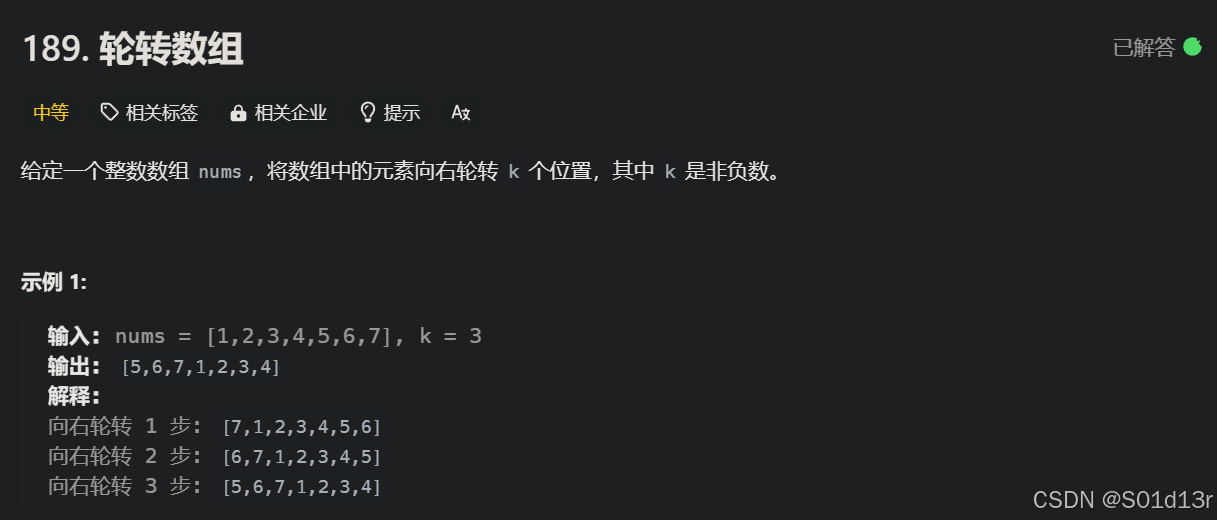
解题思路:
- 三次反转法: 首先反转整个数组,然后反转前 k 个元素,最后反转剩余的 n-k 个元素。
Java代码:
java
class Solution {
public void rotate(int[] nums, int k) {
int n = nums.length;
if (n == 0 || k == 0) return;
k %= n;
reverse(nums, 0, n - 1);
reverse(nums, 0, k - 1);
reverse(nums, k, n - 1);
}
public void reverse(int[] nums, int start, int end) {
while (start < end) {
int temp = nums[start];
nums[start] = nums[end];
nums[end] = temp;
start++;
end--;
}
}
}
复杂度分析:
- 时间复杂度: O(n)。
- 空间复杂度: O(1)。
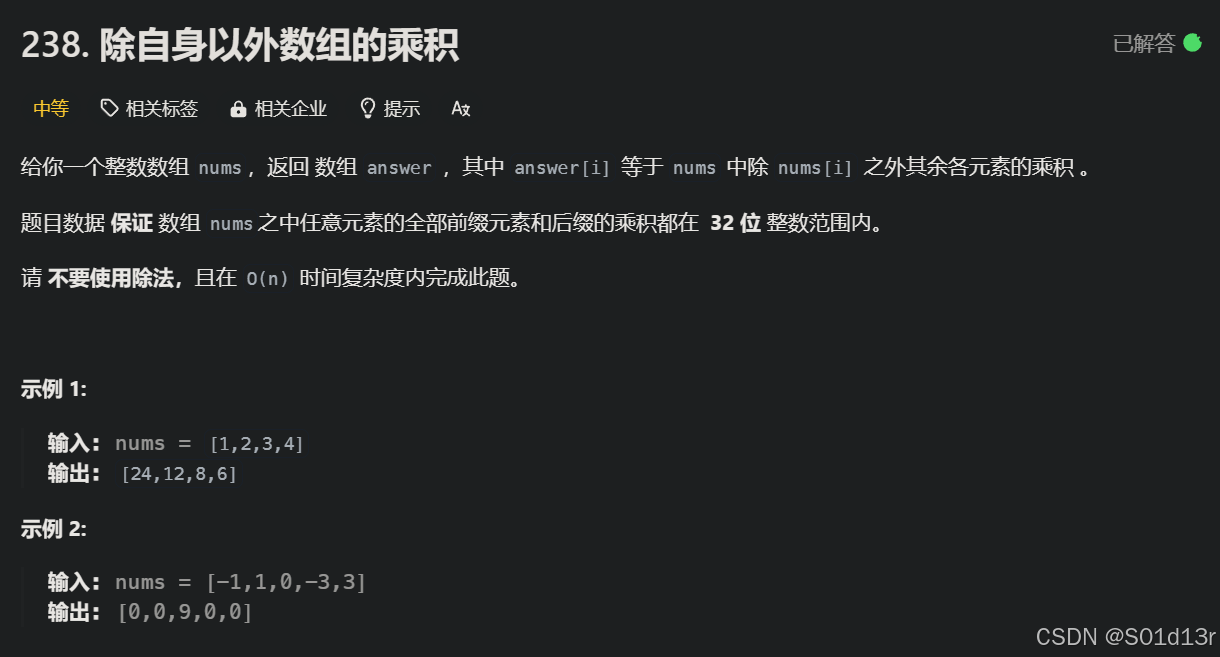
解题思路:
- 计算左侧乘积: 创建一个结果数组 result,遍历原数组 nums,计算每个元素左侧所有元素的乘积,并将其存入 result 中对应的位置。
- 计算右侧乘积并与左侧乘积结合: 从右向左遍历原数组 nums,维护一个变量 rightProduct 表示当前元素右侧所有元素的乘积。将 rightProduct 与 result 中已有的左侧乘积相乘,得到最终结果。
Java代码:
java
class Solution {
public int[] productExceptSelf(int[] nums) {
int n = nums.length;
int[] result = new int[n];
int leftProduct = 1;
for (int i = 0; i < n; i++) {
result[i] = leftProduct;
leftProduct *= nums[i];
}
int rightProduct = 1;
for (int i = n - 1; i >= 0; i--) {
result[i] *= rightProduct;
rightProduct *= nums[i];
}
return result;
}
}
复杂度分析:
- 时间复杂度: 总时间复杂度为 O(n)。
- 空间复杂度: 使用了一个额外的数组 result 来保存结果,空间复杂度为 O(n)。