1、基于.NET8,通过NuGet添加Microsoft.Web.WebView2。
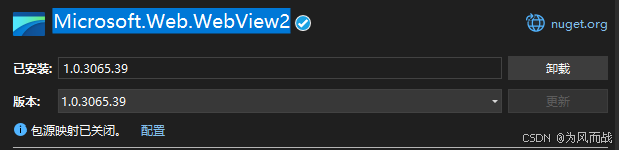
2、MainWindow.xaml代码如下。
XML
<Window x:Class="Demo.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:Pangu"
mc:Ignorable="d"
xmlns:wv2="clr-namespace:Microsoft.Web.WebView2.Wpf;assembly=Microsoft.Web.WebView2.Wpf"
Title="MainWindow" Height="450" Width="800">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="50"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<StackPanel Grid.Row="0" Orientation="Horizontal">
<Button x:Name="btnCallJS" Click="CallJSFunction_Click" Content="C# Call JavaScript" Width="120" Height="30" Margin="10,10,0,10"/>
</StackPanel>
<DockPanel Grid.Row="1">
<wv2:WebView2 Name="webView"/>
</DockPanel>
</Grid>
</Window>
3、MainWindow.xaml.cs代码如下。
cs
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using Microsoft.Web.WebView2.Core;
using System;
using System.IO;
using System.Windows;
using Path = System.IO.Path;
namespace Demo
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
// 页面加载初始化
InitializeAsync();
// 将C#对象注入到WebView2中
InitializeWebViewAsync();
}
private async void InitializeWebViewAsync()
{
await webView.EnsureCoreWebView2Async(null);
webView.CoreWebView2.AddHostObjectToScript("webBrowserObj", new ScriptCallbackObject());
await webView.CoreWebView2.AddScriptToExecuteOnDocumentCreatedAsync("var webBrowserObj = window.chrome.webview.hostObjects.webBrowserObj;");
}
private async void InitializeAsync()
{
await webView.EnsureCoreWebView2Async(null);
// 获取当前应用程序的目录
string appDirectory = AppDomain.CurrentDomain.BaseDirectory+ "Pages\\";
// 构造HTML文件的相对路径(假设HTML文件名为index.html)
string htmlFilePath = Path.Combine(appDirectory, "index.html");
// 将文件路径转换为URI
Uri htmlFileUri = new Uri(htmlFilePath, UriKind.Absolute);
// 设置WebView2的源为HTML文件的URI
webView.Source = htmlFileUri;
}
private async void CallJSFunction_Click(object sender, RoutedEventArgs e)
{
// 假设你要调用的JavaScript函数名为 ShowAlert,它不接受任何参数
string msg = "Hello from C#!";
string script = $"ShowAlert('{ msg }');";
await webView.CoreWebView2.ExecuteScriptAsync(script);
}
}
}
4、 ScriptCallbackObject.cs代码如下。
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
namespace Demo
{
/// <summary>
/// JavaScript调用C#函数类
/// </summary>
[ClassInterface(ClassInterfaceType.AutoDual)]
[ComVisible(true)]
public class ScriptCallbackObject
{
public void ShowMessage(string message)
{
MessageBox.Show(message);
}
}
}
5、index.html页面代码如下。
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>WebView2 Index</title>
<script>
document.addEventListener("DOMContentLoaded", function() {
var button = document.getElementById("btnBroadCast");
button.addEventListener("click", function() {
webBrowserObj.ShowMessage("Hello from JavaScript!");
});
});
function ShowAlert(message) {
document.getElementById("title").innerHTML = message;
}
function showAlert(message) {
document.getElementById("title").innerHTML = message;
}
</script>
</head>
<body>
<button id="StartBroadCast">开始广播</button>
<!-- <button id="btnBroadCCast" hidden="true">开始测试</button> -->
<button id="btnBroadCast">开始测试</button>
<h1 id="title"></h1>
</body>
</html>
6、运行效果如下。
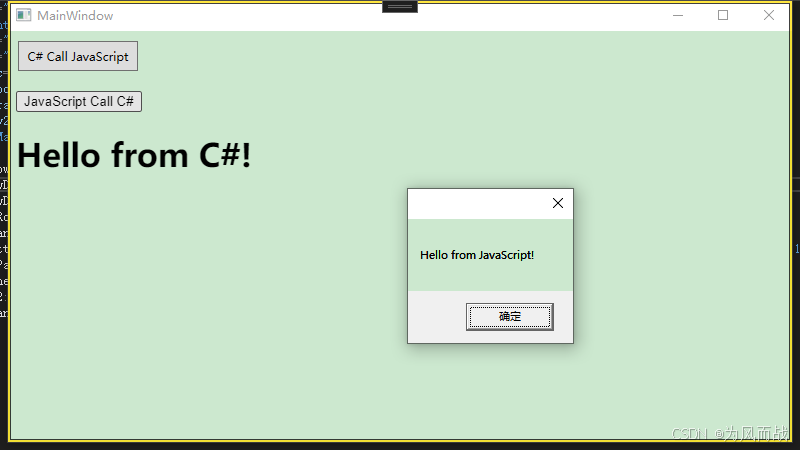