1.install pyside6
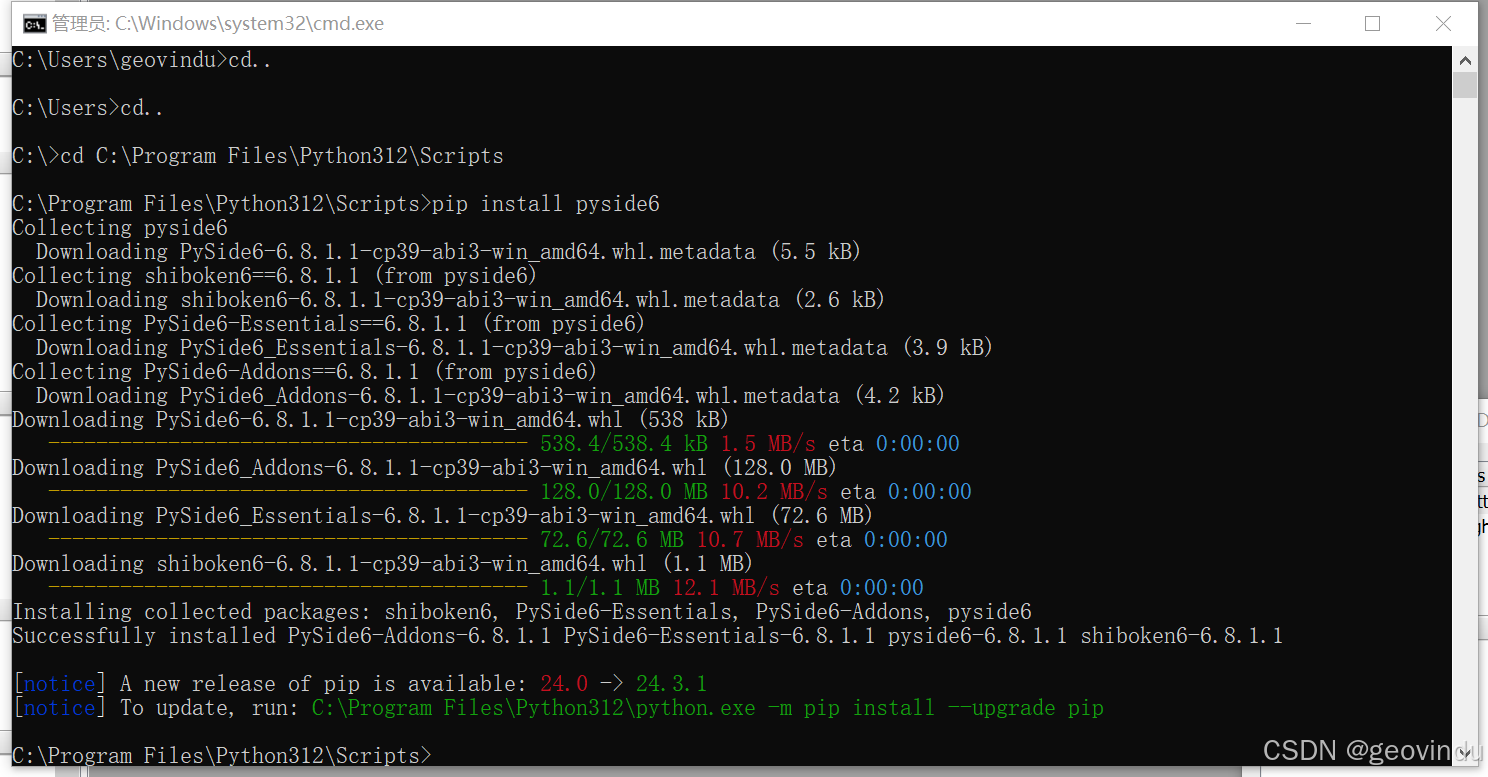
2.pyside6-designer.exe 发送到桌面快捷方式
在Python安装的所在 Scripts 文件夹下找到此文件。如C:\Program Files\Python312\Scripts
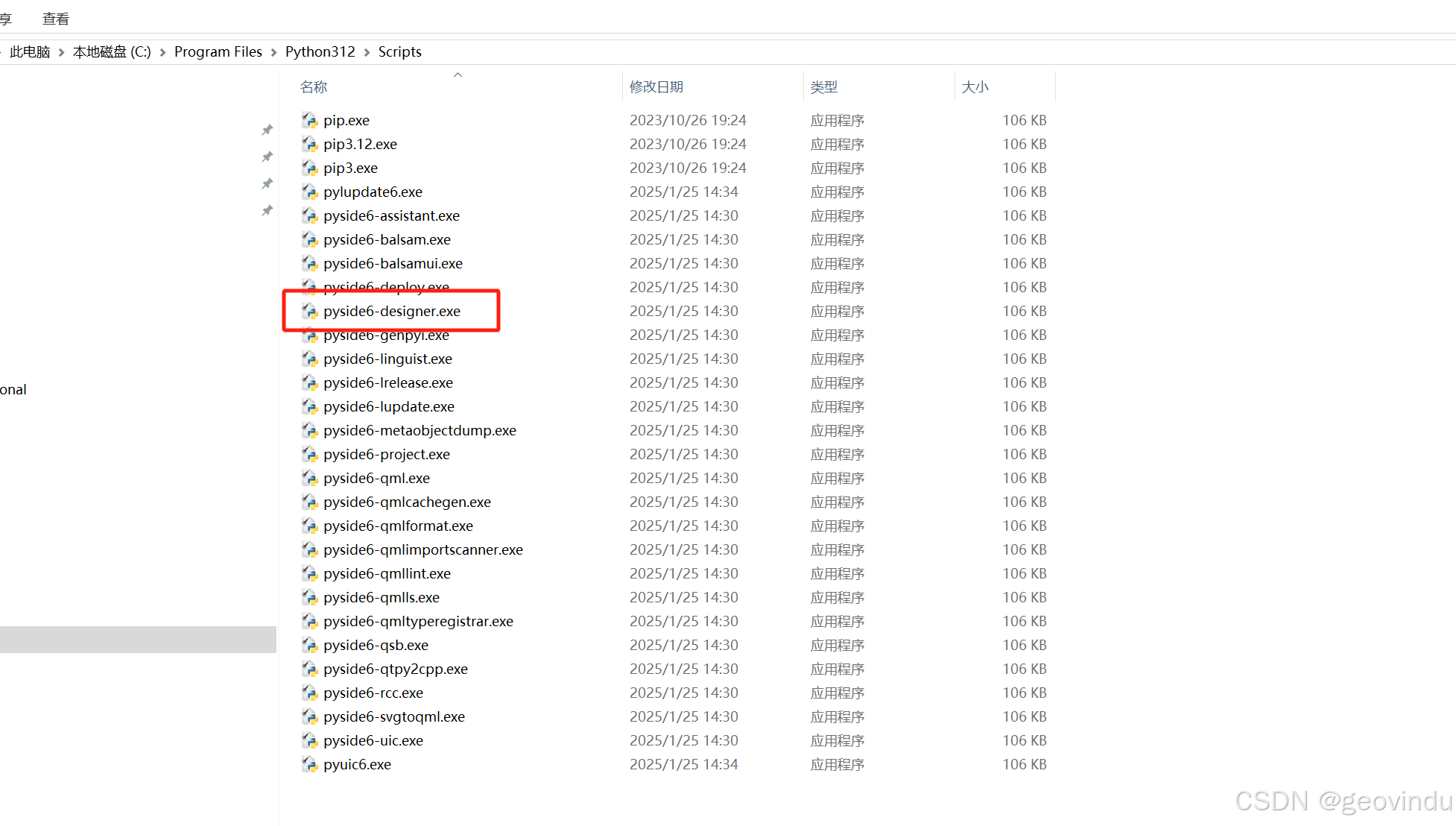
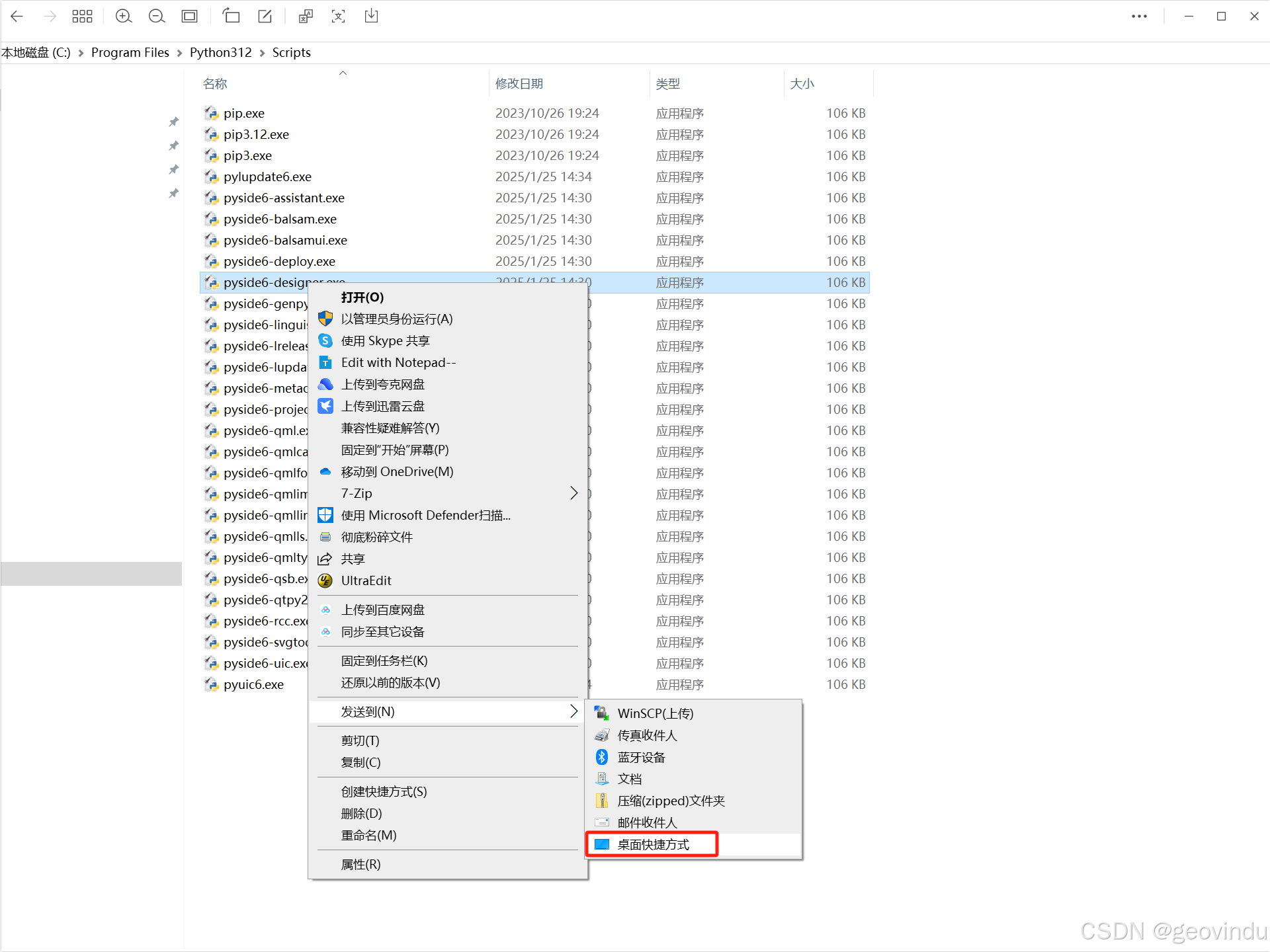
- 打开pyside6-designer 设计UI
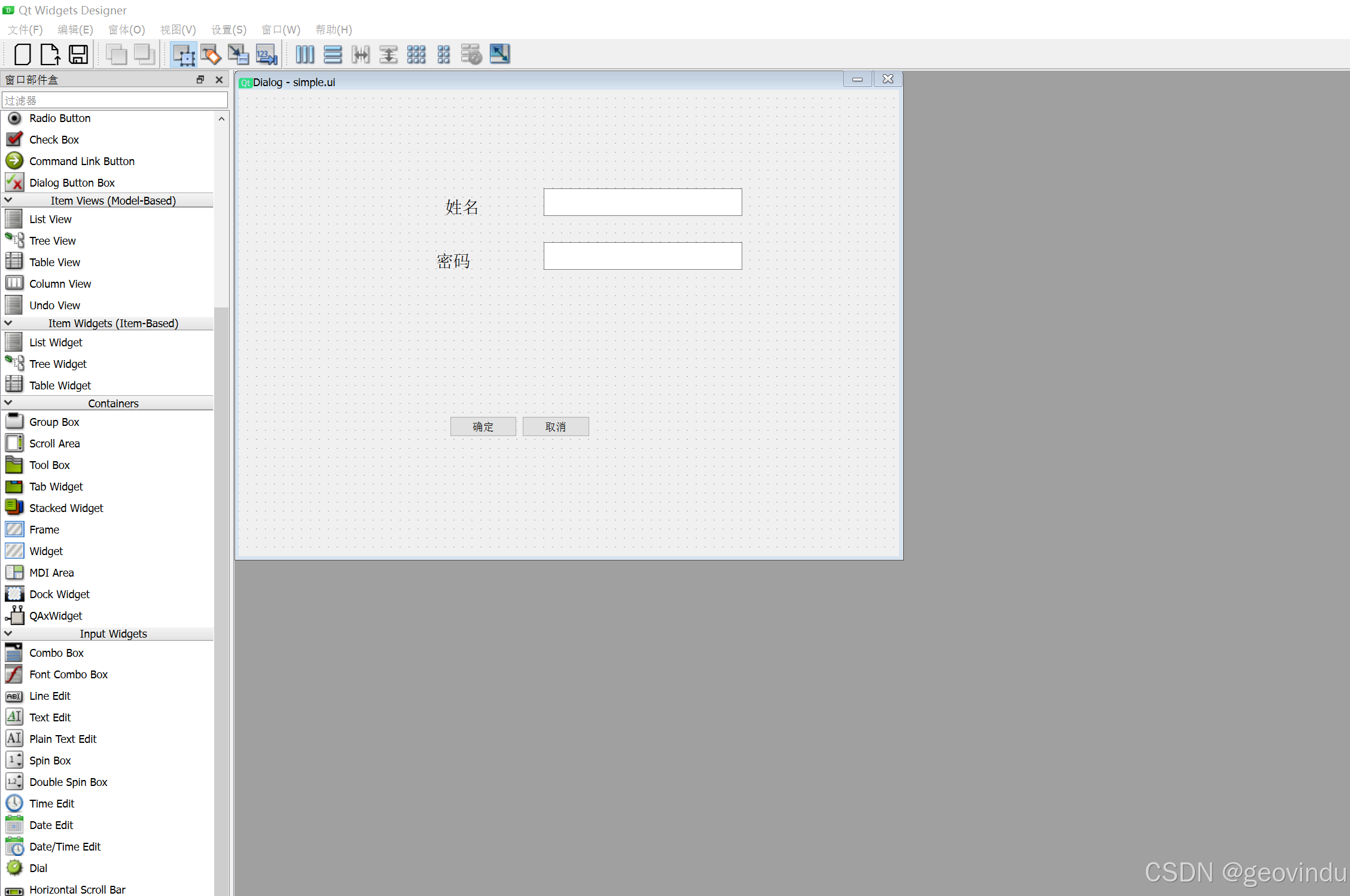
4.保存为simple.ui 文件,再转成py文件
用代码执行 pyside6-uic.exe simple.ui -o simple.py
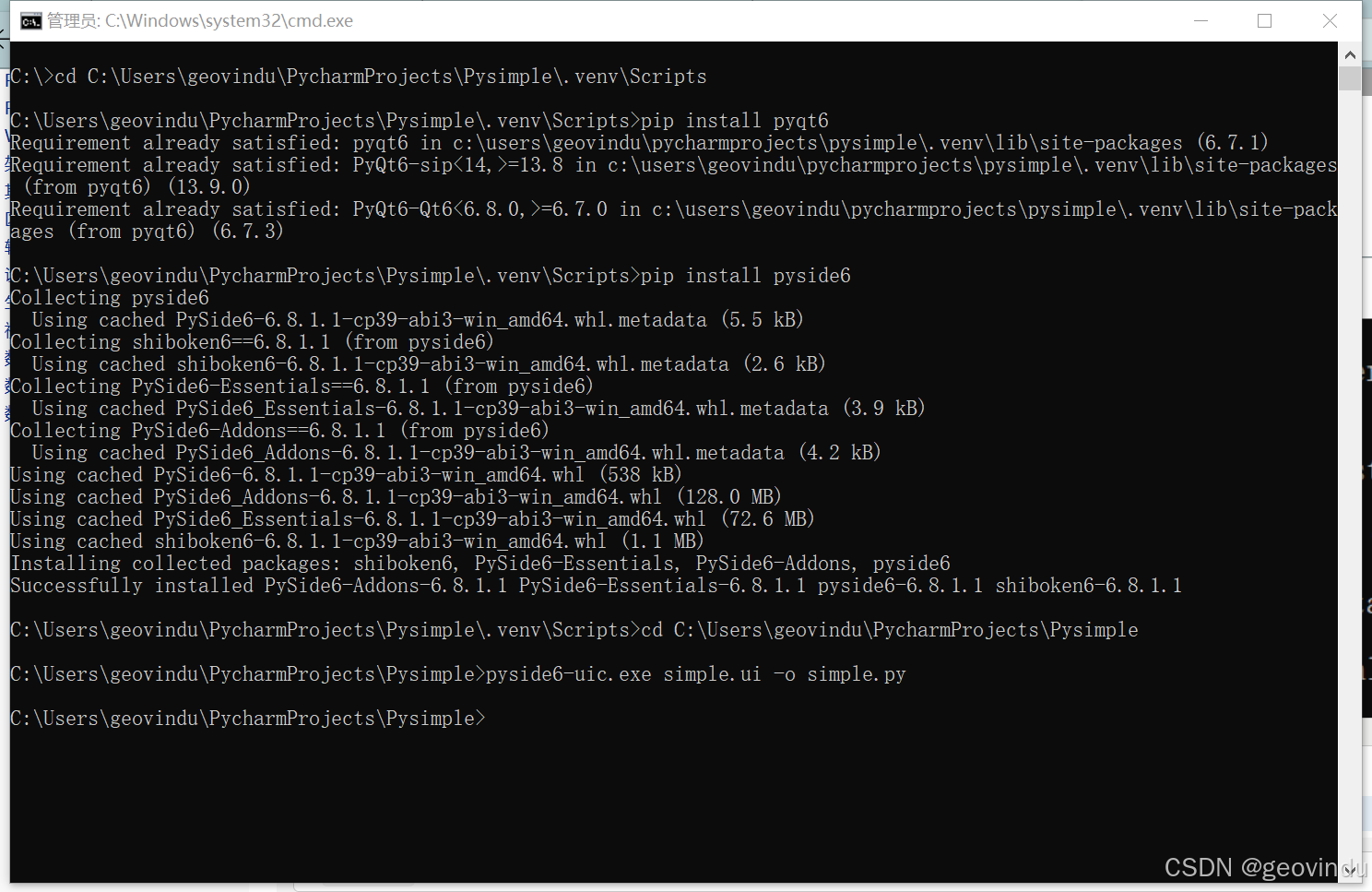
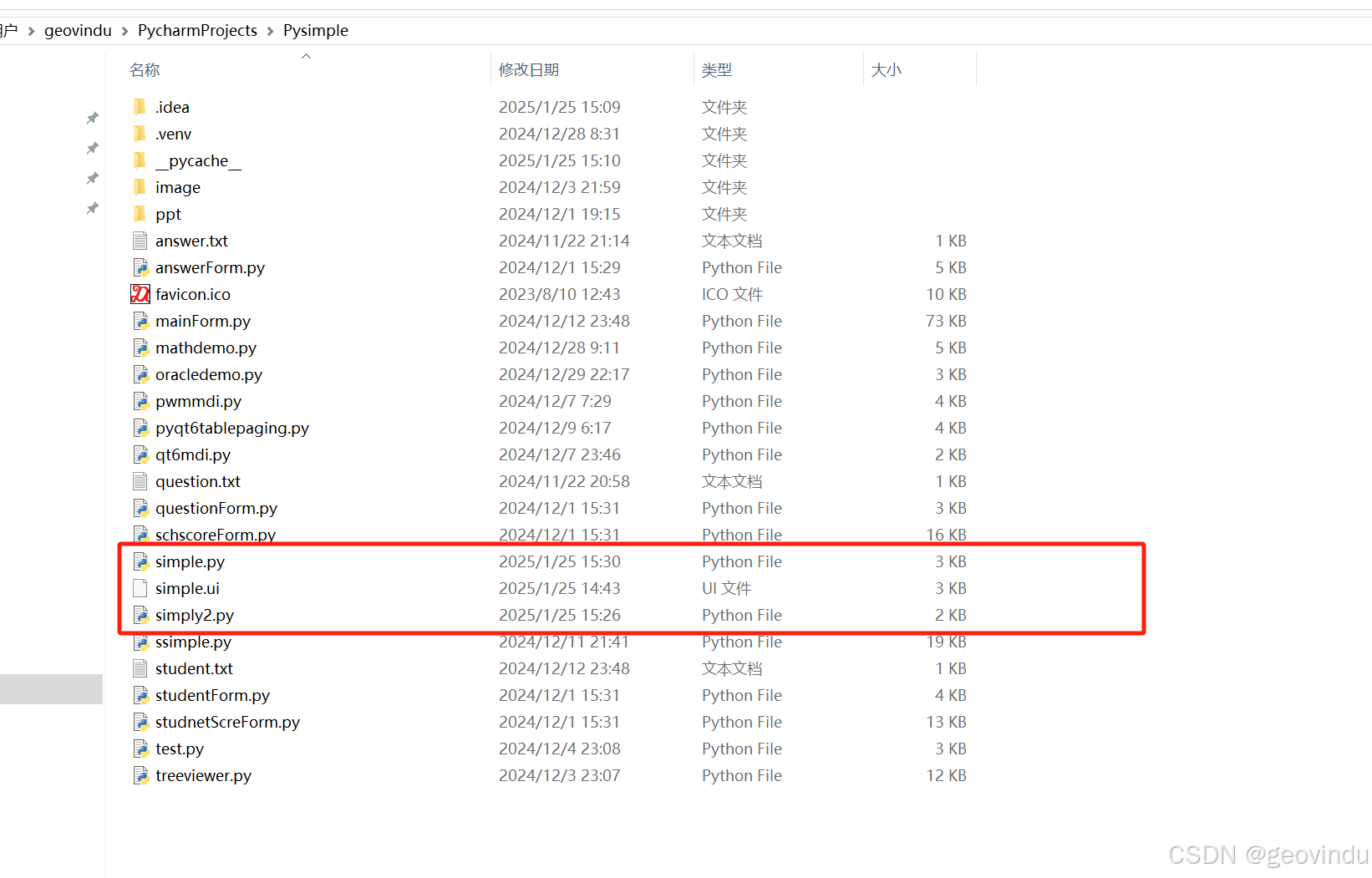
python
