*************
c++
topic: 1041. 困于环中的机器人 - 力扣(LeetCode)
*************
Inspect the topic first.
|----------------------------------------------------------------------------|
| |
And it looks really familiar with another robot topic.
|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| |
| 657. 机器人能否返回原点 - 力扣(LeetCode)https://leetcode.cn/problems/robot-return-to-origin/description/?envType=study-plan-v2&envId=programming-skills |
You gays have found what are the differences? That is direction.
Here is the code without considering the direction.
cpp
class Solution {
public:
bool isRobotBounded(string instructions) {
int x = 0;
int y = 0;
for (char c : instructions)
{
if (c == 'U') y++;
else if (c == 'D') y--;
else if (c == 'R') x++;
else if (c == 'L') x--;
}
return x == 0 && y == 0;
}
};
To see if there is any spark from the basic code to solve this problem? In the topic, the robot need to change the direction.
- L --> turn left
- R --> turn right
- G --> go
So what is to describe the direction? Maybe the coordinate axis system, like (x, y)? coordinate axis system is necessary. And besides that, need to add direction.
|--------------------|------|
| direction = 0, 向 ↑ | y++; |
| direction = 1, 向 → | x++; |
| direction = 2, 向 ↓ | y--; |
| direction = 3, 向 ← | x--; |
Good.
cpp
class Solution {
public:
bool isRobotBounded(string instructions) {
int x = 0, y = 0;
int dir = 0; // 0: North, 1: East, 2: South, 3: West
for (char c : instructions)
{
if (c == 'G')
{
if (dir == 0)
{
y++; // Move North
}
else if (dir == 1)
{
x++; // Move East
}
else if (dir == 2)
{
y--; // Move South
}
else if (dir == 3)
{
x--; // Move West
}
}
else if (c == 'L')
{
if (dir == 0)
{
dir = 3; // North -> West
}
else if (dir == 1)
{
dir = 0; // East -> North
}
else if (dir == 2)
{
dir = 1; // South -> East
} else if (dir == 3)
{
dir = 2; // West -> South
}
}
else if (c == 'R')
{
if (dir == 0)
{
dir = 1; // North -> East
}
else if (dir == 1)
{
dir = 2; // East -> South
}
else if (dir == 2)
{
dir = 3; // South -> West
} else if (dir == 3)
{
dir = 0; // West -> North
}
}
}
// Robot is in a circle if:
// 1. It returns to (0, 0), or
// 2. It's not facing North (dir != 0)
return (x == 0 && y == 0) || (dir != 0);
}
};
too many if.
The original position is dir = 0; (0, 0);
if turn left, dir = 3; (0, 0);
if continue turn left, dir = 2; (0, 0);
if continue turn left, dir = 1; (0, 0);
So you can see the pired of turns around is 4 steps. %4 maybe works.
L -> turn left -> dir - 1. but if dir == 0 and dir - 1 is negative, that unsatisfied. so add a period:
L -> turn left -> (dir - 1 + 4) % 4;
R -> turn right -> (dir + 1) % 4;
- Let's take an example.
- the position is (0, 0) and direction is 2(↓):
- if L:turn left, should be 1(↑), dir = ( 2 - 1 + 4) % 4 = 1;
- if R: turn right, should be 3(←), dir = ( 2 + 1) % 4 = 3;
so lets change a little code.
cpp
class Solution {
public:
bool isRobotBounded(string instructions) {
int x = 0;
int y = 0;
int dir = 0; // 0: North, 1: East, 2: South, 3: West
for (char c: instructions)
{
if (c == 'G')
{
if (dir == 0)
{
y++;
}
else if (dir == 1)
{
x++;
}
else if (dir == 2)
{
y--;
}
else if (dir == 3)
{
x--;
}
}
else if (c == 'L')
{
dir = (dir - 1 + 4) % 4;
}
else if (c == 'R')
{
dir = (dir + 1) % 4;
}
}
return (x == 0 && y == 0) || (dir != 0);
}
};
and the code here looks unreadable. So introoduce switch algorhythm in c++.
