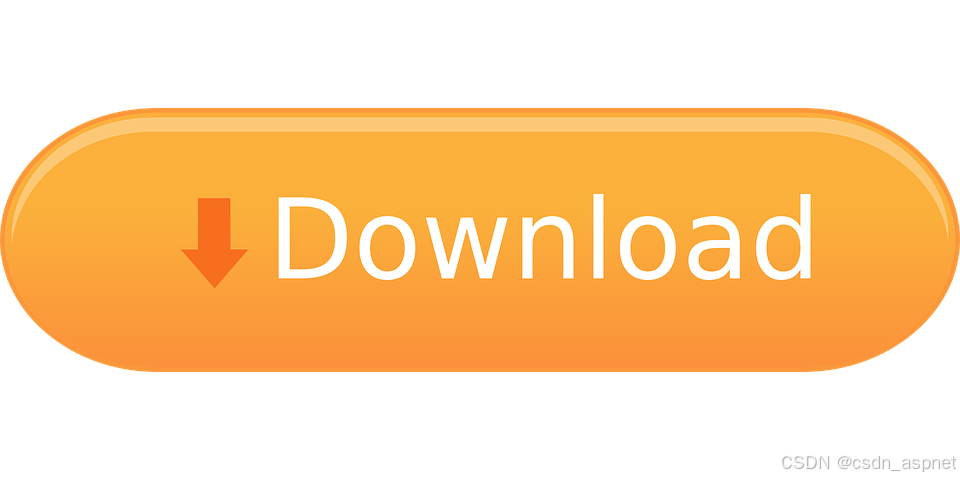
如何从 MVC 控制器(.NET Framework)下载文件。
使用 从 ASP.NET MVC 中的控制器下载任何文件类型FileStreamResult。注意:如果使用ASP.NET Core,请参阅此页面,如果想要将文件上传到服务器,请参阅此页面。
// download a zip file as an attachment
public FileStreamResult DownloadZipFile()
{
string path = Server.MapPath("/files/somefile.zip");
if (System.IO.File.Exists(path))
{
Response.AppendHeader("content-disposition", "attachment;filename=somefile.zip");
System.IO.FileStream stream = new System.IO.FileStream(path, System.IO.FileMode.Open); // don't use using keyword!
return new FileStreamResult(stream, "application/x-zip-compressed"); // the constructor will fire Dispose() when done
}
else
return null;
}
// download a jpeg as an attachment
public FileStreamResult DownloadJpegFile()
{
string path = Server.MapPath("/files/someimage.jpg");
if (System.IO.File.Exists(path))
{
Response.AppendHeader("content-disposition", "attachment;filename=someimage.jpg");
System.IO.FileStream stream = new System.IO.FileStream(path, System.IO.FileMode.Open); // don't use using keyword!
return new FileStreamResult(stream, "image/jpeg"); // the constructor will fire Dispose() when done
}
else
return null;
}
// download a text file as an attachment
public FileStreamResult DownloadTextFile()
{
string path = Server.MapPath("/files/somefile.txt");
if (System.IO.File.Exists(path))
{
Response.AppendHeader("content-disposition", "attachment;filename=somefile.txt");
System.IO.FileStream stream = new System.IO.FileStream(path, System.IO.FileMode.Open); // don't use using keyword!
return new FileStreamResult(stream, "text/plain"); // the constructor will fire Dispose() when done
}
else
return null;
}
FileContentResult
从使用字节数组的 ASP.NET MVC 控制器下载任何数据类型。
public FileContentResult DownloadTextFile()
{
byte[] bytes = System.Text.Encoding.ASCII.GetBytes("How now brown cow.");
Response.AppendHeader("content-disposition", "attachment;filename=sometext.txt");
return new FileContentResult(bytes, "text/plain");
}
或者使用FileStream
来填充字节数组。
public FileContentResult DownloadTextFile()
{
System.IO.FileStream stream = new System.IO.FileStream("somefile.txt", System.IO.FileMode.Open);
byte[] bytes = new byte[stream.Length];
stream.Read(bytes, 0, bytes.Length);
Response.AppendHeader("content-disposition", "attachment;filename=somefile.txt");
return new FileContentResult(bytes, "text/plain");
}
或者简单来说:
public FileContentResult DownloadTextFile()
{
byte[] bytes = System.IO.File.ReadAllBytes("somefile.txt");
Response.AppendHeader("content-disposition", "attachment;filename=somefile.txt");
return new FileContentResult(bytes, "text/plain");
}
下载自MemoryStream
:
public FileContentResult DownloadFromMemoryStream()
{
using(System.IO.MemoryStream ms = new System.IO.MemoryStream())
{
ms.WriteByte((byte)'a');
ms.WriteByte((byte)'b');
ms.WriteByte((byte)'c');
byte[] bytes = ms.ToArray();
Response.AppendHeader("content-disposition", "attachment;filename=somefile.txt");
return new FileContentResult(bytes, "text/plain");
}
}
如果您喜欢此文章,请收藏、点赞、评论,谢谢,祝您快乐每一天。