Django学习笔记
安装django
cmd
pip install django
创建APP
用django来写后端的时候,要把各个功能分散到各个创建好的APP去实现
在终端输入
cmd
python manage.py startapp app01(APP名称)
APP内部文件
admin.py django默认提供了admin后台管理
apps.py app启动类
models.py 对数据库进行操作
tests.py 单元测试
views.py 函数
模板和静态文件
模板
app下面创建templates来放模板
如果setting.py没有
python
'DIRS': [BASE_DIR / 'templates']
静态文件会根据app的注册顺序从各个app的templates目录中去找
如果有
会先从项目根目录下面找templates目录,根目录没有就根据app的注册顺序从各个app的templates目录中去找
静态文件
包括image,js,css,plugins
在app下创建static文件夹来存放静态文件
django特有语法
可以在html中
django
{% load static %}
引入static文件
html的路径引用可以是
<img src="{% static 'img/1.png' %}">
模板语法
python
def tpl(request):
name="Lee"
return render(request,'tpl.html',{"n1":name})
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>用户列表</h1>
<div>{{ n1 }}</div>
</body>
</html>
pymysql
mysql> create database unicom DEFAULT CHARSET utf8 COLLATE utf8_general_ci;
插入数据
mysql
create table admin(
id int not null auto_increment primary key,
username varchar(16) not null,
password varchar(64) not null,
mobile char(11) not null
)default charset=utf8;
python
import pymysql
conn=pymysql.connect(host="127.0.0.1",port=3306,user="root",charset="utf8",db='unicom')
cursor=conn.cursor(cursor=pymysql.cursors.DictCursor)
cursor.execute("insert into admin(username,password,mobile) values('yeke','2131','32423')")
conn.commit()
cursor.close()
conn.close()
使用占位符和元组
python
import pymysql
from pymysql import cursors
conn = pymysql.connect(host="127.0.0.1", port=3306, user="root", charset="utf8", db='unicom')
cursor = conn.cursor(cursor=pymysql.cursors.DictCursor)
sql="insert into admin(username,password,mobile) values(%s,%s,%s)"
cursor.execute(sql,['yeke','1231','21321'])
conn.commit()
cursor.close()
conn.close()
起名
python
import pymysql
from pymysql import cursors
conn = pymysql.connect(host="127.0.0.1", port=3306, user="root", charset="utf8", db='unicom')
cursor = conn.cursor(cursor=pymysql.cursors.DictCursor)
sql="insert into admin(username,password,mobile) values(%(n1)s,%(n2)s,%(n3)s)"
cursor.execute(sql,{"n1":'yeke',"n2":'1231',"n3":'21321'})
conn.commit()
cursor.close()
conn.close()
加输入
python
import pymysql
from pymysql import cursors
while True:
user=input("用户名:")
passwd=input("密码:")
mobile=input("手机号:")
conn = pymysql.connect(host="127.0.0.1", port=3306, user="root", charset="utf8", db='unicom')
cursor = conn.cursor(cursor=pymysql.cursors.DictCursor)
sql="insert into admin(username,password,mobile) values(%s,%s,%s)"
cursor.execute(sql,[user,passwd,mobile])
conn.commit()
cursor.close()
conn.close()
查询数据
python
import pymysql
from pymysql import cursors
## 创建APP
conn = pymysql.connect(host="127.0.0.1", port=3306, user="root", charset="utf8", db='unicom')
cursor = conn.cursor(cursor=pymysql.cursors.DictCursor)
sql="select * from admin"
cursor.execute(sql)
datalist=cursor.fetchall()
print(datalist)
conn.commit()
cursor.close()
conn.close()
ORM框架
是建立在pymsql,mysqlclient上面的
不需要写sql语句就可以操作数据库
配置mysql
安装mysqlclient
cmd
pip install mysqlclient
连接mysql要修改setting.py中数据库的配置
django
DATABASES = {
"default": {
"ENGINE": "django.db.backends.mysql",
"NAME": "django",
"USER": "root",
"PASSWORD": "root",
"HOST": "127.0.0.1",
"PORT": 3306,
}
}
确保配置正确,就可以了
在APP下的models.py中可以创建类
python
from django.db import models
# Create your models here.
class UserInfo(models.Model):
name=models.CharField(max_length=32)
password=models.CharField(max_length=32)
age=models.IntegerField()
cmd
python mange.py makemigrations
python manage.py migrate
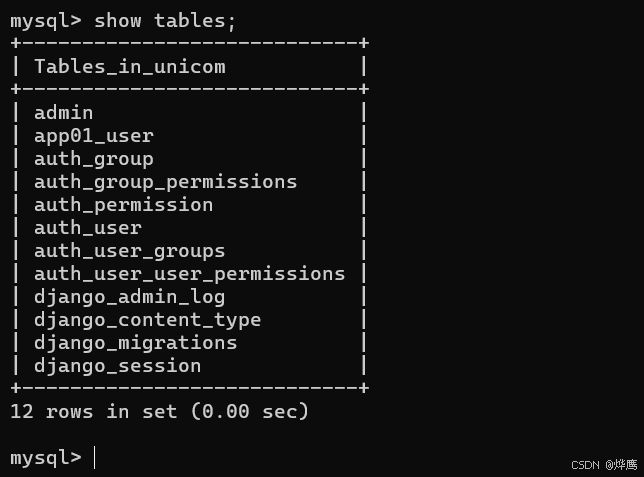