想要使用vue3导出表格内容并且图片显示在表格中(如图):
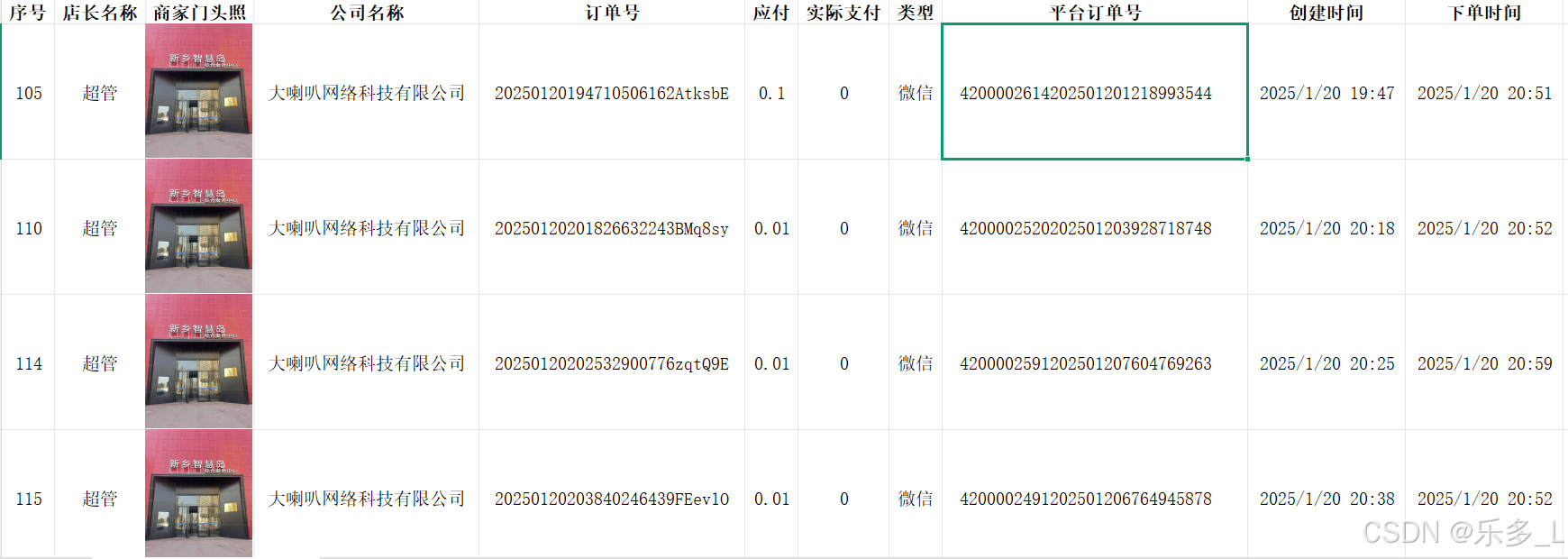
步骤如下:
下载安装插件:
安装命令:npm install js-table2excel
引入插件:
javascript
import table2excel from 'js-table2excel'
使用插件
直接上代码:
onBatchExport方法中数据的key值要与data中保持一致,否则数据无法获取到,打印出的结果就回为undefined。
我写了两种导出:
一种是全部导出;另一种是选中导出。
选中导出需要结合列表中的复选框,给复选框绑定onchange事件,声明变量,把选中的数据存到到变量中,然后给导出的数据换成变量,就可以只导出选中的列表。
下面是全部导出:
javascript
<template>
<div>
<el-button size="big" type="primary" @click="open">导出</el-button>
</div>
</template>
<script>
import table2excel from "js-table2excel";
// 账号列表总数据
const datum = ref([]);
// 导出框显示
// 导出全部数据的函数
const exportBtn = () => {
const column = [
{
title: "序号",
key: "id",
type: "text",
},
{
title: "店长名称",
key: "name",
type: "text",
},
{
title: "商家门头照",
key: "img",
type: "image",
width: 120,
height: 150,
},
{
title: "公司名称",
key: "title",
type: "text",
},
{
title: "订单号",
key: "order_num",
type: "text",
},
{
title: "应付",
key: "money",
type: "text",
},
{
title: "实际支付",
key: "fact_money",
type: "text",
},
{
title: "类型",
key: "type",
type: "text",
formatter: (row) => {
const typeMap = {
1: "微信",
2: "支付宝",
3: "抖音",
};
return typeMap[row.type] || "未知";
},
},
{
title: "平台订单号",
key: "terrace_order_num",
type: "text",
width: 500, // 自动调整宽度
numberFormat: "text",
},
{
title: "创建时间",
key: "create_time",
type: "text",
},
{
title: "下单时间",
key: "pay_time",
type: "text",
},
];
// 深拷贝数据
const tableDatas = JSON.parse(JSON.stringify(datum.value));
// 处理数据,将 type 字段的值转换为对应的文字
const processedDatas = tableDatas.map((item) => {
const typeMap = {
1: "微信",
2: "支付宝",
3: "抖音",
};
return {
...item,
type: typeMap[item.type] || "未知", // 转换 type 字段
terrace_order_num: item.terrace_order_num + " ",
};
});
// 获取当前日期并格式化
const currentDate = new Date();
const year = currentDate.getFullYear();
const month = String(currentDate.getMonth() + 1).padStart(2, "0"); // 月份从0开始,需要加1
const day = String(currentDate.getDate()).padStart(2, "0");
const formattedDate = `${year}-${month}-${day}`;
// 将日期添加到文件名中
const fileName = `订单列表导出_${formattedDate}`;
// 导出处理后的数据
table2excel(column, processedDatas, fileName);
};
