序列化 是指将对象转换为可存储或传输的格式,例如将对象转换为JSON字符串或字节流。反序列化则是将存储或传输的数据转换回对象的过程。这两个过程在数据持久化、数据交换以及与外部系统的通信中非常常见
把对象转换成josn字符串格式 这个过程就是序列化
josn字符串: 就是拥有固定格式的字符串
{ "name": "张三", "age": 25, "isStudent": true }
步骤:
1.在"引用"上右键
2.添加Nuget程序包
3.搜索"json"
4.点击下载"Newtonsoft.json"
5.在项目using Newtonsoft.json
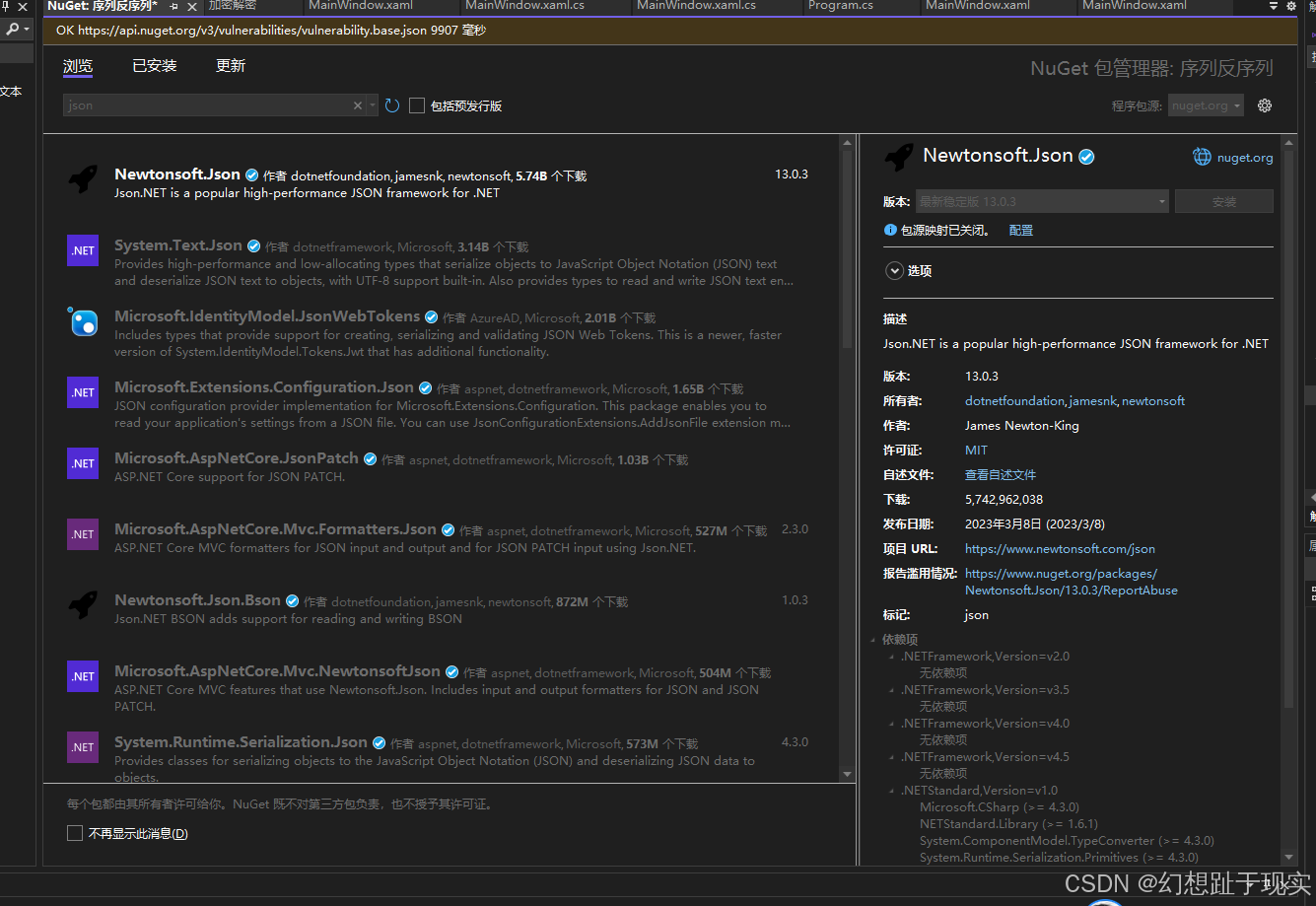
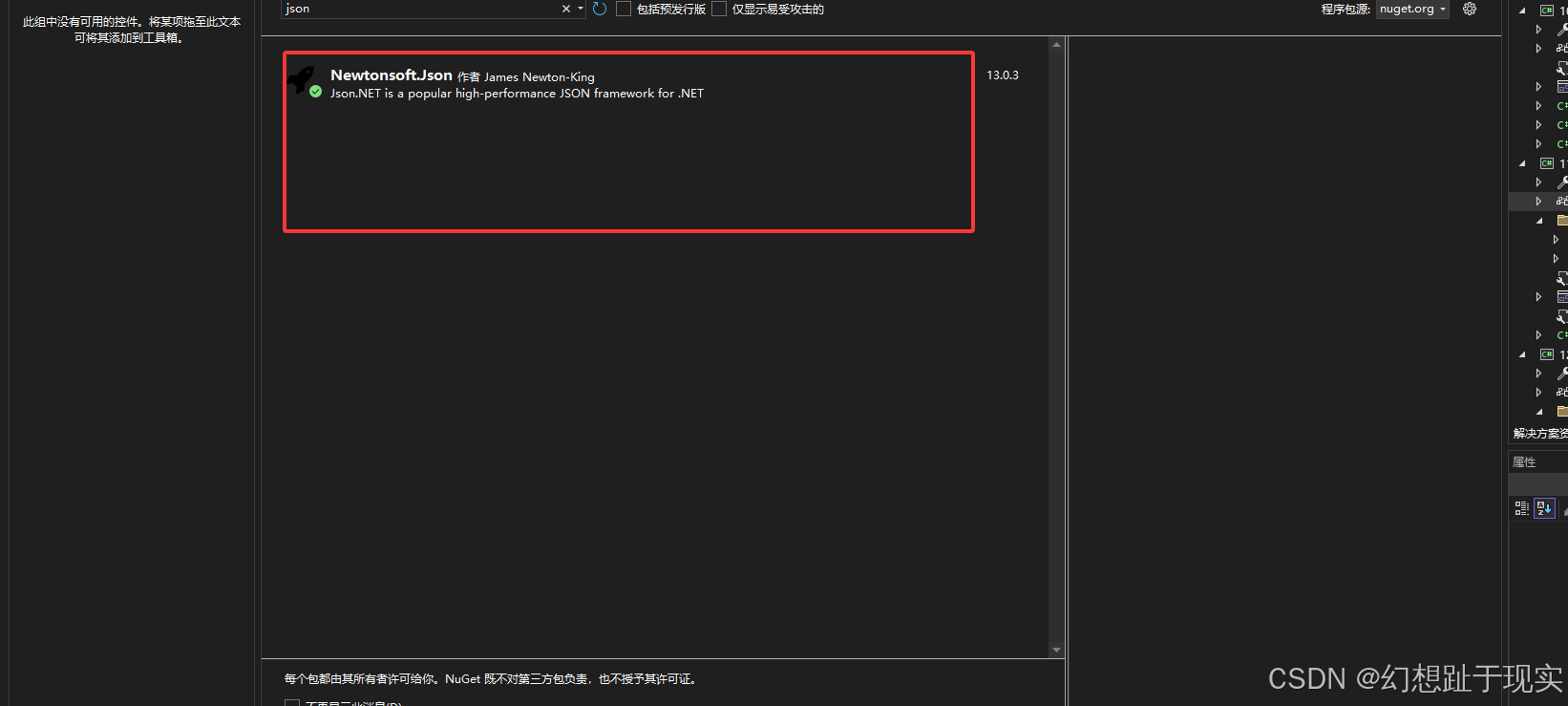
先声明两个类
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 序列反序列.Models
{
[Serializable]//表示这个类可以序列化
internal class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string Password { get; set; }
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 序列反序列.Models
{
internal class Product
{
public int Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public float Price { get; set; }
public User SaleUser { get; set; }
}
}

cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using 序列反序列.Models;
using Newtonsoft.Json;
namespace 序列反序列
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
string s=string.Empty;
private void button1_Click(object sender, RoutedEventArgs e)
{
Console.ForegroundColor = ConsoleColor.Red;
User user = new User() { Id = 1 , Name = "小张" ,Email="163.com",Password="123566"};
s=JsonConvert.SerializeObject(user);
Console.WriteLine(s);
Console.WriteLine("这上面是序列化的");
}
private void Button_Click(object sender, RoutedEventArgs e)
{
if (!string.IsNullOrWhiteSpace(s))
{
User newUser = JsonConvert.DeserializeObject<User>(s);
