在本篇博客中,我们将介绍如何使用HarmonyOS NEXT框架从零开始构建一个简单的影视App,并重点实现"今日票房"页面的功能。我们将使用ArkUI组件库来搭建用户界面,并通过网络请求获取电影票房数据。
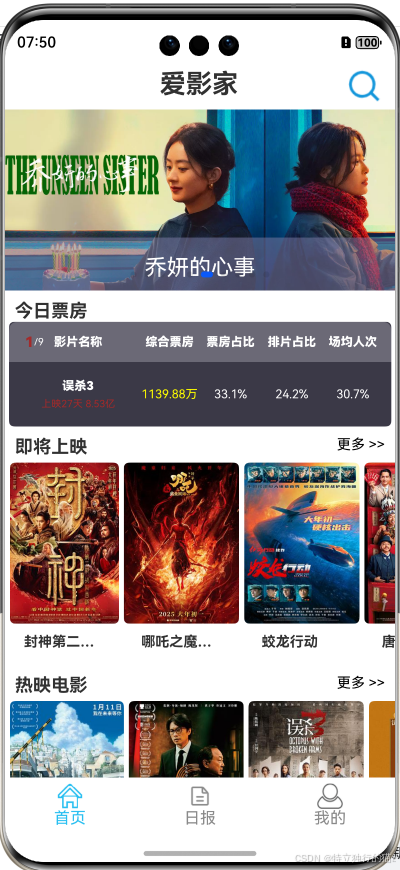
开源项目地址:https://atomgit.com/csdn-qq8864/hmmovie
项目准备
首先,我们需要创建一个新的HarmonyOS NEXT项目,并配置好必要的依赖。确保你已经安装了DevEco Studio,并创建了一个新的项目。
接口实现
在开始页面设计之前,我们需要先定义好获取电影票房数据的接口。我们使用axios来发送HTTP请求。在api
文件夹下创建一个movie.js
文件,编写获取电影票房数据的接口实现:
javascript
import {AxiosHttpRequest,HttpPromise} from '@nutpi/axios'
import {axiosClient,HttpPromise} from '../../utils/axiosClient';
// 电影票房接口
export const getPiaoMovie = (): HttpPromise<PiaoFangResp> => axiosClient.get({url:'/piaomovie'});
这里定义了一个getPiaoMovie
函数,用于发送GET请求获取电影票房数据。
数据模型定义
定义接口返回的数据模型,在bean
文件夹下创建一个PiaoFangResp.js
文件:
javascript
export interface PiaoFangRespData {
name: string; // 影片名称
release_date: string; // 上映日期
box_million: number; // 综合票房(百万)
share_box: number; // 票房占比
row_films: number; // 排片占比
row_seats: number; // 场均人次
}
export interface PiaoFangResp {
data: {
data: PiaoFangRespData[],
message: string,
};
}
页面设计与实现
接下来,我们将设计"今日票房"页面。页面的主要功能是展示今日票房最高的几部电影的相关信息,如影片名称、综合票房、票房占比、排片占比和场均人次。
我们创建一个名为TodayBoxOfficeView.ets
的文件来实现这个页面:
typescript
import { Log } from "../../utils/logutil";
import { getPiaoMovie } from "../api/movie";
import { PiaoFangRespData } from "../bean/PiaoFangResp";
import { BusinessError } from "@kit.BasicServicesKit";
@Extend(Text)
function tableHeaderText() {
.width('100%')
.height('100%')
.fontSize(12)
.fontWeight(FontWeight.Bolder)
.fontColor(Color.White)
.textAlign(TextAlign.Center)
}
@Extend(Text)
function tableBodyText() {
.width('100%')
.height('100%')
.fontSize(12)
.fontColor(Color.White)
.textAlign(TextAlign.Center)
}
@Component
export struct TodayBoxOfficeView {
private todayBoxOfficeScroller: SwiperController = new SwiperController();
@State todayBoxOfficeList: PiaoFangRespData[] = [];
build() {
Column() {
Text('今日票房').fontSize(18).fontWeight(FontWeight.Bold).padding({ left:10,top:10,right:10 }).alignSelf(ItemAlign.Start)
Column() {
Swiper(this.todayBoxOfficeScroller) {
ForEach(this.todayBoxOfficeList, (item: PiaoFangRespData) => {
TodayBoxOfficeCard({ todayBoxOffice: item })
})
}
.autoPlay(false)
.indicator(true)
.itemSpace(5)
.indicator(
Indicator.digit()
.top(10)
.left(8)
.fontColor(Color.White)
.selectedFontColor(Color.Brown)
.digitFont({ size: 10, weight: FontWeight.Normal })
.selectedDigitFont({ size: 14, weight: FontWeight.Bolder }))
}
.width('98%')
.backgroundColor('rgba(55, 53, 67, 0.98)')
.borderRadius(5)
}
.width('100%')
}
aboutToAppear(){
