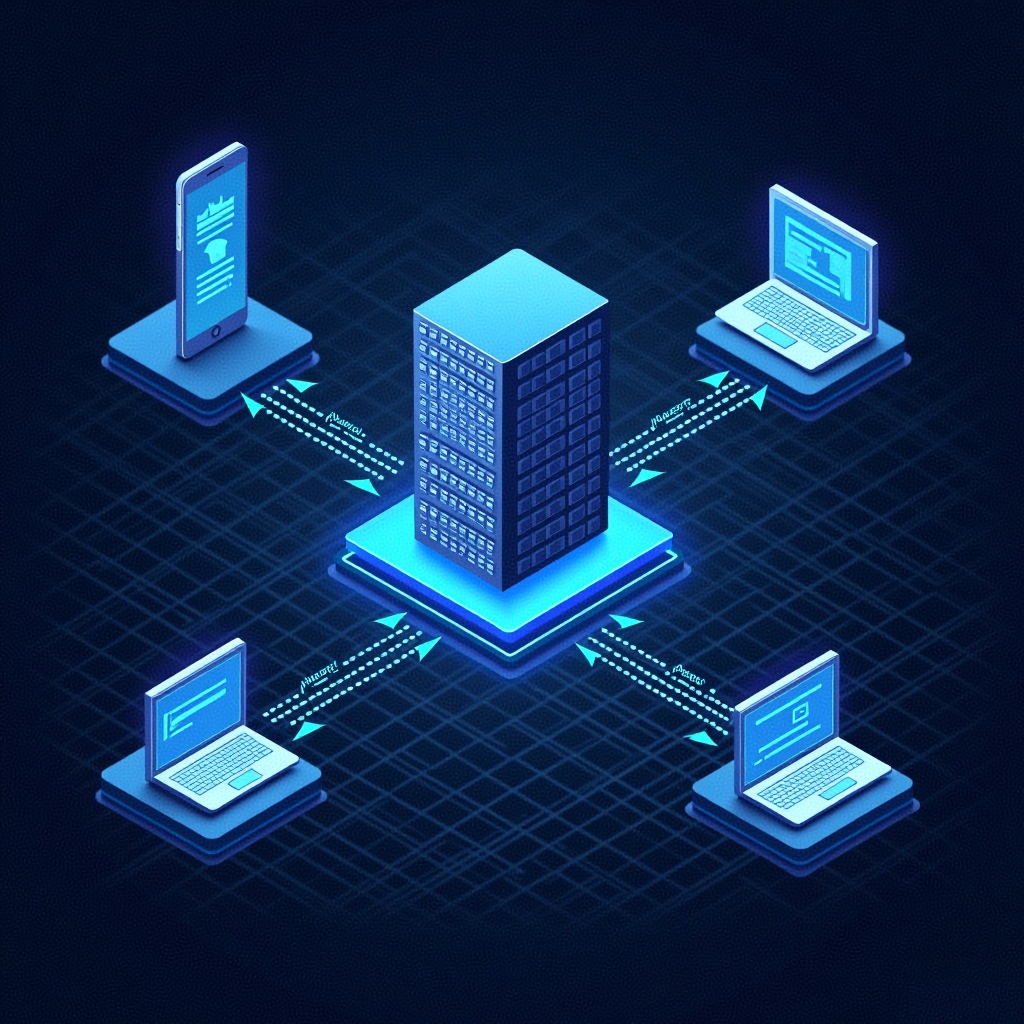
在现代 Web 开发中,HTTP 请求是前端和后端交互的核心。无论是从服务器获取数据、提交表单,还是与第三方 API 交互,HTTP 请求都扮演着至关重要的角色。Superagent 是一个轻量级且功能强大的 JavaScript 库,专门用于处理 HTTP 请求。它支持浏览器和 Node.js 环境,提供了简洁的 API 和强大的功能,能够轻松应对复杂的 HTTP 场景。
一、Superagent 简介
superagent
是一个轻量级的 HTTP 请求库,支持 Node.js 和浏览器环境。它提供了简洁的链式调用语法,使得发送 HTTP 请求变得非常直观。以下是 superagent
的一些主要特点:
- 简洁的 API:支持链式调用,代码可读性强。
- 强大的功能:支持 GET、POST、PUT、DELETE 等多种 HTTP 方法。
- 异步支持:原生支持异步操作,方便与现代 JavaScript 的
async/await
语法结合。 - 可扩展性:通过插件机制可以轻松扩展功能。
- 跨平台:同时支持浏览器和 Node.js 环境。
二、异步请求的实现
在 JavaScript 中,异步编程是处理 HTTP 请求的常见方式。superagent
原生支持 Promise
,并且可以与 async/await
结合使用,使得异步请求的代码更加简洁易读。
以下是一个使用 superagent
发送异步 GET 请求的示例:
TypeScript复制
typescript
import superagent from 'superagent';
async function fetchData(url: string) {
try {
const response = await superagent.get(url);
console.log('请求成功:', response.text);
return response;
} catch (error) {
console.error('请求失败:', error);
throw error;
}
}
// 调用函数
fetchData('https://api.example.com/data');
在上述代码中,superagent.get(url)
发起一个 GET 请求,并返回一个 Promise
。通过 await
关键字,我们可以等待请求完成并获取响应。如果请求失败,catch
块会捕获错误并进行处理。
三、错误处理
在处理 HTTP 请求时,错误处理是一个重要的环节。网络请求可能会因为多种原因失败,例如网络问题、服务器错误或请求超时。superagent
提供了强大的错误处理机制,可以通过 catch
方法或 try...catch
块来捕获错误。
以下是一个完整的错误处理示例:
TypeScript复制
typescript
import superagent from 'superagent';
async function fetchData(url: string) {
try {
const response = await superagent.get(url);
console.log('请求成功:', response.text);
return response;
} catch (error) {
if (error.response) {
// 服务器返回了响应,但状态码不是 2xx
console.error('服务器返回错误:', error.response.status, error.response.text);
} else if (error.request) {
// 请求已发出,但没有收到响应
console.error('请求超时或网络问题:', error.request);
} else {
// 请求未发出,可能是 URL 错误或配置问题
console.error('请求配置错误:', error.message);
}
throw error;
}
}
// 调用函数
fetchData('https://api.example.com/data');
在上述代码中,我们通过 error.response
检查服务器是否返回了响应,通过 error.request
检查请求是否发出但未收到响应,通过 error.message
检查其他错误原因。这种详细的错误处理机制可以帮助开发者快速定位问题。
四、并发控制
在某些场景下,我们需要同时发送多个 HTTP 请求,并等待所有请求完成后再进行后续操作。superagent
支持并发请求,可以通过 Promise.all
或 async/await
的组合来实现。
以下是一个并发请求的示例:
TypeScript复制
typescript
import superagent from 'superagent';
