预处理工具、斑点工具、卡尺工具
1.进行膨胀处理
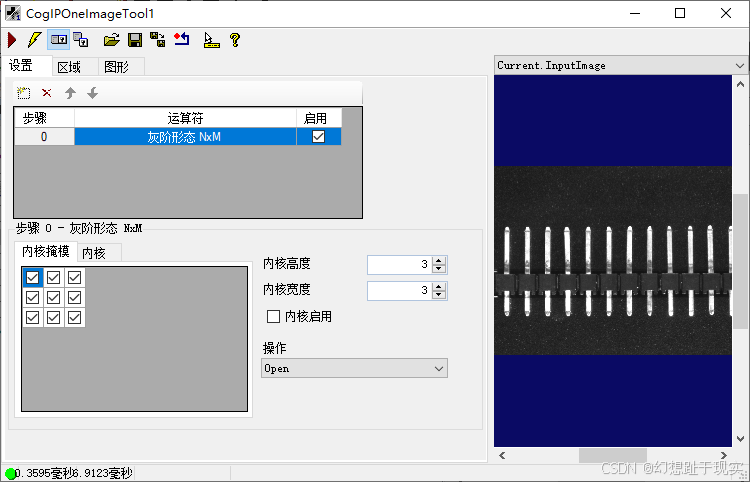
2.进行斑点处理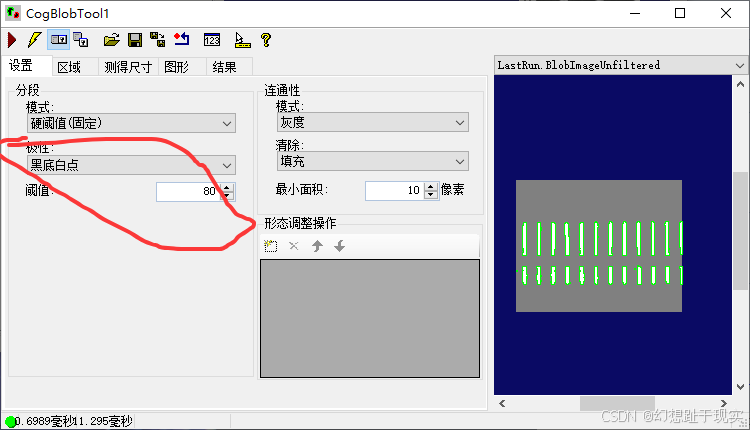
3. 过滤到小面积
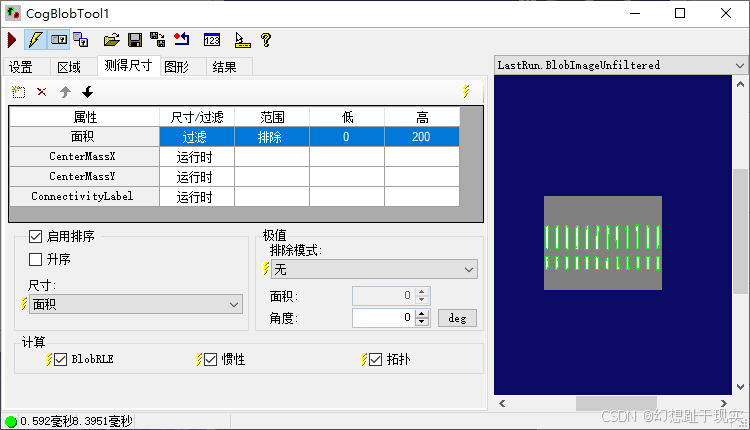
4.设置角度,排除多余的
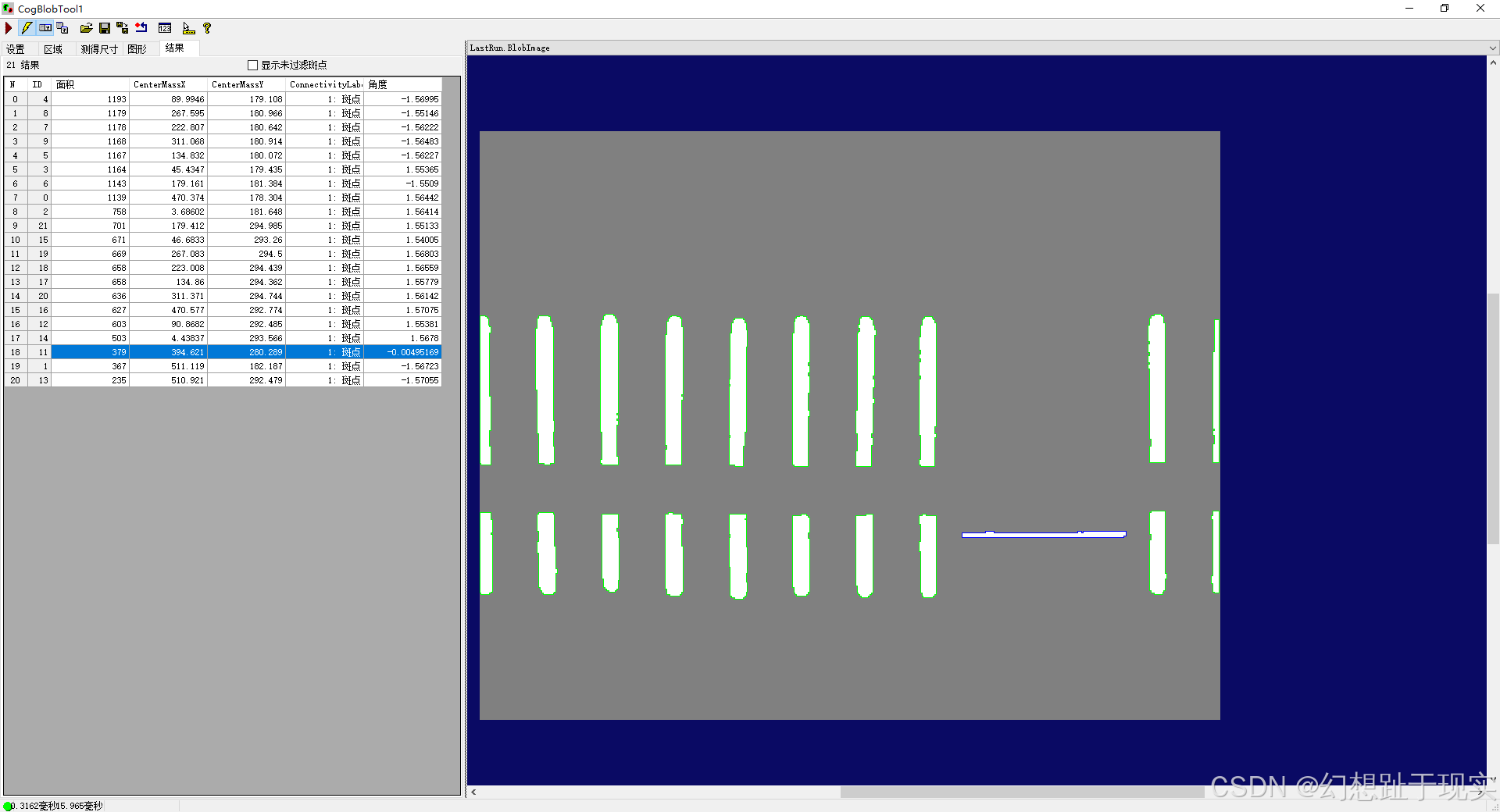
5.角度大部分在-1.5~1.5之间,所以我们要排除在 -0.1~0.2 之间
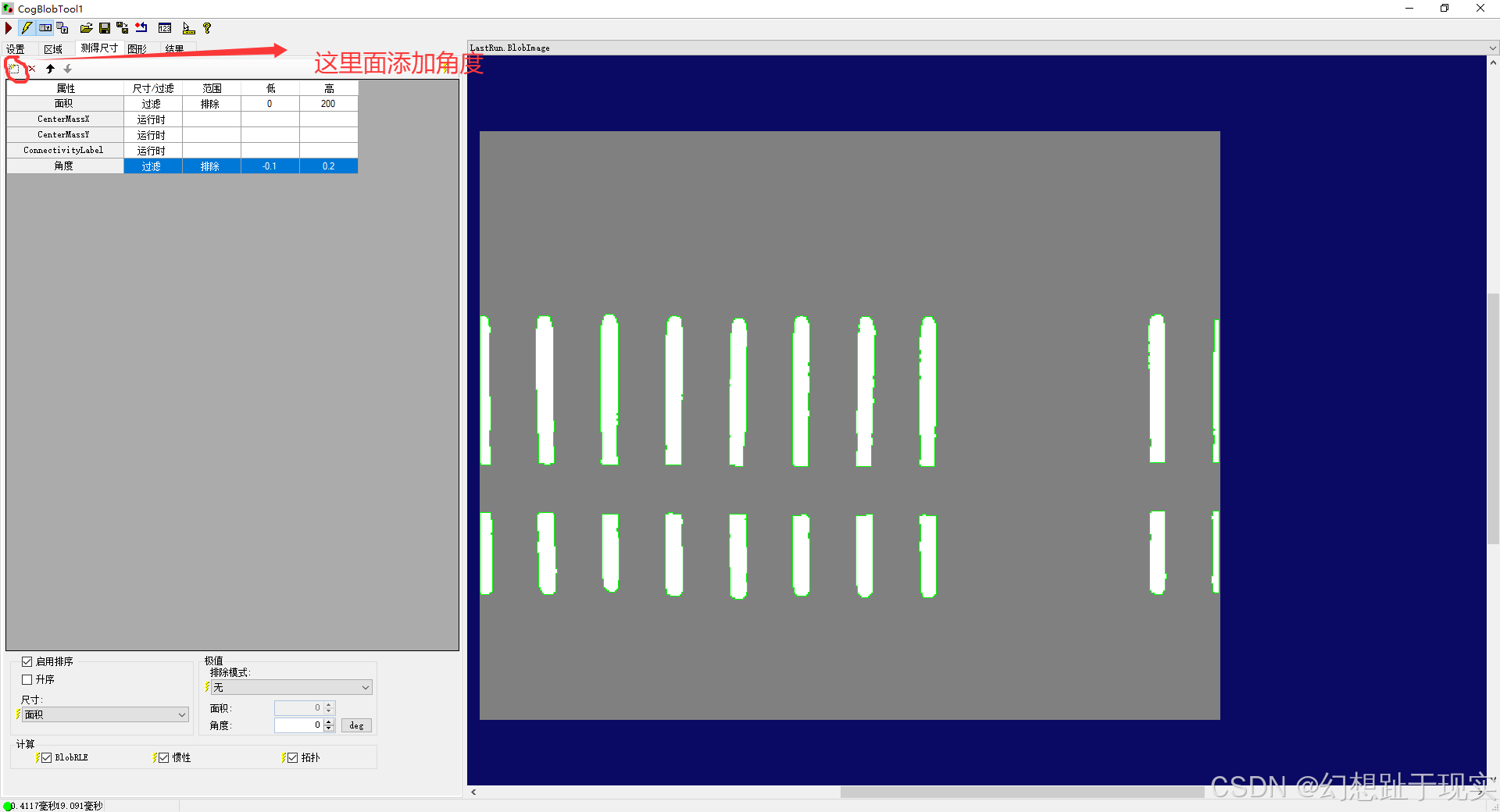
6.添加结果集
7.添加链接卡尺工具
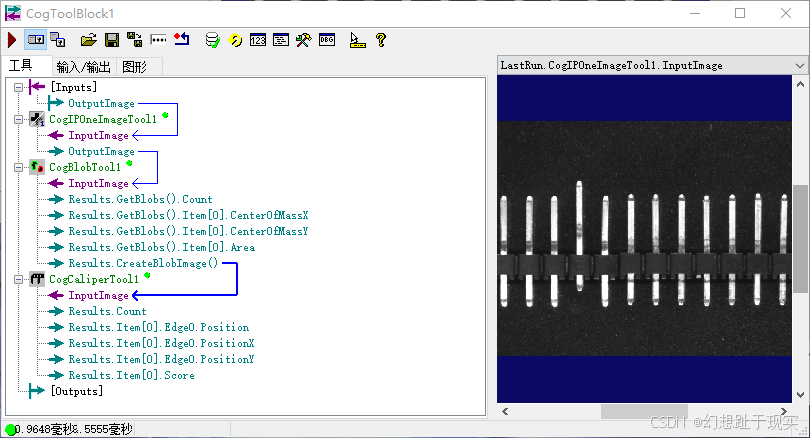
8. 设置卡尺参数
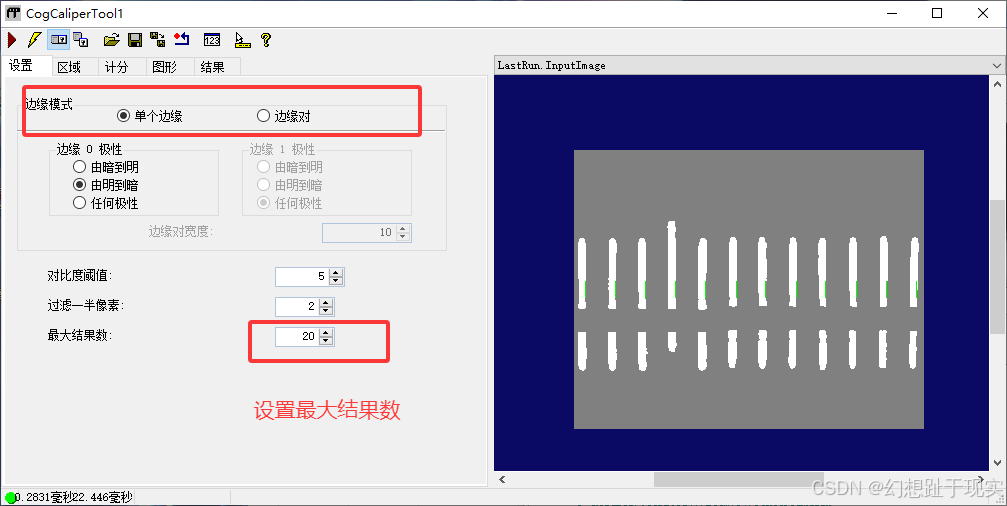
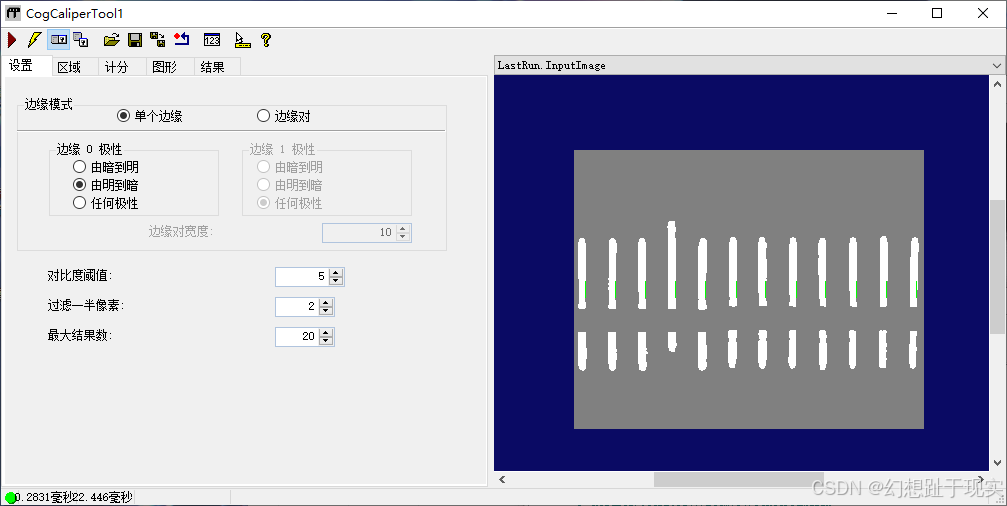
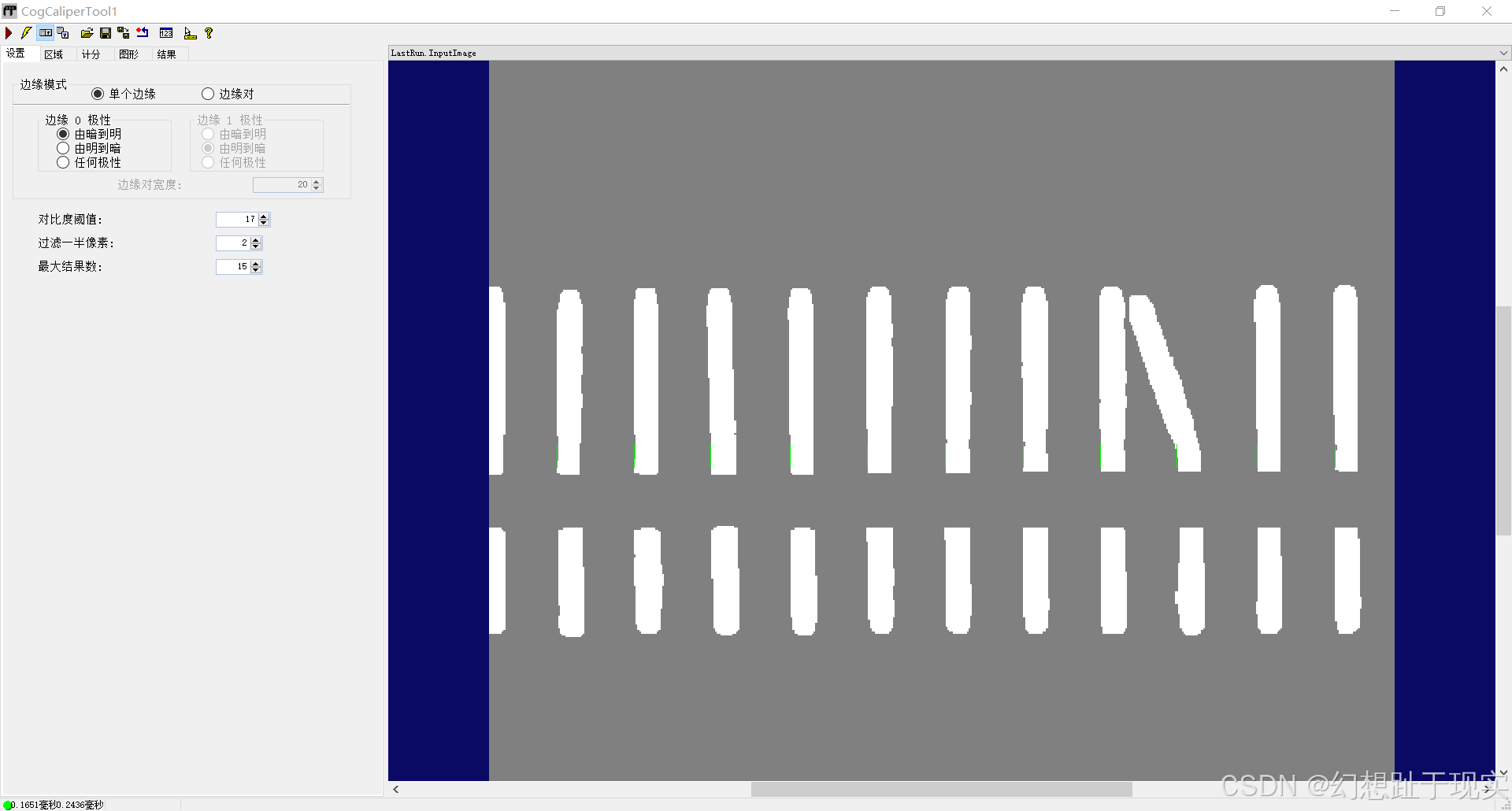
分析
- 引脚数量问题(前、中、后)
- 引脚角度问题(上引脚和下引脚有木有歪斜)
- 引脚的长度问题(或长或短)
- 文字显示图片
1.工具
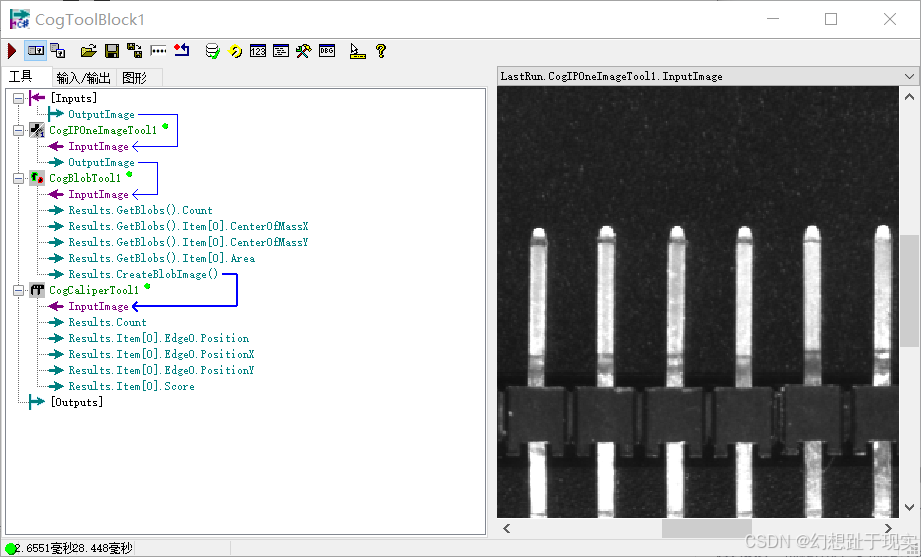
2.声明集合
cs
