1.如何创建go语言编辑界面
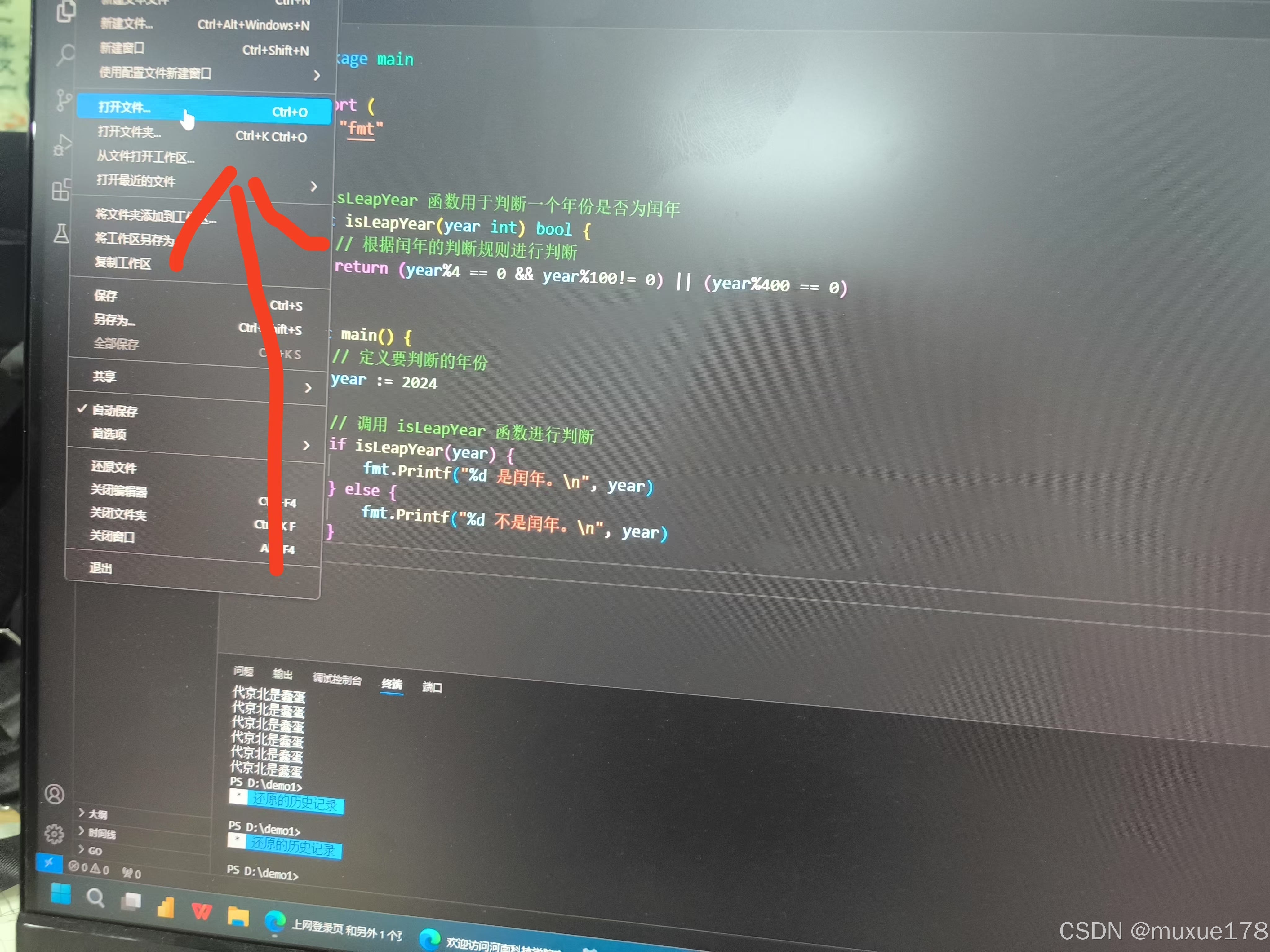
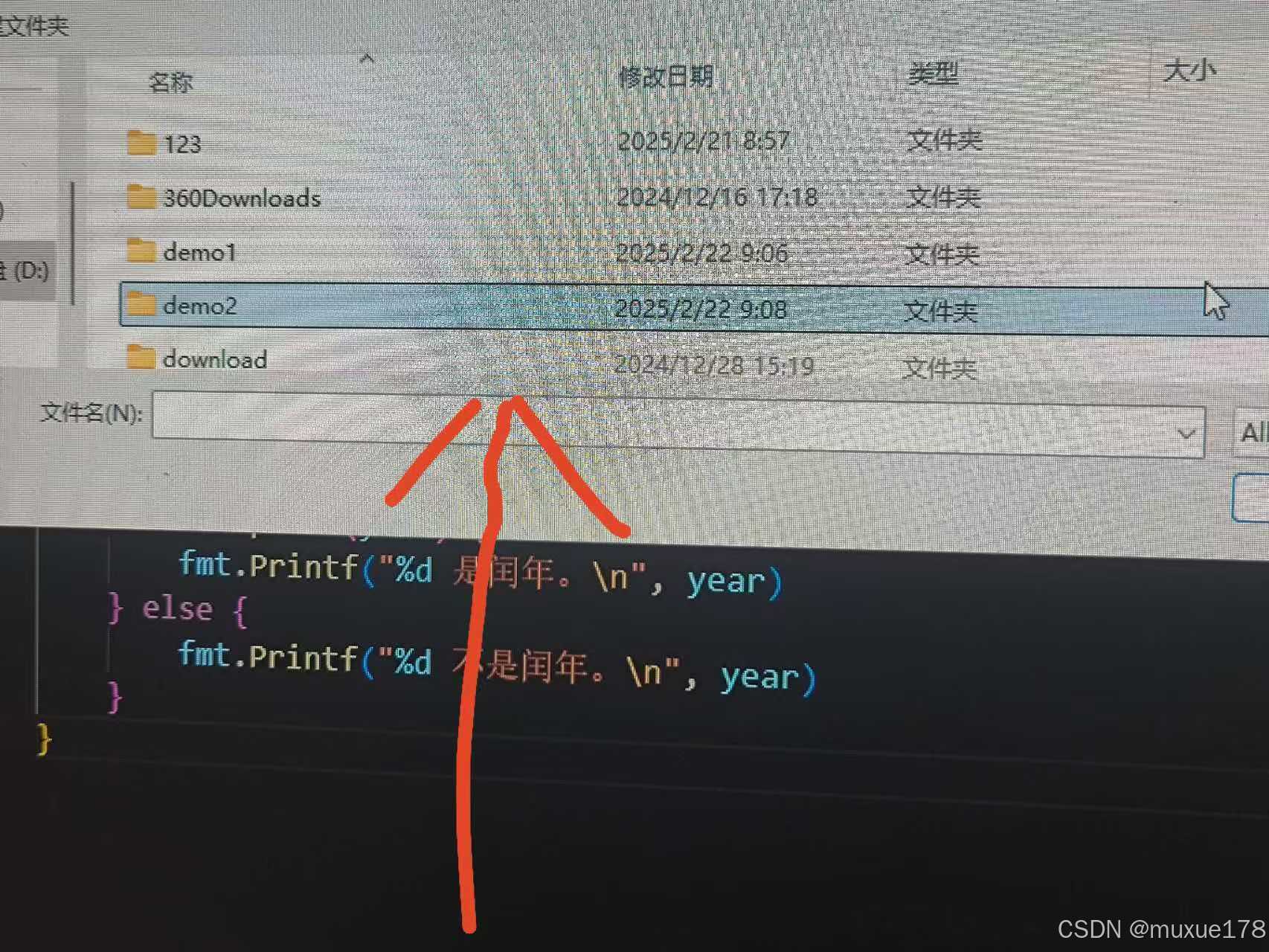
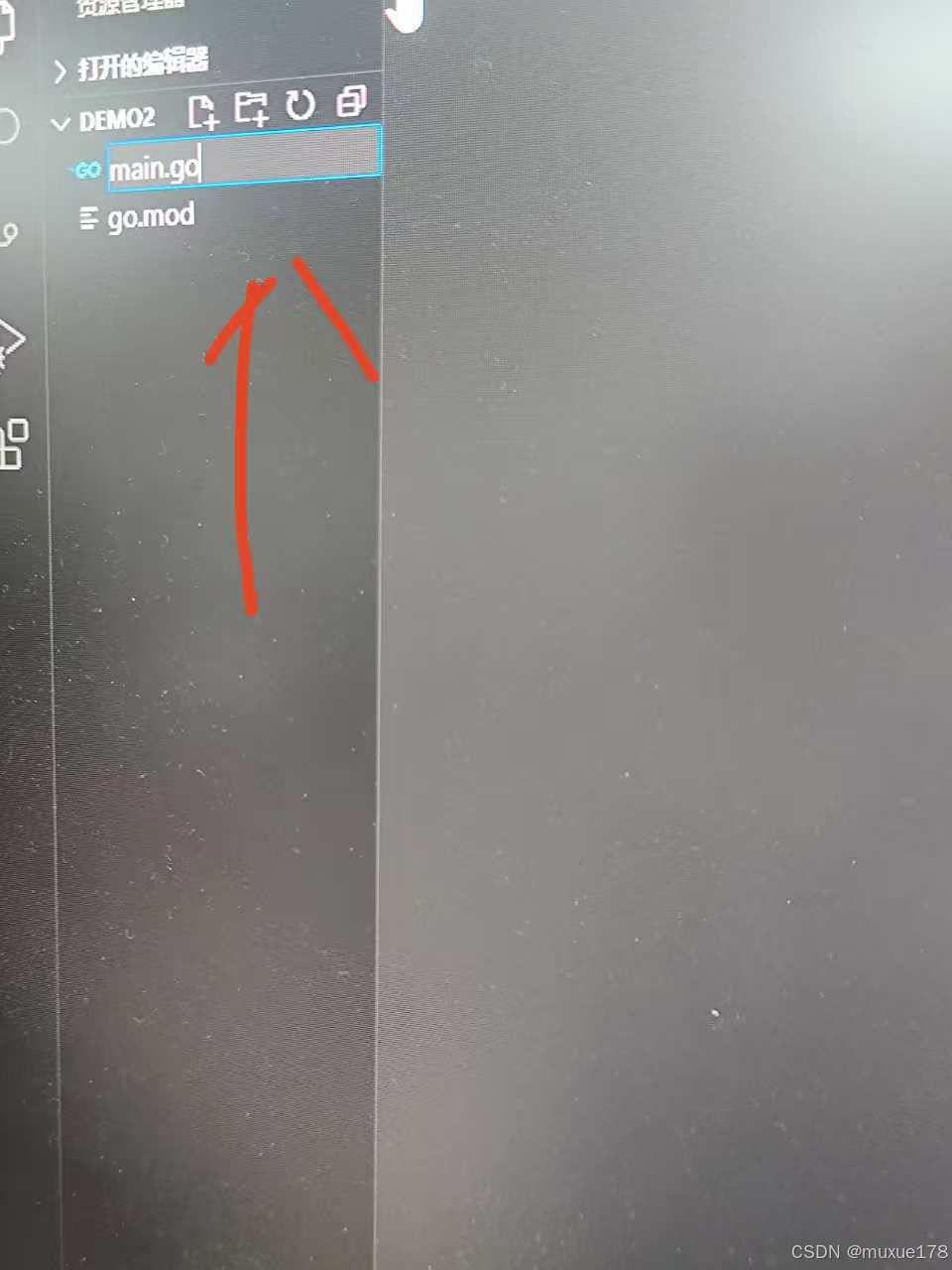
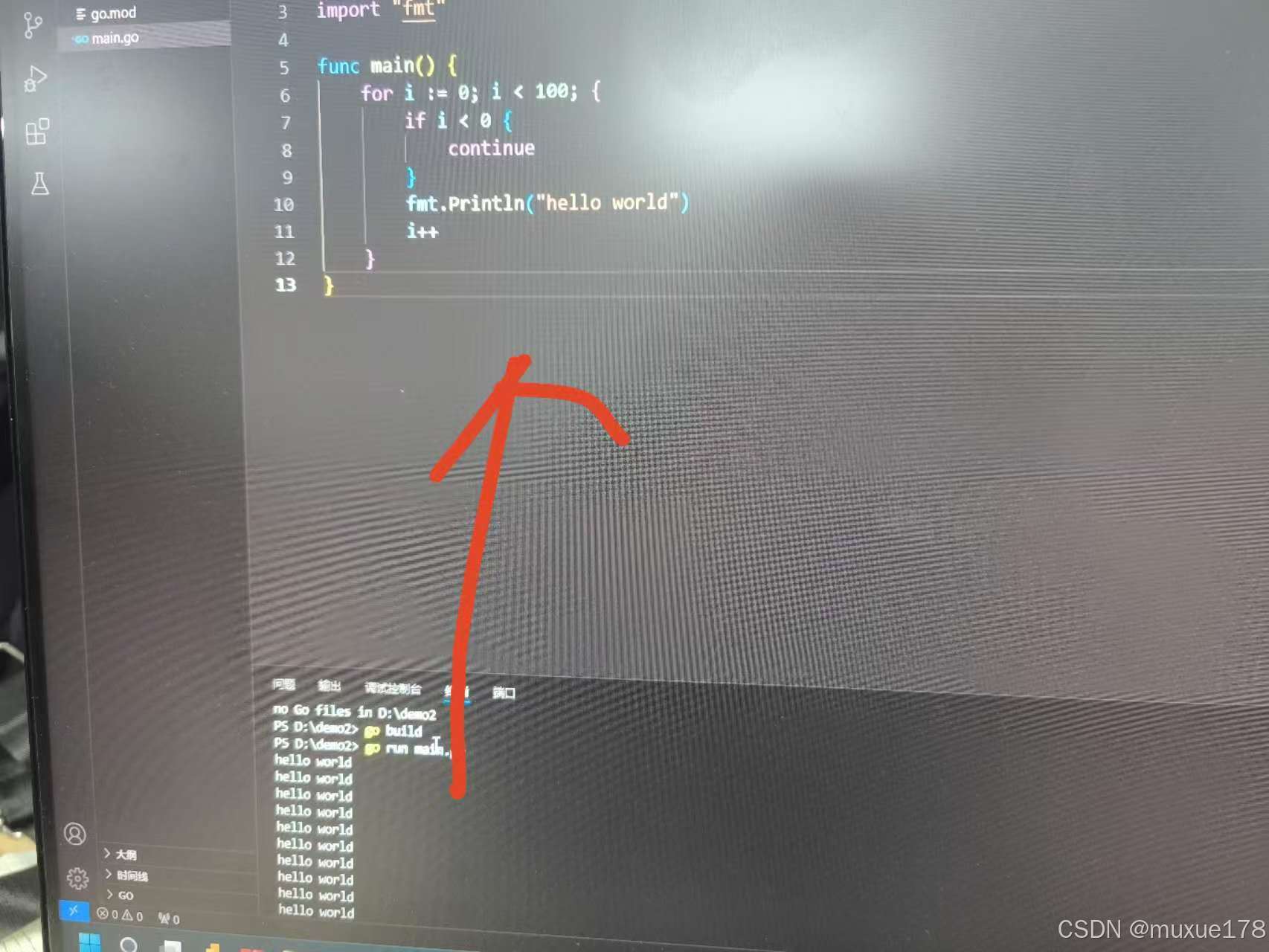
2.案例一实现简单打印"hello worlg":
Go
package main
import "fmt"
func main() {
for i := 0; i < 10; {
if i < 0 {
continue
}
fmt.Println("hello world")