</script>
全部导出:我还获取了当前的日期,导出时是名字+导出的日期。
并且:当列表中的某一行内容只有数字时,导出的表格会默认转成缩写格式。
可以把数据处理一下然后给后面加上一个HTML的空格,也就是&nbsb,这样就不会给数字默认转化为科学计数法。
选中导出:
javascript
// 声明选中的行
const selectedRows = ref([]);
// 处理选中项变化
const handleSelectionChange = (selection) => {
selectedRows.value = selection;
console.log("当前选中的数据:", selectedRows.value); // 打印选中的数据
};
// 导出选中数据的函数
const exportPick = () => {
console.log("导出选中的数据:", selectedRows.value);
if (selectedRows.value.length === 0) {
ElMessage.warning("请选择要导出的数据!");
return;
}
// 获取导出数据
const column = [
{
title: "序号",
key: "id",
type: "text",
},
{
title: "店长名称",
key: "name",
type: "text",
},
{
title: "商家门头照",
key: "img",
type: "image",
width: 150,
height: 100,
},
{
title: "公司名称",
key: "title",
type: "text",
},
{
title: "订单号",
key: "order_num",
type: "text",
},
{
title: "价格",
key: "money",
type: "text",
},
{
title: "类型",
key: "type",
type: "text",
formatter: (row) => {
const typeMap = {
1: "微信",
2: "支付宝",
3: "抖音",
};
return typeMap[row.type] || "未知";
},
},
{
title: "平台订单号",
key: "terrace_order_num",
type: "text",
},
{
title: "创建时间",
key: "create_time",
type: "text",
},
{
title: "下单时间",
key: "pay_time",
type: "text",
},
];
const tableDatas = JSON.parse(JSON.stringify(selectedRows.value)); //这里面填写你接口的数据\
// 处理数据,将 type 字段的值转换为对应的文字
const processedDatas = tableDatas.map((item) => {
const typeMap = {
1: "微信",
2: "支付宝",
3: "抖音",
};
return {
...item,
type: typeMap[item.type] || "未知", // 转换 type 字段
};
});
// 获取当前日期并格式化
const currentDate = new Date();
const year = currentDate.getFullYear();
const month = String(currentDate.getMonth() + 1).padStart(2, "0"); // 月份从0开始,需要加1
const day = String(currentDate.getDate()).padStart(2, "0");
const formattedDate = `${year}-${month}-${day}`;
// 将日期添加到文件名中
const fileName = `订单列表选中导出_${formattedDate}`;
table2excel(column, processedDatas, fileName);
};
// 打开导出确认对话框
const open = () => {
ElMessageBox.confirm("请选择要导出的数据", "导出", {
confirmButtonText: "导出全部",
cancelButtonText: "导出选中",
center: true,
})
.then(() => {
// 点击"导出全部"按钮
exportBtn();
})
.catch(() => {
// 点击"导出选中"按钮
exportPick();
});
};