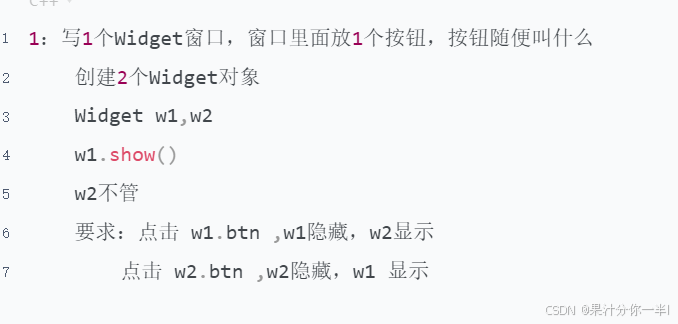
#include <QApplication>
#include <QDebug>
#include <QWidget>
#include <QTextEdit>
#include <QLineEdit>
#include <QPushButton>
#include <QBoxLayout>
#include <QLabel>
class Widget:public QWidget{
QPushButton* but;
Widget* other;
public:
Widget();
void setother(Widget& w);
void buttext(const char* text);
void click();
};
Widget::Widget() : other(NULL)
{
but = new QPushButton(this);
QVBoxLayout lay;
lay.addWidget(this);
QObject::connect(but, &QPushButton::clicked, this, &Widget::click);
}
void Widget::setother(Widget& w)
{
other=&w;
}
void Widget::buttext(const char* text)
{
but->setText(text);
}
void Widget::click()
{
this->hide();
if (other) {
other->show();
}
}
int main(int argc,char** argv)
{
QApplication app(argc,argv);
Widget w1,w2;
w1.buttext("w1.but");
w2.buttext("w2.but");
w1.setother(w2);
w2.setother(w1);
w1.show();
return app.exec();
}
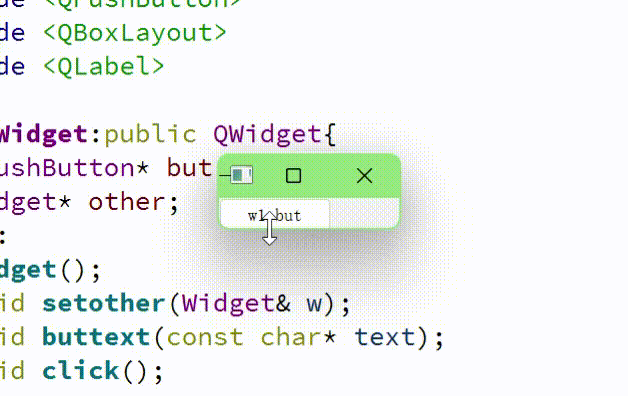