# -*- coding: utf-8 -*-
################################################################################
## Form generated from reading UI file 'simple.ui'
##
## Created by: Qt User Interface Compiler version 6.8.0
##
## WARNING! All changes made in this file will be lost when recompiling UI file!
################################################################################
from PySide6.QtCore import (QCoreApplication, QDate, QDateTime, QLocale,
QMetaObject, QObject, QPoint, QRect,
QSize, QTime, QUrl, Qt)
from PySide6.QtGui import (QBrush, QColor, QConicalGradient, QCursor,
QFont, QFontDatabase, QGradient, QIcon,
QImage, QKeySequence, QLinearGradient, QPainter,
QPalette, QPixmap, QRadialGradient, QTransform)
from PySide6.QtWidgets import (QAbstractButton, QApplication, QDialog, QDialogButtonBox,
QLabel, QSizePolicy, QTextEdit, QWidget)
class Ui_Dialog(object):
def setupUi(self, Dialog):
if not Dialog.objectName():
Dialog.setObjectName(u"Dialog")
Dialog.resize(736, 520)
self.buttonBox = QDialogButtonBox(Dialog)
self.buttonBox.setObjectName(u"buttonBox")
self.buttonBox.setGeometry(QRect(50, 360, 341, 32))
self.buttonBox.setOrientation(Qt.Orientation.Horizontal)
self.buttonBox.setStandardButtons(QDialogButtonBox.StandardButton.Cancel|QDialogButtonBox.StandardButton.Ok)
self.txtName = QTextEdit(Dialog)
self.txtName.setObjectName(u"txtName")
self.txtName.setGeometry(QRect(340, 110, 221, 31))
self.txtpassword = QTextEdit(Dialog)
self.txtpassword.setObjectName(u"txtpassword")
self.txtpassword.setGeometry(QRect(340, 170, 221, 31))
self.label = QLabel(Dialog)
self.label.setObjectName(u"label")
self.label.setGeometry(QRect(230, 120, 61, 21))
font = QFont()
font.setPointSize(14)
self.label.setFont(font)
self.label.setLineWidth(3)
self.label_2 = QLabel(Dialog)
self.label_2.setObjectName(u"label_2")
self.label_2.setGeometry(QRect(220, 180, 71, 21))
self.label_2.setFont(font)
self.retranslateUi(Dialog)
self.buttonBox.accepted.connect(Dialog.objectName)
self.buttonBox.rejected.connect(Dialog.objectName)
QMetaObject.connectSlotsByName(Dialog)
# setupUi
def retranslateUi(self, Dialog):
Dialog.setWindowTitle(QCoreApplication.translate("Dialog", u"Dialog", None))
self.label.setText(QCoreApplication.translate("Dialog", u"\u59d3\u540d", None))
self.label_2.setText(QCoreApplication.translate("Dialog", u"\u5bc6\u7801", None))