CogGraphicCollection dt = new CogGraphicCollection();
3.清空集合,声明文章工具
cs
CogBlobTool blob = mToolBlock.Tools["CogBlobTool1"]as CogBlobTool;
CogCaliperTool caliper = mToolBlock.Tools["CogCaliperTool1"]as CogCaliperTool;
dt.Clear();
4.声明List集合
主要用来接收卡尺工具的结果集
注意: using System.Collections.Generic; 引入这个集合
cs
List<CogCaliperResult> list = new List<CogCaliperResult>();
5.声明布尔类型变脸
主要为后续OK和不良进行变量筛选
cs
bool sum = true;
6.判断引脚的长度和角度
cs
//声明遍历blob工具的集合
foreach(CogBlobResult s in blob.Results.GetBlobs())
{
//声明一个矩形
CogRectangleAffine res = new CogRectangleAffine();
// GetBoundingBox:边界框,通常用于获取某个图像特征或对象的边界框
// CogBlobAxisConstants 是一个枚举类型 Principal 可能表示"主轴"或"主要方向"
// CogBlobAxisConstants.Principal 这是一个参数,指定了边界框的计算方式
res = s.GetBoundingBox(CogBlobAxisConstants.Principal);
//进行弧度转角度 angle/180*π
double angle = Math.Abs(CogMisc.RadToDeg(s.Angle));
// 判断这个角度是否大于2 文章中最大也是1.5多不会超过2
if(Math.Abs(angle - 90) > 2)
{
dt.Add(res);
sum = false; //更改变量
}
// GetMeasure 用来获取Blob特征的方法 CogBlobMeasureConstants 枚举值
// CogBlobMeasureConstants.BoundingBoxExtremaAngleHeight: 这是一个枚举值 表示"边界框的极值角度高度";这种测量方式考虑了便捷框的方向,不仅仅是水平或垂直方向的高度
double h = s.GetMeasure(CogBlobMeasureConstants.BoundingBoxExtremaAngleHeight);
// 判断Y上半轴
if(s.CenterOfMassY < 250)
{
if(h < 100 || h > 130)
{
res.Color = CogColorConstants.Red;
dt.Add(res);
sum = false;
}
