Web3.py 学习笔记 📚
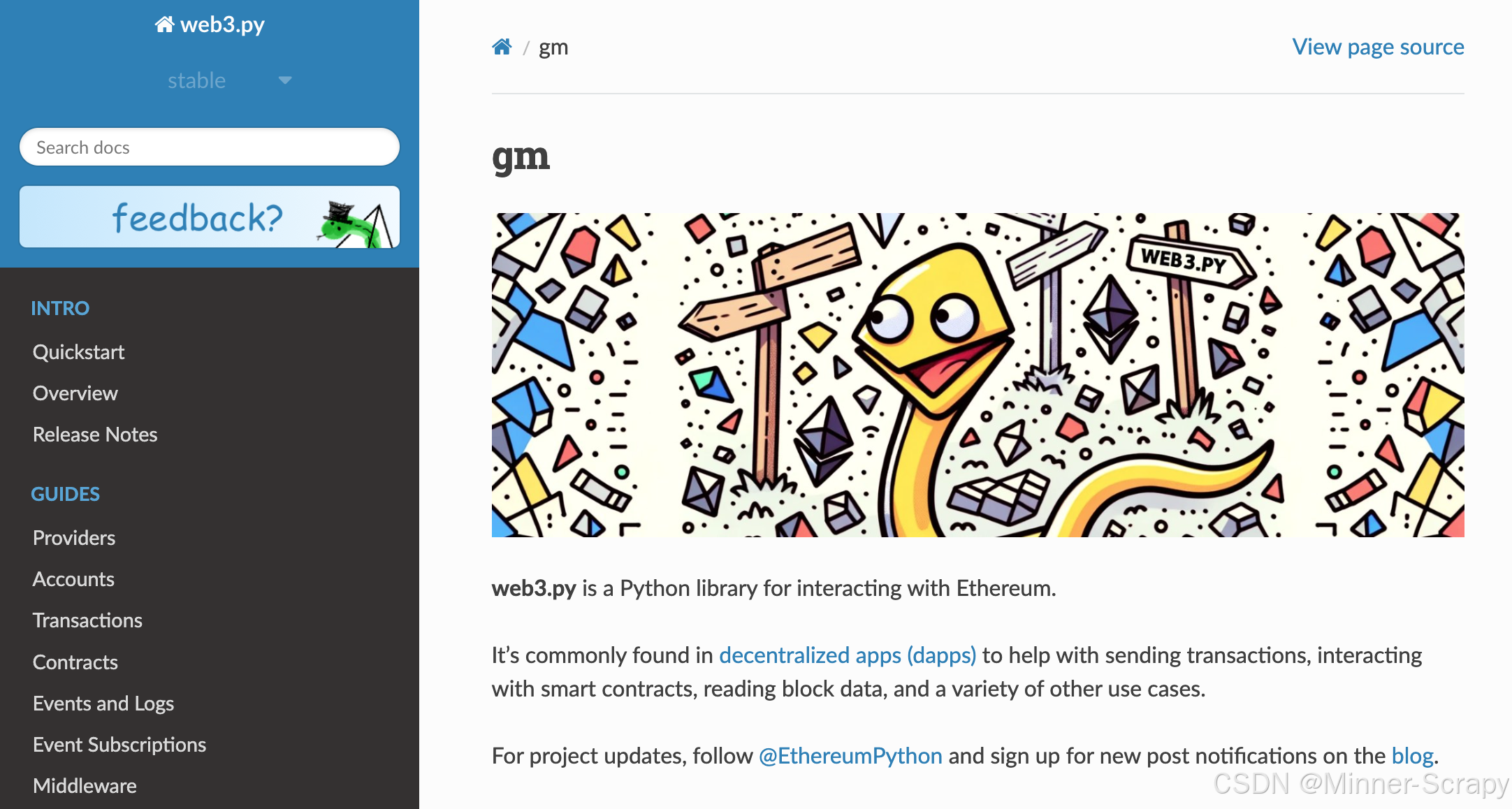
1. Web3.py 简介 🌟
Web3.py 是一个 Python 库,用于与以太坊区块链进行交互。它就像是连接 Python 程序和以太坊网络的桥梁。
1.1 主要功能
- 查询区块链数据(余额、交易等)
- 发送交易
- 与智能合约交互
- 管理以太坊账户
2. 安装和配置 🔧
2.1 安装
bash
# 使用 pip 安装
pip install web3
# 或使用 conda 安装
conda install -c conda-forge web3
2.2 基础配置
python
from web3 import Web3
# 连接到以太坊网络(这里使用 Infura 提供的节点)
w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR-PROJECT-ID'))
# 检查是否连接成功
print(f"是否连接成功: {w3.is_connected()}")
3. 基础操作示例 💡
3.1 查询账户余额
python
def check_eth_balance(address):
# 确保地址格式正确
if not w3.is_address(address):
return "无效的以太坊地址"
# 获取余额(单位:Wei)
balance_wei = w3.eth.get_balance(address)
# 转换为 ETH(1 ETH = 10^18 Wei)
balance_eth = w3.from_wei(balance_wei, 'ether')
return f"地址余额: {balance_eth} ETH"
# 使用示例
address = "0x742d35Cc6634C0532925a3b844Bc454e4438f44e"
print(check_eth_balance(address))
3.2 创建新钱包
python
from eth_account import Account
import secrets
def create_new_wallet():
# 生成随机私钥
private_key = secrets.token_hex(32)
account = Account.from_key(private_key)
return {
"address": account.address,
"private_key": private_key
}
# 使用示例
wallet = create_new_wallet()
print(f"钱包地址: {wallet['address']}")
print(f"私钥: {wallet['private_key']}")
3.3 查询区块信息
python
def get_block_info(block_number='latest'):
# 获取区块信息
block = w3.eth.get_block(block_number)
info = {
"区块号": block.number,
"时间戳": block.timestamp,
"交易数量": len(block.transactions),
"矿工地址": block.miner,
"难度": block.difficulty
}
return info
