题目描述
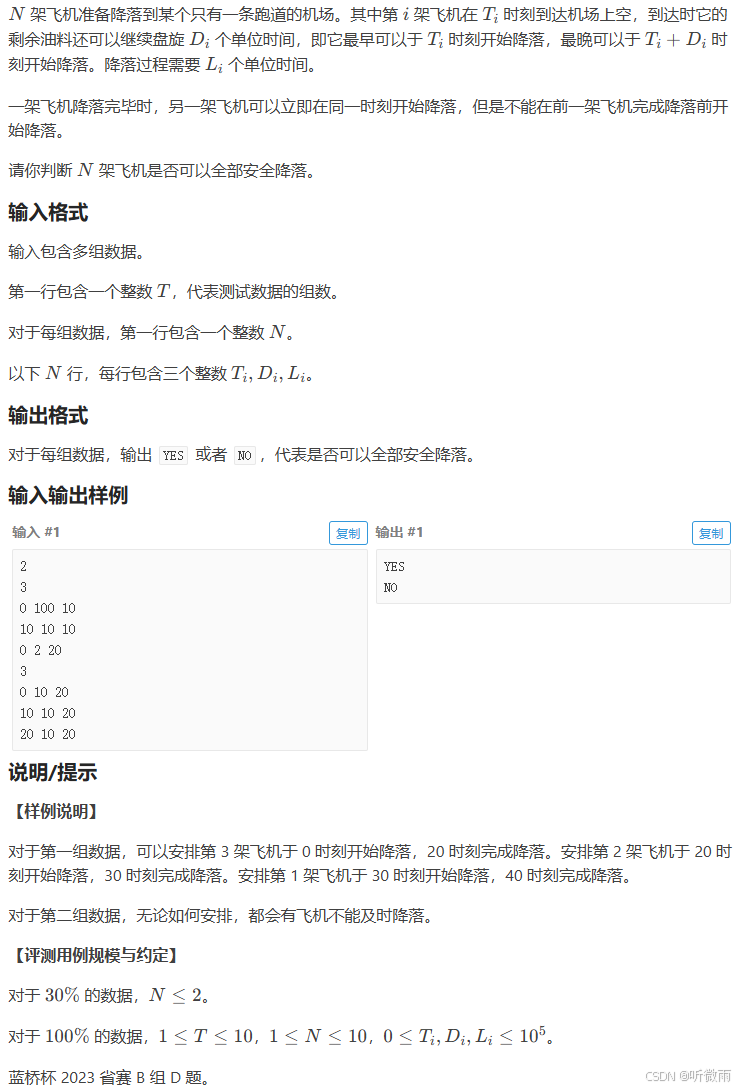
题解
这道题数据范围很小,所以可以用搜索做。具体题解看下方代码。
代码
带注释版代码
c
#include<iostream>
#include<cstring>
using namespace std;
const int N=10;
struct Plane{ // 存每个飞机的t,d,l
int t;
int d;
int l;
}planes[N];
int n;
bool st[N]; // 用来判断该飞机有没有降落
bool dfs(int u,int last){ // u代表当前降落飞机数,last代表当前的时间
if(u==n){ // 如果降落了n个飞机,说明所有飞机安全降落
return true;
}
// dfs有n条分支(当前可以选择n个飞机进行降落)
for(int i=0;i<n;i++){
// 如果!st[i]代表飞机没有降落,
// 并且飞机达到的时间和盘旋的时间大于当前的时间,就说明这个飞机是安全的(没有到达或者正在盘旋中)
if(!st[i]&&planes[i].t+planes[i].d>=last){
st[i]=true; // 飞机降落
// 下一个飞机进行降落,并且当前时间要进行更新。
// last更新是,如果last大于飞机到达时间的话,则该飞机在last时刻降落,并耗时l时,last更新为last+l
// 如果last小于飞机到达时间的话,那么需要等飞机到达后再降落,所有last更新为t+l时
// 如果所有飞机都安全降落了,即u==n,return true,那么dfs回溯时,下方条件判断为true,也return true,终止后续递归
if(dfs(u+1,max(last,planes[i].t)+planes[i].l))return true;
// 回溯要把修改复原
st[i]=false;
}
}
// 如果当前找不到降落的飞机,返回false
return false;
}
int main(){
int T;
cin>>T;
for(int i=0;i<T;i++){
cin>>n;
for(int j=0;j<n;j++){
int t,d,l;
cin>>t>>d>>l;
planes[j]={t,d,l};
}
cout<<(dfs(0,0)?"YES":"NO")<<endl;
// 因为上面的dfs满足u==n,会直接return true,并层层回溯返回true,
//这个时候不会将修改复原(st[i]=false),所以要把可能存在的st[i]==true的情况消灭,重新初始化一下st数组
memset(st,0,sizeof st);
}
}
不带注释版代码
c
#include<iostream>
#include<cstring>
using namespace std;
const int N=10;
struct Plane{
int t;
int d;
int l;
}planes[N];
int n;
bool st[N];
bool dfs(int u,int last){
if(u==n){
return true;
}
for(int i=0;i<n;i++){
if(!st[i]&&planes[i].t+planes[i].d>=last){
st[i]=true;
if(dfs(u+1,max(last,planes[i].t)+planes[i].l))return true;
st[i]=false;
}
}
return false;
}
int main(){
int T;
cin>>T;
for(int i=0;i<T;i++){
cin>>n;
for(int j=0;j<n;j++){
int t,d,l;
cin>>t>>d>>l;
planes[j]={t,d,l};
}
cout<<(dfs(0,0)?"YES":"NO")<<endl;
memset(st,0,sizeof st);
}
}