i++
}
}
运行结果:
Go
PS D:\demo2> go mod init DEMO2
go: creating new go.mod: module DEMO2
PS D:\demo2> go build
no Go files in D:\demo2
PS D:\demo2> go build
PS D:\demo2> go run main.go
hello world
hello world
hello world
hello world
hello world
hello world
hello world
hello world
hello world
hello world
3.案例二实现字符转化为二维码并渲染到web页面
Go
package main
import (
"fmt"
"image/png"
"log"
"net/http"
"github.com/skip2/go-qrcode"
)
// 处理二维码生成和页面渲染
func generateQRCodeHandler(w http.ResponseWriter, r *http.Request) {
if r.Method == http.MethodPost {
// 获取表单中输入的文本
text := r.FormValue("text")
if text == "" {
http.Error(w, "请输入要转换为二维码的文本", http.StatusBadRequest)
return
}
// 生成二维码图片
qr, err := qrcode.New(text, qrcode.Medium)
if err!= nil {
http.Error(w, "生成二维码时出错", http.StatusInternalServerError)
return
}
// 设置响应头,指定返回的是 PNG 图片
w.Header().Set("Content-Type", "image/png")
// 将二维码图片编码为 PNG 格式并写入响应
if err := png.Encode(w, qr.Image(256)); err!= nil {
