目录
1、Spring监听器简介
Spring 监听器(Listener)用于监听应用程序中的事件,并在事件发生时执行特定操作。常见的事件包括应用启动、关闭、请求处理等。Spring 提供了多种监听器接口,例如ApplicationListener、ServletRequestListener、HttpSessionListener等,开发者可以通过实现这些接口或者添加对应的注解来监听和处理事件。
2、事件(Event)
在Spring事件顶层类为EventObject抽象类,ApplicationEvent继承了EventObject,是所有事件的基础类,自定义事件需要继承此类。Spring中提供了SpringApplicationEvent,在该类下提供一些实现类。
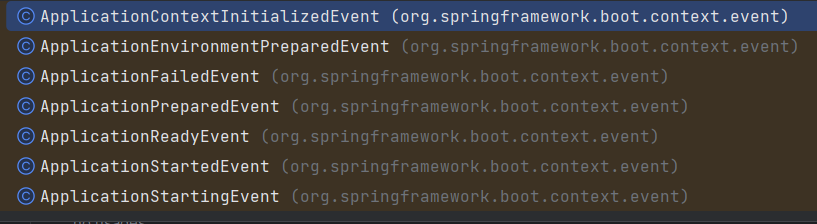
继承这些类的自定义类都可用作为事件类进行发布,事件通常用于在系统中发生某些操作,通知其他的模块进行一些相应的操作,例如系统启动事件、客户注册系统等。
3、监听器(Listener)
Spring中的监听器ApplicationListener,属于函数式接口,实现该接口的类可用监听特点类型的事件,当检查到特点类型事件时,可用自动进行一些用户开发的操作。
java
@FunctionalInterface
public interface ApplicationListener<E extends ApplicationEvent> extends EventListener {
void onApplicationEvent(E event);
default boolean supportsAsyncExecution() {
return true;
}
static <T> ApplicationListener<PayloadApplicationEvent<T>> forPayload(Consumer<T> consumer) {
return (event) -> {
consumer.accept(event.getPayload());
};
}
}
3、事件发布器
Spring中发布事件的接口ApplicationEventPublisher,用于发布事件。ApplicationContext上下文接口继承了该类,可以发布事件。
java
@FunctionalInterface
public interface ApplicationEventPublisher {
default void publishEvent(ApplicationEvent event) {
this.publishEvent((Object)event);
}
void publishEvent(Object event);
}
4、监听器使用
4.1、自定义事件
创建自定义事件类,自定义事件类需要继承ApplicationEvent。
java
package com.gs.listener;
import lombok.AllArgsConstructor;
import lombok.Data;
import org.springframework.context.ApplicationEvent;
public class CustomEvent extends ApplicationEvent {
private String message;
public CustomEvent(Object source, String message) {
super(source);
this.message = message;
}
public String getMessage(){
return this.message;
}
}
4.2、自定义监听器
自定义监听器需要实现ApplicationListener接口。
java
package com.gs.listener;
import org.springframework.context.ApplicationListener;
@Component
public class CustomEvenListener implements ApplicationListener<CustomEvent> {
@Override
public void onApplicationEvent(CustomEvent event) {
System.out.println("接受客户消息" + event.getMessage());
}
}
4.3、发布事件
自定义事件发布器需要实现ApplicationEventPublisher接口
java
package com.gs.listener;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.stereotype.Component;
@Component
public class CustomEventPublisher {
@Autowired
private ApplicationEventPublisher publisher;
public void publishCustomEvent(String message){
System.out.println("发布自定义事件");
publisher.publishEvent(new CustomEvent(this,"这是一个自定义测试事件"));
}
}
4.4、测试
java
package com.gs.listener;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class CustomEventTest implements CommandLineRunner {
@Autowired
private CustomEventPublisher publisher;
@Override
public void run(String... args) throws Exception {
publisher.publishCustomEvent("Hello Spring Event");
}
}
运行结果:

4.5、使用注解方式监听
通过在方法上使用@EventListener注解进行监听
java
package com.gs.listener;
import org.springframework.context.ApplicationListener;
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Component;
@Component
public class CustomEvenListener {
@EventListener
public void handleCustomEvent(CustomEvent event) {
System.out.println("接受客户消息: " + event.getMessage());
}
}
4.6、异步事件处理
默认情况下,Spring事件是同步处理的。如果希望事件处理异步进行,可以使用@Async
注解。例如:
java
package com.gs.listener;
import org.springframework.context.ApplicationListener;
import org.springframework.context.event.EventListener;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Component;
@Component
public class CustomEvenListener {
@Async
@EventListener
public void handleCustomEvent(CustomEvent event) {
System.out.println("接受客户消息: " + event.getMessage());
}
}
启动类开启异步:
java
@EnableAsync
@SpringBootApplication
public class DemoProjectApplication {
public static void main(String[] args) {
SpringApplication.run(DemoProjectApplication.class, args);
}
}
5、总结
Spring的监听器机制提供了一种灵活的方式来处理应用程序中的事件。通过自定义事件和监听器,可以实现模块之间的解耦,提高代码的可维护性和可扩展性。同时,Spring还提供了注解方式和异步处理的支持,使得事件处理更加方便和高效。