一、介绍
二、入门案例
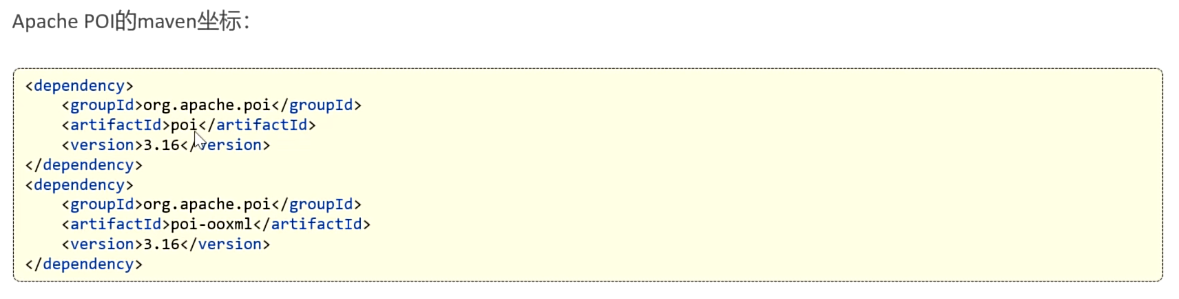
java
package com.sky.test;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
/*
* 使用POI操作Excel文件
* */
public class POITest {
public static void write() throws IOException {
//在内存中创建一个excel文件
XSSFWorkbook excel= new XSSFWorkbook();
//在excel文件中创建一个sheet页
XSSFSheet sheet=excel.createSheet("info");
//在Sheet中创建行对象,rownum编号从0开始
XSSFRow row=sheet.createRow(1);
//在行上创建单元格 索引从0开始 并设置单元格内容
row.createCell(1).setCellValue("姓名哈哈哈");
row.createCell(2).setCellValue("城市哈哈哈");
//创建一个新行 第三行
row=sheet.createRow(2);
row.createCell(1).setCellValue("张三");
row.createCell(2).setCellValue("伤害");
//创建一个新行 第四行
row=sheet.createRow(3);
row.createCell(1).setCellValue("李四");
row.createCell(2).setCellValue("南京");
//通过输出流 将内存中的excel文件写入到磁盘
FileOutputStream out = new FileOutputStream(new File("D:\\1、黑马程序员Java项目《苍穹外卖》企业级开发实战\\资料\\资料\\day12\\info.xlsx"));
excel.write(out);
out.close();
excel.close();
}
public static void main(String[] args) throws IOException {
write();
}
}
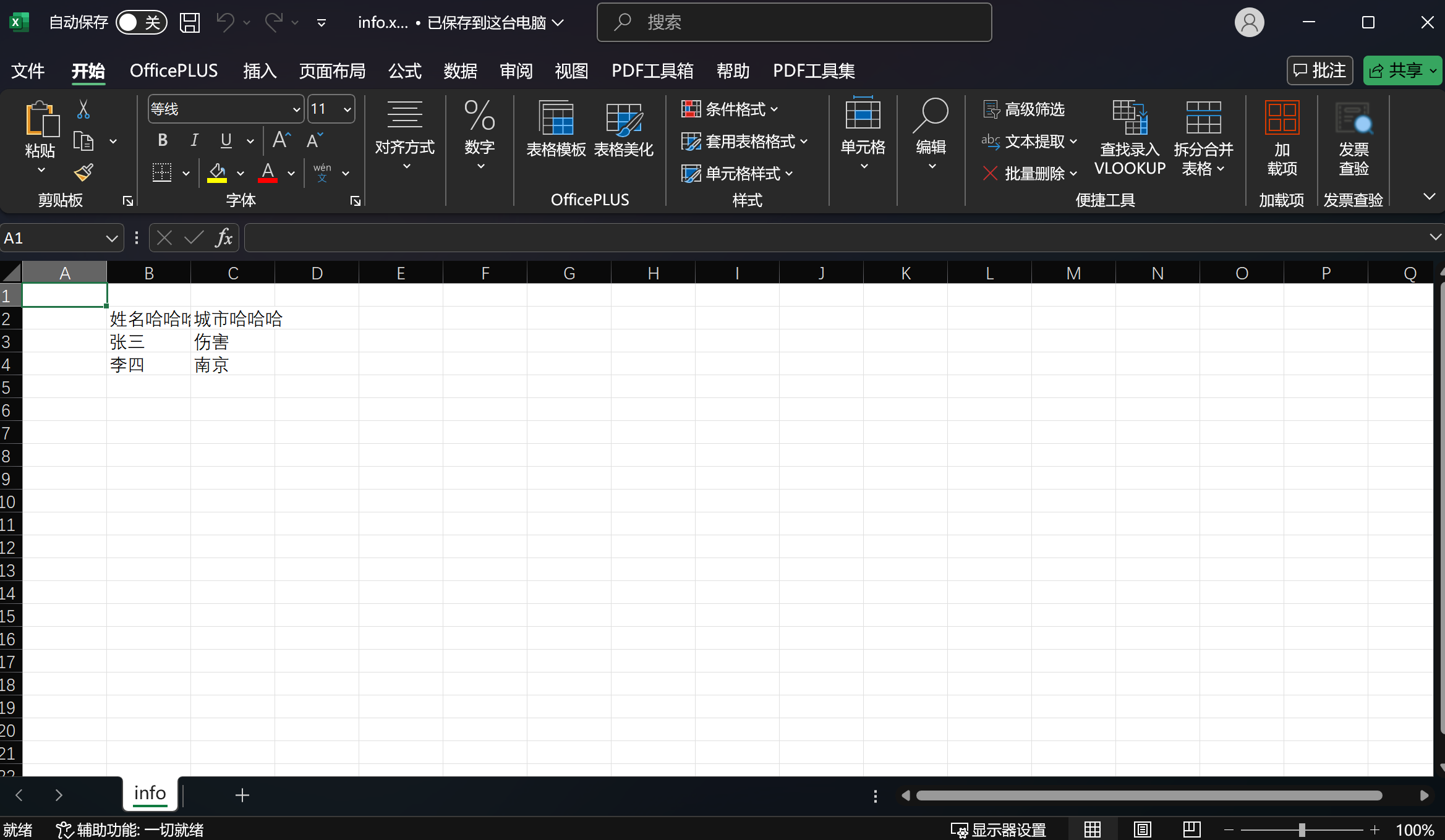
java
