导入hutool依赖
<!--hutool-->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.36</version>
获取验证码接口
java
@Autowired
private Captcha captcha;
private final static Long VALIDATE_CODE = 60 * 1000L;
@RequestMapping("/getCaptcha")
public void getCaptcha(HttpServletResponse response, HttpSession session) throws IOException {
// 设置响应内容类型
response.setContentType("image/png");
// 直接把验证码写入浏览器,没有返回值
LineCaptcha lineCaptcha = CaptchaUtil.createLineCaptcha(captcha.getWidth(), captcha.getHeight(),4,4);
String code = lineCaptcha.getCode();
session.setAttribute(captcha.getSession().getKey(), code);
session.setAttribute(captcha.getSession().getDate(),new Date());// 获取当前时间
lineCaptcha.write(response.getOutputStream());
}
检查验证码接口
java
@RequestMapping("/check")
public boolean check(String mycaptcha, HttpSession session) {
System.out.println("[captchaCheck]用户输入的验证码:" + mycaptcha);
String code = (String)session.getAttribute(captcha.getSession().getKey());
if(!StringUtils.hasLength(code)) {
return false;
}
Date date = (Date)session.getAttribute(captcha.getSession().getDate());
if(mycaptcha.equalsIgnoreCase(code) && VALIDATE_CODE >= System.currentTimeMillis() - date.getTime()) {
return true;
}
return false;
}
前后端交互
html
<img id="captcha_img" src="/captcha/getCaptcha" alt="看不清?换一张" onclick="change()">
js
let check = false;
function change() {
event.preventDefault(); // 阻止表单默认提交行为
$.ajax({
type: "get",
url: "/captcha/getCaptcha",
success: function (result) {
console.log("[getCaptcha]刷新的验证码:" + result);
$("#captcha_img").attr("src", "/captcha/getCaptcha");
}
})
}
function login() {
event.preventDefault(); // 阻止表单默认提交行为
$.ajax({
type: "get",
url: "/login",
data: {
username: $("#username").val(),
password: $("#password").val(),
},
success: function (result) {
console.log(result);
if(result === false) {
alert("用户名或密码错误!!");
}
else if(result === true && check === false) {
alert("验证码错误!!");
location.reload();
}
else if(result === true && check === true) {
location.href = "recognize.html";
}
// if(result === true && check === true) {
// location.href = "recognize.html";
// }
// else {
// alert("用户名或密码错误!!");
// }
}
})
$.ajax({
type: "post",
url: "/captcha/check",
data: {
mycaptcha: $("#captcha").val()
},
success: function (result) {
console.log(result);
if(result === false) {
check = false;
// alert("验证码错误!!");
// location.reload();
}
else {
check = true;
}
}
})
}
测试
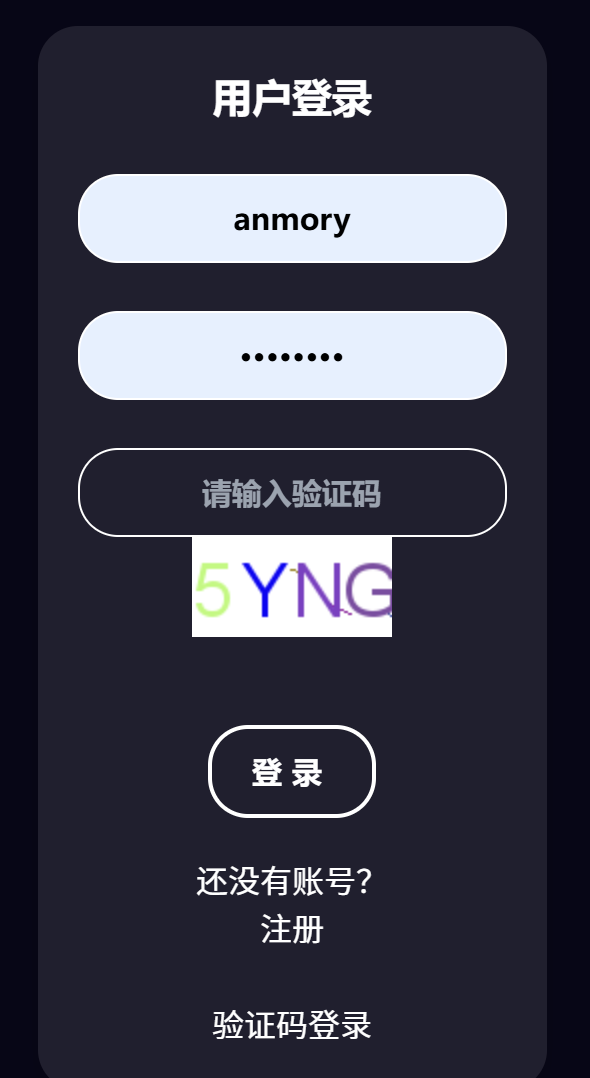