一.问题分析
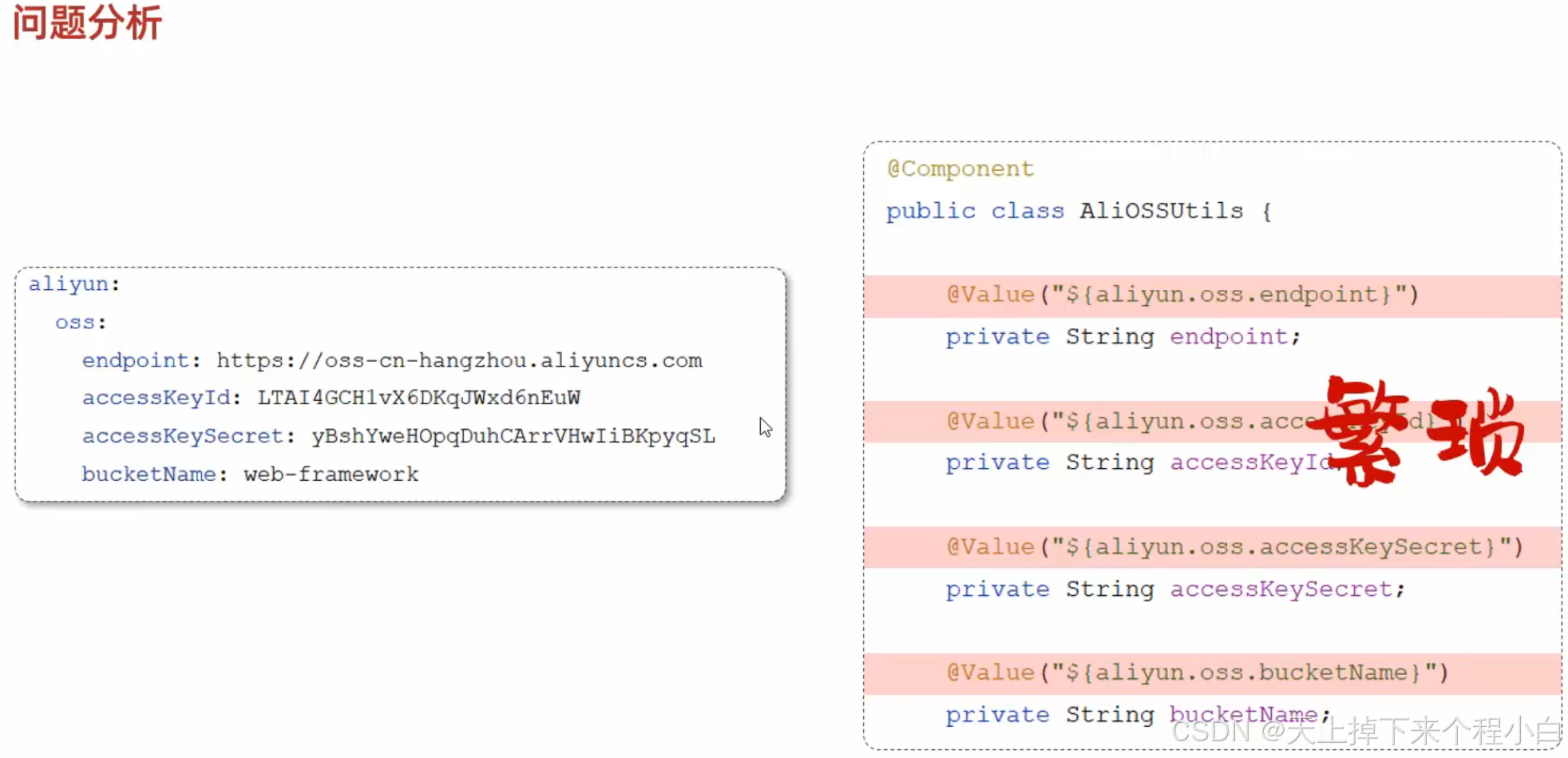
在之前我们配置阿里云OSS对象存储模型时,使用@Value注解将配置文件中的属性注入到阿里云OSS的utils工具类当中。但是如果要注入的属性特别多,那么使用@Value注解将十分繁琐,因为要一个一个的注入。
为了解决上述问题,我们可以引入@ConfigurationProperties注解来一起注入。
二.@ConfigurationProperties注解
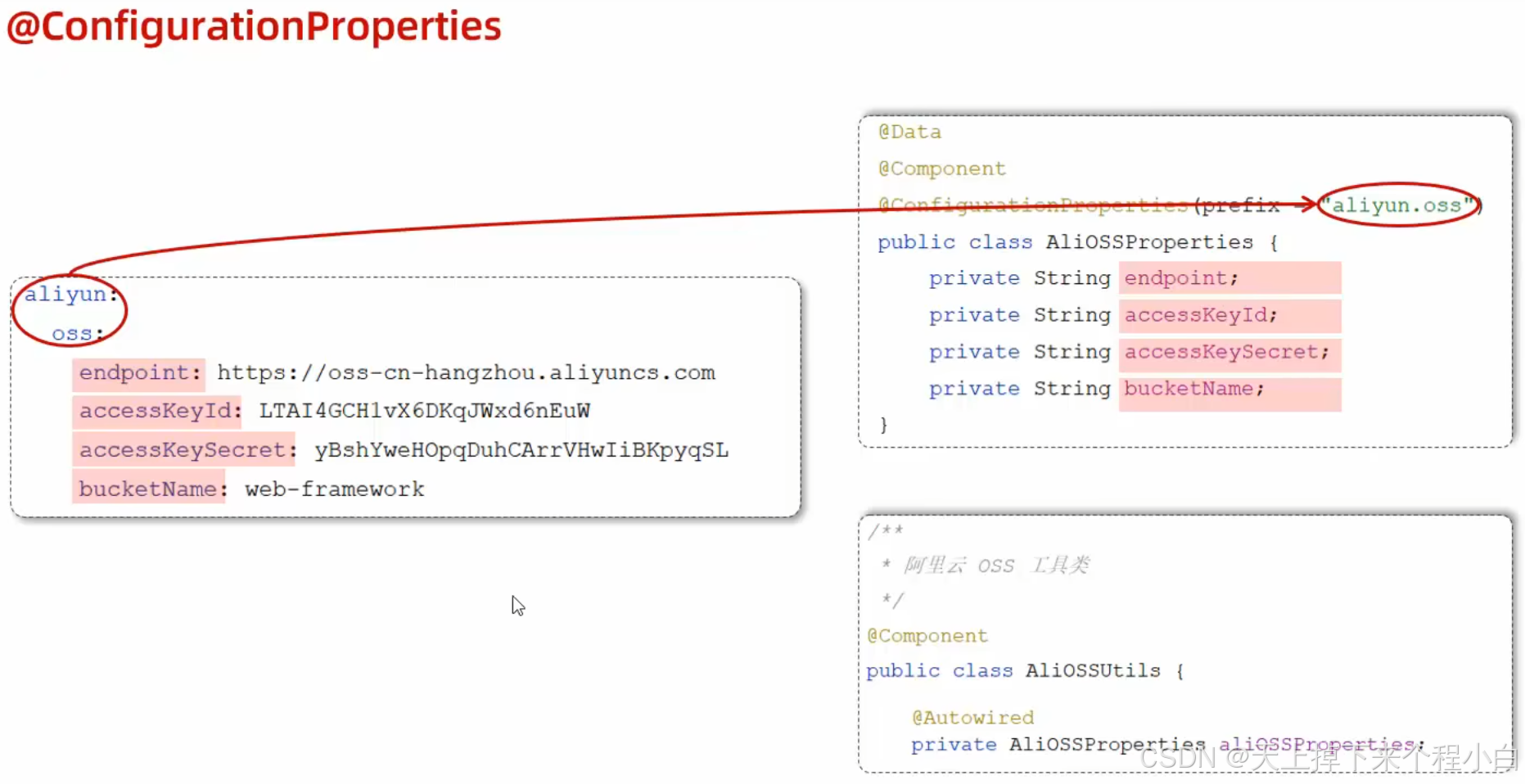
首先我们需要定义一个AliOSSProperties.java的属性类,将aliyun.oss的属性加入进去。并为该类加上@Data注解提供get/set方法,加上@Component注解将该类的实例对象作为bean对象注入到容器中,从而可以使用@Autowired注解进行依赖注入。
AliOSSProperties.java
java
package com.gjw.util;
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@Data
@ConfigurationProperties(prefix = "aliyun.oss")
public class AliOSSProperties {
private String endpoint;
private String accessKeyId;
private String accessKeySecret;