http.Error(w, "写入二维码图片时出错", http.StatusInternalServerError)
return
}
return
}
// 显示 HTML 表单页面
fmt.Fprint(w, `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>生成二维码</title>
</head>
<body>
<h1>请输入要转换为二维码的文本</h1>
<form method="post">
<input type="text" name="text" placeholder="输入文本">
<button type="submit">生成二维码</button>
</form>
</body>
</html>
`)
}
func main() {
// 注册处理函数
http.HandleFunc("/", generateQRCodeHandler)
// 启动服务器,监听 8080 端口
log.Println("Server started at http://localhost:8080")
log.Fatal(http.ListenAndServe(":8080", nil))
}
运行结果:
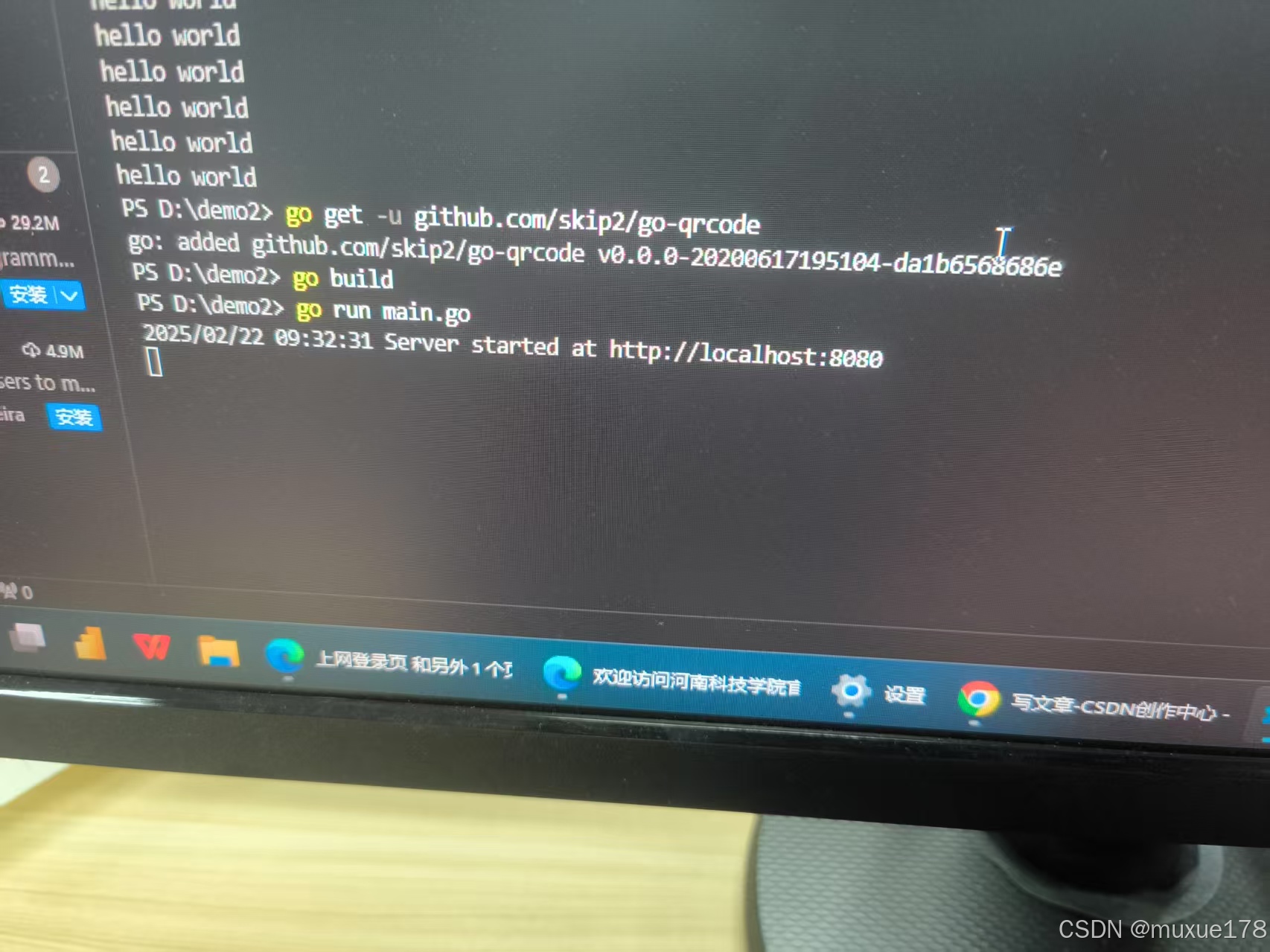
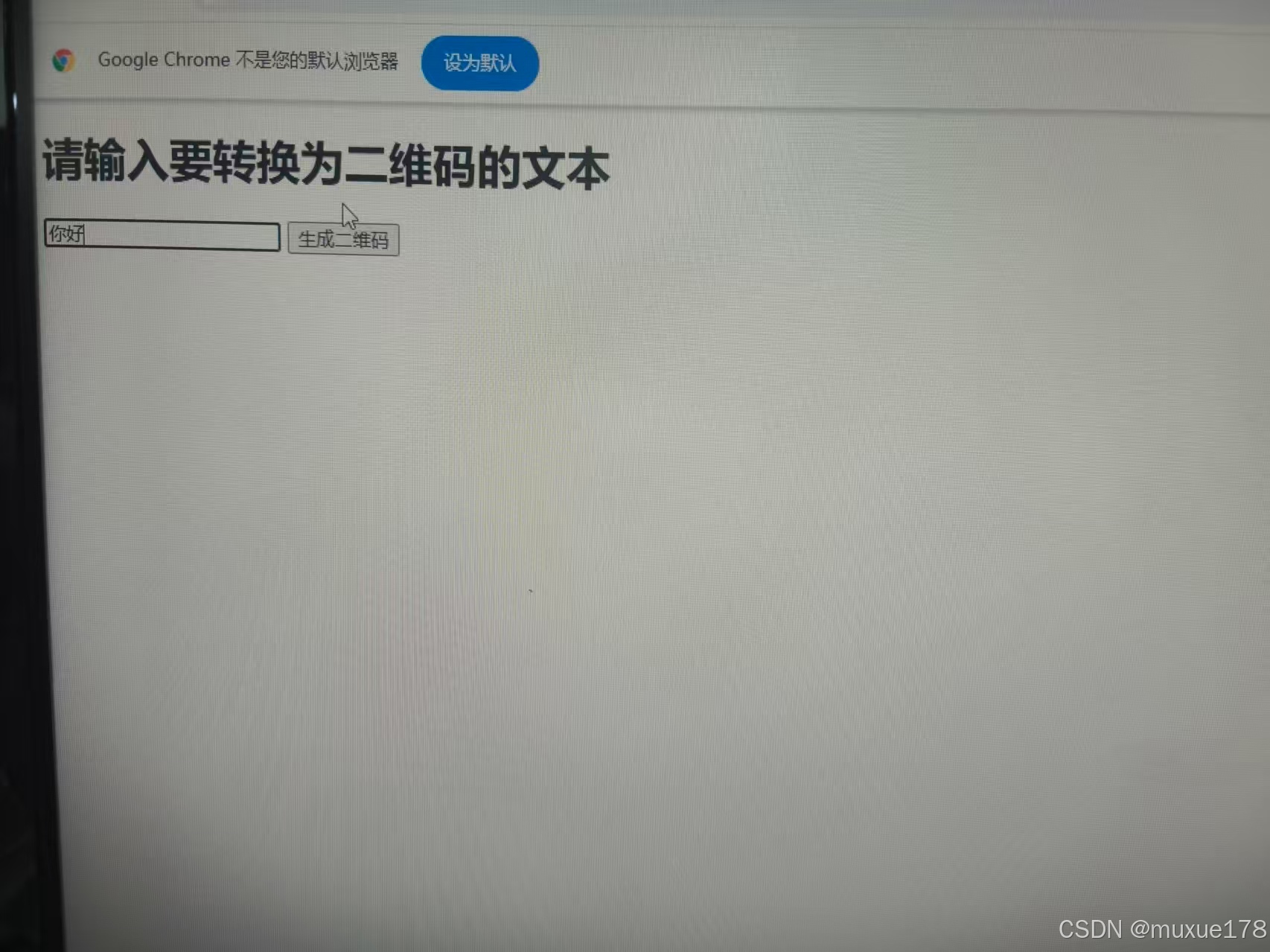
