchaServlet
*/
@WebServlet("/CaptchaServlet")
public class CaptchaServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public CaptchaServlet() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//定义图形验证码的长、宽、验证码字符数、干扰元素个数
CircleCaptcha captcha = CaptchaUtil.createCircleCaptcha(200, 100, 4, 20);
//CircleCaptcha captcha = new CircleCaptcha(200, 100, 4, 20);
//图形验证码写出,可以写出到文件,也可以写出到流
//captcha.write("d:/circle.png");
//验证图形验证码的有效性,返回boolean值
//captcha.verify("1234");
// 获取当前会话,如果不存在则创建一个新的会话
HttpSession session = request.getSession(true);
// 将生成的验证码的值保存到会话中,这里假设使用"captchaCode"作为属性名
session.setAttribute("captchaCode", captcha.getCode());
captcha.write(response.getOutputStream());
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
java
package Servlet;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import db.MysqlUtil;
/**
* Servlet implementation class LoginServlet
*/
@WebServlet("/LoginServlet")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public LoginServlet() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setCharacterEncoding("utf-8");
response.setContentType("text/json;charset=utf-8");
// 获取用户输入的验证码
String userInputCaptcha = request.getParameter("captchaInput");
// 获取保存验证码的会话
HttpSession session = request.getSession(false);
if (session!= null) {
// 从会话中获取之前生成并保存的验证码
String savedCaptcha = (String) session.getAttribute("captchaCode");
if (savedCaptcha!= null) {
// 比较用户输入的验证码和保存的验证码是否一致,忽略大小写
if (savedCaptcha.equalsIgnoreCase(userInputCaptcha)) {
// 验证码验证通过,继续进行账号和密码验证
String acc = request.getParameter("account");
String pass = request.getParameter("password");
String role = request.getParameter("role");
System.out.println(acc);
System.out.println(pass);
System.out.println(role);
String sql = "";
// 根据不同角色选择不同的数据库表进行查询验证
if ("user".equals(role)) {
sql = "SELECT * FROM user WHERE account = \"" + acc + "\" and password =\"" + pass + "\"";
} else if ("admin".equals(role)) {
sql = "SELECT * FROM admin WHERE account = \"" + acc + "\" and password =\"" + pass + "\"";
} else if ("merchant".equals(role)) {
sql = "SELECT * FROM merchants WHERE account = \"" + acc + "\" and password =\"" + pass + "\"";
}
int num = MysqlUtil.getCount(sql);
System.out.println(num);
String res = "{\"code\":0,\"message\":\"登陆失败\"}";
if (num > 0) {
res = "{\"code\":1,\"message\":\"登陆成功\"}";
// 登录成功,设置相关Cookie
// 设置标识用户已登录的Cookie,例如名为"isLoggedIn",值为"true"
Cookie loggedInCookie = new Cookie("isLoggedIn", "true");
// 设置Cookie的有效期,这里设置为1小时(单位是秒),可根据实际需求调整
loggedInCookie.setMaxAge(3600);
response.addCookie(loggedInCookie);
// 设置保存用户账号的Cookie,名为"userAccount",值为用户输入的账号
Cookie userAccountCookie = new Cookie("userAccount", acc);
userAccountCookie.setMaxAge(3600);
response.addCookie(userAccountCookie);
// 设置标识用户角色的Cookie,名为"user_role",值为对应的角色
Cookie roleCookie = new Cookie("user_role", role);
roleCookie.setMaxAge(3600);
response.addCookie(roleCookie);
if ("merchant".equals(role)) {
String idQuerySql = "SELECT merchant_id FROM merchants WHERE account = \"" + acc + "\" and password =\"" + pass + "\"";
String nameQuerySql = "SELECT merchant_name FROM merchants WHERE account = \"" + acc + "\" and password =\"" + pass + "\"";
Connection conn = null;
PreparedStatement pstmt = null;
PreparedStatement pstmtName = null;
ResultSet rs = null;
ResultSet rsName = null;
try {
// 建立数据库连接(这里假设你已经配置好了数据库连接相关信息,如URL、用户名、密码等)
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/shangcheng", "root", "root");
pstmt = conn.prepareStatement(idQuerySql);
pstmtName = conn.prepareStatement(nameQuerySql);
rs = pstmt.executeQuery();
rsName = pstmtName.executeQuery();
if (rs.next()&&rsName.next()) {
// 从结果集中获取merchant_id字段的值,假设merchant_id是整型,根据实际类型调整
int merchantId = rs.getInt("merchant_id");
String merchantName = rsName.getString("merchant_name");
Cookie merchantIdCookie = new Cookie("merchant_id", String.valueOf(merchantId));
merchantIdCookie.setMaxAge(3600);
response.addCookie(merchantIdCookie);
Cookie merchantNameCookie = new Cookie("merchant_name", merchantName);
merchantNameCookie.setMaxAge(3600);
response.addCookie(merchantNameCookie);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭资源,释放连接、语句和结果集对象,避免资源泄漏
try {
if (rs!= null) rs.close();
if (pstmt!= null) pstmt.close();
if (conn!= null) conn.close();
if (rsName!= null) rsName.close();
if (pstmtName!= null) pstmtName.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
// 返回数据
response.getWriter().write(res);
} else {
// 验证码验证不通过,直接返回错误信息
String res = "{\"code\": -1, \"message\":\"验证码错误,请重新输入\"}";
response.getWriter().write(res);
}
} else {
// 如果从会话中取不到保存的验证码,可能是会话过期等原因,返回相应错误信息
String res = "{\"code\": -2, \"message\":\"验证码已过期,请重新获取验证码\"}";
response.getWriter().write(res);
}
} else {
// 如果获取不到会话,可能是客户端未正常建立会话等原因,返回相应错误信息
String res = "{\"code\": -3, \"message\":\"未获取到会话,请重新操作\"}";
response.getWriter().write(res);
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
通过CaptchaServlet获取验证码 然后经过LoginServlet进行登录的处理返回给前端页面