private String bucketName;
}
我们要使用@ConfigurationProperties注解,并在@ConfigurationProperties注解中定义prefix属性来指定要配置的属性名的前缀。指定之后,@ConfigurationProperties注解就可以实现将对应属性和配置文件中的属性相对应,进而实现值传递。
AliOSSUtils.java
java
package com.gjw.util;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import lombok.Data;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.UUID;
/**
* 阿里云 OSS 工具类
*/
@Data
@Component // 使用Component实现控制反转(该工具类不属于controller,service,mapper/Dao)
public class AliOSSUtils {
/*
@Value("${aliyun.oss.endpoint}")
private String endpoint;
@Value("${aliyun.oss.accessKeyId}")
private String accessKeyId;
@Value("${aliyun.oss.accessKeySecret}")
private String accessKeySecret;
@Value("${aliyun.oss.bucketName}")
private String bucketName;
*/
@Autowired
private AliOSSProperties aliOSSProperties;
/**
* 实现上传图片到OSS
*/
public String upload(MultipartFile file) throws IOException {
String endpoint = aliOSSProperties.getEndpoint();
String accessKeyId = aliOSSProperties.getAccessKeyId();
String accessKeySecret = aliOSSProperties.getAccessKeySecret();
String bucketName = aliOSSProperties.getBucketName();
// 获取上传的文件的输入流
InputStream inputStream = file.getInputStream();
// 避免文件覆盖
String originalFilename = file.getOriginalFilename();
String fileName = UUID.randomUUID().toString() + originalFilename.substring(originalFilename.lastIndexOf("."));
//上传文件到 OSS
