目录
[第一种 for+break](#第一种 for+break)
[第二种 for+exit](#第二种 for+exit)
[第三种 使用标志变量,可读性比较好的方法](#第三种 使用标志变量,可读性比较好的方法)
[第四种 标志变量+ do-while 可读性还要好](#第四种 标志变量+ do-while 可读性还要好)
韩信有一对兵,他想知道有多少人,便让士兵排队报数。按从1至5报数,最末一个士兵报的数为1;按1至6报数,最末一个士兵报的数为5;按从1至7报数,最末一个士兵报的数为4;最后按1至11报数,最末一个士兵报的10。请编程计算韩信至少有多少兵。
思路:
求余数。假定韩信有x个兵,那么x需要满足
x%5==1 && x%6==5 && x%7==4 &&x %11 ==10
第一种 for+break
cs
#include <stdio.h>
int main()
{
int x;
for (x=1;;x++)
{
if (x%5==1&& x%6==5 && x%7==4 && x%11 ==10)
{
printf("一共有%d个兵",x);
break; //终止循环
}
}
}
第二种 for+exit
cs
#include <stdio.h>
#include <stdlib.h> //新增头文件
int main()
{
int x;
for (x=1;;x++)
{
if (x%5==1&& x%6==5 && x%7==4 && x%11 ==10)
{
printf("一共有%d个兵",x);
exit(0); //直接结束程序运行
}
}
}
第三种 使用标志变量,可读性比较好的方法
cs
#include<stdio.h>
int main()
{
int find=0; //定义标志变量find,find=0未找到
int i,x;
for (x=1;find==0;x++)
{
if (x%5==1 && x%6==5 && x%7==4 &&x %11 ==10)
{
printf("一共有%d个兵",x);
find=1; //找到了,改成1,后面就进不了for循环
}
}
}
其中 for (x=1;find==0;x++)可以替换成 for (x=1;!find;x++)
第四种 标志变量+ do-while 可读性还要好
cs
#include<stdio.h>
int main()
{
int x=0; //因为do while循环会对x加1,这里才写0
int find=0; //定义标志变量,0是没找到
do
{
x++;
find = (x%5==1 && x%6==5 && x%7==4 &&x %11 ==10);
}while (find==0); //没找到才会循环
printf("一共有%d个兵",x);
}
其中while 后面的find==0 可以替换成 !find
运行结果是一样的,如下
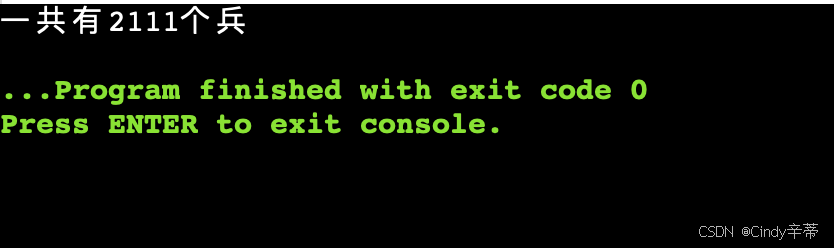