async function fetchMultipleData(urls: string[]) {
try {
const requests = urls.map(url => superagent.get(url));
const responses = await Promise.all(requests);
responses.forEach((response, index) => {
console.log(`请求 ${urls[index]} 成功:`, response.text);
});
return responses;
} catch (error) {
console.error('并发请求失败:', error);
throw error;
}
}
// 调用函数
fetchMultipleData(['https://api.example.com/data1', 'https://api.example.com/data2']);
在上述代码中,urls.map(url => superagent.get(url))
创建了一个包含多个请求的数组,Promise.all
用于并发执行这些请求,并等待所有请求完成。如果任何一个请求失败,Promise.all
会抛出错误。
五、代理设置
在某些情况下,我们可能需要通过代理服务器发送 HTTP 请求,例如在爬虫或跨域请求中。superagent
支持代理设置,可以通过 agent
方法创建一个代理实例。
以下是一个使用代理服务器的示例:
TypeScript复制
typescript
import superagent from 'superagent';
const proxyHost = 'ip.16yun.cn';
const proxyPort = 31111;
const agent = superagent.agent({
proxy: {
host: proxyHost,
port: proxyPort,
},
});
async function fetchDataWithProxy(url: string) {
try {
const response = await agent.get(url).set('User-Agent', 'Mozilla/5.0');
console.log('请求成功:', response.text);
return response;
} catch (error) {
console.error('请求失败:', error);
throw error;
}
}
// 调用函数
fetchDataWithProxy('https://www.example.com');
在上述代码中,superagent.agent({ proxy: { host, port } })
创建了一个代理实例,所有通过该实例发送的请求都会经过指定的代理服务器。
六、请求头的自定义
在发送 HTTP 请求时,自定义请求头是一个常见的需求。例如,我们可能需要设置 User-Agent
、Authorization
或其他自定义头。superagent
提供了 .set()
方法,用于设置请求头。
以下是一个自定义请求头的示例:
TypeScript复制
typescript
import superagent from 'superagent';
async function fetchDataWithHeaders(url: string) {
try {
const response = await superagent.get(url)
.set('User-Agent', 'Mozilla/5.0')
.set('Authorization', 'Bearer YOUR_ACCESS_TOKEN');
console.log('请求成功:', response.text);
return response;
} catch (error) {
console.error('请求失败:', error);
throw error;
}
}
// 调用函数
fetchDataWithHeaders('https://api.example.com/data');
在上述代码中,.set('User-Agent', 'Mozilla/5.0')
和 .set('Authorization', 'Bearer YOUR_ACCESS_TOKEN')
分别设置了用户代理和授权头。
七、超时设置
在某些场景下,我们可能需要为 HTTP 请求设置超时时间。superagent
提供了 .timeout()
方法,用于设置请求的超时时间。
以下是一个超时设置的示例:
TypeScript复制
typescript
import superagent from 'superagent';
async function fetchDataWithTimeout(url: string, timeout: number) {