# retranslateUi
加上点击事件
python
# -*- coding: utf-8 -*-
################################################################################
## Form generated from reading UI file 'simple.ui'
##
## Created by: Qt User Interface Compiler version 6.8.0
##
## WARNING! All changes made in this file will be lost when recompiling UI file!
################################################################################
from PySide6.QtCore import (QCoreApplication, QDate, QDateTime, QLocale,
QMetaObject, QObject, QPoint, QRect,
QSize, QTime, QUrl, Qt)
from PySide6.QtGui import (QBrush, QColor, QConicalGradient, QCursor,
QFont, QFontDatabase, QGradient, QIcon,
QImage, QKeySequence, QLinearGradient, QPainter,
QPalette, QPixmap, QRadialGradient, QTransform)
from PySide6.QtWidgets import (QAbstractButton, QApplication, QDialog, QDialogButtonBox,
QLabel, QSizePolicy, QTextEdit, QWidget,QVBoxLayout)
class Ui_Dialog(object):
"""
"""
def setupUi(self, Dialog):
"""
:param Dialog:
:return:
"""
if not Dialog.objectName():
Dialog.setObjectName(u"Dialog")
Dialog.resize(736, 520)
self.buttonBox = QDialogButtonBox(Dialog)
self.buttonBox.setObjectName(u"buttonBox")
self.buttonBox.setGeometry(QRect(90, 280, 341, 32))
self.buttonBox.setOrientation(Qt.Orientation.Horizontal)
self.buttonBox.setStandardButtons(QDialogButtonBox.StandardButton.Cancel|QDialogButtonBox.StandardButton.Ok)
self.txtName = QTextEdit(Dialog)
self.txtName.setObjectName(u"txtName")
self.txtName.setGeometry(QRect(340, 110, 221, 31))
self.txtpassword = QTextEdit(Dialog)
self.txtpassword.setObjectName(u"txtpassword")
self.txtpassword.setGeometry(QRect(340, 170, 221, 31))
self.label = QLabel(Dialog)
self.label.setObjectName(u"label")
self.label.setGeometry(QRect(230, 120, 61, 21))
font = QFont()
font.setPointSize(14)
self.label.setFont(font)
self.label.setLineWidth(3)
self.label_2 = QLabel(Dialog)
self.label_2.setObjectName(u"label_2")
self.label_2.setGeometry(QRect(230, 180, 71, 21))
self.label_2.setFont(font)
self.retranslateUi(Dialog)
self.buttonBox.accepted.connect(Dialog.objectName)
self.buttonBox.rejected.connect(Dialog.objectName)
self.buttonBox.clicked.connect(self.buttonClicked)
QMetaObject.connectSlotsByName(Dialog)
# setupUi
def buttonClicked(self,button):
role = self.buttonBox.standardButton(button)
if role == QDialogButtonBox.StandardButton.Ok:
print("Save clicked")
elif role == QDialogButtonBox.StandardButton.Cancel:
print("Cancel clicked")
def retranslateUi(self, Dialog):
Dialog.setWindowTitle(QCoreApplication.translate("Dialog", u"Dialog\u6d4b\u8bd5", None))
#if QT_CONFIG(tooltip)
self.buttonBox.setToolTip(QCoreApplication.translate("Dialog", u"\u6309\u786e\u5b9a", None))
#endif // QT_CONFIG(tooltip)
self.label.setText(QCoreApplication.translate("Dialog", u"\u59d3\u540d", None))
self.label_2.setText(QCoreApplication.translate("Dialog", u"\u5bc6\u7801", None))
