这几天看到了小红书上有大量TikTok用户涌入,app端上外国用户描述的英文信息,因此想着研究一下Android端如何实现多语言功能,以下用一个最简单的demo演示一下:
1. 创建不同语言的资源文件
- 英语资源文件 (
res/values/strings.xml
):
XML
<resources>
<string name="app_name">My App</string>
<string name="greeting">Hello!</string>
<string name="change_language">Change Language</string>
</resources>
选中res目录,右键选中New -> Android Resource Directory
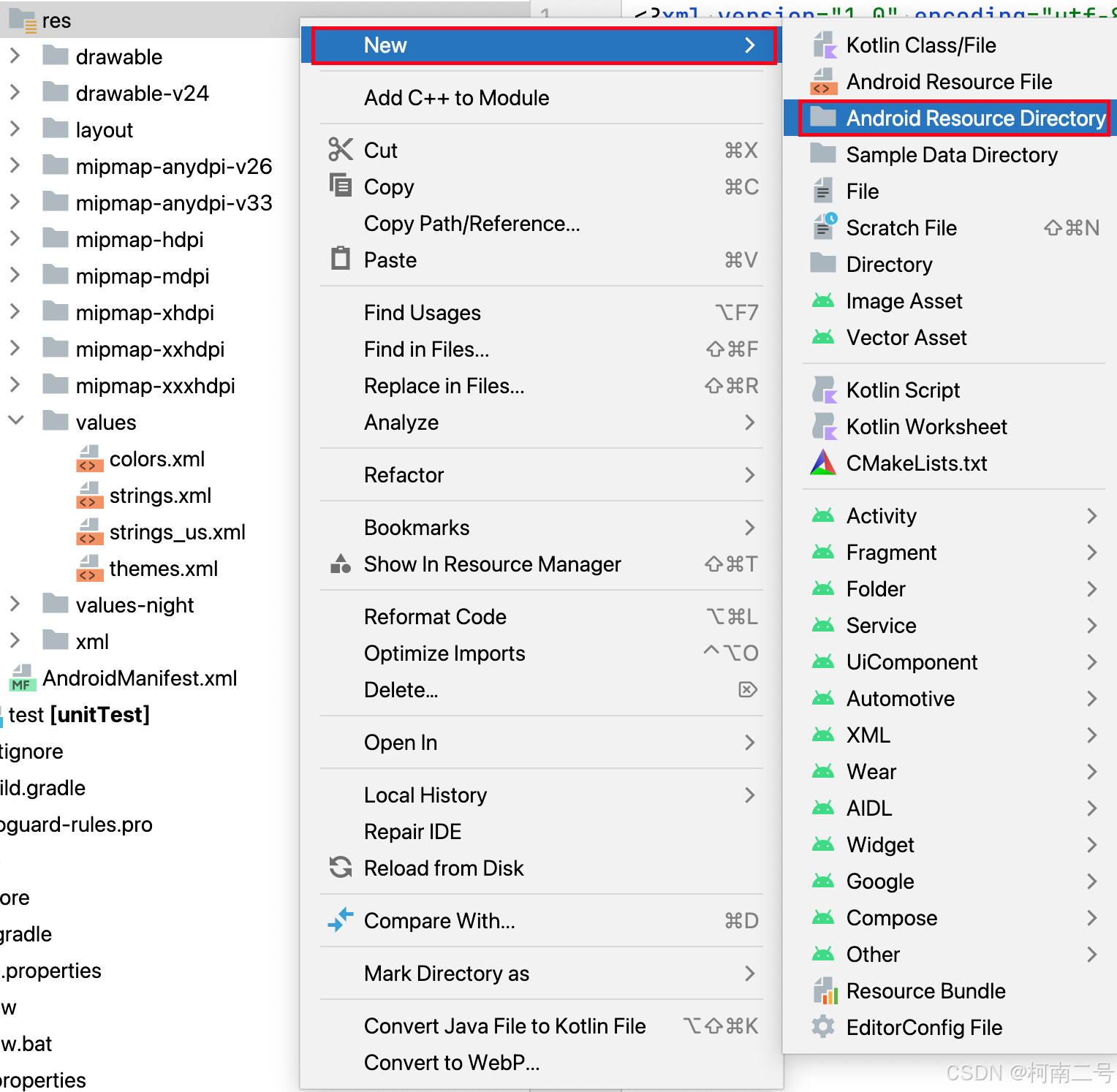
然后选中Chinese 选择中文
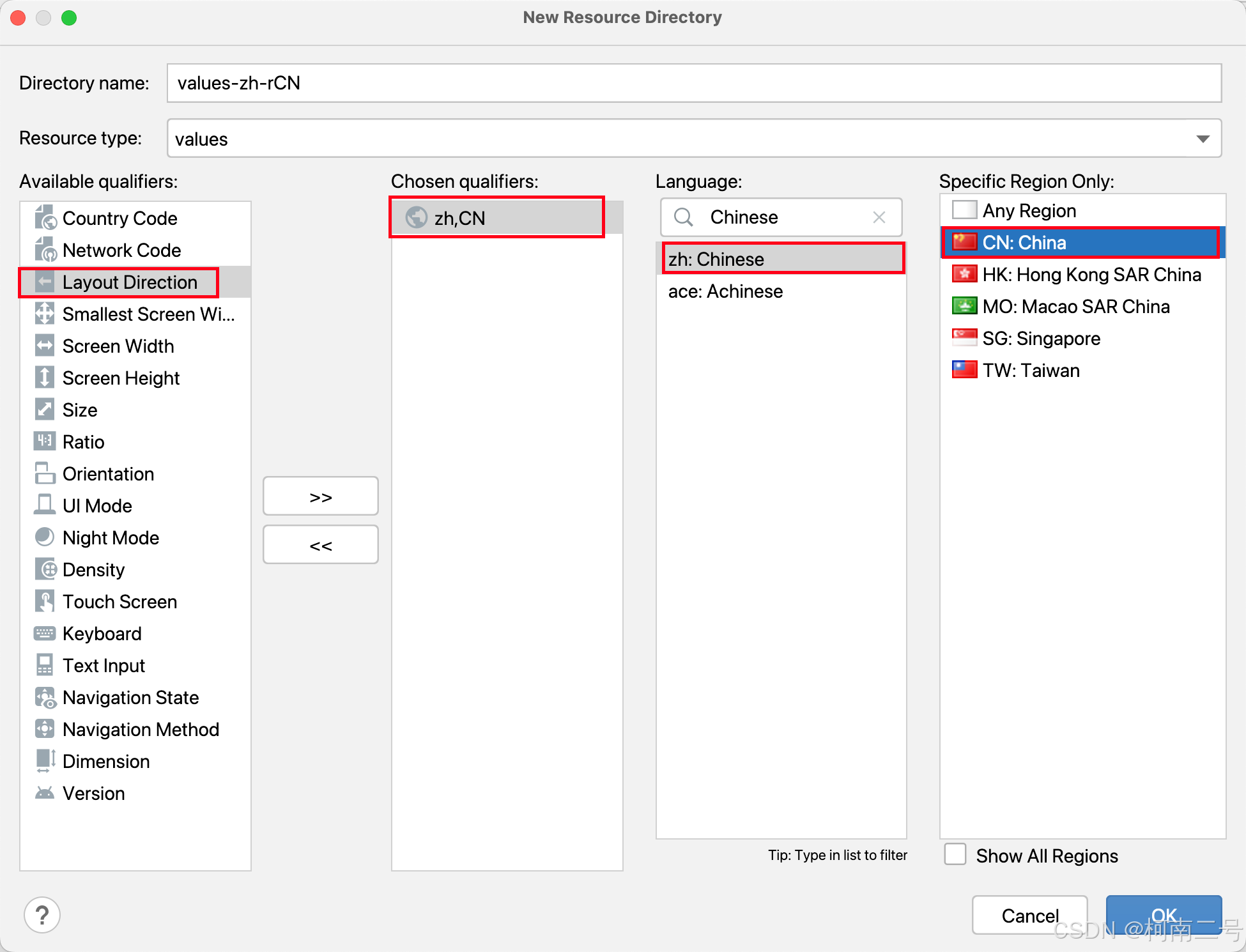
然后再在values-zh-rCN目录下,新建strings.xml文件
中文资源文件 (res/values-zh-rCN/strings.xml
):
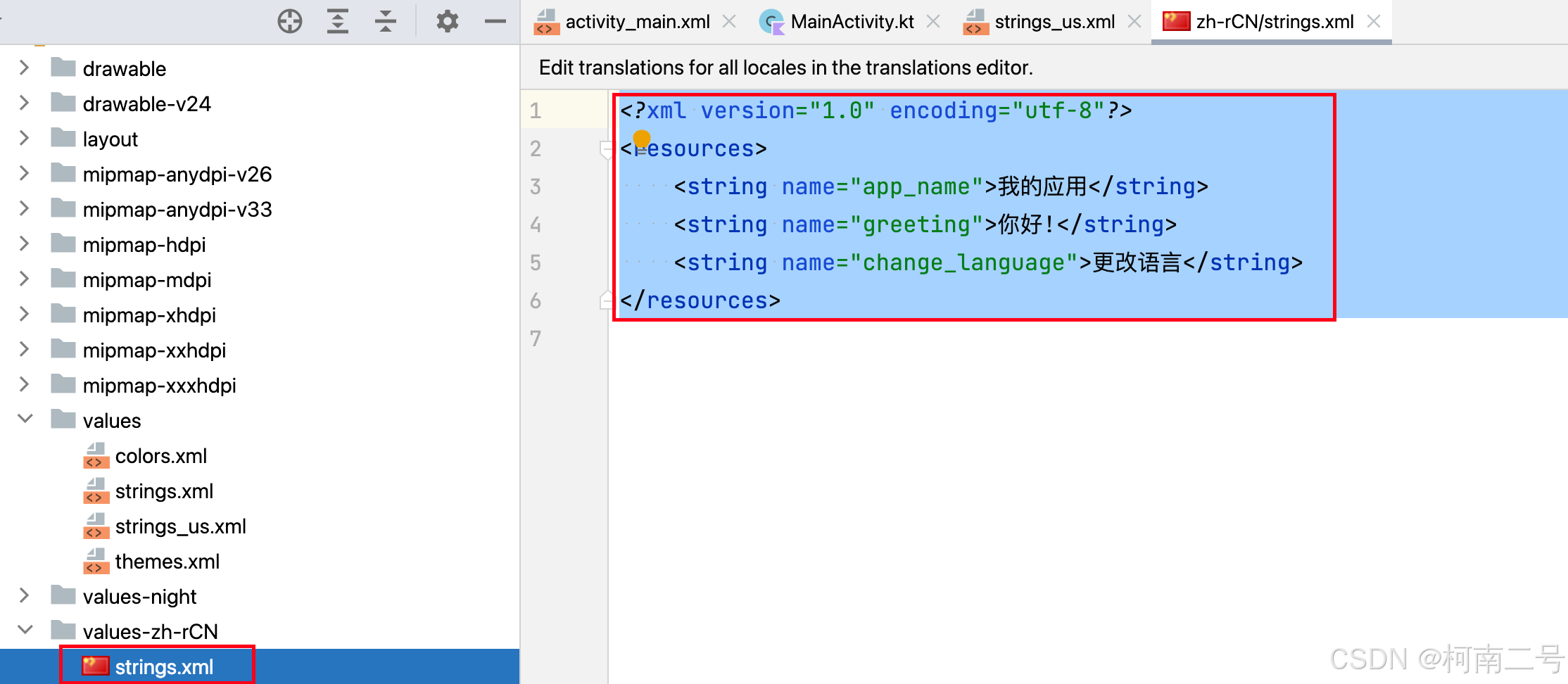
XML
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">我的应用</string>