try {
const response = await superagent.get(url).timeout(timeout);
console.log('请求成功:', response.text);
return response;
} catch (error) {
if (error.cause.code === 'ETIMEDOUT') {
console.error('请求超时:', error);
} else {
console.error('请求失败:', error);
}
throw error;
}
}
// 调用函数
fetchDataWithTimeout('https://api.example.com/data', 5000); // 超时时间设置为 5000 毫秒
在上述代码中,.timeout(timeout)
设置了请求的超时时间。如果请求在指定时间内未完成,superagent
会抛出一个超时错误。
八、文件上传
在某些场景下,我们需要通过 HTTP 请求上传文件。superagent
提供了强大的文件上传功能,支持单文件和多文件上传。
以下是一个文件上传的示例:
TypeScript复制
typescript
import superagent from 'superagent';
import fs from 'fs';
async function uploadFile(url: string, filePath: string) {
try {
const fileStream = fs.createReadStream(filePath);
const response = await superagent.post(url)
.attach('file', fileStream, filePath);
console.log('文件上传成功:', response.text);
return response;
} catch (error) {
console.error('文件上传失败:', error);
throw error;
}
}
// 调用函数
uploadFile('https://api.example.com/upload', './example.txt');
在上述代码中,.attach('file', fileStream, filePath)
将文件附加到请求中,并设置文件字段名为 file
。
九、请求拦截与响应拦截
在某些场景下,我们可能需要对请求或响应进行全局处理,例如添加日志、修改请求头或处理响应数据。superagent
提供了拦截器机制,可以通过 .use()
方法实现。
以下是一个请求拦截和响应拦截的示例:
TypeScript复制
typescript
import superagent from 'superagent';
// 请求拦截器
superagent.Request.prototype.use((req, next) => {
console.log('请求拦截:', req.url);
next();
});
// 响应拦截器
superagent.Response.prototype.use((res, next) => {
console.log('响应拦截:', res.status);
next();
});
async function fetchDataWithInterceptors(url: string) {
try {
const response = await superagent.get(url);
console.log('请求成功:', response.text);
return response;
} catch (error) {
console.error('请求失败:', error);
throw error;
}
}
// 调用函数
fetchDataWithInterceptors('https://api.example.com/data');
在上述代码中,superagent.Request.prototype.use
和 superagent.Response.prototype.use
分别实现了请求拦截和响应拦截。
十、总结
superagent
是一个功能强大且易于使用的 HTTP 请求库,支持异步操作、错误处理、并发控制、代理设置、请求头自定义、文件上传等多种复杂场景。通过合理使用 superagent
的功能,开发者可以高效地处理复杂的 HTTP 请求,提升开发效率和代码质量。
在实际开发中,superagent
可以与 Node.js 或浏览器环境无缝结合,适用于各种 HTTP 请求相关的场景。无论是简单的数据获取,还是复杂的 API 调用,superagent
都是一个值得信赖的选择。
希望本文的介绍和示例代码能够帮助你更好地理解和使用 superagent
,在处理复杂的 HTTP 请求时更加得心应手。