public static void read() throws IOException {
//创建输入流
FileInputStream fileInputStream = new FileInputStream(new File("D:\\1、黑马程序员Java项目《苍穹外卖》企业级开发实战\\资料\\资料\\day12\\info.xlsx"));
//读取磁盘上已经存在的excel文件
XSSFWorkbook excel = new XSSFWorkbook(fileInputStream);
//读取Excel文件中的第一个sheet页 索引从0开始
XSSFSheet sheet = excel.getSheetAt(0);
//获取sheet中最后一行的行号
int lastRowNum = sheet.getLastRowNum();
for(int i=1;i<=lastRowNum;i++){
//获得某一行
XSSFRow row = sheet.getRow(i);
//获得单元格对象中的文本内容
String stringCellValue = row.getCell(1).getStringCellValue();
String stringCellValue1 = row.getCell(2).getStringCellValue();
System.out.println(stringCellValue +" "+stringCellValue1);
}
excel.close();
fileInputStream.close();
}
public static void main(String[] args) throws IOException {
read();
}
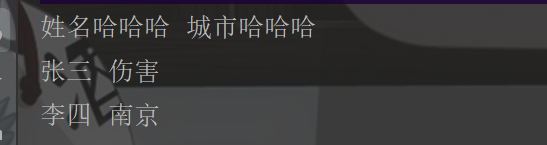
三、导出数据
需求分析和设计:
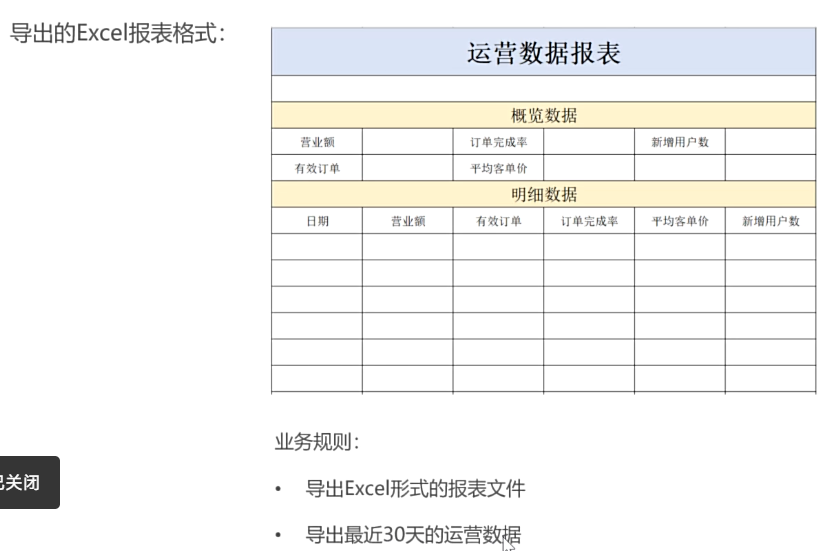
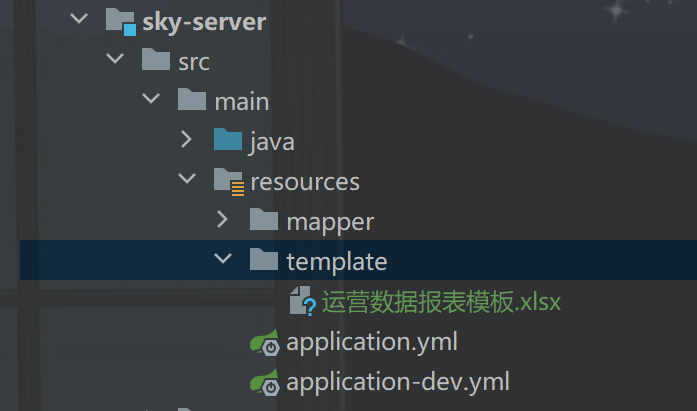
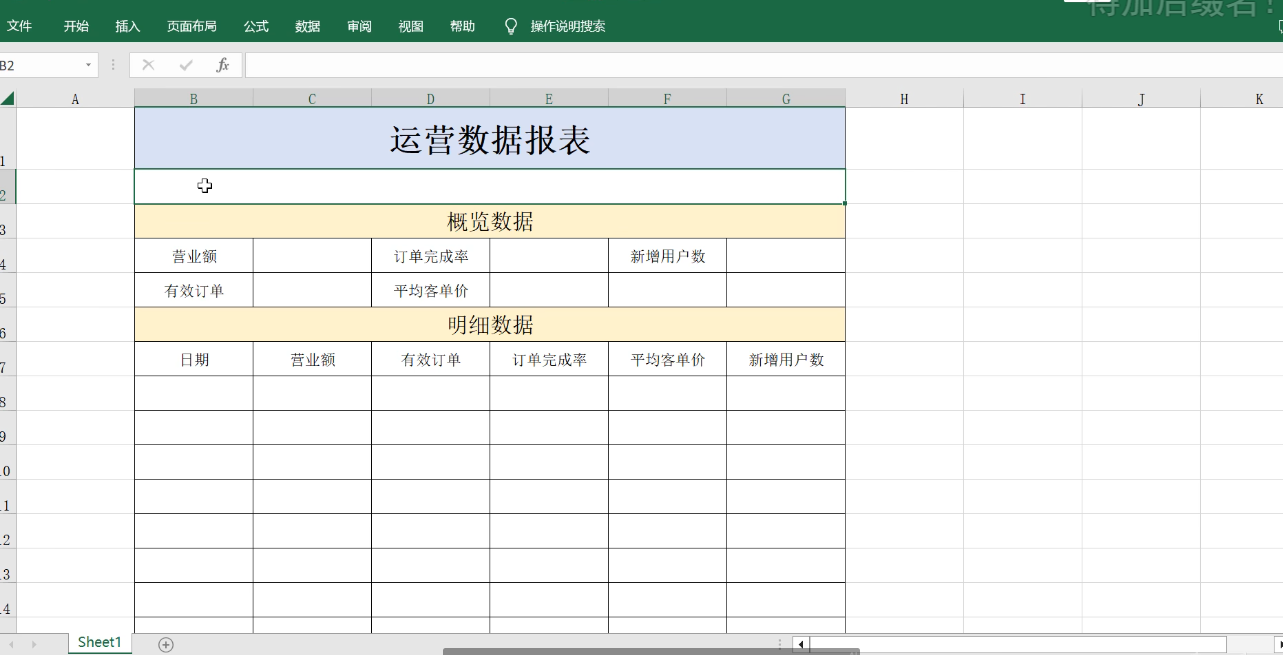
Controller:
java
/**
* 导出数据
* */
@GetMapping("/export")
@ApiOperation("导出运营数据Excel报表")
public void export(HttpServletResponse response) throws IOException {