Console.WriteLine(newUser.Name);
}
else
{
Console.WriteLine("先序列化");
}
}
}
}
序列化
cs
Console.ForegroundColor = ConsoleColor.Red;
User user = new User() { Id = 1 , Name = "小张" ,Email="163.com",Password="123566"};
s=JsonConvert.SerializeObject(user);
Console.WriteLine(s);
Console.WriteLine("这上面是序列化的");
反序列化
cs
if (!string.IsNullOrWhiteSpace(s))
{
User newUser = JsonConvert.DeserializeObject<User>(s);
Console.WriteLine(newUser.Name);
}
else
{
Console.WriteLine("先序列化");
}
效果
对象嵌套序列化
cs
Product product = new Product()
{
Id = 1,
Name = "小明",
Description = "小明有一个狗",
Price = 1000.123f,
SaleUser = new User { Name = "小兰", Id = 2 }
};
string s = JsonConvert.SerializeObject(product, Formatting.Indented);
Console.WriteLine(s);
Product product1 = JsonConvert.DeserializeObject<Product>(s);
Console.WriteLine(product1.Name);
Console.WriteLine(product1.SaleUser.Name);
数组序列化
cs
User user1 = new User()
{
Id = 1,
Name = "小丑",
Email = "[email protected]",
Password = "1452"
};
User user2 = new User() { Id = 2, Name = "效率", Email = "[email protected]", Password = "246810" };
object[] objs = { user1, user2, true, "不知道" };
s2 = JsonConvert.SerializeObject(objs, Formatting.Indented);
Console.WriteLine(s2);
数组反序列化
cs
if (!string.IsNullOrWhiteSpace(s2))
{
object[] objs = JsonConvert.DeserializeObject<object[]>(s2);
Console.WriteLine(objs[1]);//字符串
User u = JsonConvert.DeserializeObject<User>(objs[1].ToString());
Console.WriteLine(u.Name);
}