<string name="greeting">你好!</string>
<string name="change_language">更改语言</string>
</resources>
这里需要删掉values目录下的strings.xml,不然这里面的会和strings_us.xml的资源冲突。
2. 创建一个语言设置工具类
此类负责更改应用的语言并更新界面。
Kotlin
import android.content.Context
import android.content.res.Configuration
import android.os.Build
import java.util.*
object LocaleHelper {
fun setLocale(context: Context, languageCode: String?) {
if (languageCode == null) {
return
}
val locale = Locale(languageCode)
Locale.setDefault(locale)
val config = Configuration()
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR1) {
config.setLocale(locale)
} else {
config.locale = locale
}
context.resources.updateConfiguration(
config,
context.resources.displayMetrics
)
// 保存语言设置
val preferences = context.getSharedPreferences("app_prefs", Context.MODE_PRIVATE)
val editor = preferences.edit()
editor.putString("language", languageCode)
editor.apply()
}
fun getLanguage(context: Context): String? {
val preferences = context.getSharedPreferences("app_prefs", Context.MODE_PRIVATE)
return preferences.getString("language", "en") // 默认语言为英语
}
}
3. 在MainActivity实现语言切换
主活动中添加按钮来切换语言,并显示当前的语言。
Kotlin
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private TextView greetingText;
