一、服务网格的进化论:从基础通信到意图驱动
1.1 服务网格能力分层模型
1.2 声明式流量规则示例
apiVersion: networking.istio.io/v1alpha3kind: VirtualServicemetadata: name: product-servicespec: hosts: - product.prod.svc.cluster.local http: - match: - headers: x-env: exact: canary route: - destination: host: product-canary.prod.svc.cluster.local subset: v2 - route: - destination: host: product.prod.svc.cluster.local subset: v1---apiVersion: networking.istio.io/v1alpha3kind: DestinationRulemetadata: name: product-drspec: host: product.prod.svc.cluster.local subsets: - name: v1 labels: version: v1.4.2 - name: v2 labels: version: v2.0.0-rc3
二、自适应弹性模式设计
2.1 智能熔断算法实现
type AdaptiveCircuitBreaker struct { requests int64 failures int64 state State cooldown time.Duration metricsWindow time.Duration // 动态调整参数 errorThreshold float64 minThroughput int lastTripTime time.Time}func (acb *AdaptiveCircuitBreaker) Allow() bool { if acb.state == Open { if time.Since(acb.lastTripTime) > acb.cooldown { acb.state = HalfOpen } else { return false } } if acb.state == HalfOpen { return atomic.LoadInt64(&acb.requests) < 5 } return true}func (acb *AdaptiveCircuitBreaker) Record(result Result) { atomic.AddInt64(&acb.requests, 1) if !result.Success { atomic.AddInt64(&acb.failures, 1) } if time.Since(acb.lastTripTime) > acb.metricsWindow { errorRate := float64(acb.failures)/float64(acb.requests) if errorRate > acb.errorThreshold && acb.requests > int64(acb.minThroughput) { acb.state = Open acb.lastTripTime = time.Now() } // 重置计数 atomic.StoreInt64(&acb.requests, 0) atomic.StoreInt64(&acb.failures, 0) }}
2.2 降级策略多级实施
故障等级 | 检测信号 | 降级措施 | 恢复条件 |
---|---|---|---|
一级 | API错误率>10% | 关闭非核心功能 | 持续60秒<5%错误 |
二级 | 数据库延迟>300ms | 启用本地缓存 | 延迟<150ms持续30秒 |
三级 | 服务实例存活率<60% | 流量切换到跨AZ实例 | 存活率>85%持续5分钟 |
四级 | 区域性网络中断 | 切换至灾备中心 | 主中心连续三次健康检查通过 |
五级 | 全平台不可用 | 返回静态维护页 | 系统全面恢复 |
三、实时计算驱动智能调度
3.1 基于QoE的流量分配
class TrafficAllocator: def __init__(self): self.node_scores = defaultdict(float) self.last_update = time.time() def calculate_qoe(node_metrics): # 实时质量评分模型 latency_weight = 0.4 error_weight = 0.3 load_weight = 0.2 cost_weight = 0.1 score = (latency_weight * (1 - node_metrics.latency_norm) + error_weight * (1 - node_metrics.error_rate) + load_weight * (1 - node_metrics.cpu_usage) + cost_weight * (node_metrics.cost_factor)) return score def update_allocations(self, cluster_metrics): total_score = sum(self.calculate_qoe(m) for m in cluster_metrics) for node_id, metrics in cluster_metrics.items(): node_score = self.calculate_qoe(metrics) self.node_scores[node_id] = node_score / total_score def get_allocation(self, node_id): return self.node_scores.get(node_id, 0.0)