getPiaoMovie().then((res) => {
Log.debug(res.data.message);
for (const itm of res.data.data) {
this.todayBoxOfficeList.push(itm);
}
}).catch((err: BusinessError) => {
Log.debug("request", "err.data.code:%d", err.code);
Log.debug("request", err.message);
});
}
}
在TodayBoxOfficeView
结构中,我们使用Column
布局来组织页面结构,Text
组件来显示标题。Swiper
组件用于展示票房列表,ForEach
组件根据获取到的票房数据动态生成票房卡片。
定义单个票房卡片组件,TodayBoxOfficeCard
结构用于展示单个电影的票房信息:
typescript
@Component
struct TodayBoxOfficeCard {
@Prop todayBoxOffice: PiaoFangRespData;
build() {
Column() {
GridRow({
columns: 6
}) {
GridCol({ span: 2 }) {
Text('影片名称')
.tableHeaderText()
}
GridCol() {
Text('综合票房')
.tableHeaderText()
}
GridCol() {
Text('票房占比')
.tableHeaderText()
}
GridCol() {
Text('排片占比')
.tableHeaderText()
}
GridCol() {
Text('场均人次')
.tableHeaderText()
}
}
.width('100%')
.height(40)
.backgroundColor('rgba(108, 106, 119, 0.98)')
.borderRadius({ topLeft: 8, topRight: 8 })
.padding({ left: 8, right: 8 })
GridRow({
columns: 6
}) {
GridCol({ span: 2 }) {
Column({ space: 5 }) {
Text(this.todayBoxOffice.name)
.fontSize(12)
.fontWeight(FontWeight.Bolder)
.fontColor(Color.White)
Text(this.todayBoxOffice.release_date)
.fontSize(10)
.fontColor(Color.Brown)
}
.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
}
.width('100%')
.height('100%')
GridCol() {
Text(this.todayBoxOffice.box_million)
.tableBodyText()
.fontColor(Color.Yellow)
}
.width('100%')
.height('100%')
GridCol() {
Text(this.todayBoxOffice.share_box)
.tableBodyText()
}
.width('100%')
.height('100%')
GridCol() {
Text(this.todayBoxOffice.row_films)
.tableBodyText()
}
.width('100%')
.height('100%')
GridCol() {
Text(this.todayBoxOffice.row_seats)
.tableBodyText()
}
.width('100%')
.height('100%')
}
.width('100%')
.height(64)
.padding({ top: 16, left: 8, right: 8, bottom: 16 })
}
.width('100%')
}
}
TodayBoxOfficeCard
组件通过GridRow
和GridCol
布局来展示每部电影的详细信息。我们定义了两个装饰器函数:tableHeaderText
和tableBodyText
,用于设置表头和表格内容的样式。
页面加载数据
在TodayBoxOfficeView
组件生命周期函数aboutToAppear
中,我们调用getPiaoMovie
接口获取电影票房数据,并将数据存储在todayBoxOfficeList
状态变量中。todayBoxOfficeList
是PiaoFangRespData
类型的数组,用于存储电影票房信息。
结语
通过本篇博客,我们介绍了如何在HarmonyOS NEXT中实现一个简单的"今日票房"页面。从接口定义到页面布局,我们展示了如何使用ArkUI组件库来构建用户界面,并通过网络请求动态加载数据。希望这篇博客能帮助你更好地理解HarmonyOS NEXT的开发流程。如果你有任何问题或建议,请随时留言交流。
作者介绍
作者:csdn猫哥
原文链接:https://blog.csdn.net/yyz_1987
团队介绍
坚果派团队由坚果等人创建,团队拥有12个华为HDE带领热爱HarmonyOS/OpenHarmony的开发者,以及若干其他领域的三十余位万粉博主运营。专注于分享HarmonyOS/OpenHarmony、ArkUI-X、元服务、仓颉等相关内容,团队成员聚集在北京、上海、南京、深圳、广州、宁夏等地,目前已开发鸿蒙原生应用和三方库60+,欢迎交流。
版权声明
本文为博主原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。