基于手势识别的音量控制系统
项目效果
这是一个结合了计算机视觉和系统控制的实用项目,通过识别手势来实现音量的无接触控制,同时考虑到了用户隐私,加入了实时人脸遮罩功能。
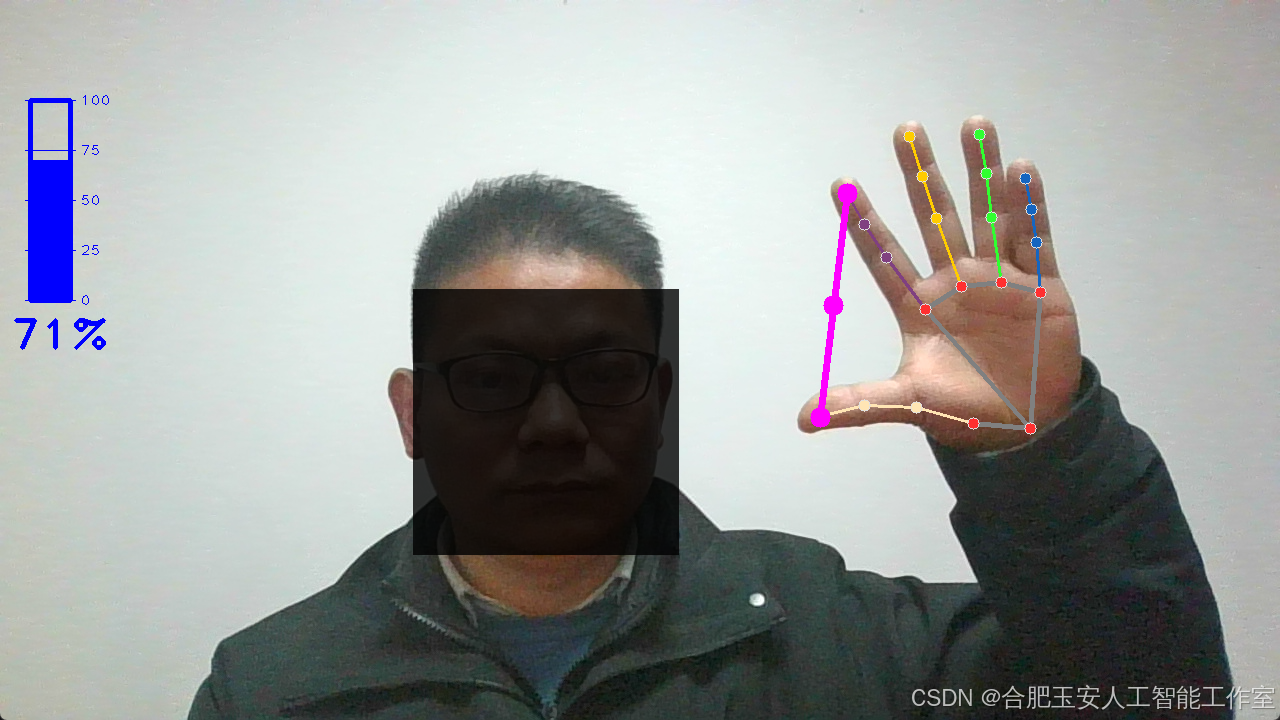
核心功能实现
1. 手势识别与音量映射
系统使用 MediaPipe 框架进行手部关键点检测,通过计算大拇指和食指之间的距离来控制音量:
python
def _process_landmarks(self, hand_landmarks):
# 获取手指关键点
thumb = hand_landmarks.landmark[4] # 大拇指指尖
index = hand_landmarks.landmark[8] # 食指指尖
# 计算手指间距离
distance = math.hypot(
(thumb.x - index.x) * self.width,
(thumb.y - index.y) * self.height
)
# 将距离映射到音量范围
volume = np.interp(distance, [30, 200], [self.min_volume, self.max_volume])
return volume, distance
2. 音量平滑控制
为了避免音量突变,实现了平滑过渡机制:
python
def _smooth_volume(self, current_volume):
# 使用移动平均实现平滑过渡
self.volume_history.append(current_volume)
if len(self.volume_history) > self.smooth_factor:
self.volume_history.pop(0)
return sum(self.volume_history) / len(self.volume_history)
3. 人脸隐私保护
使用 MediaPipe 的人脸检测功能,实时为人脸添加遮罩:
python
def _apply_face_mask(self, image, face_landmarks):
mask = image.copy()
# 创建人脸轮廓
face_points = np.array([
[landmark.x * self.width, landmark.y * self.height]
for landmark in face_landmarks.landmark
], dtype=np.int32)
# 绘制遮罩
cv2.fillPoly(mask, [face_points], self.face_mask_color)
return cv2.addWeighted(image, 1 - self.face_mask_alpha,
mask, self.face_mask_alpha, 0)
4. 视觉反馈系统
实现了直观的音量显示界面:
python
def _draw_volume_bar(self, image, volume_percentage):
# 绘制音量条背景
cv2.rectangle(image, (50, 150), (85, 400), (255, 0, 0), 3)
# 计算当前音量高度
bar_height = int(250 * (volume_percentage / 100))
cv2.rectangle(image, (50, 400 - bar_height),
(85, 400), (255, 0, 0), cv2.FILLED)
# 显示音量百分比
cv2.putText(image, f'{int(volume_percentage)}%',
(40, 450), cv2.FONT_HERSHEY_PLAIN,
3, (255, 0, 0), 3)
技术要点
1. 实时性能优化
- 使用 MediaPipe 的高效手势识别模型
- 优化图像处理流程,减少不必要的计算
- 实现帧率监控,保证流畅体验
2. 交互设计
- 直观的手势映射:手指距离与音量大小成正比
- 实时视觉反馈:音量条显示和百分比指示
- 平滑过渡:避免音量突变带来的不适感
3. 隐私保护
- 实时人脸检测和遮罩
- 可配置的遮罩样式
- 无需额外设置的自动保护机制
核心代码解析
1. 初始化配置
python
def __init__(self):
self.mp_hands = mp.solutions.hands
self.mp_face = mp.solutions.face_mesh
self.hands = self.mp_hands.Hands(
min_detection_confidence=0.7,
min_tracking_confidence=0.5
)
self.face_mesh = self.mp_face.FaceMesh(
max_num_faces=1,
min_detection_confidence=0.5,
min_tracking_confidence=0.5
)
2. 音量控制逻辑
python
def _update_volume(self, volume):
# 获取系统音频接口
devices = AudioUtilities.GetSpeakers()
interface = devices.Activate(IAudioEndpointVolume._iid_,
CLSCTX_ALL, None)
volume_control = cast(interface, POINTER(IAudioEndpointVolume))
# 设置新音量
volume_control.SetMasterVolumeLevelScalar(volume, None)
3. 主循环处理
python
def process_frame(self, frame):
# 图像预处理
frame_rgb = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 手势识别
hand_results = self.hands.process(frame_rgb)
if hand_results.multi_hand_landmarks:
volume, distance = self._process_landmarks(
hand_results.multi_hand_landmarks[0]
)
smooth_volume = self._smooth_volume(volume)
self._update_volume(smooth_volume)
# 人脸检测和遮罩
face_results = self.face_mesh.process(frame_rgb)
if face_results.multi_face_landmarks:
frame = self._apply_face_mask(
frame, face_results.multi_face_landmarks[0]
)
return frame
使用效果
系统运行时,用户可以通过自然的手势来控制系统音量,无需接触任何物理设备。同时,实时的视觉反馈让用户能够精确地控制音量大小。人脸遮罩功能在保护用户隐私的同时,不会影响系统的正常使用。