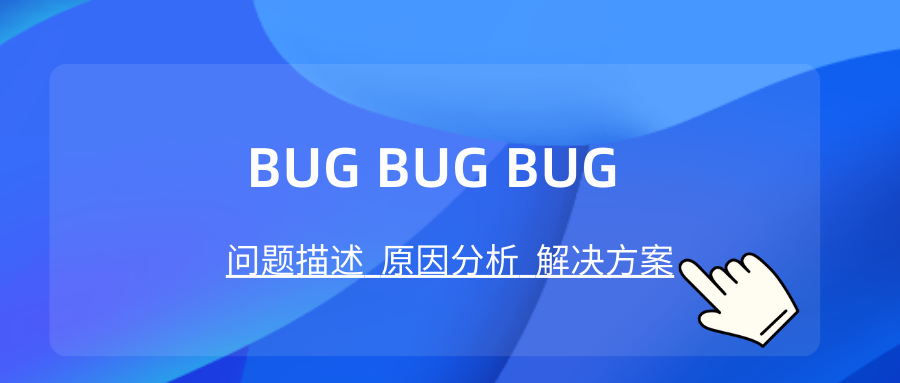
🤍 前端开发工程师、技术日更博主、已过CET6
🍨 阿珊和她的猫_CSDN博客专家、23年度博客之星前端领域TOP1
🕠 牛客 高级专题作者、打造专栏《前端面试必备》 、《2024面试高频手撕题》、《前端求职突破计划》
🍚 蓝桥云课 签约作者、上架课程《Vue.js 和 Egg.js 开发企业级健康管理项目》、《带你从入门到实战全面掌握 uni-app》
文章目录
问题描述
在Vue.js应用中,开发者经常会遇到 [Vue warn]: Duplicate keys detected: 'xxx'. This may cause an update error.
的错误提示。该错误表明在组件渲染过程中,Vue.js检测到重复的键值,这可能会导致渲染错误。
原因分析
- 使用对象作为键值 :在
v-for
循环中,如果使用对象作为键值,而这些对象在数据中重复,就会导致这个错误。因为JavaScript中对象引用的性质,相同的对象引用可能被视为不同的键,从而导致重复键警告。 - 数组重复值:即使是在数组中,如果键值是数组元素的重复值,也会导致这个错误。
- 同一层DOM节点的重复键 :在同一层DOM节点上使用
v-for
循环时,如果键值重复,会导致该错误。
解决方案
1. 使用唯一标识符
确保在 v-for
循环中为每个元素分配一个唯一的键值。可以使用数组索引或元素的唯一标识符(如ID)。
vue
<template>
<ul>
<li v-for="(item, index) in items" :key="index">
{{ item.message }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: [
{ message: 'Item 1' },
{ message: 'Item 2' },
{ message: 'Item 3' }
]
};
}
};
</script>
2. 使用唯一ID
如果元素有唯一的ID,应使用该ID作为键值。
vue
<template>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.message }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, message: 'Item 1' },
{ id: 2, message: 'Item 2' },
{ id: 3, message: 'Item 3' }
]
};
}
};
</script>
3. 避免使用对象作为键值
尽量不要在 v-for
循环中使用对象作为键值,改用索引或其他唯一标识符。
vue
<template>
<ul>
<li v-for="(item, index) in items" :key="index">
{{ item.message }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
items: [
{ message: 'Item 1' },
{ message: 'Item 2' },
{ message: 'Item 3' }
]
};
}
};
</script>
4. 使用 .sync
修饰符
在父组件中使用 .sync
修饰符可以简化子组件通知父组件修改数据的过程,从而避免一些因键值重复引起的更新错误。
vue
<!-- 子组件 -->
<template>
<div>
<input :value="localValue" @input="updateValue" />
</div>
</template>
<script>
export default {
props: ['value'],
data() {
return {
localValue: this.value
};
},
methods: {
updateValue(event) {
this.localValue = event.target.value;
this.$emit('update:value', this.localValue);
}
}
};
</script>
vue
<!-- 父组件 -->
<template>
<div>
<child-component :value.sync="parentValue"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentValue: 'Initial Value'
};
}
};
</script>
总结
[Vue warn]: Duplicate keys detected: 'xxx'. This may cause an update error.
错误通常是由于在 v-for
循环中使用了重复的键值引起的。通过使用唯一标识符(如数组索引或元素的唯一ID)、避免使用对象作为键值、以及使用 .sync
修饰符等方法,可以有效解决这个问题。
通过这些方法,开发者可以提高代码的健壮性,减少运行时错误,提升应用的稳定性和用户体验。建议开发者在编写 v-for
循环时,特别注意确保键值的唯一性,从而避免潜在的渲染错误。