在 Python 编程学习过程中,turtle
库是一个非常有趣且实用的工具,它可以帮助我们轻松绘制各种图形。结合for
循环、random
模块以及自定义方法等知识点,能够创作出丰富多彩的图案。下面就来分享一下相关的学习笔记。
一、基础知识点回顾
(一)for
循环
for
循环是 Python 中用于遍历可迭代对象(如列表、字符串、范围等)的一种控制结构。其基本语法为:
收起
python
for 变量 in 可迭代对象:
循环体代码
在turtle
绘图中,常利用for
循环来重复执行绘图动作,比如绘制多边形时重复向前移动和旋转的操作。
(二)random
模块
random
模块用于生成随机数。在绘图时,它能为图形添加随机性,比如随机选择颜色。常用的函数有random.choice()
,用于从序列(如列表)中随机选择一个元素。例如:
收起
python
import random
colors = ['red', 'green', 'blue']
random_color = random.choice(colors)
(三)方法与main
入口
自定义方法(函数)可以将重复的代码块封装起来,提高代码的复用性。在 Python 中,定义方法的语法如下:
收起
python
def 方法名(参数列表):
方法体代码
return 返回值(可选)
if __name__ == '__main__':
是 Python 中的一个特殊语句,它确保在当前脚本作为主程序运行时,其下的代码才会被执行。当该脚本被其他模块导入时,这部分代码不会被执行,方便进行模块的测试和封装。
二、使用turtle
库绘制多边形花朵
(一)代码实现
收起
python
import random
import turtle
def draw_flower(count, length):
colors = ['red', 'green', 'blue', 'yellow', 'orange', 'purple']
t = turtle.Turtle()
t.shape("turtle")
for i in range(count):
t.color(random.choice(colors))
t.forward(length)
t.left(360 / count)
turtle.done()
if __name__ == '__main__':
draw_flower(8, 100)
(二)代码解析
- 导入模块 :使用
import random
和import turtle
分别导入random
模块和turtle
库。 - 定义
draw_flower
方法 :该方法接受两个参数count
(表示多边形的边数,即花朵的花瓣数)和length
(表示边长) 。 - 设置画笔属性 :创建
turtle.Turtle()
对象t
,并设置画笔形状为turtle
。 - 绘制花朵 :利用
for
循环,循环次数为count
。在每次循环中,通过random.choice(colors)
随机选择一种颜色,然后让画笔向前移动length
的距离,再向左旋转360/count
度,这样就能绘制出一个由不同颜色多边形组成的花朵形状。 - 主程序入口 :在
if __name__ == '__main__':
代码块中,调用draw_flower(8, 100)
,绘制一个有 8 片花瓣,边长为 100 的花朵。
通过花朵绘制方式的学习,不仅深入了解了turtle
库的绘图功能,还熟练掌握了for