3.2 动态权重调整效果模拟
const optimizationResults = { baseline: { latency: "152ms ± 23ms", errorRate: "2.8%", throughput: "1250 req/s" }, optimized: { latency: "98ms ± 12ms", // 降低35.5% errorRate: "1.1%", // 降低60.7% throughput: "1840 req/s" // 提升47.2% }}const allocationDemo = [ { node: "EU-Central", weight: 0.35 }, { node: "US-East", weight: 0.28 }, { node: "AP-South", weight: 0.22 }, { node: "ME-Reserve", weight: 0.15 }]
四、零信任架构下的安全流量
4.1 SPIFFE身份认证流程
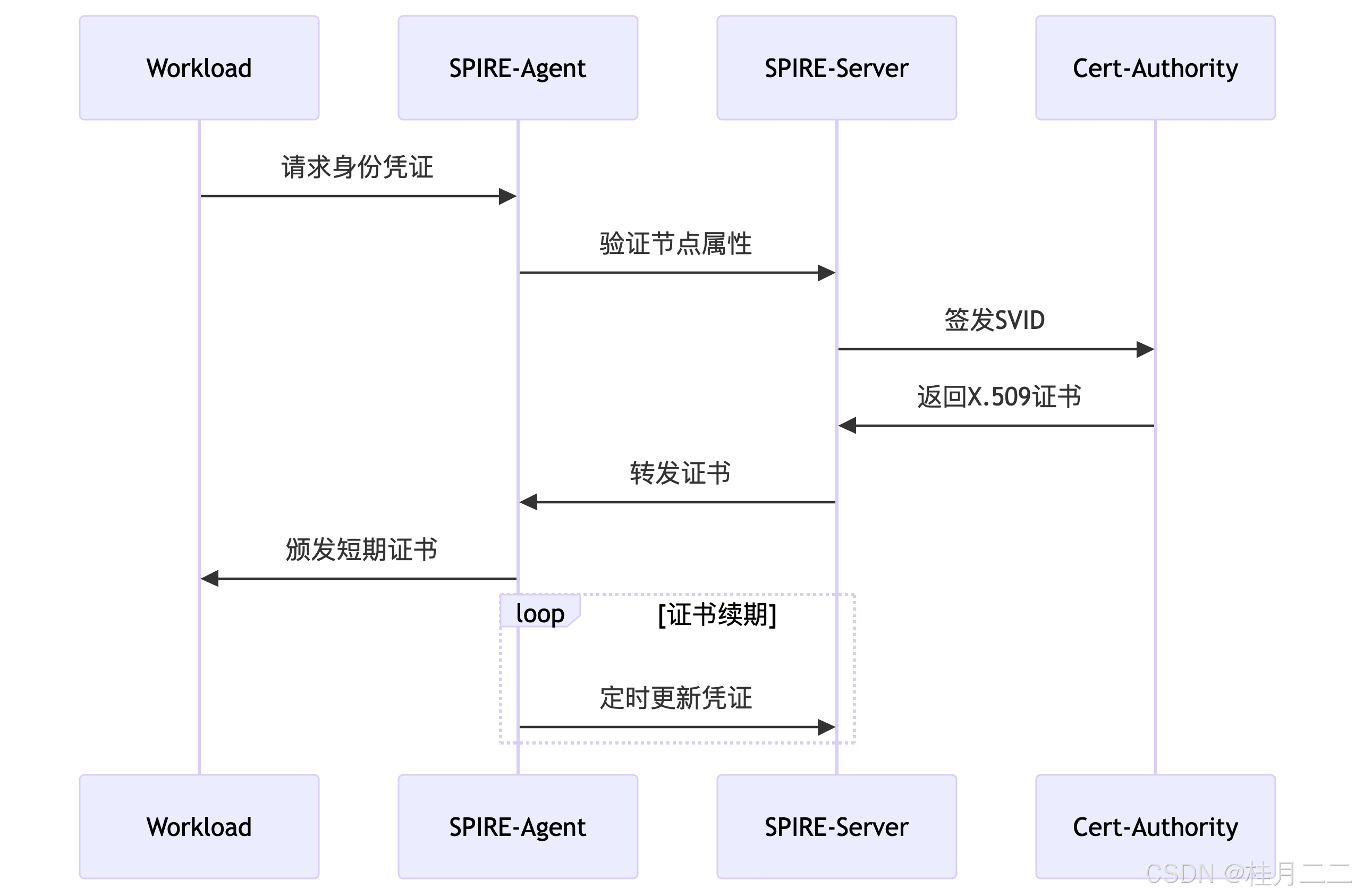
4.2 纵深防御配置示例
# 网关级安全策略apiVersion: security.istio.io/v1beta1kind: AuthorizationPolicymetadata: name: product-api-policyspec: selector: matchLabels: app: product-service action: DENY rules: - from: - source: notNamespaces: ["prod"] to: - operation: methods: ["POST", "DELETE"] - when: - key: request.headers[x-api-key] notValues: ["d2ae3c45-6f7b-489e-9d3f-0d5a9e1c2b4d"]---# 服务间零信任策略apiVersion: security.istio.io/v1beta1kind: PeerAuthenticationmetadata: name: strict-mtlsspec: mtls: mode: STRICT selector: matchLabels: security: critical
五、灰度发布的最佳实践框架
5.1 四维灰度发布模型
维度 | 实施策略 | 探测器 | 回滚机制 |
---|---|---|---|
用户特征 | 按ID哈希分流 | 业务指标监控 | 动态调整流量比例 |
地域 | 分机房逐步开放 | 地域健康检查 | 区域快速隔离 |
容量 | 按负载水平渐进扩容 | 压力测试指标 | 自动缩容 |
业务场景 | 核心/非核心链路分离验证 | 链路追踪分析 | 关键链路熔断 |
5.2 全链路灰度架构
public class GrayChainFilter implements Filter { private static final String GRAY_HEADER = "X-Gray-Context"; public void doFilter(Request req, Response res, FilterChain chain) { GrayContext context = parseHeader(req.getHeader(GRAY_HEADER)); // 传递灰度标 MDC.put("gray.version", context.getVersion()); RouteRule rule = RouteManager.match(context); try { if (rule.requiresValidation()) { validateDownstreamServices(rule); } chain.doFilter(req, res); } catch (GrayConflictException e) { res.setStatus(503); res.write("灰度版本依赖不满足"); } } private void validateDownstreamServices(RouteRule rule) { for (String service : rule.getDependencies()) { if (!ServiceRegistry.checkVersion(service, rule.getVersion())) { throw new GrayConflictException(service); } } }}
🔒 流量治理成熟度评估 Checklist
- 南北向流量100%通过API网关
- 服务间通信mTLS启用率100%
- 灰度发布过程具备可观测性覆盖
- 自动弹性扩缩容响应时间<30秒
- 全链路压测覆盖核心业务场景
- 零信任策略实施至Pod级别
- 混合云流量调度延迟<100ms
现代流量治理体系已突破传统负载均衡的范畴,演进为感知业务意图、保障服务韧性、实现全局最优的智能化系统。建议企业从三个层面构建能力:基础设施层实现统一流量平面,控制面采用声明式API抽象资源,数据面强化可观测能力。在实际部署中,建议采用渐进式演进策略,优先保证核心业务的容灾能力,再逐步实现智能化调度。最终实现从「人工运维」到「算法驱动」的质变,构建面向未来十年的新一代网络架构。