}
// 下半轴
else{
if(h < 50 || h > 65)
{
res.Color = CogColorConstants.Red;
sum = false;
dt.Add(res);
}
}
}
7.添加卡尺工具数量
cs
for(int i = 0;i < caliper.Results.Count;i++)
{
list.Add(caliper.Results[i]);
}
8.排序(升序)
cs
list.Sort((m, n) => {return m.PositionX.CompareTo(n.PositionX);});
9.判断引脚的最开始是否有空缺
cs
if(list[0].PositionX > 50)
{
CogRectangleAffine res = new CogRectangleAffine();
res.CenterX = list[0].PositionX / 2;
res.CenterY = list[0].PositionY;
res.SideXLength = list[0].PositionX - 10;
res.SideYLength = 200;
res.Color = CogColorConstants.Red;
dt.Add(res);
sum = false;
}
10. 遍历寻找缺失部分
cs
//循环遍历list集合
for(int i = 0;i < list.Count - 1;i++)
{
double sn = list[i + 1].PositionX - list[i].PositionX;
//判断引脚之间的间距是否超过该值
if(sn > 80)
{
CogRectangleAffine res = new CogRectangleAffine();
res.CenterX = (list[i + 1].PositionX + list[i].PositionX) / 2;
res.CenterY = list[i].PositionY;
res.SideXLength = sn - 10;
res.SideYLength = 200;
res.Color = CogColorConstants.Red;
dt.Add(res);
sum = false;
}
}
//声明文本
CogGraphicLabel Label = new CogGraphicLabel();
Label.Font=new Font("楷体",20);
//判断sum
if(sum)
{
Label.SetXYText(100, 100, "OK");
Label.Color = CogColorConstants.Green;
}
else{
Label.SetXYText(100, 100, "NG");
Label.Color = CogColorConstants.Red;
}
dt.Add(Label);
11.循环遍历显示到文中
cs