log.info("导出数据..");
reportService.exportBussinessData(response);
}
Servcie:
java
/**
* 导出运营数据报表
* */
void exportBussinessData(HttpServletResponse response) throws IOException;
java
/**
* 导出运营数据报表
* */
@Override
public void exportBussinessData(HttpServletResponse response) throws IOException {
//查询数据库,获取营业数据
LocalDate dateBegin = LocalDate.now().minusDays(30);
LocalDate dateEnd = LocalDate.now().minusDays(1);
BusinessDataVO businessData = workspaceService.getBusinessData(
LocalDateTime.of(dateBegin, LocalTime.MIN),
LocalDateTime.of(dateEnd, LocalTime.MAX));
//将数据写入到excel文件 POI写入
InputStream input = this.getClass().getClassLoader().getResourceAsStream("template/运营数据报表模板.xlsx");
//基于一个模板文件创建一个新的Excel文件
try {
XSSFWorkbook excel=new XSSFWorkbook(input);
//填充数据
//按名字获取sheet页
XSSFSheet sheet = excel.getSheet("Sheet1");
//填充时间--获得第二行
sheet.getRow(1).getCell(1).setCellValue("时间: "+dateBegin+"至"+dateEnd);
sheet.getRow(3).getCell(2).setCellValue(businessData.getTurnover());
sheet.getRow(3).getCell(4).setCellValue(businessData.getOrderCompletionRate());