# retranslateUi
python
# -*- coding: utf-8 -*-
# 版权所有 2024 涂聚文有限公司
# 许可信息查看:言語成了邀功盡責的功臣,還需要行爲每日來值班嗎
# 描述:pip install pyqt6
# Author : geovindu,Geovin Du 涂聚文.
# IDE : PyCharm 2023.1 python 3.11
# os : windows 10
# database : mysql 9.0 sql server 2019, poostgreSQL 17.0
# Datetime : 2024/12/7 23:37
# User : geovindu
# Product : PyCharm
# Project : Pysimple
# File : simply2.py
# explain : 学习
import sys
from PySide6.QtWidgets import QApplication,QWidget
from simple import Ui_Dialog
class Windows(Ui_Dialog,QWidget):
"""
"""
def __init__(self):
"""
"""
super().__init__()
self.setupUi(self)
if __name__ == '__main__':
app=QApplication(sys.argv)
win=Windows()
win.show()
m=app.exec()
sys.exit(m)
测试运行结果:
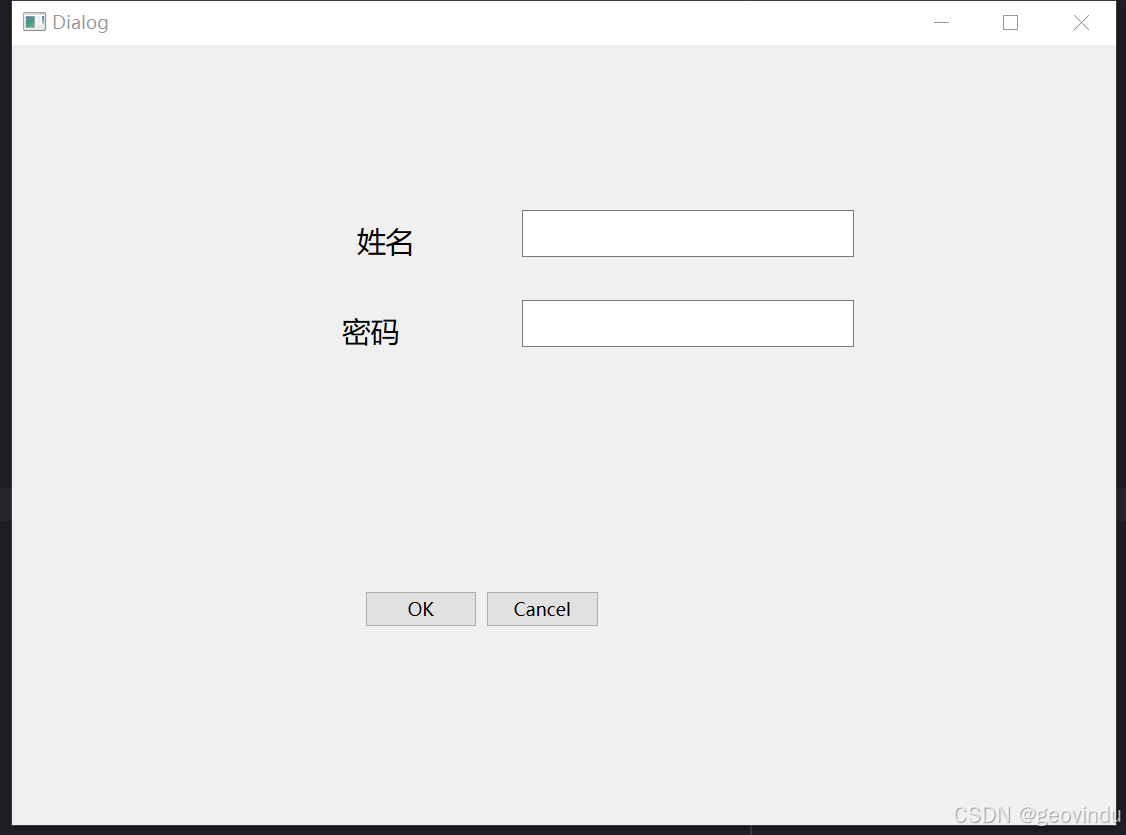