foreach(ICogGraphic n in dt)
{
mToolBlock.AddGraphicToRunRecord(n, lastRecord, "CogIPOneImageTool1.InputImage", "");
}
12.全部代码
cs
#region namespace imports
using System;
using System.Collections;
using System.Collections.Generic;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
using Cognex.VisionPro;
using Cognex.VisionPro.ToolBlock;
using Cognex.VisionPro3D;
using Cognex.VisionPro.ImageProcessing;
using Cognex.VisionPro.Blob;
using Cognex.VisionPro.Caliper;
#endregion
public class CogToolBlockAdvancedScript : CogToolBlockAdvancedScriptBase
{
#region Private Member Variables
private Cognex.VisionPro.ToolBlock.CogToolBlock mToolBlock;
#endregion
CogGraphicCollection dt = new CogGraphicCollection();
/// <summary>
/// Called when the parent tool is run.
/// Add code here to customize or replace the normal run behavior.
/// </summary>
/// <param name="message">Sets the Message in the tool's RunStatus.</param>
/// <param name="result">Sets the Result in the tool's RunStatus</param>
/// <returns>True if the tool should run normally,
/// False if GroupRun customizes run behavior</returns>
public override bool GroupRun(ref string message, ref CogToolResultConstants result)
{
// To let the execution stop in this script when a debugger is attached, uncomment the following lines.