private Button changeLanguageButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// 获取保存的语言设置并设置语言
String language = LocaleHelper.getLanguage(this);
LocaleHelper.setLocale(this, language);
setContentView(R.layout.activity_main);
greetingText = findViewById(R.id.greetingText);
changeLanguageButton = findViewById(R.id.changeLanguageButton);
// 设置问候语
greetingText.setText(getString(R.string.greeting));
// 切换语言
changeLanguageButton.setText(getString(R.string.change_language));
changeLanguageButton.setOnClickListener(v -> {
if ("en".equals(language)) {
LocaleHelper.setLocale(MainActivity.this, "zh");
} else {
LocaleHelper.setLocale(MainActivity.this, "en");
}
// 重新启动活动以应用语言更改
restartActivity();
});
}
private void restartActivity() {
Intent intent = getIntent();
finish();
startActivity(intent);
}
}
4. 布局文件 activity_main.xml
该布局包含一个文本视图来显示问候语,一个按钮来切换语言。
XML
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/greetingText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/greeting"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/changeLanguageButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/change_language"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/greetingText"
android:layout_marginTop="16dp" />
</androidx.constraintlayout.widget.ConstraintLayout>
5. 启动应用时设置语言
在 onCreate
方法中检查用户的语言设置并应用。
Kotlin
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// 获取用户的语言设置
String language = LocaleHelper.getLanguage(this);
LocaleHelper.setLocale(this, language);
setContentView(R.layout.activity_main);
}
6. 语言切换效果
-
用户点击按钮时,当前语言会切换,问候语和按钮文本会根据当前选择的语言更新。
-
语言设置会被保存在
SharedPreferences
中,确保用户下次启动应用时,语言设置不会丢失。
7. 运行效果
-
应用启动时会加载系统或用户保存的语言设置。
-
显示对应的问候语和按钮文本。
-
用户点击"Change Language"按钮后,应用语言会切换为中文或英文,并刷新界面。
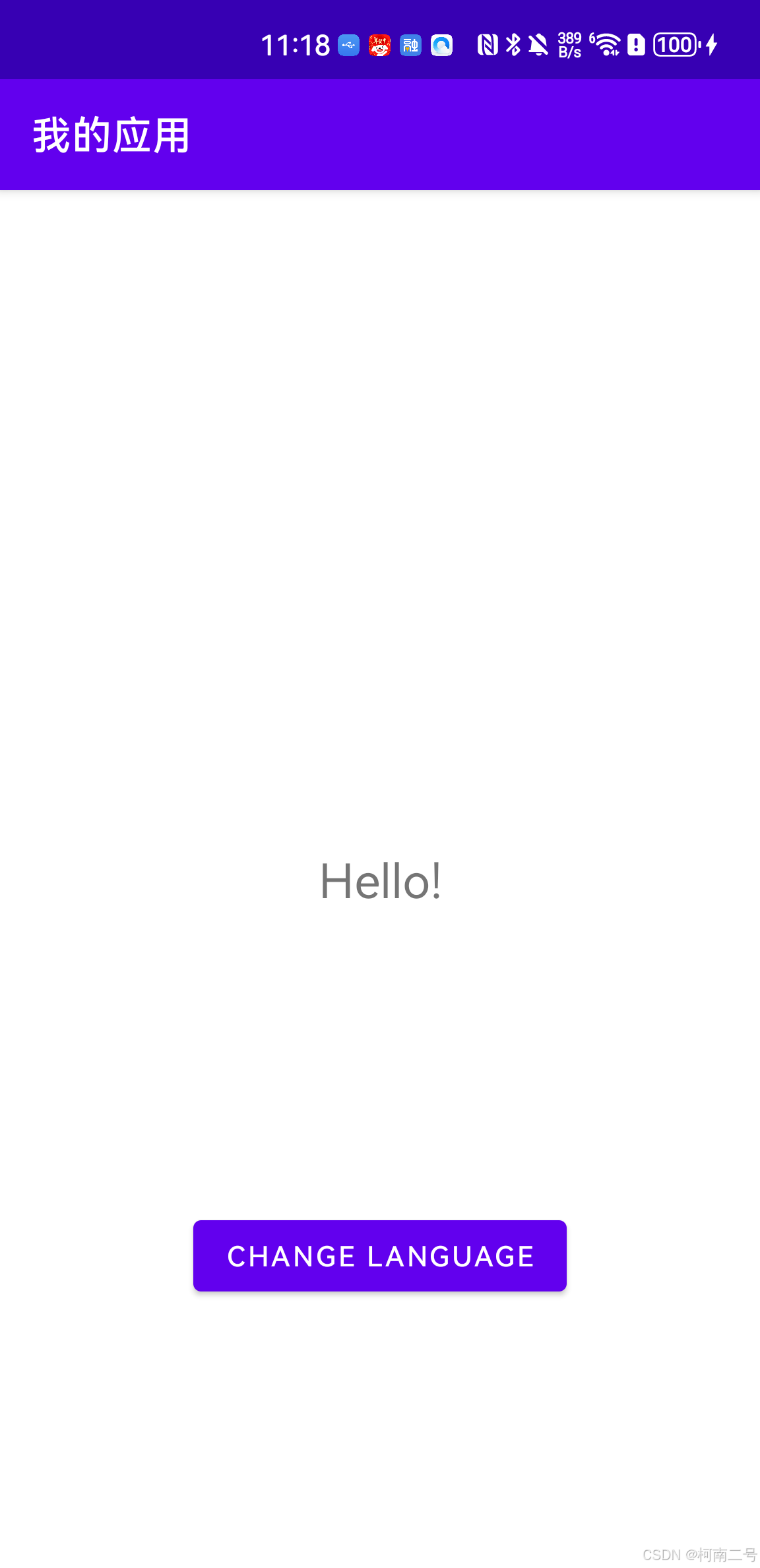
点击按钮之后,可以看到已经切换了语言
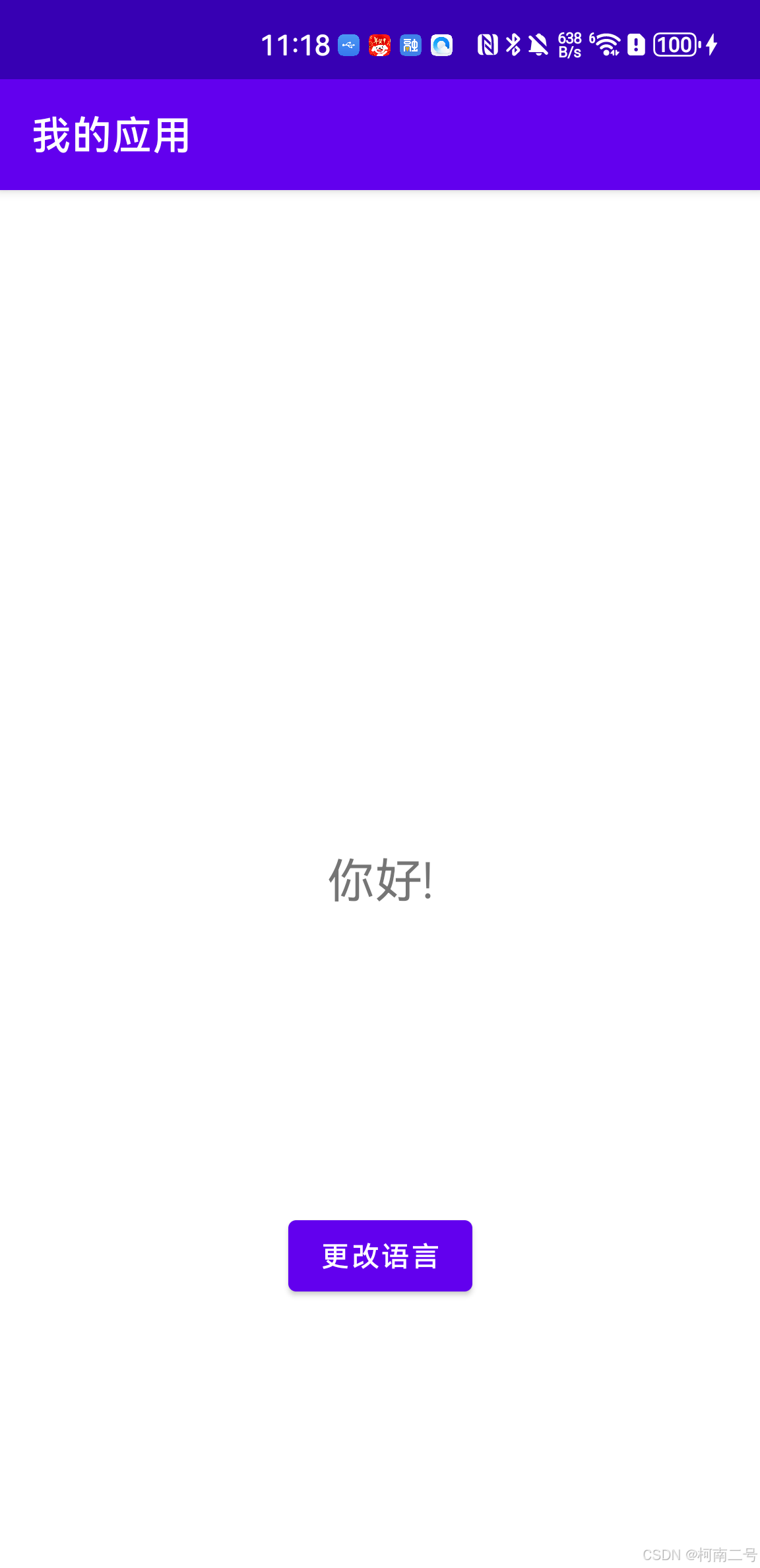