sheet.getRow(3).getCell(6).setCellValue(businessData.getNewUsers());
sheet.getRow(4).getCell(2).setCellValue(businessData.getValidOrderCount());
sheet.getRow(4).getCell(4).setCellValue(businessData.getUnitPrice());
//明细数据
for(int i=0;i<30;i++){
LocalDate localDate = dateBegin.plusDays(i);
BusinessDataVO businessData1 = workspaceService.getBusinessData(
LocalDateTime.of(dateBegin, LocalTime.MIN),
LocalDateTime.of(dateBegin, LocalTime.MAX));
sheet.getRow(7+i).getCell(1).setCellValue(localDate.toString());
sheet.getRow(7+i).getCell(2).setCellValue(businessData1.getTurnover());
sheet.getRow(7+i).getCell(3).setCellValue(businessData1.getValidOrderCount());
sheet.getRow(7+i).getCell(4).setCellValue(businessData1.getOrderCompletionRate());
sheet.getRow(7+i).getCell(5).setCellValue(businessData1.getUnitPrice());
sheet.getRow(7+i).getCell(6).setCellValue(businessData1.getNewUsers());
}
//通过输出流将excel文件导出到浏览器
ServletOutputStream outputStream = response.getOutputStream();
excel.write(outputStream);
//关闭资源
outputStream.close();
excel.close();
} catch (IOException e) {
e.printStackTrace();
}
}