// #if DEBUG
// if (System.Diagnostics.Debugger.IsAttached) System.Diagnostics.Debugger.Break();
// #endif
CogBlobTool blob = mToolBlock.Tools["CogBlobTool1"]as CogBlobTool;
CogCaliperTool caliper = mToolBlock.Tools["CogCaliperTool1"]as CogCaliperTool;
dt.Clear();
List<CogCaliperResult> list = new List<CogCaliperResult>();
// Run each tool using the RunTool function
bool sum = true;
foreach(ICogTool tool in mToolBlock.Tools)
mToolBlock.RunTool(tool, ref message, ref result);
foreach(CogBlobResult s in blob.Results.GetBlobs())
{
CogRectangleAffine res = new CogRectangleAffine();
res = s.GetBoundingBox(CogBlobAxisConstants.Principal);
double angle = Math.Abs(CogMisc.RadToDeg(s.Angle));
if(Math.Abs(angle - 90) > 2)
{
dt.Add(res);
sum = false;
}
double h = s.GetMeasure(CogBlobMeasureConstants.BoundingBoxExtremaAngleHeight);
if(s.CenterOfMassY < 250)
{
if(h < 100 || h > 130)
{
res.Color = CogColorConstants.Red;
dt.Add(res);
sum = false;
}
}
else{
if(h < 50 || h > 65)
{
res.Color = CogColorConstants.Red;
sum = false;
dt.Add(res);
}
}
}
for(int i = 0;i < caliper.Results.Count;i++)
{
list.Add(caliper.Results[i]);
}
list.Sort((m, n) => {return m.PositionX.CompareTo(n.PositionX);});
if(list[0].PositionX > 50)
{
CogRectangleAffine res = new CogRectangleAffine();
res.CenterX = list[0].PositionX / 2;
res.CenterY = list[0].PositionY;
res.SideXLength = list[0].PositionX - 10;
res.SideYLength = 200;
res.Color = CogColorConstants.Red;
dt.Add(res);
sum = false;
}
for(int i = 0;i < list.Count - 1;i++)
{
double sn = list[i + 1].PositionX - list[i].PositionX;
if(sn > 80)
{
CogRectangleAffine res = new CogRectangleAffine();
res.CenterX = (list[i + 1].PositionX + list[i].PositionX) / 2;
res.CenterY = list[i].PositionY;
res.SideXLength = sn - 10;
res.SideYLength = 200;
res.Color = CogColorConstants.Red;
dt.Add(res);
sum = false;
}
}
CogGraphicLabel Label = new CogGraphicLabel();
Label.Font=new Font("楷体",20);
if(sum)
{
Label.SetXYText(100, 100, "OK");
Label.Color = CogColorConstants.Green;
}
else{
Label.SetXYText(100, 100, "NG");
Label.Color = CogColorConstants.Red;
}
dt.Add(Label);
return false;
}
#region When the Current Run Record is Created
/// <summary>
/// Called when the current record may have changed and is being reconstructed
/// </summary>
/// <param name="currentRecord">
/// The new currentRecord is available to be initialized or customized.</param>
public override void ModifyCurrentRunRecord(Cognex.VisionPro.ICogRecord currentRecord)
{
}
#endregion
#region When the Last Run Record is Created
/// <summary>
/// Called when the last run record may have changed and is being reconstructed
/// </summary>
/// <param name="lastRecord">
/// The new last run record is available to be initialized or customized.</param>
public override void ModifyLastRunRecord(Cognex.VisionPro.ICogRecord lastRecord)
{
foreach(ICogGraphic n in dt)
{
mToolBlock.AddGraphicToRunRecord(n, lastRecord, "CogIPOneImageTool1.InputImage", "");
}
}
#endregion
#region When the Script is Initialized
/// <summary>
/// Perform any initialization required by your script here
/// </summary>
/// <param name="host">The host tool</param>
public override void Initialize(Cognex.VisionPro.ToolGroup.CogToolGroup host)
{
// DO NOT REMOVE - Call the base class implementation first - DO NOT REMOVE
base.Initialize(host);
// Store a local copy of the script host
this.mToolBlock = ((Cognex.VisionPro.ToolBlock.CogToolBlock)(host));
}
#endregion
}