循环、random
模块以及自定义方法的使用。在后续的学习中,可以继续探索turtle
库的其他功能,创作出更复杂、更精美的图形。
python
import random
import turtle
def draw_flower( length):
colors = ['red', 'green', 'blue', 'yellow', 'orange', 'purple']
t = turtle.Turtle()
t.shape("turtle")
#t.color(random.choice(colors))
t.fillcolor("yellow")
t.begin_fill()
t.circle(length)
t.end_fill()
t.penup()
t.goto(-25,70)
t.pendown()
t.fillcolor("black")
t.begin_fill()
t.circle(5)
t.end_fill()
t.penup()
t.goto(25, 70)
t.pendown()
t.fillcolor("black")
t.begin_fill()
t.circle(5)
t.end_fill()
t.penup()
t.goto(-25, 45)
t.rt(90)
t.pendown()
t.width(5)
t.circle(25,160)
turtle.done()
if __name__ == '__main__':
draw_flower( 60)
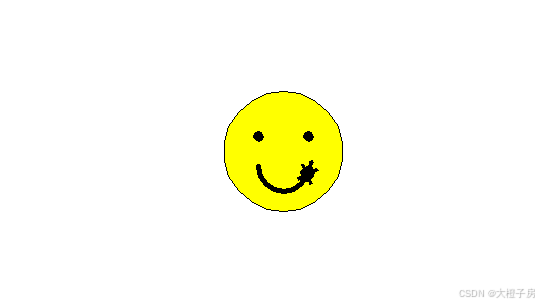
python
import turtle
def draw_flower( length):
t = turtle.Turtle()
t.shape("turtle")
t.penup()
t.goto(0, 63)
t.pendown()
t.left(180)
t.fillcolor("black")
t.begin_fill()
t.circle(length * 2, 180)
t.end_fill()
t.fillcolor("white")
t.begin_fill()
t.circle(length * 2, 180)
t.end_fill()
t.penup()
t.goto(0, 63)
t.pendown()
t.fillcolor("white")
t.begin_fill()
t.circle(length,180)
t.end_fill()
t.penup()
t.goto(0, -63*3)
t.pendown()
t.fillcolor("black")
t.begin_fill()
t.circle(length , 180)
t.end_fill()
t.penup()
t.goto(0, length/3)
t.fillcolor("black")
t.begin_fill()
t.circle(length/3)
t.end_fill()
t.penup()
t.goto(0, (-63*2)+length/3)
t.fillcolor("white")
t.begin_fill()
t.circle(length / 3)
t.end_fill()
t.penup()
turtle.done()
if __name__ == '__main__':
draw_flower( 63)
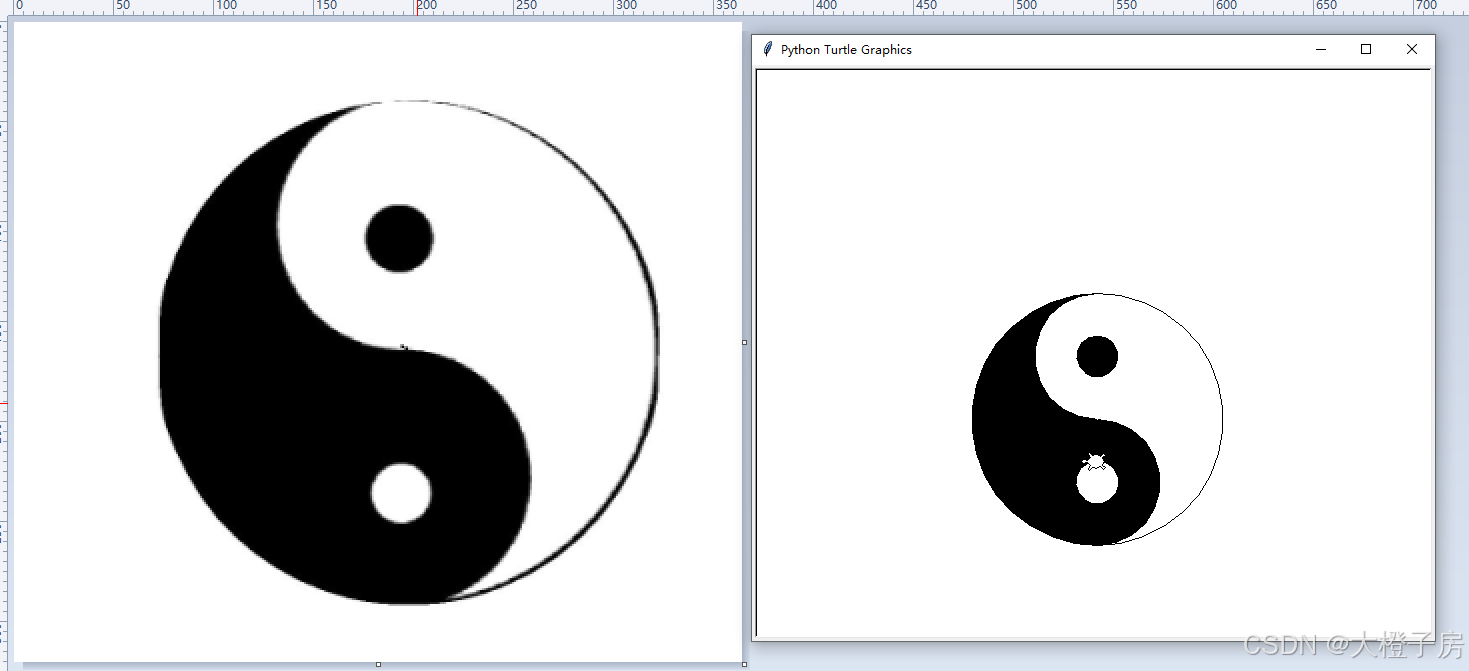