cpp
switch (expression) {
case value1:
// 当 expression == value1 时执行的代码
break;
case value2:
// 当 expression == value2 时执行的代码
break;
// 可以有任意数量的 case
default:
// 当 expression 不匹配任何 case 时执行的代码
}
for a little example:
cpp
#include <iostream>
using namespace std;
int main() {
int choice = 2;
switch (choice) {
case 1:
cout << "选择 1" << endl;
break;
case 2:
cout << "选择 2" << endl;
break;
case 3:
cout << "选择 3" << endl;
break;
default:
cout << "无效选择" << endl;
}
return 0;
}
//输出2
Then use switch in the program.
cpp
class Solution {
public:
bool isRobotBounded(string instructions) {
int x = 0;
int y = 0;
int dir = 0; // 0: North, 1: East, 2: South, 3: West
for (char c : instructions)
{
if (c == 'G')
{
switch (dir)
{
case 0:
y++;
break;
case 1:
x++;
break;
case 2:
y--;
break;
case 3:
x--;
break;
}
}
else if (c == 'L')
{
dir = (dir - 1 + 4) % 4;
}
else if (c == 'R')
{
dir = (dir + 1) % 4;
}
}
return (x == 0 && y == 0) || (dir != 0);
}
};
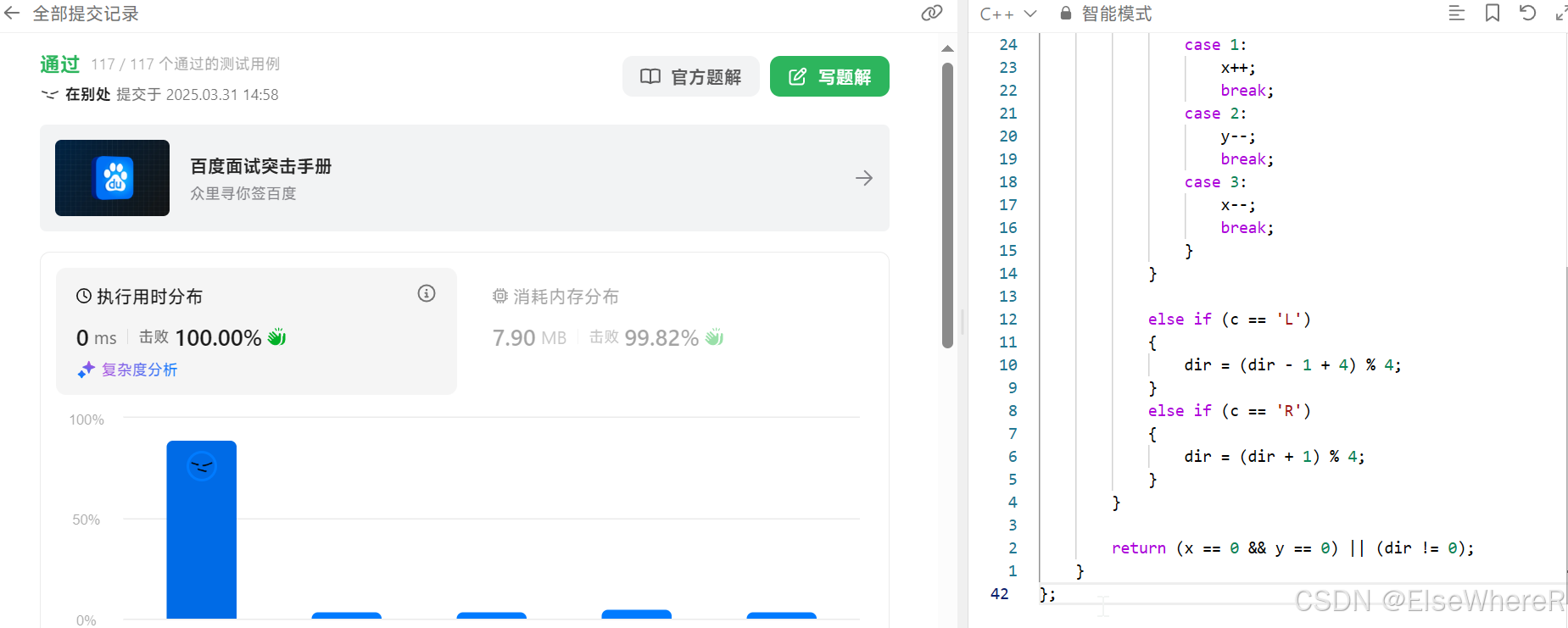