# 使用示例
print(get_block_info())
4. 实用工具函数 🛠️
4.1 单位转换
python
# Wei 转换为 ETH
wei_amount = 1000000000000000000 # 1 ETH
eth_amount = w3.from_wei(wei_amount, 'ether')
print(f"{wei_amount} Wei = {eth_amount} ETH")
# ETH 转换为 Wei
eth_amount = 2.5
wei_amount = w3.to_wei(eth_amount, 'ether')
print(f"{eth_amount} ETH = {wei_amount} Wei")
4.2 地址验证
python
def is_valid_address(address):
"""验证以太坊地址是否有效"""
return w3.is_address(address)
# 使用示例
address = "0x742d35Cc6634C0532925a3b844Bc454e4438f44e"
print(f"地址是否有效: {is_valid_address(address)}")
5. 监控交易示例 👀
python
def monitor_transactions(address, num_blocks=10):
"""监控指定地址的最近交易"""
current_block = w3.eth.block_number
for block_num in range(current_block - num_blocks, current_block + 1):
block = w3.eth.get_block(block_num, full_transactions=True)
for tx in block.transactions:
if tx['from'].lower() == address.lower() or tx['to'].lower() == address.lower():
print(f"\n发现交易:")
print(f"交易哈希: {tx['hash'].hex()}")
print(f"从: {tx['from']}")
print(f"到: {tx['to']}")
print(f"数量: {w3.from_wei(tx['value'], 'ether')} ETH")
# 使用示例
address = "0x742d35Cc6634C0532925a3b844Bc454e4438f44e"
monitor_transactions(address)
6. 注意事项 ⚠️
-
安全提示:
- 永远不要在代码中直接写入私钥
- 使用环境变量存储敏感信息
- 重要操作先在测试网络验证
-
性能建议:
- 批量查询时注意请求频率
- 适当使用缓存
- 处理好异常情况
-
成本考虑:
- 注意 gas 费用
- 监控网络拥堵情况
- 合理设置 gas 价格
7. 常见问题解答 ❓
-
连接失败:
- 检查网络连接
- 验证 Infura Project ID
- 确认节点服务是否可用
-
交易失败:
- 确保有足够的 ETH 支付 gas
- 检查 nonce 值是否正确
- 验证 gas 价格是否合理
8. 学习资源 📚
-
官方文档:
-
工具网站:
-
测试网络:
- Goerli
- Sepolia
- Mumbai(Polygon 测试网)