OSS ossClient = new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
ossClient.putObject(bucketName, fileName, inputStream);
//文件访问路径
String url = endpoint.split("//")[0] + "//" + bucketName + "." + endpoint.split("//")[1] + "/" + fileName;
// 关闭ossClient
ossClient.shutdown();
return url;// 把上传到oss的路径返回
}
}
在AliOSSUtils.java中,我们通过依赖注入将AliOSSProperties的实例化对象注入进来,并调用其get方法即可完成对应配置的属性值的获取,进而在工具类中使用。
三.@ConfigurationProperties和@Value注解的对比
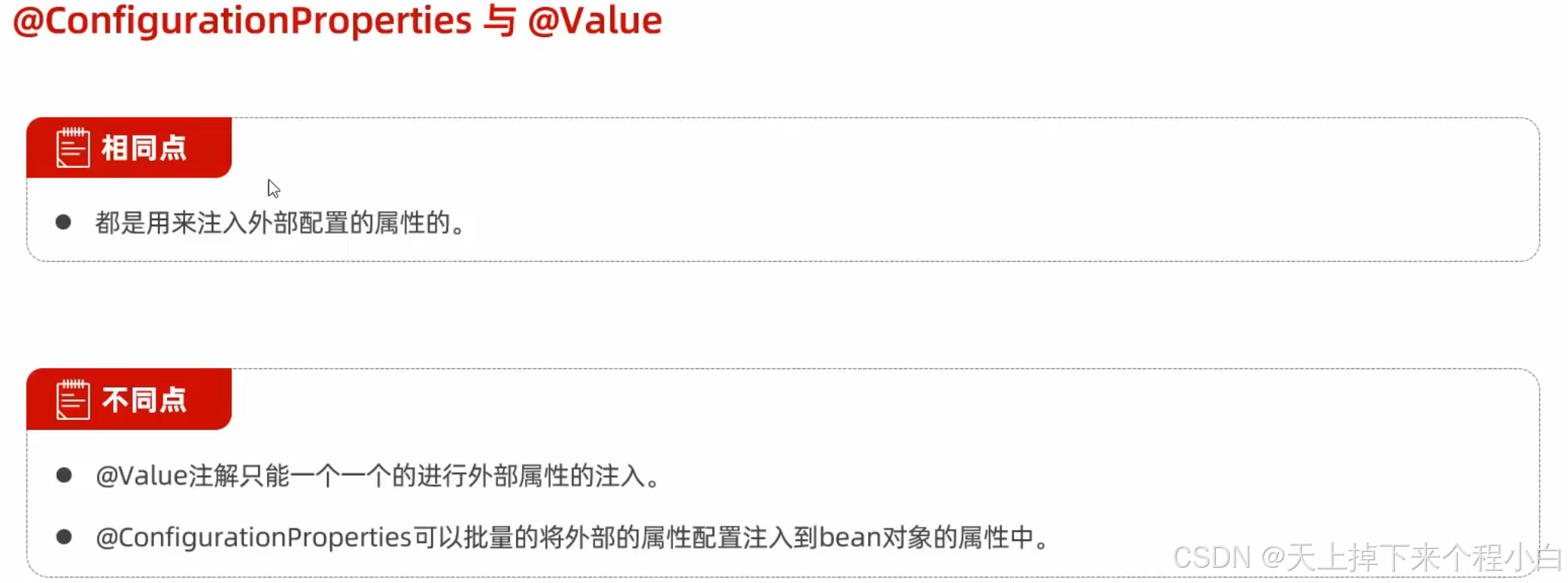
四.解决出现的警告(可选)
当使用@ConfigurationProperties注解后,会出现警告,但不影响程序运行。原因是我们没有配置依赖。在pom文件中配置一下该依赖即可,配置后我们在yml文件中输入aliyun后会自动提示出来其要配置的属性值。
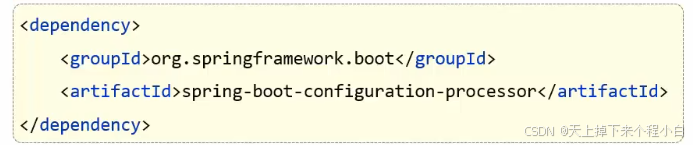
pom文件中加入此,解决。
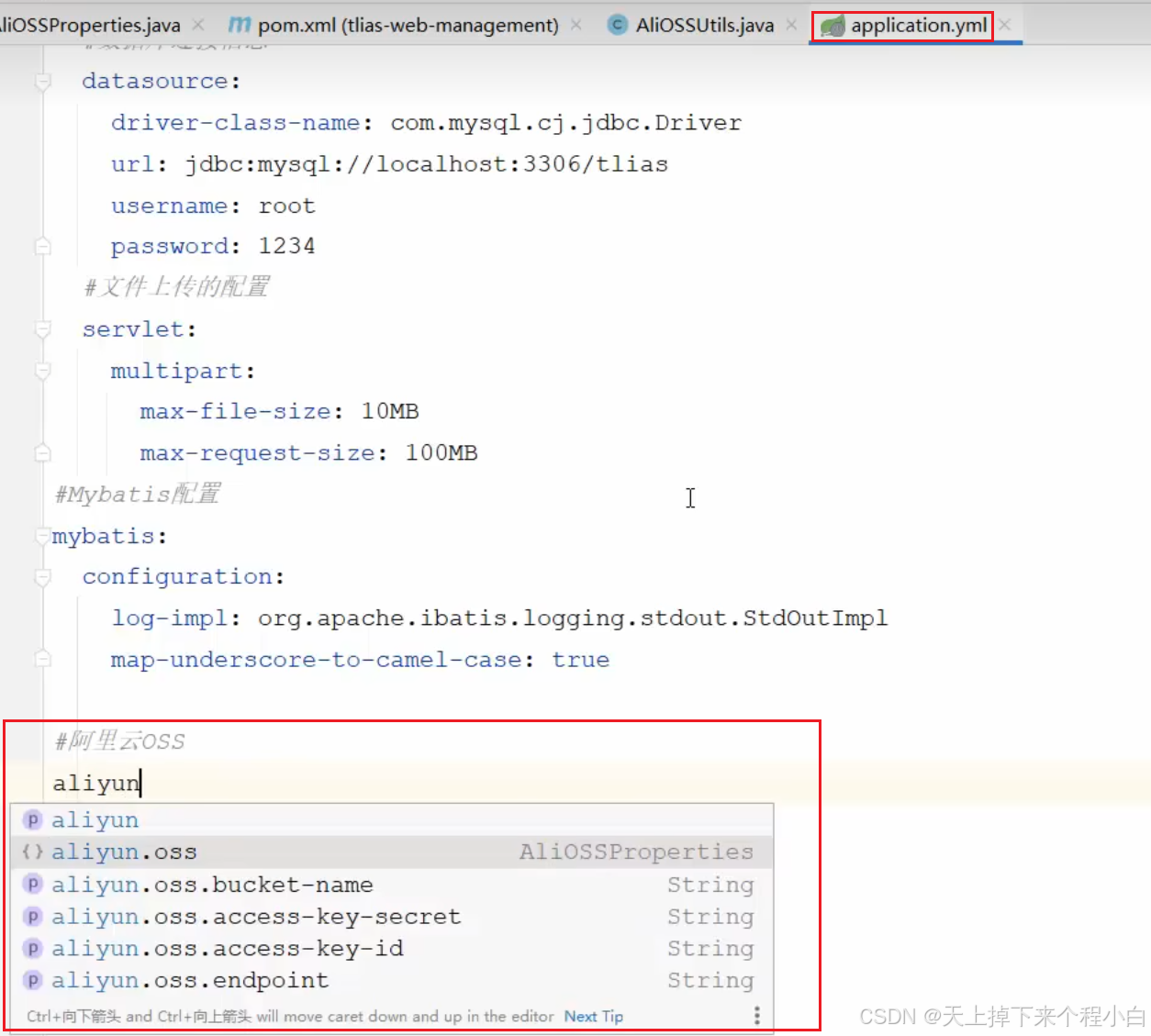